Overcoming Java gRPC Compatibility Issues in Microservices
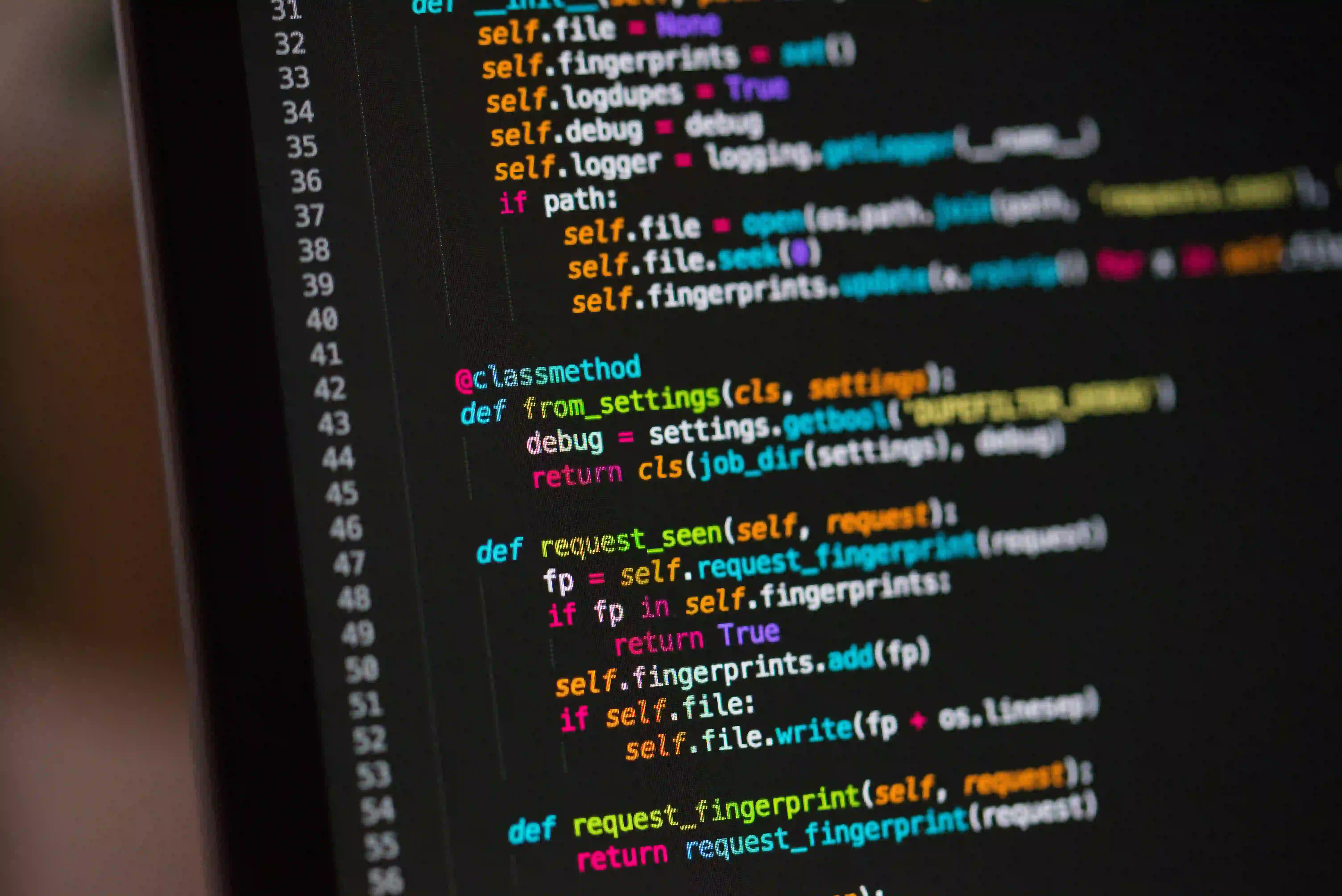
Overcoming Java gRPC Compatibility Issues in Microservices
In the age of microservices architecture, creating scalable and efficient systems is paramount. One of the most powerful tools for building such systems in Java is gRPC (Google Remote Procedure Call). However, developers often encounter compatibility issues while working with gRPC in a Java environment. This blog post outlines common compatibility challenges and provides solutions you can implement to ensure smooth operations in your microservices architecture.
Understanding gRPC and Microservices
gRPC is a modern open-source high-performance RPC framework that can run in any environment. It uses Protocol Buffers (protobuf) as its interface description language, which is crucial for defining service methods and message types. The use of HTTP/2 enhances performance by providing multiplexing, allowing multiple streams over the same connection.
Microservices refer to an architectural style that structures an application as a collection of small autonomous services, each responsible for a specific business capability. Employing gRPC in microservice architecture can streamline communications between these services.
Common Compatibility Issues
-
Protobuf Versioning: The evolving nature of Protocol Buffers can lead to incompatibilities when different microservices use varying versions.
