Mastering the Fork-Join Framework: Avoiding Common Pitfalls
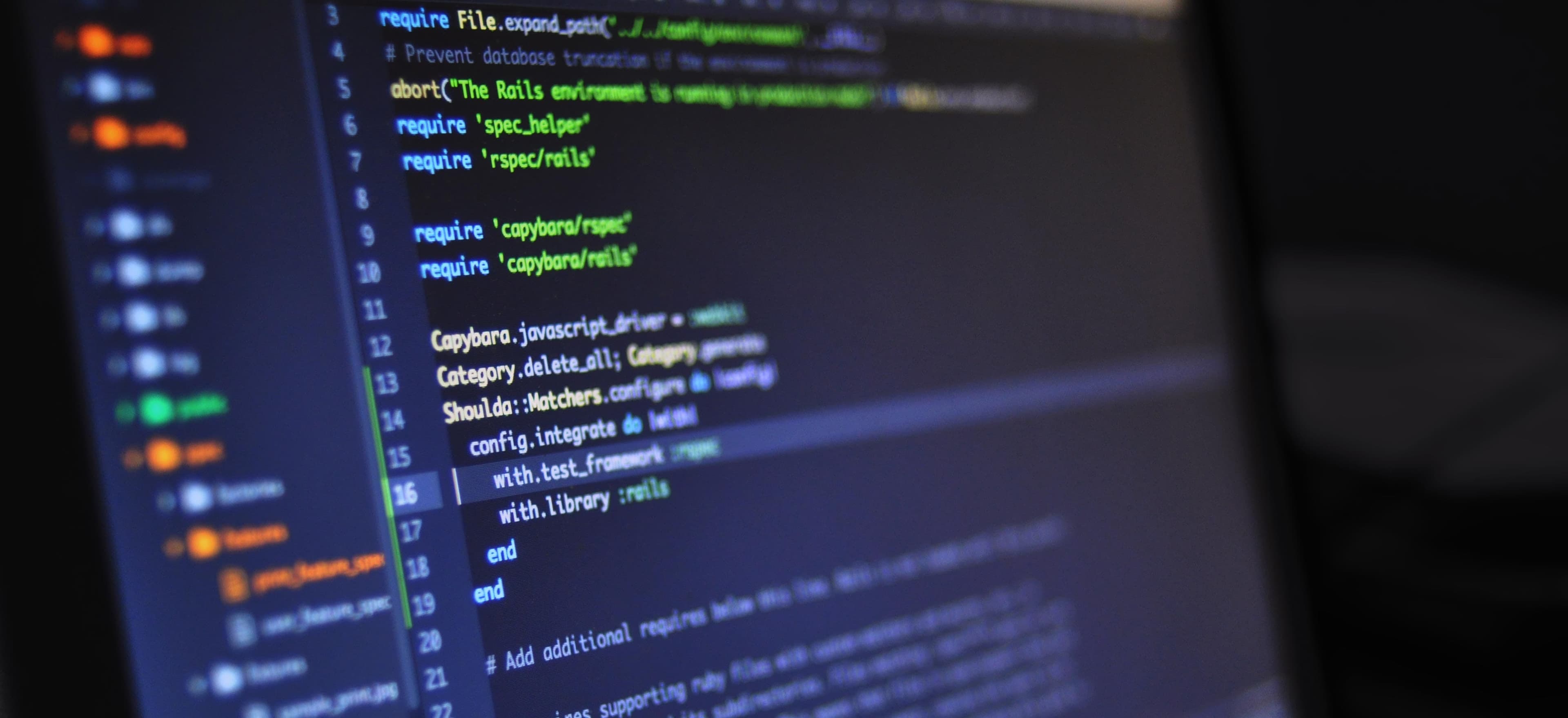
- Published on
Mastering the Fork-Join Framework: Avoiding Common Pitfalls
The Fork-Join Framework is an essential tool in Java for parallel programming, particularly when working with tasks that can be broken down into smaller, independent subtasks. Introduced in Java 7 as part of the java.util.concurrent
package, this framework allows developers to take full advantage of multi-core processors, leading to more efficient execution of code. However, as with any powerful tool, it’s important to use it wisely to avoid common pitfalls.
In this blog post, we will explore the Fork-Join Framework, why it is beneficial, and the common mistakes developers make while using it. We will present best practices along with code snippets to ensure you can harness the true power of this framework.
Understanding the Fork-Join Framework
The Fork-Join Framework is designed for a divide-and-conquer algorithm. This means it recursively splits a task into smaller sub-tasks, processes them in parallel, and combines the results. This is particularly useful for operations requiring significant computation, such as sorting large arrays or performing complex mathematical calculations.
