Common Eclipse IDE Errors and How to Fix Them
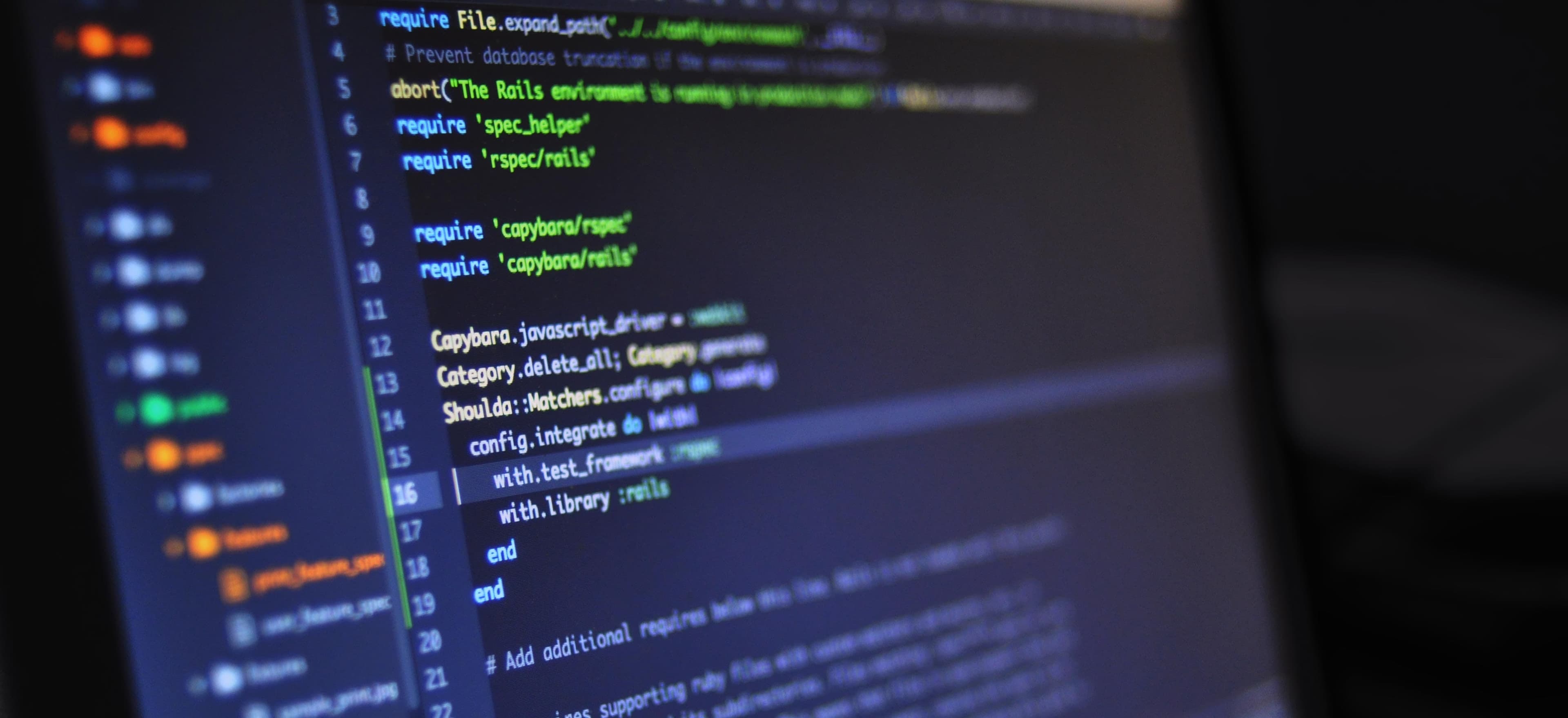
- Published on
Common Eclipse IDE Errors and How to Fix Them
Eclipse is a powerful Integrated Development Environment (IDE) widely used for Java development and other programming languages. However, developers frequently run into errors that can disrupt their workflow. This blog post will explore the most common errors faced in the Eclipse IDE and provide solutions to fix them effectively.
Beginning Insights
Eclipse, although robust, can be tricky, especially for newcomers. The good news is that most errors are easily fixable with a little guidance. Whether you're struggling with build errors, classpath issues, or workspace glitches, this guide will help you troubleshoot and resolve these common problems.