-
gRPC Library Versions: Dependencies on specific versions of the gRPC Java libraries can create challenges, especially if services are deployed independently.
-
Protocol Definition Changes: Changes in the proto files can lead to breaking changes for services that rely on those definitions.
-
Transport Layer Differences: Differences in transport layer configurations can lead to connectivity issues.
Solution Strategies
1. Managing Protobuf Versions
One of the most prevalent compatibility issues arises from different protobuf versions. To mitigate this:
- Use a Dependency Management Tool: Tools like Maven or Gradle can help in managing dependencies effectively.
Example in Maven:
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>3.21.0</version>
</dependency>
This makes sure that all microservices use the same version of protobuf, minimizing compatibility issues.
2. Aligning gRPC Versions
Ensuring all microservices use the same gRPC library version can drastically reduce issues. Create a shared pom.xml
or build.gradle
file that defines the gRPC version.
Example in Gradle:
ext {
grpcVersion = '1.45.0'
}
dependencies {
implementation "io.grpc:grpc-netty:${grpcVersion}"
implementation "io.grpc:grpc-protobuf:${grpcVersion}"
implementation "io.grpc:grpc-stub:${grpcVersion}"
}
This promotes a consistent environment across all services, avoiding version mismatches.
3. Protocol Definition Versioning
When changing the proto definitions, consider versioning your APIs. Here’s how to do it effectively:
-
Add new fields instead of modifying existing ones. This way, older services can still operate without issues.
-
Utilize oneof fields: These allow you to define different options in a clean manner.
Example of a protocol definition with versioning:
syntax = "proto3";
package service.v1;
message User {
string id = 1;
string name = 2;
// New Field
string email = 3;
}
// Version 2 of User
package service.v2;
message User {
string id = 1;
string name = 2;
string email = 3;
// New Field
string phone_number = 4;
}
By versioning your proto files, you create backward compatibility, allowing multiple microservices to thrive without immediate refactoring.
4. Standardizing Configuration
Differences in gRPC server and client configurations can lead to difficulties in service calls. Here’s how to address configuration mismatches:
-
Central Configuration Management: Use tools like Spring Cloud Config or Consul to standardize service configurations.
-
Consistent Timeout and Retry Policies: Make sure all services implement the same timeout and retry policies to manage failures effectively.
Example of gRPC client configuration in Java:
ManagedChannel channel = ManagedChannelBuilder.forAddress("localhost", 50051)
.usePlaintext() // Make sure to set this correctly for your environment
.idleTimeout(30, TimeUnit.SECONDS) // Idle timeout configuration
.intercept(new ClientInterceptor() { /* Implement your interceptor */})
.build();
5. Testing and CI/CD Integration
To ensure that compatibility issues do not affect production, integrate testing into your CI/CD pipeline.
-
Contract Testing: Implement consumer-driven contracts to ensure that changes in the service's API don’t break other services.
-
End-to-End Integration Tests: Create integration tests that go through all services and ensure interactions occur as intended.
Example of a simple JUnit test for a gRPC service:
@Test
public void testUserService() {
UserServiceGrpc.UserServiceBlockingStub stub = UserServiceGrpc.newBlockingStub(channel);
User user = User.newBuilder().setId("1").setName("John").setEmail("john@example.com").build();
Response response = stub.createUser(user);
assertEquals("Success", response.getMessage());
}
6. Utilizing gRPC Features
Leverage built-in gRPC features that enhance compatibility and performance:
-
Load Balancing: Use gRPC’s built-in load balancing with service discovery tools like Consul or Eureka to manage service instances dynamically.
-
Streaming: Take advantage of gRPC’s bi-directional streaming to handle heavy loads more efficiently.
Example demonstrating bi-directional streaming:
public StreamObserver<UserRequest> createUser(StreamObserver<UserResponse> responseObserver) {
return new StreamObserver<UserRequest>() {
@Override
public void onNext(UserRequest request) {
// Process the incoming request
// Send a response back to the client
responseObserver.onNext(UserResponse.newBuilder().setMessage("User created").build());
}
@Override
public void onError(Throwable t) {
// Handle error
}
@Override
public void onCompleted() {
responseObserver.onCompleted();
}
};
}
Closing the Chapter
Overcoming Java gRPC compatibility issues in microservices requires a combination of strategic planning and operational diligence. Whether you’re dealing with protobuf versioning, library mismatches, or transport layer configurations, maintaining standardization across your services is crucial.
By implementing the strategies outlined in this post, such as effective version handling, leveraging protobuf features, and continuous testing, you can build a robust microservices architecture that fully exploits the capabilities of gRPC.
For more information on gRPC and Java, check out the official gRPC documentation and explore related articles that can help solidify your understanding.
This blog concludes with an invitation for comments and further discussions. If you have any experiences or tips regarding gRPC compatibility issues, please share!