Common Thymeleaf Template Errors in Spring Boot Blogs
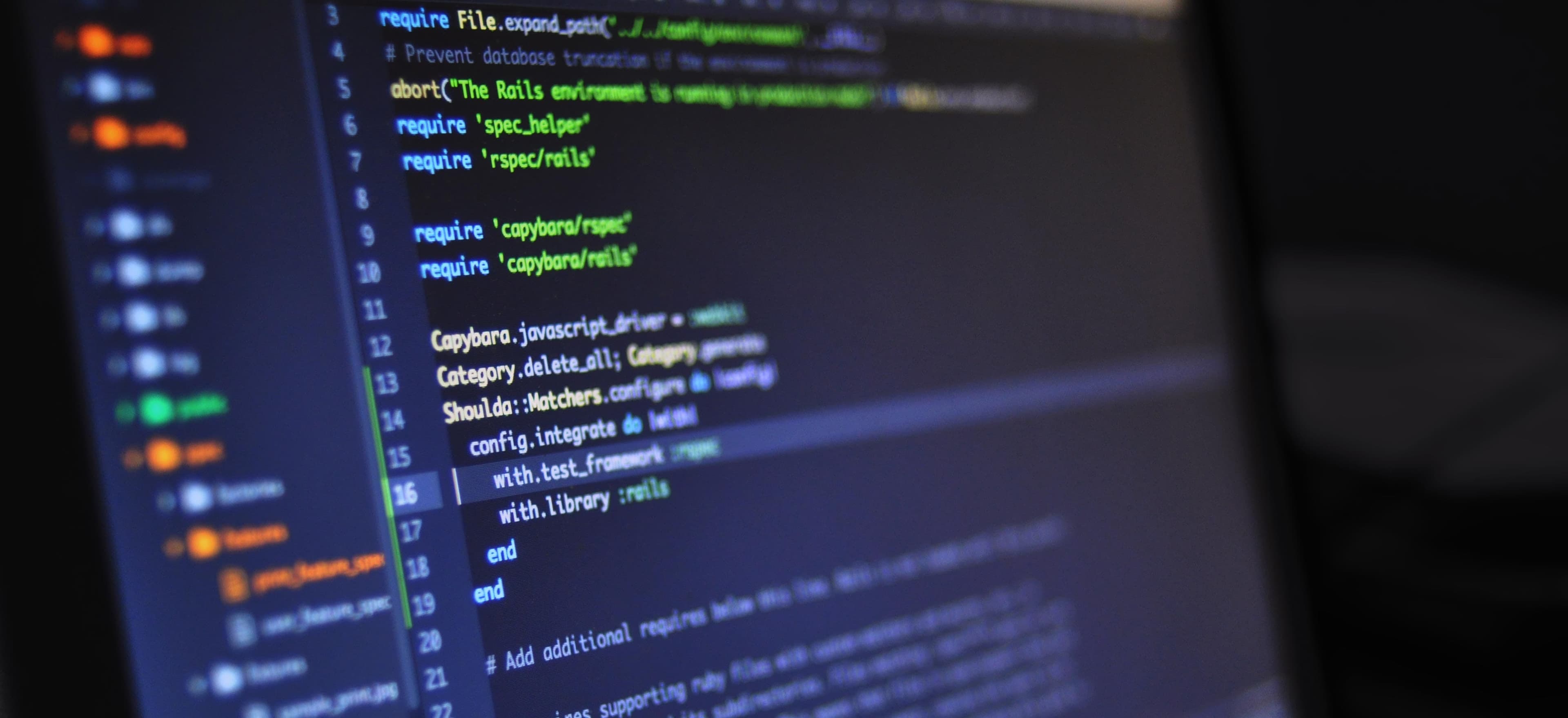
- Published on
Common Thymeleaf Template Errors in Spring Boot
Thymeleaf is a powerful and flexible templating engine that integrates seamlessly with Spring Boot applications. While it's user-friendly, developers often encounter common errors that can disrupt the smooth functioning of an application. In this blog post, we will explore the most prevalent Thymeleaf template errors in Spring Boot, examine their causes, and provide solutions to prevent them.
Why Use Thymeleaf?
Before diving into the errors, let’s quickly review why Thymeleaf is a popular choice as a templating engine:
- Natural Templating: Thymeleaf templates are HTML files that can be rendered by web browsers or processed by the server. This characteristic allows for easier collaboration between designers and developers.
