Common Pitfalls When Building REST APIs with Micronaut
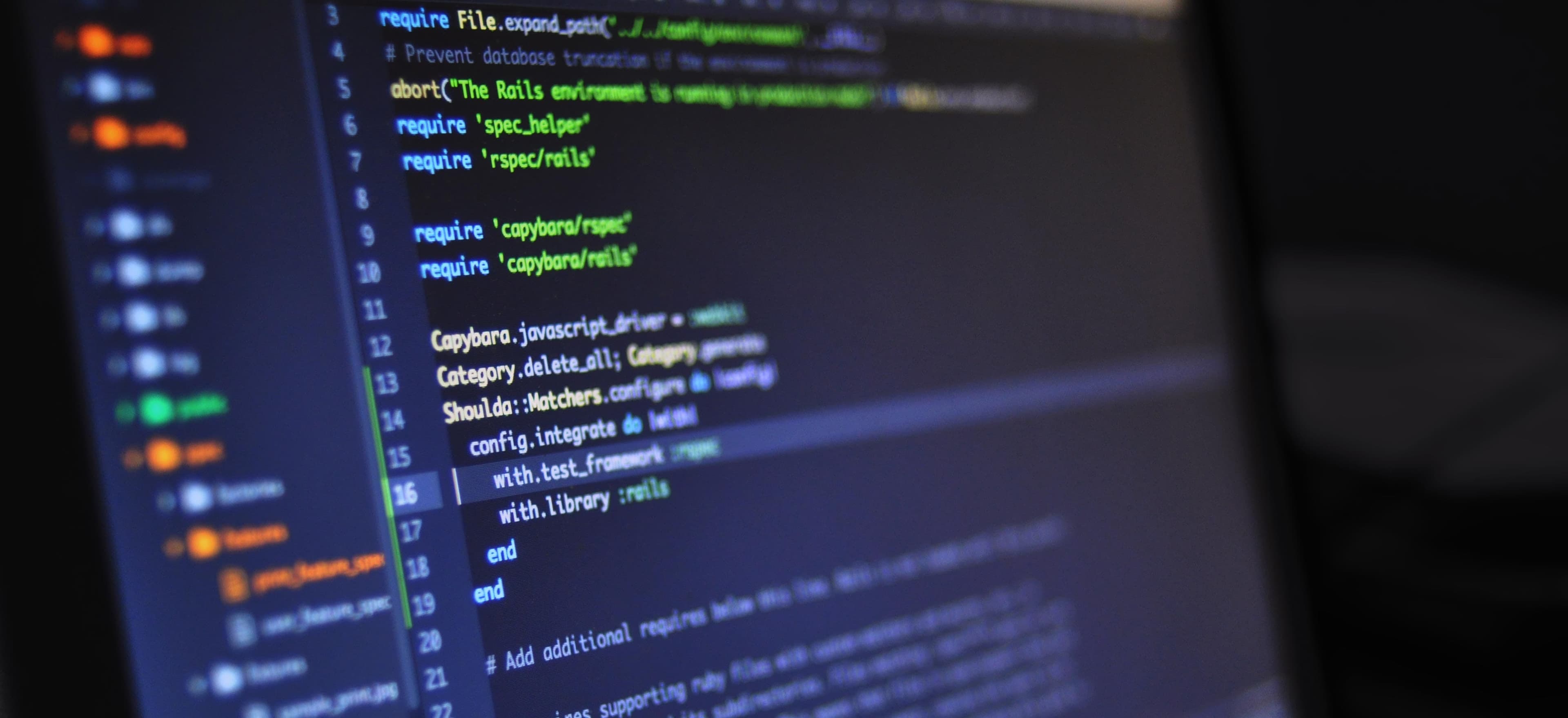
- Published on
Common Pitfalls When Building REST APIs with Micronaut
When it comes to building REST APIs, developers often gravitate towards frameworks that promise speed, efficiency, and ease of use. Micronaut is one such framework, designed to create microservices with exceptional startup time and low memory footprint. However, like any technology, pitfalls can await the unwary. In this post, we will explore common pitfalls when building REST APIs with Micronaut, and how to avoid them.
1. Ignoring Dependency Injection Best Practices
One of the key features of Micronaut is its built-in dependency injection (DI) system. However, developers sometimes overlook best practices for DI, leading to tightly coupled components that are hard to maintain and test.
Why It Matters
Proper use of DI encourages loose coupling, which makes the codebase easier to manage, test, and extend in the future.
Example Code
import jakarta.inject.Singleton;
@Singleton
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public User getUserById(Long id) {
return userRepository.findById(id)
.orElseThrow(() -> new UserNotFoundException(id));
}
}
Commentary
In this example, UserService
is injected with a UserRepository
. This makes UserService
component more testable, as mocking UserRepository
is straightforward during unit testing. Avoid newing up dependencies inside your classes. Always prefer constructor injection where possible.