Key Components
- ForkJoinPool: This is the core component responsible for managing threads and executing ForkJoinTasks.
- ForkJoinTask: This is an abstract class that represents a task that can be forked and joined. You can extend it via
RecursiveTask
(for tasks that return a result) orRecursiveAction
(for tasks that do not).
Why Use the Fork-Join Framework?
- Efficient Resource Utilization: By utilizing all available CPU cores, it significantly reduces execution time for large tasks.
- Simplified Parallelism: It provides a straightforward way to implement parallelism without dealing with complex thread management.
Common Pitfalls and How to Avoid Them
1. Overhead of Task Splitting
The Pitfall: Splitting tasks into small pieces can introduce overhead that outweighs the benefits of parallel execution. When tasks are too granular, the cost of managing many small tasks can contribute to longer run times.
Solution: Use a proper threshold for splitting tasks. Implement a threshold based on the size of the input.
Example Code Snippet
import java.util.concurrent.RecursiveTask;
public class SumTask extends RecursiveTask<Long> {
private static final int THRESHOLD = 1000;
private final long[] data;
private final int start;
private final int end;
public SumTask(long[] data, int start, int end) {
this.data = data;
this.start = start;
this.end = end;
}
@Override
protected Long compute() {
if (end - start <= THRESHOLD) {
// Process the data directly if the size is below the threshold
long sum = 0;
for (int i = start; i < end; i++) {
sum += data[i];
}
return sum;
} else {
// Split the task into two subtasks
int mid = (start + end) / 2;
SumTask leftTask = new SumTask(data, start, mid);
SumTask rightTask = new SumTask(data, mid, end);
leftTask.fork(); // Begin executing the left task asynchronously
return rightTask.compute() + leftTask.join(); // Process right task and combine results
}
}
}
2. Blocking Operations
The Pitfall: Using blocking I/O operations within tasks can severely affect performance by preventing other threads from proceeding.
Solution: Be cautious about blocking calls within your tasks. Where possible, use non-blocking operations or ensure that blocking calls are made within smaller tasks or outside the Fork-Join framework.
Example Code Snippet
public class NetworkTask extends RecursiveAction {
@Override
protected void compute() {
// Avoid implementing blocking operations directly in ForkJoinTask
// Instead, break the logic into smaller pieces if blocking is necessary
try {
// Simulate a network call
String response = makeNetworkCall();
} catch (Exception e) {
e.printStackTrace();
}
}
private String makeNetworkCall() {
// Simulating a blocking I/O
return "Data from network";
}
}
3. Inefficient Merging of Results
The Pitfall: Merging results inefficiently can negate the benefits of parallelism. Poor merge implementations can lead to high computational costs.
Solution: Optimize the merge logic to ensure it is lightweight.
Example Code Snippet
@Override
protected Long reduce(Long result1, Long result2) {
// Efficiently merge results
return result1 + result2;
}
4. Not Handling Exceptions Properly
The Pitfall: Exceptions thrown during the execution of tasks can lead to unpredictable behavior. A new thread is created for each task, and if an exception occurs, it may not propagate as expected.
Solution: Use Future.get()
to retrieve results and exceptions.
Example Code Snippet
ForkJoinPool pool = new ForkJoinPool();
SumTask task = new SumTask(data, 0, data.length);
pool.submit(task);
try {
Long result = task.get(); // Retrieves result or throws exception
} catch (Exception e) {
e.printStackTrace(); // Handle the exception
}
5. Not Tuning the ForkJoinPool
The Pitfall: The default configuration of ForkJoinPool
might not suit all applications. Not tuning it might lead to subpar performance.
Solution: Specify the parallelism level and consider utilizing other configurations such as setAsyncMode()
.
Example Code Snippet
ForkJoinPool customPool = new ForkJoinPool(8); // Pool size based on the number of available processors
customPool.submit(new SumTask(data, 0, data.length));
The Closing Argument
Mastering the Fork-Join Framework in Java opens up immense potential for building high-performance parallel applications. Understanding its architecture, benefits, and common pitfalls is crucial for effective usage. By keeping an eye on how tasks are split, merge results efficiently, and handle exceptions properly, you can leverage this powerful framework to its fullest extent.
For more detailed insights, check out these resources:
- Oracle Documentation on Fork-Join Framework
- Java Concurrency in Practice
By implementing these practices, you can ensure a smoother experience while maximizing the performance of your parallel applications. Happy coding!
Checkout our other articles