Common Challenges in Implementing Redis with Java
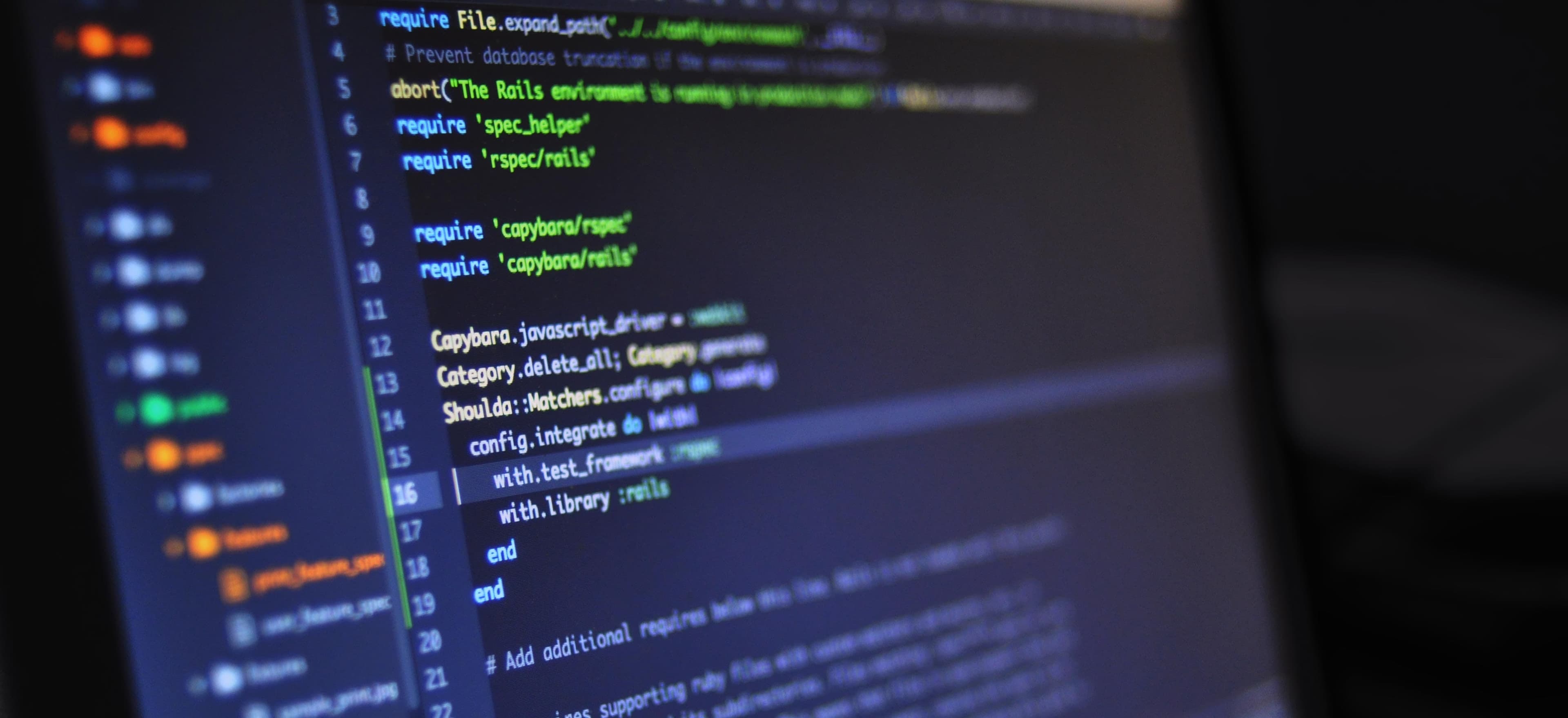
- Published on
Common Challenges in Implementing Redis with Java
Redis, an in-memory data structure store, is celebrated for its speed, flexibility, and performance. It's widely used for caching, real-time analytics, and session storage. When integrating Redis into a Java application, developers often face several common challenges. In this blog post, we'll discuss these challenges in depth, provide code snippets to illustrate solutions, and discuss best practices.