2. Not Utilizing Micronaut's Native Compilation
Micronaut supports native image compilation via GraalVM, which can significantly reduce the memory footprint and startup time of your application.
Why It Matters
Failing to leverage this capability leads to longer startup times in a production environment, which can negate the performance benefits that Micronaut offers.
How to Enable Native Compilation
To enable native compilation, add the following plugin to your build.gradle
:
plugins {
id 'io.micronaut.application' version '2.5.0' // Replace with the correct version
id 'com.github.johnramsden.graalvm-native' version '0.9.0'
}
You’ll also need to set up your application.yml
file correctly:
micronaut:
application:
name: example-app
native-image:
enabled: true
Commentary
Following these steps will allow you to compile your application to a native image. By doing so, the performance improvements become significant, especially for microservices where fast startup and lower resource consumption are essential.
3. Mismanaging Exception Handling
Another common pitfall is failing to implement a coherent exception handling strategy. Poor exception management can lead to unhandled exceptions being thrown and unexpected HTTP status codes being returned.
Why It Matters
A coherent error handling strategy ensures consistent responses to API consumers and makes debugging easier.
Example Code
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.ExceptionHandler;
import io.micronaut.http.HttpResponse;
@Controller
public class UserController {
// Assume that UserService is injected
public User getUser(Long id) {
return userService.getUserById(id);
}
@ExceptionHandler(UserNotFoundException.class)
public HttpResponse<String> handleUserNotFound(UserNotFoundException e) {
return HttpResponse.notFound(e.getMessage());
}
}
Commentary
In this controller, a custom exception handler for UserNotFoundException
ensures that a 404 status code is returned if a user is not found. By centralizing error management, you can also easily add logging or metrics.
4. Underestimating Testing
Testing is often seen as a secondary concern, leading to a lack of unit and integration tests. This can cause significant issues down the line when bugs can easily slip through.
Why It Matters
A lack of tests can make code modification risky and can lead to unexpected behavior in production.
Example Code
import io.micronaut.test.extensions.junit5.annotation.MicronautTest;
import org.junit.jupiter.api.Test;
import jakarta.inject.Inject;
import static org.assertj.core.api.Assertions.assertThat;
@MicronautTest
public class UserServiceTest {
@Inject
UserService userService;
@Test
void testGetUserById() {
User user = userService.getUserById(1L);
assertThat(user).isNotNull();
assertThat(user.getId()).isEqualTo(1L);
}
}
Commentary
Using Micronaut's testing support, we inject the UserService
and verify that the API behaves as expected. Tests like these ensure that your application is robust and that functionalities remain intact during updates.
5. Skipping Documentation
Without clear API documentation, your REST service could present challenges to clients. Often times developers skip this stage thinking documentation comes after the code.
Why It Matters
Good API documentation is essential for maintenance, onboarding new team members, and providing guidance to API consumers.
Tools for API Documentation
Micronaut offers integration with Swagger and OpenAPI for automatic generation of API documentation.
Example Setup in build.gradle
dependencies {
implementation "io.micronaut.openapi:micronaut-openapi"
implementation "io.swagger.core.v3:swagger-annotations"
}
Commentary
Once you have the appropriate dependencies, you can annotate your controllers with Swagger annotations. This will allow you to auto-generate documentation that is easily shared with your team and API consumers.
6. Overlooking Security Features
Security is often an afterthought, but neglecting it can expose your API to vulnerabilities.
Why It Matters
Failing to secure APIs can lead to data breaches and unauthorized access.
Example Code for Securing Endpoints
import io.micronaut.security.annotation.Secured;
import io.micronaut.http.annotation.Controller;
import io.micronaut.http.annotation.Get;
@Secured("ROLE_USER")
@Controller("/user")
public class SecureUserController {
@Get("/{id}")
public User getUser(Long id) {
return userService.getUserById(id);
}
}
Commentary
This code snippet demonstrates how to secure a controller by requiring the user to have the ROLE_USER
. Micronaut offers a variety of security integrations which can help secure your applications.
Final Thoughts
Building REST APIs with Micronaut can be highly rewarding, but it's essential to avoid common pitfalls. By following best practices in dependency injection, leveraging native compilation, implementing proper exception handling, prioritizing testing, documenting thoroughly, and ensuring security, you can create robust and maintainable APIs.
Further Reading
- Micronaut Documentation
- GraalVM Documentation
- Swagger/OpenAPI for Micronaut
With mindful implementation and continual learning, developers can unlock the full potential of Micronaut, leading to efficient and scalable APIs.
Checkout our other articles