Mastering Backpressure in Reactive Streams with Java
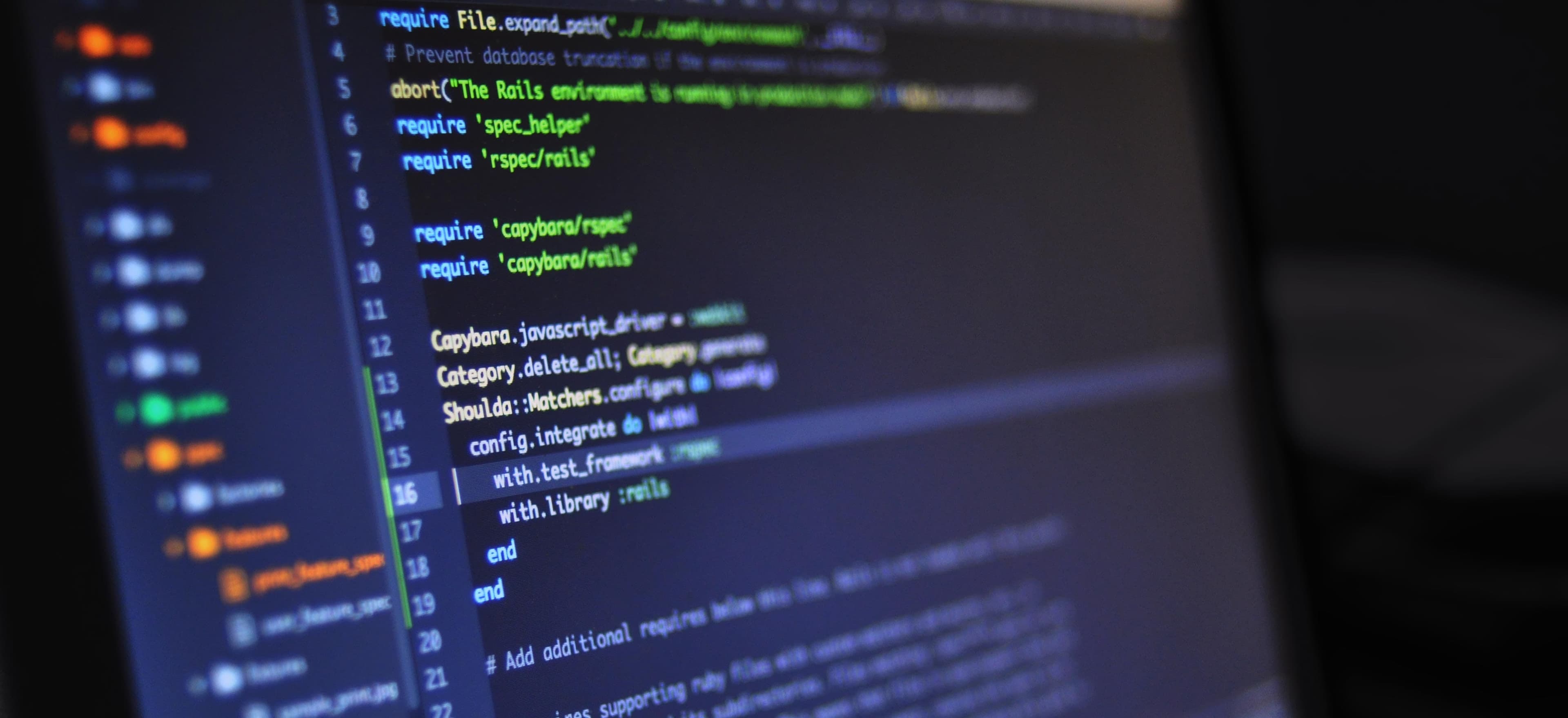
- Published on
Mastering Backpressure in Reactive Streams with Java
Backpressure is a crucial concept in Reactive Programming, particularly in Java. It addresses the challenge of controlling the flow of data between producers and consumers in a way that prevents overwhelming the consumer. With the increasing adoption of asynchronous programming, understanding how to manage backpressure is essential for building robust applications.
