Mastering Java's Process API: Common Pitfalls Uncovered
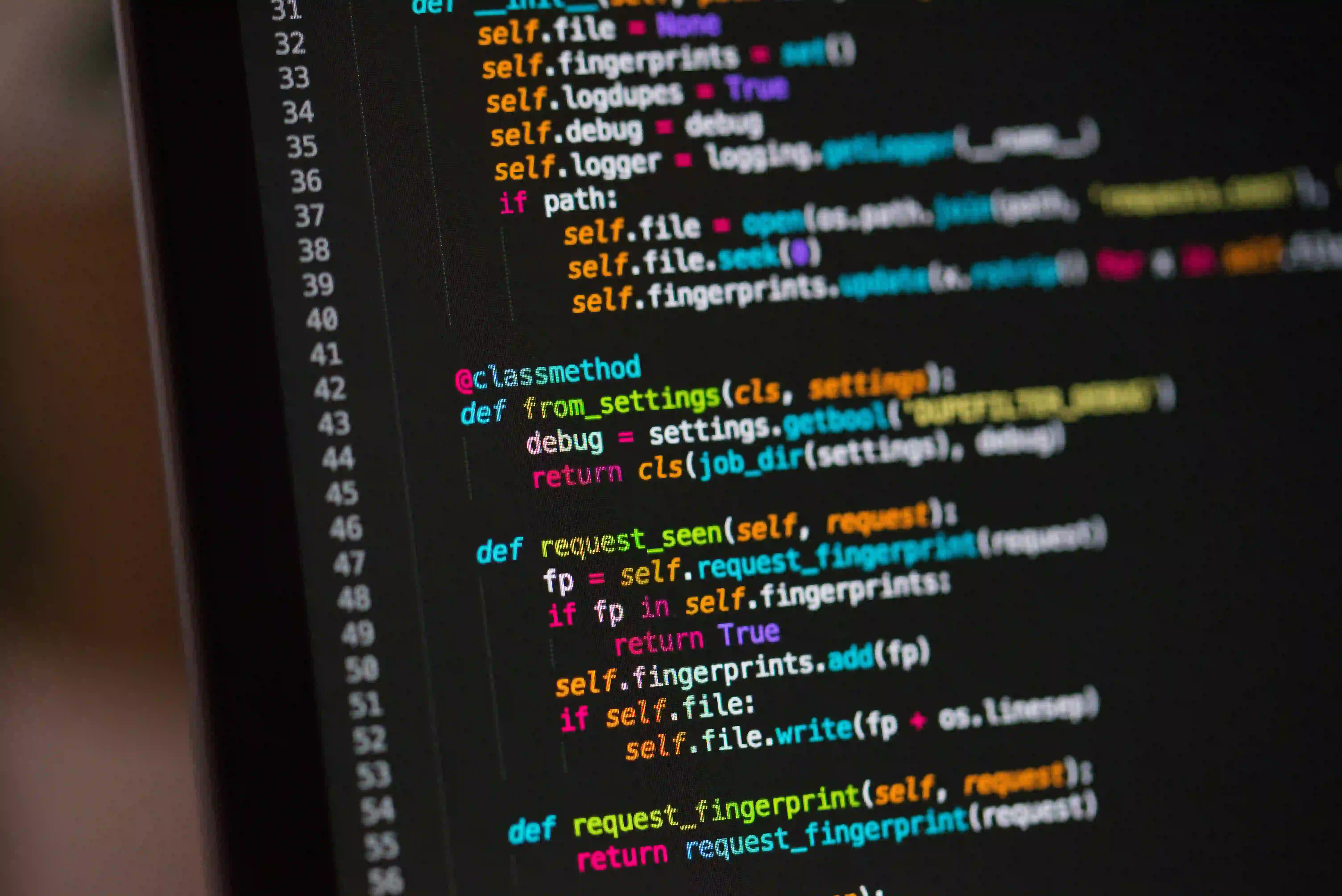
Mastering Java's Process API: Common Pitfalls Uncovered
Java's Process API has been a powerful tool for developers, allowing them to create and manage native processes from within a Java application. Despite its capabilities, many developers face challenges when using the Process API, often leading to unexpected behaviors and hard-to-debug issues. In this blog post, we will explore some common pitfalls when working with Java's Process API, how to avoid them, and provide you with practical examples to enhance your understanding.
