Common Pitfalls When Implementing Akka in Java
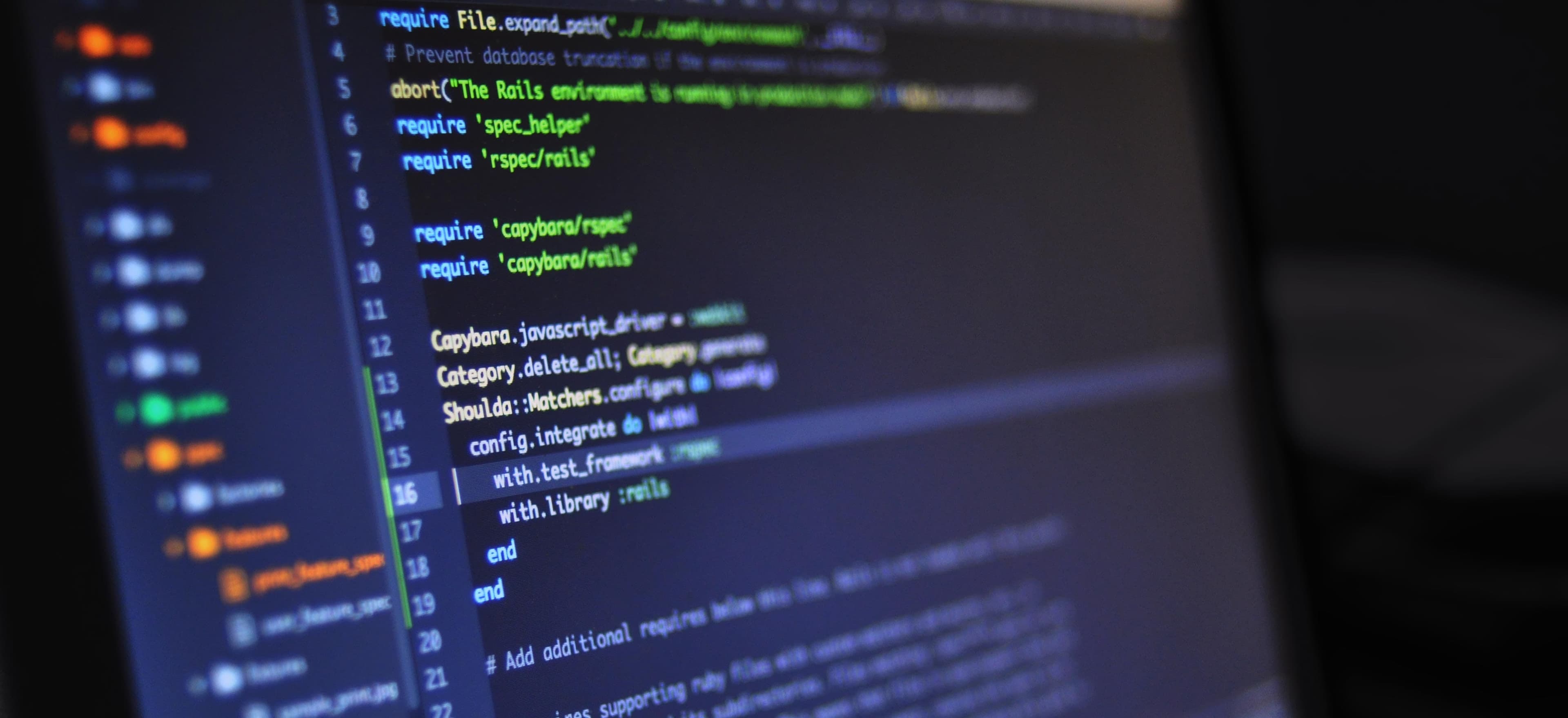
- Published on
Common Pitfalls When Implementing Akka in Java
When it comes to building responsive, resilient, and elastic systems, Akka is a powerful toolkit that operates on the principles of the Actor model. While Akka was primarily designed for Scala, it has a robust Java API that allows Java developers to leverage its capabilities. However, as with any technology, there are potential pitfalls when implementing Akka in Java. This blog post will explore these common challenges and provide guidance on how to navigate them effectively.
Understanding the Actor Model
Before diving into common pitfalls, let’s clarify the Actor model. In this programming paradigm, an actor is a fundamental unit of computation. Actors:
- Send messages to other actors.
- Create new actors.
- Maintain state.
- Do not share mutable state.
In essence, the Actor model promotes concurrency by isolating thread-bound data and managing it through messaging.
Pitfall 1: Ignoring the Actor’s Stateless Nature
Issue
One of the fundamental principles of the Actor model is that actors should be stateless. Many developers new to Akka make the mistake of storing application state within actors.
Solution
Instead of maintaining mutable state, use:
- Persistence: Utilize Akka Persistence to keep state changes in a database.
