Troubleshooting Common Java & RabbitMQ Integration Issues
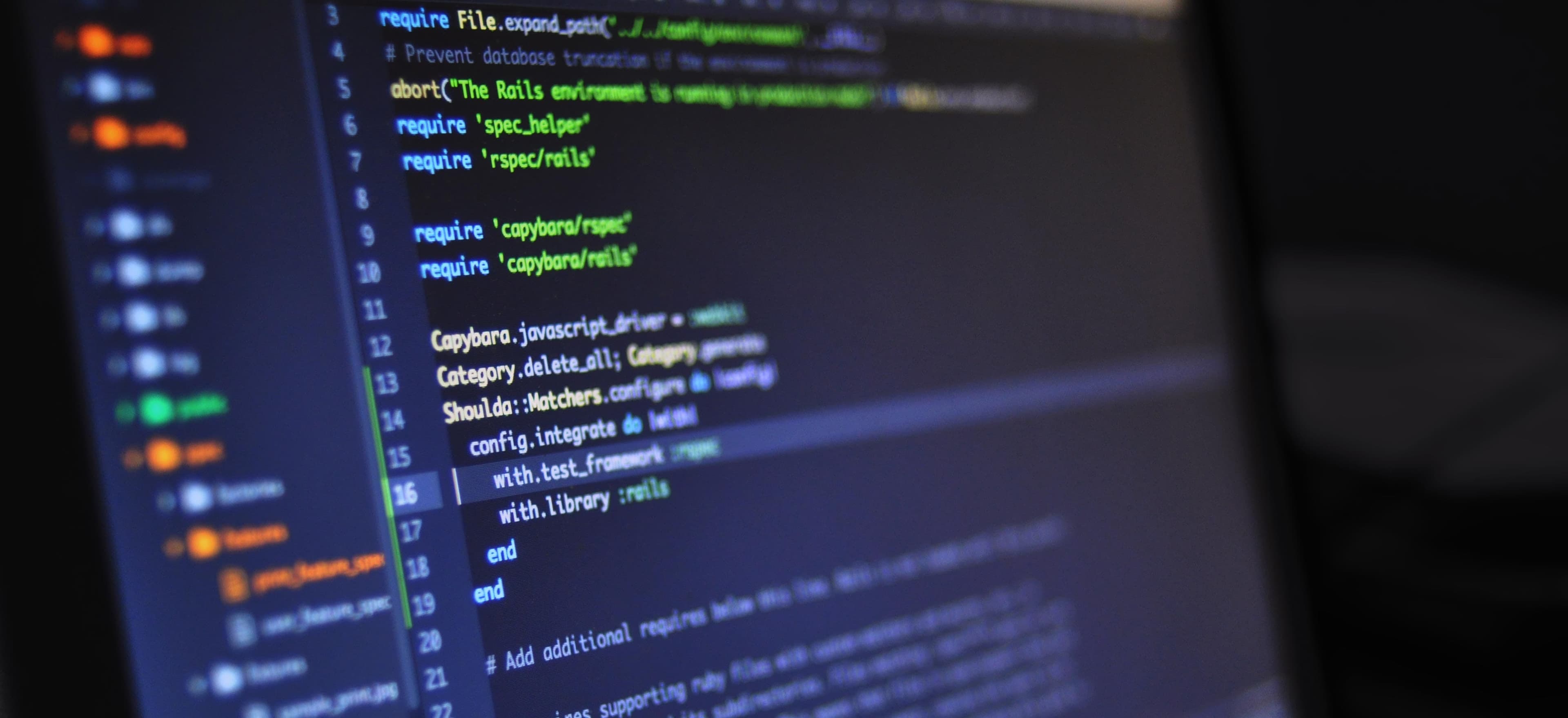
- Published on
Troubleshooting Common Java & RabbitMQ Integration Issues
As applications become more complex, the need for reliable message queuing solutions like RabbitMQ becomes increasingly apparent. Integrating RabbitMQ with Java can streamline application architecture and improve performance. However, it can also present challenges. This blog post aims to address common integration issues between Java applications and RabbitMQ, provide troubleshooting techniques, and offer code snippets that illustrate effective error handling and logging.
Understanding RabbitMQ
RabbitMQ is an open-source message broker that allows applications to communicate through a common message bus. It follows the Advanced Message Queuing Protocol (AMQP) and operates on the principle of sending messages between producers (the applications that send messages) and consumers (the applications that receive them).
For a comprehensive understanding of RabbitMQ, check out the official RabbitMQ documentation.
Common Issues in Java and RabbitMQ Integration
1. Connection Problems
One of the most frequent issues encountered when integrating Java applications with RabbitMQ is connection-related problems. This may happen due to various reasons such as incorrect credentials, unreachable RabbitMQ server, or firewall restrictions.
