Common OAuth 2.0 Pitfalls in Spring Security Implementation
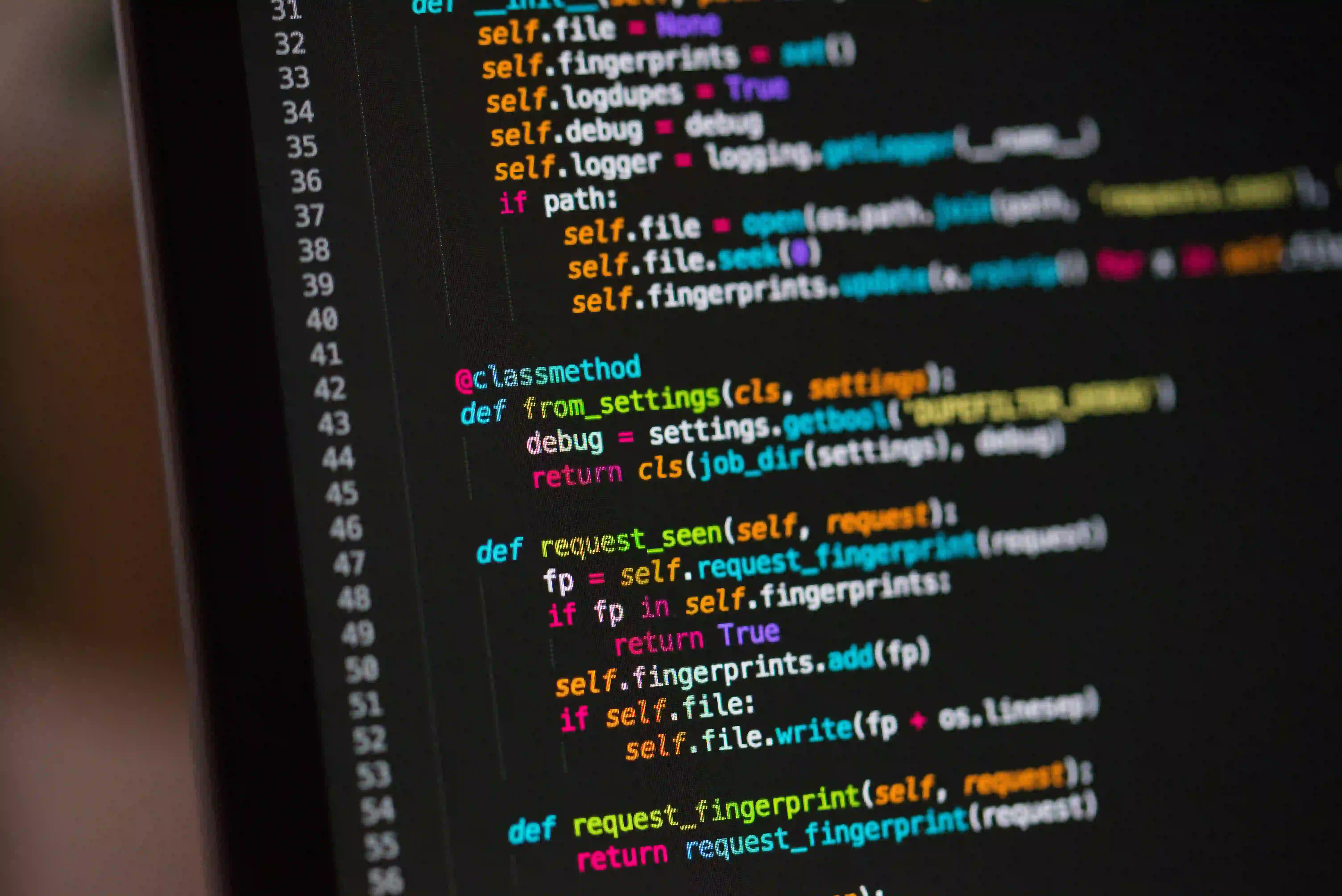
Common OAuth 2.0 Pitfalls in Spring Security Implementation
OAuth 2.0 is an industry-standard protocol used for authorization. One of its main advantages is that it allows third-party applications to obtain limited access to a web service on behalf of a user. Spring Security offers robust support for implementing OAuth 2.0, but there are several common pitfalls developers encounter. This blog aims to shed light on those pitfalls and provide insights into avoiding them effectively.
Understanding OAuth 2.0
Before diving deep into pitfalls, it's essential to understand what OAuth 2.0 is. It’s an authorization framework that enables applications to grant limited access to their resources without exposing user credentials. This is especially useful in modern web applications where resources may be spread across different domains.
Spring Security and OAuth 2.0
Spring Security’s OAuth 2.0 support provides a way to secure your REST APIs and web applications. However, following a few best practices can save you from common mistakes. Let’s explore some of these pitfalls.
