Common Pitfalls When Implementing WebSockets in Spring Boot
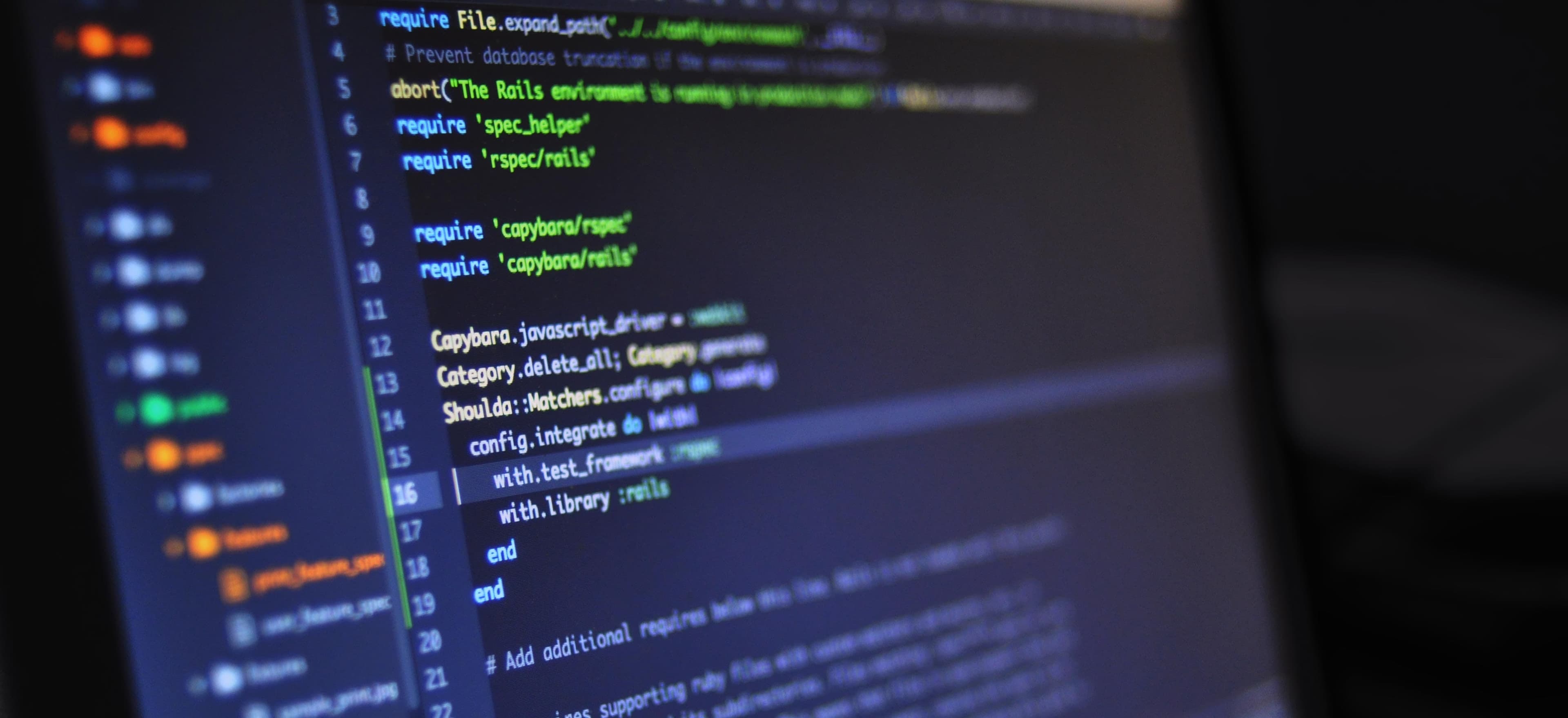
- Published on
Common Pitfalls When Implementing WebSockets in Spring Boot
WebSockets have become a dominant solution for real-time communication in web applications. They offer a two-way, full-duplex communication channel over a single, long-lived connection. Spring Boot, with its robust capabilities, provides support for WebSockets, making it easier to implement real-time features. However, there are common pitfalls developers encounter when integrating WebSockets into their Spring Boot applications. In this blog post, we will explore these pitfalls, along with code snippets and best practices to ensure smooth implementation.
Understanding WebSockets
Before diving into common pitfalls, let's take a moment to understand what WebSockets are. Unlike traditional HTTP requests where clients and servers communicate in a request-response fashion, WebSockets allow for persistent connections. This means both the client and the server can send messages independently. This is particularly useful in scenarios like chat applications, live notifications, and real-time data feeds.