In this blog post, we will explore the mechanisms of backpressure in Reactive Streams using Java, focusing on key libraries such as Project Reactor and RxJava. We'll discuss why backpressure is important, how to implement it, and provide code snippets to illustrate these concepts effectively.
Table of Contents
What is Backpressure?
Backpressure is a strategy to handle situations where a data producer generates data more quickly than a consumer can process it. In a reactive system, this can lead to data loss, increased latency, or even application crashes.
The principle behind backpressure is simple: it allows the consumer to signal the producer about its capacity to process data, effectively applying a form of flow control.
Example Scenario
Consider a web scraper that collects URLs from a website and processes them. If the scraper retrieves URLs at a rapid rate, but the processing of each URL is slow, the system can get overwhelmed. Backpressure provides a solution to manage this discrepancy.
Why is Backpressure Important?
Backpressure serves several critical roles in reactive applications:
- Resource Management: By controlling data flow, backpressure helps manage system resources effectively.
- Scalability: Applications can handle varying data loads without crashing, leading to improved scalability and reliability.
- Latency Reduction: It helps avoid backlogs which could increase response times.
To gain a deeper insight into the benefits of reactive programming, visit Reactive Manifesto.
Implementing Backpressure in Java
Using Project Reactor
Project Reactor is a widely used library for building reactive applications in Java. It provides built-in support for backpressure through its core types: Mono
and Flux
.
Basic Backpressure Handling
Here is a simple example using Flux
with backpressure handling:
import reactor.core.publisher.Flux;
public class BackpressureExample {
public static void main(String[] args) {
Flux.range(1, 100)
.onBackpressureBuffer(10) // Buffering strategy
.subscribe(
item -> {
try {
// Simulate processing time
Thread.sleep(100);
System.out.println("Processed: " + item);
} catch (InterruptedException e) {
e.printStackTrace();
}
},
error -> System.err.println("Error: " + error),
() -> System.out.println("Completed")
);
}
}
Code Commentary
- Flux.range(1, 100): Generates a range of integers from 1 to 100.
- onBackpressureBuffer(10): Here, we tell the system to buffer up to 10 items if the consumer is not ready to process immediately.
- Thread.sleep(100): Simulates a time-consuming processing task, allowing us to observe the effects of backpressure.
This implementation demonstrates backpressure beautifully. The producer generates items, but if the subscriber (consumer) cannot keep up, the system buffers items up to a limit.
Using RxJava
RxJava is another prevalent reactive programming library in Java. It handles backpressure through the Flowable
type.
Backpressure in RxJava
Here is an example of how to implement backpressure using RxJava:
import io.reactivex.Flowable;
public class RxJavaBackpressureExample {
public static void main(String[] args) {
Flowable.range(1, 100)
.onBackpressureBuffer(10)
.subscribe(
item -> {
try {
// Simulate processing time
Thread.sleep(100);
System.out.println("Processed: " + item);
} catch (InterruptedException e) {
e.printStackTrace();
}
},
error -> System.err.println("Error: " + error),
() -> System.out.println("Completed")
);
}
}
Code Commentary
- The principles are similar to those in Project Reactor. A
Flowable
is created to generate a range of values. - onBackpressureBuffer(10): This strategy tells the
Flowable
to buffer items until the consumer is ready. - The sleep method mimics time-consuming tasks, showcasing how buffering works under load.
In Conclusion, Here is What Matters
Mastering backpressure in reactive streams is essential for creating reliable and scalable applications. As we have seen, both Project Reactor and RxJava provide effective tools for implementing backpressure strategies, allowing developers to manage the flow of data between producers and consumers efficiently.
By adopting reactive programming and backpressure management, your applications will not only perform better but also respond gracefully to varying data loads.
For more information on reactive programming and its principles, check out ReactiveX, which provides resources and tutorials to get started with reactive programming.
Feel free to leave your thoughts, questions, or insights in the comments below. Happy coding!
Checkout our other articles