Understanding the Process API
The Process API in Java, introduced in JDK 5, is primarily found in the java.lang.ProcessBuilder
and java.lang.Runtime
classes. It allows Java applications to execute external processes and interact with them programmatically.
Benefits of Using the Process API
-
Cross-Platform Compatibility: Java's built-in libraries often ensure you can run your code across different operating systems without modification.
-
Resource Management: You can manage the standard input, output, and error streams of the subprocess effectively.
-
Asynchronous Execution: You can spawn processes and interact with them without blocking your main application thread.
Getting Started with ProcessBuilder
Before delving into pitfalls, let’s start with an example that demonstrates how to use the ProcessBuilder
class to execute a simple command.
import java.io.BufferedReader;
import java.io.InputStreamReader;
public class ProcessExample {
public static void main(String[] args) {
try {
// Create a process builder for the command
ProcessBuilder processBuilder = new ProcessBuilder("ping", "-c", "5", "google.com");
// Start the process
Process process = processBuilder.start();
// Read the output from the command
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
// Wait for the process to finish
int exitCode = process.waitFor();
System.out.println("Exited with error code: " + exitCode);
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary on the Code
-
The
ProcessBuilder
is instantiated with the command to execute (ping -c 5 google.com
), demonstrating concise command formulation. -
InputStreamReader, wrapped in BufferedReader, helps read the process's output line-by-line, managing memory and performance efficiently.
-
The
waitFor
method ensures that the application halts until the external process completes its execution.
Common Pitfalls in Using Java's Process API
1. Ignoring Output and Error Streams
One of the most critical errors developers make is neglecting to handle the standard output and error streams of the subprocess. If these streams are not properly consumed, the subprocess may hang.
Solution:
Always read from both output and error streams to prevent the process from blocking.
// Code to consume both output and error streams
ProcessBuilder processBuilder = new ProcessBuilder("your-command");
Process process = processBuilder.start();
// Create reader for output and error streams
BufferedReader outputReader = new BufferedReader(new InputStreamReader(process.getInputStream()));
BufferedReader errorReader = new BufferedReader(new InputStreamReader(process.getErrorStream()));
// Read outputs
String outputLine;
while ((outputLine = outputReader.readLine()) != null) {
System.out.println("OUTPUT: " + outputLine);
}
// Read errors
String errorLine;
while ((errorLine = errorReader.readLine()) != null) {
System.err.println("ERROR: " + errorLine);
}
int exitCode = process.waitFor();
2. Not Configuring the Working Directory
When executing a process, the working directory is often crucial. Failing to set up the working directory can lead to file not found exceptions or unexpected behavior.
Solution:
Use the directory
method of ProcessBuilder
to set the working directory explicitly.
ProcessBuilder processBuilder = new ProcessBuilder("your-command");
processBuilder.directory(new File("/path/to/your/directory"));
3. Handling Non-Zero Exit Codes
Another common mistake is ignoring the exit code of the process. The exit code can provide valuable information regarding the execution status of the external command.
Solution:
Check the exit code and respond accordingly.
int exitCode = process.waitFor();
if (exitCode != 0) {
System.err.println("Process terminated with exit code: " + exitCode);
} else {
System.out.println("Process completed successfully.");
}
4. Resource Leaks Due to Unmanaged Processes
When processes are spawned, they consume system resources. If the Process API is not used carefully, it can lead to resource leaks, particularly if processes are not terminated or their streams are not closed.
Solution:
Always ensure proper resource management by closing streams and terminating processes as needed.
try {
// Your process logic here
} finally {
outputReader.close();
errorReader.close();
process.destroy();
}
Further Enhancements
While the basic functionality of the Process API is robust, leveraging additional features can improve your applications significantly. Here are a couple of resources for further reading:
-
Official Java Documentation on ProcessBuilder provides detailed information on options and configurations.
-
Java Concurrency in Practice offers insights into managing threads and processes efficiently.
Closing the Chapter
Mastering Java's Process API involves understanding both the capabilities and the common pitfalls associated with it. By adhering to best practices, managing input/output streams effectively, and carefully handling subprocesses, you can build robust Java applications that leverage native commands efficiently.
Practice makes perfect; so, the more you explore the Process API, the more adept you'll become. Share your experiences and any challenges you've faced in the comments below. Happy coding!