- Event Sourcing: Generate events that can be replayed to recover the state.
Here is a simple example of an actor that adheres to statelessness while employing events for state management.
import akka.actor.AbstractActor;
import akka.event.Logging;
import akka.event.LoggingAdapter;
public class EventSourcingActor extends AbstractActor {
private LoggingAdapter log = Logging.getLogger(getContext().getSystem(), this);
@Override
public Receive createReceive() {
return receiveBuilder()
.match(Command.class, this::onCommand)
.build();
}
private void onCommand(Command command) {
log.info("Processing command: {}", command);
// Imagine saving to a database here instead of mutable state
getSender().tell(new Response("Success"), getSelf());
}
}
In this code, where an actor processes input commands correctly, we avoid retaining mutable states by delegating stored information to a database.
Pitfall 2: Not Handling Failed Messages
Issue
Another frequent oversight is a lack of error handling when messages fail to process. In Akka, if an actor throws an exception while handling a message, the default behavior is to terminate the actor.
Solution
Implementing proper supervision strategies is crucial. You can define how a supervisor (usually a parent actor) should handle its children in case of failure.
Here’s a simplified example of a supervisor actor:
import akka.actor.AbstractActor;
import akka.actor.Props;
import akka.actor.SupervisorStrategy;
import akka.actor.OneForOneStrategy;
import akka.japi.pf.DeciderBuilder;
public class SupervisorActor extends AbstractActor {
@Override
public SupervisorStrategy supervisorStrategy() {
return new OneForOneStrategy()
.match(IllegalArgumentException.class, e -> SupervisorStrategy.restart())
.match(Exception.class, e -> SupervisorStrategy.escalate());
}
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, msg -> getContext().actorOf(Props.create(ChildActor.class)).tell(msg, getSelf()))
.build();
}
}
In this example, if an actor throws an IllegalArgumentException
, it will be restarted, while other exceptions will escalate further.
Pitfall 3: Misunderstanding Actor Lifecycle
Issue
Each actor in Akka follows a specified lifecycle that developers must understand. Not adhering to these lifecycle events can lead to resource leaks or unhandled states.
Solution
Override key lifecycle methods (like preStart
, postStop
, etc.) properly. These are executed at specific moments, ensuring that resources are correctly allocated and released.
@Override
public void preStart() {
// Initialize resources like databases or caches
}
@Override
public void postStop() {
// Release resources, close connections, etc.
log.info("Actor stopped and resources released.");
}
By properly managing the lifecycle of your actors, you can ensure smooth operations and health of your application.
Pitfall 4: Blocking Operations
Issue
Developers new to Akka often fall into the trap of performing blocking operations inside an actor. Doing this goes against the principles of reactive programming, which can lead to performance bottlenecks.
Solution
For any blocking calls (like calling a web service or accessing a database), use Futures or schedule these interactions in a separate thread.
Here’s an example of non-blocking execution using a future:
import akka.actor.AbstractActor;
import akka.dispatch.OnComplete;
import akka.pattern.Patterns;
import akka.util.Timeout;
import scala.concurrent.Future;
import java.util.concurrent.CompletionStage;
public class NonBlockingActor extends AbstractActor {
@Override
public Receive createReceive() {
return receiveBuilder()
.match(String.class, this::handleRequest)
.build();
}
private void handleRequest(String request) {
Timeout timeout = new Timeout(Duration.create(5, TimeUnit.SECONDS));
Future<Object> futureResponse = Patterns.ask(requestService, request, timeout);
futureResponse.onComplete(new OnComplete<Object>() {
@Override
public void onComplete(Throwable failure, Object result) {
if (result != null) {
getSender().tell(result, getSelf());
}
}
}, getContext().dispatcher());
}
}
Using Futures allows actors to free up threads while waiting for responses, adhering to the reactive principles that Akka promotes.
Pitfall 5: Overusing Actor Hierarchies
Issue
While actor hierarchies can be beneficial for certain applications, overusing them often leads to complexity and hard-to-manage code.
Solution
Use actor hierarchies wisely. Create a clear and manageable structure that reflects the domain model. Flat hierarchies are often easier to debug and understand.
Closing the Chapter
Implementing Akka in Java can be immensely powerful, but it comes with its set of challenges. By understanding and acknowledging these common pitfalls, developers can avoid common mistakes that new users make when adopting this powerful tool.
Are you looking to dive deeper into reactive programming concepts? Check out Akka Documentation for more comprehensive guidance on implementing Akka properly. Moreover, exploring articles on Reactive Programming Patterns can enhance your understanding of effective patterns to use with Akka.
Keep this guide handy as you embark on your Akka journey in Java, and remember that careful design leads to resilient, scalable applications. Happy coding!