- Spring Integration: Thymeleaf is designed for use with Spring MVC, making it a natural fit for Spring Boot applications.
- Extensible: You can create your own custom attributes and dialects, offering flexibility for specific application needs.
Now that we have a brief understanding of Thymeleaf, let’s jump into the common errors you might encounter.
1. Template Not Found
One of the most frequent errors is the template not found error. You may encounter a message like this in your console:
Whitelabel Error Page
This application has no explicit mapping for /error, so you are seeing this as a fallback.
Cause
This usually occurs when Thymeleaf cannot locate the specified template file. The template files must reside in the src/main/resources/templates
directory.
Solution
Ensure that your file is correctly located, and that you are referring to it in your controller properly. For instance:
@GetMapping("/welcome")
public String welcome(Model model) {
model.addAttribute("message", "Welcome to Thymeleaf!");
return "welcome"; // Ensure welcome.html is present in src/main/resources/templates
}
Additional Tips
- Use
.html
extensions in your Thymeleaf template filenames. - Validate that the template file is spelled correctly (case-sensitive).
2. Misconfigured Template Resolver
Another common error is an Invalid template
exception that arises when your template resolver is not configured correctly.
Cause
This typically happens when the default Spring Boot setup doesn't include the right configuration for the Thymeleaf Engine.
Solution
To set up the template resolver properly, make sure your application.properties
(or application.yml
) contains the following properties:
spring.thymeleaf.prefix=classpath:/templates/
spring.thymeleaf.suffix=.html
These properties define where to find the Thymeleaf templates and their file extensions.
3. Thymeleaf Syntax Errors
Thymeleaf syntax must be followed precisely. A common error occurs when a Thymeleaf attribute is incorrectly formatted, such as using th:text
instead of th: text
.
Cause
A simple typo can lead to Thymeleaf not rendering the template correctly.
Solution
Carefully review your syntax as follows:
<h1 th:text="${message}">Default Message</h1>
This line should generate a header with whatever message is in the model. Misspelling th:text
will yield no output.
Best Practices
- Use an IDE with Thymeleaf support that can highlight such errors.
- Regularly test your templates to catch syntax mistakes early.
4. Unused Thymeleaf Attributes Returns HTML on Browser
You might find that when you include unused Thymeleaf attributes, they return plain HTML instead of being processed by Thymeleaf.
Cause
This can occur when you're using Thymeleaf attributes in a browser without running the web server, which renders your templates normally.
Solution
Ensure you are running your Spring Boot application and accessing your application in the browser through routes defined in your controllers.
Example
Here's a simple controller with Thymeleaf integration:
@Controller
public class HomeController {
@GetMapping("/")
public String home(Model model) {
model.addAttribute("title", "Home");
return "index"; // Renders index.html
}
}
5. Missing or Incorrect Model Attributes
Occasionally, developers forget to add model attributes they reference in templates, which leads to rendering errors.
Cause
Referencing a variable that isn't added to the model in your controller will lead to a null
output in the final rendered template.
Solution
Ensure all referenced attributes are included in the model:
@GetMapping("/greeting")
public String greeting(Model model) {
model.addAttribute("name", "World");
return "greeting"; // Make sure `${name}` exists in greeting.html
}
Example Template
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Greeting</title>
</head>
<body>
<h1 th:text="'Hello, ' + ${name}">Hello, Guest!</h1>
</body>
</html>
6. CSS and JavaScript Resources Not Loaded
Another frequent issue is not loading CSS and JavaScript resources properly within Thymeleaf templates.
Cause
Incorrect paths can result from wrong context paths or the absence of declared resources.
Solution
Utilize the @{}
syntax for linking resources correctly:
<link rel="stylesheet" th:href="@{/css/styles.css}" />
<script th:src="@{/js/scripts.js}"></script>
Additional Strategy
Put your static resources in the src/main/resources/static
directory to ensure they are accessible.
Closing Remarks
While Thymeleaf is an excellent templating engine, it's crucial to be aware of common pitfalls. Understanding these errors will enable you to troubleshoot quickly, saving valuable development time. By incorporating best practices into your coding routine, such as proper resource management and syntax checks, you can create efficient and error-free applications.
For additional resources, consider visiting the Thymeleaf Documentation to deepen your knowledge.
By knowing these errors and their solutions and practicing diligently, you will improve your Thymeleaf proficiency and build more robust Spring Boot applications. Happy coding!
Checkout our other articles