Solution: Validate Connection Parameters
ConnectionFactory factory = new ConnectionFactory();
factory.setHost("localhost");
factory.setPort(5672); // Default RabbitMQ port
factory.setUsername("guest");
factory.setPassword("guest");
try (Connection connection = factory.newConnection()) {
System.out.println("Connection established.");
} catch (IOException | TimeoutException e) {
System.err.println("Failed to establish connection: " + e.getMessage());
e.printStackTrace();
}
Why: This code snippet demonstrates how to establish a connection to RabbitMQ. Always try to handle exceptions gracefully to diagnose where the connection fails. Ensure the host, port, username, and password match those configured in your RabbitMQ server.
2. Queue Not Found
When a consumer attempts to listen to a queue that does not exist, you will encounter a "queue not found" error. This problem can arise if the queue was not declared or if it was deleted.
Solution: Declare Queue Before Use
try (Connection connection = factory.newConnection();
Channel channel = connection.createChannel()) {
String queueName = "myQueue";
channel.queueDeclare(queueName, true, false, false, null);
System.out.println("Queue declared successfully.");
} catch (IOException | TimeoutException e) {
System.err.println("Queue declaration failed: " + e.getMessage());
e.printStackTrace();
}
Why: This snippet showcases how to declare a queue properly. Queues should generally be declared in the code before sending or consuming messages to avoid runtime errors.
3. Message Format Issues
Incompatible message formats can lead to deserialization problems when consumers try to read messages produced by the producers.
Solution: Use JSON for Serialization
import com.fasterxml.jackson.databind.ObjectMapper;
public class MessageProducer {
private static final ObjectMapper objectMapper = new ObjectMapper();
public static void sendMessage(Channel channel, String queueName, Object message) throws IOException {
String jsonMessage = objectMapper.writeValueAsString(message);
channel.basicPublish("", queueName, null, jsonMessage.getBytes());
System.out.println("Sent message: " + jsonMessage);
}
}
Why: Utilizing JSON for message formatting provides a common format that can be easily parsed in various languages. The ObjectMapper
from the Jackson library simplifies the conversion process.
4. Message Acknowledgment Issues
RabbitMQ provides mechanisms for acknowledging message receipt. Improper acknowledgment may cause messages to be re-delivered or lost entirely.
Solution: Implement Acknowledgments
public class MessageConsumer {
public void consumeMessages(Channel channel, String queueName) throws IOException {
DeliverCallback deliverCallback = (consumerTag, delivery) -> {
String message = new String(delivery.getBody(), StandardCharsets.UTF_8);
System.out.println("Received message: " + message);
// Acknowledge message
channel.basicAck(delivery.getEnvelope().getDeliveryTag(), false);
};
channel.basicConsume(queueName, false, deliverCallback, consumerTag -> {});
}
}
Why: This example demonstrates how to acknowledge messages after they have been processed. Using manual acknowledgment (false
in basicConsume
), we ensure messages are only removed from the queue once processed successfully.
5. Flow Control Issues
Both RabbitMQ and Java applications can use flow control features to manage message delivery rates. Uncontrolled message production can overwhelm consumers if not properly handled.
Solution: Apply Rate Limiting
public void sendMessageWithRateLimit(Channel channel, String queueName, Object message) throws IOException {
// Implement a simple rate limiter with Thread.sleep
int messageRate = 1000; // Max messages per second
long sleepTime = 1000L / messageRate;
while (true) {
sendMessage(channel, queueName, message);
try {
Thread.sleep(sleepTime);
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
break;
}
}
}
Why: Rate limiting controls the message flow rate, preventing consumer overload and ensuring stable operation.
Best Practices for Java and RabbitMQ Integration
- Use Connection Pooling: Instead of creating new connections for every work unit, consider using a connection pool to manage connections efficiently.
- Prefer Asynchronous Communication: Utilize asynchronous constructs for communication to avoid blocking threads.
- Implement Error Handling: Incorporate error handling as shown in previous examples to ensure robustness.
- Monitor Performance: Use RabbitMQ monitoring tools and libraries, such as Prometheus or Grafana, to visualize metrics and troubleshoot issues effectively.
Lessons Learned
Integrating RabbitMQ with Java applications can significantly enhance communication and workflow efficiency. However, various integration issues may arise, from connection problems to message acknowledgment errors. By following the strategies and code examples provided in this blog, you can effectively troubleshoot common integration issues.
To learn more about RabbitMQ and Java integration, visit the official RabbitMQ Java client documentation.
For further reading on message-driven architectures, you might find this article useful: Designing Microservices with Messaging.
By implementing these practices and continuously monitoring your systems, you can ensure that your integration with RabbitMQ is both robust and efficient. Happy coding!