Setting Up WebSockets in Spring Boot
To implement WebSockets in Spring Boot, you'll generally start by adding the necessary dependencies. Here’s a basic dependency required in your pom.xml
file if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-websocket</artifactId>
</dependency>
In your Spring Boot application, you can enable WebSocket support by creating a configuration class.
import org.springframework.context.annotation.Configuration;
import org.springframework.web.socket.config.annotation.EnableWebSocketMessageBroker;
import org.springframework.web.socket.config.annotation.StompEndpointRegistry;
import org.springframework.web.socket.config.annotation.WebSocketMessageBrokerConfigurer;
@Configuration
@EnableWebSocketMessageBroker
public class WebSocketConfig implements WebSocketMessageBrokerConfigurer {
@Override
public void configureMessageBroker(MessageBrokerRegistry config) {
config.enableSimpleBroker("/topic");
config.setApplicationDestinationPrefixes("/app");
}
@Override
public void registerStompEndpoints(StompEndpointRegistry registry) {
registry.addEndpoint("/ws").withSockJS();
}
}
Pitfall 1: Not Configuring STOMP Endpoints Properly
One common mistake developers make is inadequately configuring STOMP (Simple Text Oriented Messaging Protocol) endpoints. STOMP adds a layer of client-side application protocol on top of WebSockets.
For instance, if you forget to register the STOMP endpoints in your configuration, your frontend JavaScript will fail to communicate.
registry.addEndpoint("/ws").withSockJS();
Ensure that this line exists; otherwise, clients will not be able to connect to your WebSocket server.
Pitfall 2: Ignoring Connection Events
Not handling connection events (i.e., connecting, disconnecting, and error handling) can lead to a poor user experience. Users expect real-time applications to respond appropriately when connections drop or fail to establish.
You can easily manage connection events in your JavaScript code:
const socket = new SockJS('/ws');
const stompClient = Stomp.over(socket);
stompClient.connect({}, function(frame) {
console.log('Connected: ' + frame);
}, function(error) {
console.log('Connection Error: ' + error);
});
In the above code, the stompClient.connect
method includes a callback for connection error handling. Ignoring these callbacks may result in clients not being alerted if connections fail or if there are issues during communication.
Pitfall 3: Not Handling Sessions Properly
WebSockets operate over a persistent connection, which may cause session handling issues if not configured properly. Each WebSocket connection doesn't automatically share HTTP session information. Hence, you may lose user context or authentication tokens.
To share sessions, you can use Spring's built-in mechanisms to manage users:
@MessageMapping("/chat")
public void handleChat(Message message, Principal principal) {
messageService.sendMessage(principal.getName(), message);
}
In this example, the Principal
interface is used to retrieve the authenticated user. Proper session management is critical for applications requiring user authentication.
Pitfall 4: Not Validating User Input
Security is paramount, especially in real-time web applications. One pitfall is the failure to validate user input effectively. Treating WebSocket messages similarly to HTTP requests is essential.
Consider a message handling method that looks like this:
@MessageMapping("/chat")
public void handleChat(Message message) {
// Message processing...
}
Before processing message
, ensure you validate its contents to prevent injection attacks or other malicious actions.
if (message.getContent() == null || message.getContent().isEmpty()) {
throw new IllegalArgumentException("Message content cannot be empty");
}
Pitfall 5: Not Scaling WebSocket Servers Properly
When developing applications with WebSockets, especially those expected to handle high loads, it's crucial to consider scalability. If you only run your application on a single server, client connections may be limited.
Using Spring Boot's built-in support with an appropriate message broker like RabbitMQ or ActiveMQ can help scale your WebSocket connections.
Consider integrating RabbitMQ for a more resilient solution:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-amqp</artifactId>
</dependency>
This will enable better handling of messages between multiple instances of your WebSocket servers.
Key Takeaways
Implementing WebSockets in Spring Boot can be a rewarding journey, leading to improved real-time communication in your applications. However, it comes with its set of challenges and pitfalls. By paying attention to proper endpoint configuration, connection handling, session management, input validation, and scalability, you can avoid common issues when developing real-time applications.
For more resources on Spring WebSockets, consider checking out the official Spring WebSocket documentation and Spring Boot Documentation.
In closing, take the time to understand and test your WebSocket implementation thoroughly. With thoughtful consideration and diligent practices, you can create a robust application capable of meeting real-time user demands efficiently.
Checkout our other articles