Understanding Java Bytecode: Common Pitfalls to Avoid
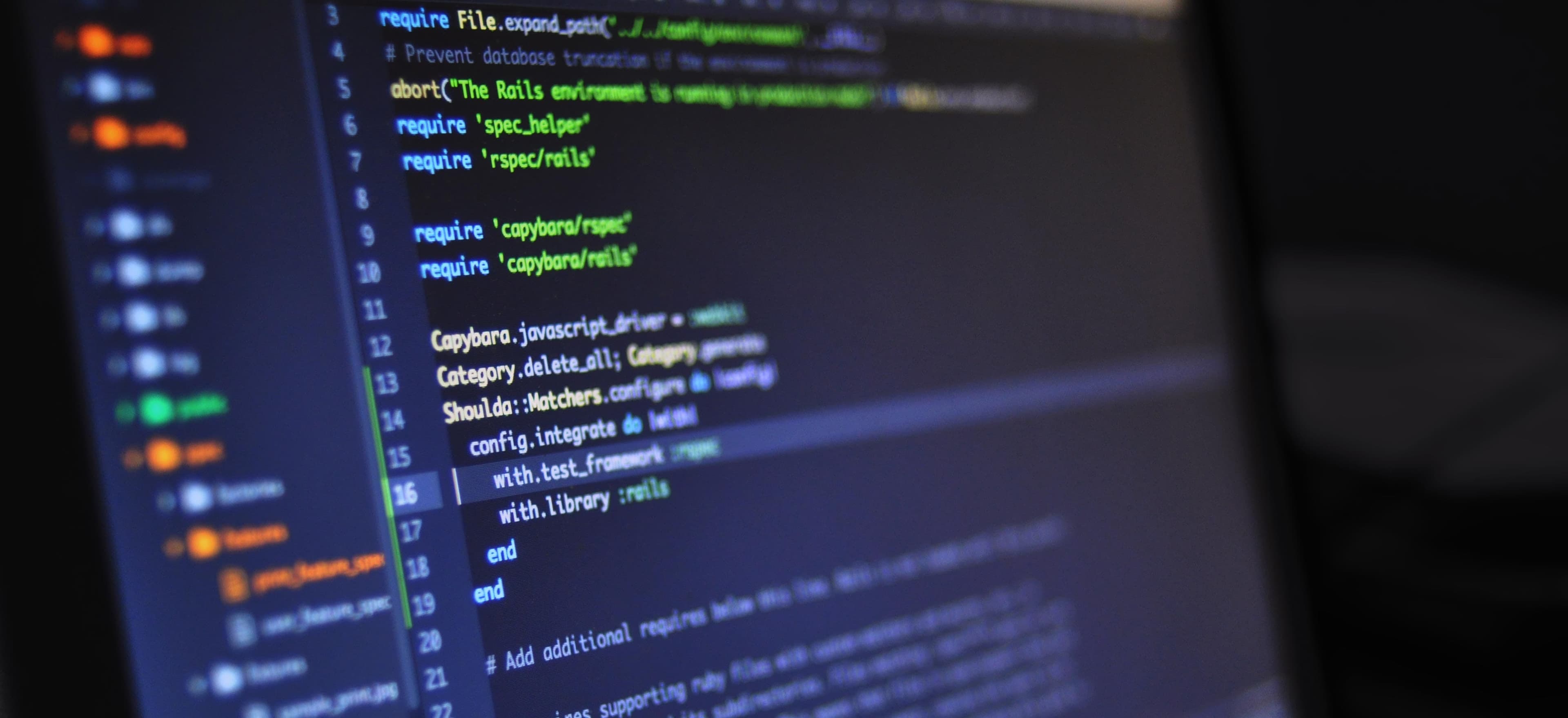
- Published on
Understanding Java Bytecode: Common Pitfalls to Avoid
Java is one of the most popular programming languages, known for its portability, ease of use, and robust features. One of the key aspects that sets Java apart from many other languages is that it compiles source code into an intermediate form known as bytecode. This bytecode is what the Java Virtual Machine (JVM) interprets and executes.
However, working with Java bytecode can introduce a number of pitfalls for developers. Understanding these can save you a lot of time, effort, and potential frustration. In this article, we will discuss what Java bytecode is, how it works, and the most common pitfalls to avoid when dealing with it.
What is Java Bytecode?
