Mastering CompletableFuture: Tackling Callback Hell in Java
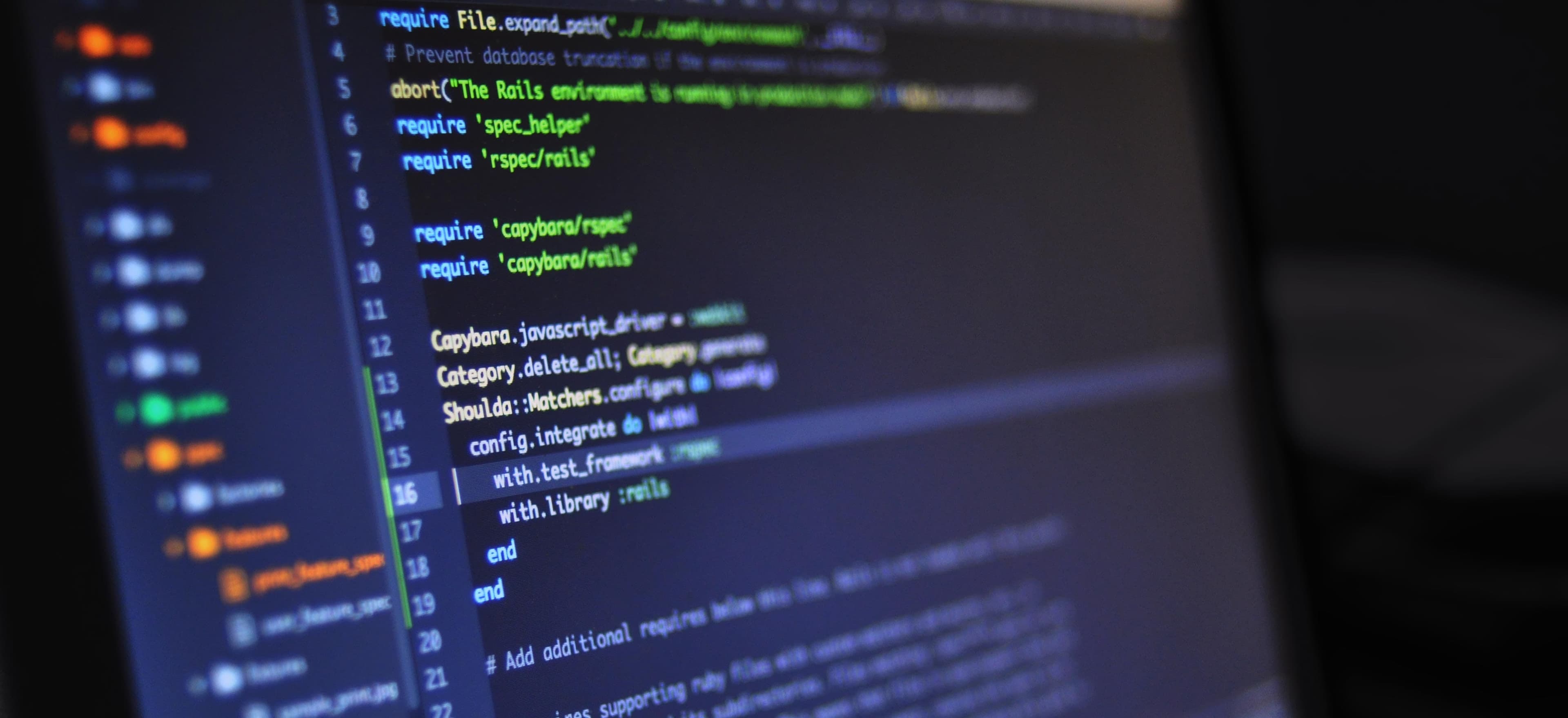
- Published on
Mastering CompletableFuture: Tackling Callback Hell in Java
Java has evolved tremendously since its inception. By introducing features that enhance concurrency and simplify asynchronous programming, it has become easier for developers to create high-performance applications. One such feature is the CompletableFuture
. In this blog post, we'll dive deep into mastering CompletableFuture
, exploring its capabilities, advantages, and how it effectively tackles the notorious callback hell.
Understanding CompletableFuture
A CompletableFuture
represents a future result of an asynchronous computation. It not only represents a value that may not yet exist but also allows the execution of actions once the computation is complete. From its initial release in Java 8, it has empowered developers to write cleaner, more manageable code in scenarios that involve asynchronous programming.
Why Use CompletableFuture?
- Non-blocking: Unlike traditional blocking calls which can lead to performance bottlenecks,
CompletableFuture
allows tasks to run asynchronously. - Composability: You can define a sequence of dependent asynchronous tasks that are easy to read and maintain.