1. Misunderstanding the Roles of OAuth 2.0
A critical pointer is understanding the different roles in the OAuth 2.0 framework:
- Resource Owner: The user who authorizes the application.
- Client: The application trying to access user resources.
- Authorization Server: The server that authenticates the resource owner and issues access tokens.
- Resource Server: The server that hosts the resources.
Why It Matters:
Misunderstanding these roles can lead to incorrect configurations. For example, defining the client as a resource owner can create security vulnerabilities.
Example Configuration
Here's how you would typically configure these roles in a Spring application.
@Configuration
@EnableAuthorizationServer
public class OAuth2AuthorizationServerConfig extends AuthorizationServerConfigurerAdapter {
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient("clientId")
.secret("clientSecret")
.authorizedGrantTypes("authorization_code")
.scopes("read", "write");
}
}
By clearly defining roles, you ensure your application adheres to OAuth 2.0 specifications.
2. Ignoring Proper Scopes
Scopes determine the level of access that is granted to client applications. A pitfall developers often fall into is using overly broad scopes.
Why It Matters:
Broad scopes can result in applications obtaining more data than necessary, increasing the likelihood of data leaks.
Example of Scopes
{
"clients": {
"clientId": {
"scopes": ["profile", "email"],
"resourceIds": ["api"]
}
}
}
In the example above, the client has two specific scopes. Always limit scopes to the minimum necessary.
3. Not Validating Redirect URIs
The redirect URI is where the user is sent after authorization. Not validating the redirect URI can lead to redirection attacks.
Why It Matters:
If attackers know the redirect URI, they can intercept the authorization code which may compromise user data.
Example Validation Method
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) {
endpoints
.authorizationCodeServices(authorizationCodeServices())
.redirectResolver(new DefaultRedirectResolver());
}
Always ensure that redirect URIs are whitelisted and validated against known values.
4. Failure to Secure Token Storage
The access token is a critical component in OAuth 2.0, and its storage is often overlooked.
Why It Matters:
If access tokens are stored insecurely, they can be intercepted or leaked, allowing unauthorized access to user data.
Secure Storage Example
Use secure storage methods like HTTP-only cookies or encrypted storage mechanisms.
http
.sessionManagement()
.sessionCreationPolicy(SessionCreationPolicy.STATELESS)
.and()
.csrf().disable()
.headers().frameOptions().sameOrigin();
Implementing security headers can protect tokens during storage and transmission.
5. Missing Token Revocation Mechanism
While OAuth 2.0 provides tokens, it also must provide a mechanism to revoke them.
Why It Matters:
If an access token is compromised, the lack of a revocation mechanism can leave user data vulnerable.
Example of Revocation Endpoint
Spring can be configured to revoke tokens as follows:
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) {
endpoints
.tokenStore(tokenStore())
.reuseRefreshTokens(false) // Disable refreshing to revoke on logout.
;
}
Implementing a revocation endpoint is key to ensuring that tokens can be invalidated immediately upon user logout or when a session is expired.
6. Not Handling Token Expiration Gracefully
Tokens in OAuth 2.0 have expiration times. Beyond this time frame, a token must be refreshed or reissued.
Why It Matters:
Ignoring token expiration can disrupt user experiences, leading to failures when accessing resources.
Token Refresh Example
Spring allows refreshing tokens easily:
@Override
public void configure(AuthorizationServerEndpointsConfigurer endpoints) {
endpoints
.tokenStore(tokenStore())
.reuseRefreshTokens(true); // Allow token reuse.
}
Always implement a refresh token strategy to enhance user experience.
7. Lack of Monitoring and Logging
Security breaches can happen despite the best implementations. Therefore, monitoring user activities and logging important events are crucial.
Why It Matters:
Timely detection is essential in mitigating potential risks before they escalate into serious issues.
Example Logging Configuration
Using AOP (Aspect-Oriented Programming) for logging can streamline monitoring:
@Aspect
public class LoggingAspect {
@Before("execution(* com.yourpackage..*.*(..))")
public void logBefore(JoinPoint joinPoint) {
// Log method entry with details
}
}
Logging helps in auditing and tracking access patterns.
The Last Word
Implementing OAuth 2.0 with Spring Security can be a rewarding endeavor, providing a robust framework for secure authorization. However, understanding and avoiding common pitfalls is critical. Properly defining roles, validating redirect URIs, securing token storage, handling token expiration, and maintaining logging are essential for building a secure application.
By recognizing the importance of these aspects, you can enhance your application’s security significantly, protecting both user data and your service.
For further reading, you may refer to the official Spring Security documentation and the OAuth 2.0 RFC 6749. Implementing these best practices will help you create a secure and reliable OAuth 2.0 solution in your Spring applications.