1. Java Build Path Errors
Eclipse users often encounter Java Build Path Errors. When a project cannot find the necessary libraries or dependencies, it usually results in errors such as "Build path is incorrect" or "Cannot find the class".
How to Fix it
- Check Libraries in Build Path: Right-click on your project and select Properties > Java Build Path. Ensure all required libraries are included.
- Refresh Project: Sometimes, simply refreshing your project can resolve path issues. Right-click on your project and select
Refresh
or use the F5 key.
Here's how you can verify your project setup in Java:
public class Example {
public static void main(String[] args) {
System.out.println("Hello, World!"); // Simple output to verify setup
}
}
If you can run this code without issues, your setup is likely correct.
2. Workspace Corruption Issues
Eclipse stores metadata in the workspace and sometimes that data can become corrupted. Symptoms include the inability to open the IDE or certain projects failing to load.
How to Fix it
- Start with a New Workspace: Open Eclipse and choose
File
>Switch Workspace
>Other
> Create a new workspace. This often solves corruption issues. - Clean Your Workspace: Use
Project
>Clean...
from the menu. This will rebuild all the projects.
3. Out of Memory Errors
If you are running large applications or multiple projects, you might encounter the dreaded java.lang.OutOfMemoryError
.
How to Fix it
- Increase Heap Size: You’ll need to modify the
eclipse.ini
file located in your Eclipse installation directory. Add or modify the following lines:
-Xms512m
-Xmx2048m
This increases the initial heap size to 512 MB and the maximum to 2048 MB.
- Close Unused Projects: Keeping only the necessary projects open in the workspace can improve performance.
4. Unable to Start the Eclipse
Many users experience issues where the IDE fails to start correctly.
How to Fix it
-
Check the Log File: If Eclipse fails to start, check the
.log
file located in the.metadata
folder within your workspace. -
Edit the Configuration: Open the
eclipse.ini
file and check if any configurations are incorrect or corrupted.
5. Interface Freezing or Crashing
Sometimes, Eclipse becomes unresponsive. This may happen due to various reasons like insufficient memory or excessive plugins.
How to Fix it
-
Disable Unused Plugins: Go to
Help
>Eclipse Marketplace
, and review the installed plugins. Removing unnecessary ones can enhance performance. -
Increase Heap Size: Refer to the previous section for instructions on how to modify the
eclipse.ini
file.
6. Missing JRE System Library
When you create a project and you see an error about the "JRE System Library", it means that Eclipse is unable to find the Java Runtime Environment.
How to Fix it
-
Right-click on Project: Go to Properties > Java Build Path and check the
Libraries
tab. -
Add the JRE Library: If not listed, click on
Add Library…
>JRE System Library
, and select an appropriate JRE.
public class JreTest {
public static void main(String[] args) {
System.out.println("JRE Setup Successful!"); // Confirm JRE is added
}
}
7. Error During Project Import
Sometimes importing a project can result in errors like "Project already exists" or "Error importing project".
How to Fix it
-
Use Import Wizard: Go to
File
>Import...
and selectGeneral
>Existing Projects into Workspace
. Make sure to uncheck the option "Copy projects into workspace". -
Check for Conflicts: Ensure there are no pre-existing projects with the same name in your workspace.
8. Code Syntax Errors andWarnings
Syntax issues should be handled promptly to maintain clean code.
How to Fix it
-
Verify Code Check: Ensure you have the necessary Java Development Kit (JDK) installed.
-
Fix Syntax: Rectify the syntax errors highlighted by the IDE. For instance, a common mistake might be a missing semicolon:
public class SyntaxError {
public static void main(String[] args) {
System.out.println("Track your syntax errors."); // Missing semicolon here
} // End of class
}
A Final Look
Eclipse is indeed a sophisticated tool for Java programming, but the errors outlined above can hinder productivity. However, with these troubleshooting tips, you can resolve most of the common errors quickly and efficiently.
Keep an eye on your workspace setup, library paths, and memory allocation to maintain a seamless development environment. For further reading, explore the Eclipse Documentation to dive deeper into troubleshooting and optimizing your coding environment.
By being aware of these common challenges and equipping yourself with the knowledge to solve them, you'll be well on your way to becoming an adept Eclipse user.
Happy coding!
Checkout our other articles