Table of Contents
- Challenge 1: Configuration and Connection Management
- Challenge 2: Data Serialization
- Challenge 3: Handling Failures and Latency
- Challenge 4: Understanding Redis Data Types
- Challenge 5: Performance Tuning
- Conclusion
Challenge 1: Configuration and Connection Management
The first challenge lies in configuring the Redis connection correctly. Using the right libraries can simplify this process. A popular choice for Java applications is the Jedis library. To begin, you need to set up your Redis connection.
Here’s a simple example:
import redis.clients.jedis.Jedis;
public class RedisConnection {
private Jedis jedis;
public RedisConnection(String host, int port) {
jedis = new Jedis(host, port);
}
public Jedis getJedis() {
return jedis;
}
public void close() {
if (jedis != null) {
jedis.close();
}
}
}
public class Main {
public static void main(String[] args) {
RedisConnection redisConnection = new RedisConnection("localhost", 6379);
Jedis jedis = redisConnection.getJedis();
// Use redis operations here
redisConnection.close(); // Close connection to avoid leaks
}
}
Why it matters: Proper configuration ensures reduced latency and maximizes the performance of your application. It's essential to close connections to prevent resource leaks.
Challenge 2: Data Serialization
Serialization is crucial when dealing with complex data structures. Redis primarily stores data in string format, which means that if you're working with objects, you need to convert your objects into a format Redis understands.
Using libraries like Jackson or Gson can facilitate this process:
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.ObjectMapper;
public class RedisDataSerializer {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static String serialize(Object obj) throws JsonProcessingException {
return objectMapper.writeValueAsString(obj);
}
public static <T> T deserialize(String json, Class<T> valueType) throws JsonProcessingException {
return objectMapper.readValue(json, valueType);
}
}
Why it matters: Efficient serialization and deserialization enhance application performance. The right choice of libraries can significantly affect your application's efficiency and runtime.
Challenge 3: Handling Failures and Latency
Network operations can be unpredictable. With Redis, connection failures or high latency can lead to performance bottlenecks. Implementing retry mechanisms or fallbacks can ensure your application remains robust.
Here's an example of a basic retry mechanism:
public void safeRedisOperation(Runnable operation) {
int attempts = 0;
while (attempts < 3) {
try {
operation.run();
return; // Success, exit the method
} catch (Exception e) {
attempts++;
System.out.println("Retry attempt: " + attempts);
if (attempts >= 3) throw e; // Rethrow after 3 attempts
}
}
}
Why it matters: A retry mechanism can improve the resilience of your application. Rather than facing a total failure, it allows your application to recover from transient issues seamlessly.
Challenge 4: Understanding Redis Data Types
Another challenge is understanding the various data types Redis provides: strings, hashes, lists, sets, and sorted sets. Each type serves different use cases and has unique methods for manipulation.
For example, to manage a user session with a hash:
public void setUserSession(String userId, User user) throws JsonProcessingException {
jedis.hset("session:" + userId, "data", RedisDataSerializer.serialize(user));
}
public User getUserSession(String userId) throws JsonProcessingException {
String userJson = jedis.hget("session:" + userId, "data");
return RedisDataSerializer.deserialize(userJson, User.class);
}
Why it matters: Understanding when to use each Redis data structure can significantly improve your application's efficiency. Using the right data types enables you to optimize read and write operations.
Challenge 5: Performance Tuning
Lastly, the challenge of performance tuning should not be overlooked. Common configurations like memory limits, eviction policies, and persistence strategies can drastically affect your Redis performance.
Here’s an example of setting up a max memory policy in Redis configuration:
# In redis.conf
maxmemory 256mb
maxmemory-policy allkeys-lru
Why it matters: Tuning Redis for your workload can ensure that it performs optimally. By understanding your application's specific needs, you can configure Redis to avoid unnecessary memory consumption and improve speed.
The Closing Argument
Integrating Redis with Java comes with its own set of challenges, from configuration and serialization to handling failures and performance tuning. By understanding these challenges and implementing the discussed strategies, developers can build efficient, high-performing applications.
For a more in-depth look into Redis and its usage, you can also explore the official Redis documentation here.
By addressing these common challenges, you can leverage Redis's power effectively, ensuring your Java applications run smoothly and efficiently.
Remember, knowledge of the tools and their best practices will help you overcome these hurdles and make the most out of Redis. Happy coding!
Checkout our other articles