- Error Handling: It provides a fluent way to handle exceptions and complete tasks even in the event of failure.
- Thread Management: It integrates seamlessly with Java's Executor framework, allowing better control over thread management.
The Problem of Callback Hell
Callback hell occurs when multiple nested callbacks lead to code that is hard to read and maintain. For example, a common scenario in Java is when callbacks are used in asynchronous operations like reading files, making HTTP requests, or querying databases. This can create deeply nested structures that are difficult to follow.
Consider the following example that depicts callback hell with traditional approaches:
public void loadData() {
fetchDataFromDatabase((data) -> {
processData(data, (processedData) -> {
sendDataToClient(processedData, (response) -> {
System.out.println("Response sent: " + response);
});
});
});
}
public void fetchDataFromDatabase(DatabaseCallback callback) {
// simulate async database fetching
}
public void processData(String data, ProcessCallback callback) {
// simulate async data processing
}
public void sendDataToClient(String data, ClientCallback callback) {
// simulate async response sending
}
While this works, the readability and maintainability quickly diminish as the number of callbacks increases.
Using CompletableFuture to Simplify Asynchronous Code
By leveraging CompletableFuture
, we can transform the callback structure into a more intuitive and readable format. Let's reimagine the previous example using CompletableFuture
.
Refactoring with CompletableFuture
First, ensure you import the required classes:
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.ExecutionException;
Now, let's rewrite the previous example:
public CompletableFuture<String> loadData() {
return fetchDataFromDatabase()
.thenCompose(this::processData)
.thenCompose(this::sendDataToClient);
}
public CompletableFuture<String> fetchDataFromDatabase() {
return CompletableFuture.supplyAsync(() -> {
// simulate async database fetching
return "data from database";
});
}
public CompletableFuture<String> processData(String data) {
return CompletableFuture.supplyAsync(() -> {
// simulate async data processing
return "processed " + data;
});
}
public CompletableFuture<String> sendDataToClient(String data) {
return CompletableFuture.supplyAsync(() -> {
// simulate async response sending
return "Response sent: " + data;
});
}
Key Improvements
- Readability: Each asynchronous operation is represented as a
CompletableFuture
. The use ofthenCompose
makes the chain of operations clear. - Error Handling: Instead of convoluted error checking for each callback, you can chain error handling using
exceptionally
orhandle
. - Maintainability: Adding or modifying operations can be done easily due to the linear structure.
Now let’s delve into how we can handle exceptions efficiently within this structure.
Error Handling with CompletableFuture
Error handling in asynchronous programming is crucial to avoid unexpected crashes. The CompletableFuture
API provides simple ways to manage errors.
For example:
public CompletableFuture<String> loadData() {
return fetchDataFromDatabase()
.thenCompose(this::processData)
.thenCompose(this::sendDataToClient)
.exceptionally(ex -> {
// logging the error and handling it
System.err.println("Error occurred: " + ex.getMessage());
return "Default Response"; // or any default handling
});
}
When to Use exceptionally vs. handle
- exceptionally: Use this when you want to handle exceptions and produce a different result without any recovery in context. It only processes Exception cases.
- handle: Use this for both result and exception processing. It allows you to handle a successful outcome or an exception and produce a result accordingly.
Running Multiple CompletableFutures
Often in real-world applications, you may need to run multiple tasks in parallel. CompletableFuture
shines here as well with methods like allOf()
and anyOf()
.
Running Tasks in Parallel
public CompletableFuture<Void> loadDataInParallel() {
CompletableFuture<String> dbFuture = fetchDataFromDatabase();
CompletableFuture<String> apiFuture = fetchDataFromApi();
return CompletableFuture.allOf(dbFuture, apiFuture)
.thenAccept(v -> {
System.out.println("Fetched from DB: " + dbFuture.join());
System.out.println("Fetched from API: " + apiFuture.join());
});
}
Explanation
In this snippet, fetchDataFromApi()
is another asynchronous method fetching data from an API. We use allOf()
to wait until both futures are complete. Once completed, we can extract the values and process them as needed.
Final Thoughts
CompletableFuture is a powerful tool that provides a cleaner and more manageable approach to asynchronous programming in Java. It combats the callback hell by providing a fluent API, enhancing readability, maintainability, and making error handling simpler.
By leveraging the potentials of CompletableFuture, you can write asynchronous code that is not just functional but also elegant. As you continue your journey in mastering Java, pairing your knowledge of CompletableFuture with other modern techniques will enable you to build robust and efficient applications.
For those who want to dig deeper into CompletableFuture capabilities, consider exploring Oracle’s official documentation or experimenting with various asynchronous patterns.
Happy coding!
Checkout our other articles