Bytecode is a low-level set of instructions that is generated by the Java compiler (javac) from your Java source code. It acts as an intermediary layer between Java source code (which is human-readable) and machine code (which is executed by the hardware). The JVM then takes this bytecode and executes it on any platform that has a JVM, aligning with Java's core philosophy: "Write Once, Run Anywhere".
Key Benefits of Bytecode
- Portability: Bytecode can run on any platform that has the JVM, making Java applications highly portable.
- Performance: The JVM can optimize bytecode execution through Just-In-Time (JIT) compilation.
- Security: Bytecode runs in a controlled environment, allowing for robust security checks.
The Common Pitfalls
While the benefits of Java bytecode are clear, there are common pitfalls developers may encounter. Below, we'll discuss these pitfalls along with relevant code examples.
1. Not Understanding the JVM Execution Model
One common mistake developers make is not fully understanding how the JVM executes bytecode. Many assume that the JVM translates bytecode into machine code linearly, but this isn't the case.
Example:
class Test {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
When compiling this class with javac Test.java
, bytecode is generated. However, upon execution with java Test
, the JVM may not run the main
method directly. Instead, it may perform optimizations based on runtime analysis.
Why Avoid This Pitfall: Failing to understand the JVM execution model can lead to performance issues and incorrect assumptions about how your code will behave.
2. Mismanagement of Class and Package Names
Another common issue is not fully adhering to Java’s naming conventions for classes and packages. Remember that package names are case-sensitive and must adhere to a specific structure.
Example:
package myPackage; // This is the correct declaration
public class MyClass {
// Class implementation
}
Failure to adhere to package naming conventions can lead to runtime exceptions or, worse, conflicts with other libraries.
What to Do: Always follow Java naming conventions; use lowercase for package names and uppercase for class names to avoid conflicts.
3. Overlooking Bytecode Manipulation Tools
Java bytecode can also be manipulated using various libraries such as ASM, Javassist, and BCEL. However, many developers overlook these tools and stick to traditional coding practices.
Example:
Using ASM to modify bytecode:
import org.objectweb.asm.*;
public class MyClassAdapter extends ClassVisitor {
public MyClassAdapter() {
super(Opcodes.ASM9);
}
@Override
public void visitEnd() {
visitMethod(Opcodes.ACC_PUBLIC, "newMethod", "()V", null, null);
super.visitEnd();
}
}
Why This Matters: Utilizing bytecode manipulation can allow for greater flexibility and optimization of your Java applications. Ignoring these tools can limit the capabilities of your projects.
4. Not Utilizing Reflection Wisely
Reflection can be a great tool in Java, enabling developers to inspect classes, interfaces, and methods at runtime. However, overusing it can introduce significant performance overhead and reduce the clarity of your code.
Example:
Method[] methods = MyClass.class.getDeclaredMethods();
for (Method method : methods) {
System.out.println(method.getName());
}
Using reflection incurs performance costs because the JVM needs to perform additional checks and lookups.
Recommendation: Use reflection sparingly and only when necessary. Consider alternate design patterns, such as Dependency Injection, that can often eliminate the need for reflection.
5. Ignoring Exception Handling in Bytecode
Exception handling is another area where many developers stumble. While the Java language provides a robust way to manage exceptions, the bytecode that implements this can become complex and lead to performance issues if not handled properly.
Example:
try {
// Code that may throw an exception
} catch (IOException e) {
// Handle exception
} finally {
// Cleanup code
}
Ignoring exception handling can lead to resource leaks or undefined behavior, particularly when dealing with I/O operations.
Best Practice: Always use exception handling best practices. This not only secures your code but also maintains performance through better resource management.
Closing Remarks
Understanding Java bytecode is fundamental for any Java developer who aims to build efficient, portable, and robust applications. By recognizing the common pitfalls discussed in this article, such as understanding the JVM execution model, proper class/package management, leveraging bytecode manipulation, judicious use of reflection, and implementing exception handling correctly, you can enhance your Java development skills significantly.
For more information on Java best practices and bytecode manipulation tools, you may explore:
- The Java Virtual Machine Specification
- ASM: A Java bytecode manipulation framework
- Java Reflection: Overview
By navigating these challenges effectively, you can improve the performance and reliability of your Java applications, ensuring that they resonate with the core strengths of the language. Embrace the journey of learning, and happy coding!
Checkout our other articles