Avoiding Deadlocks: Essential Concurrency Design Patterns
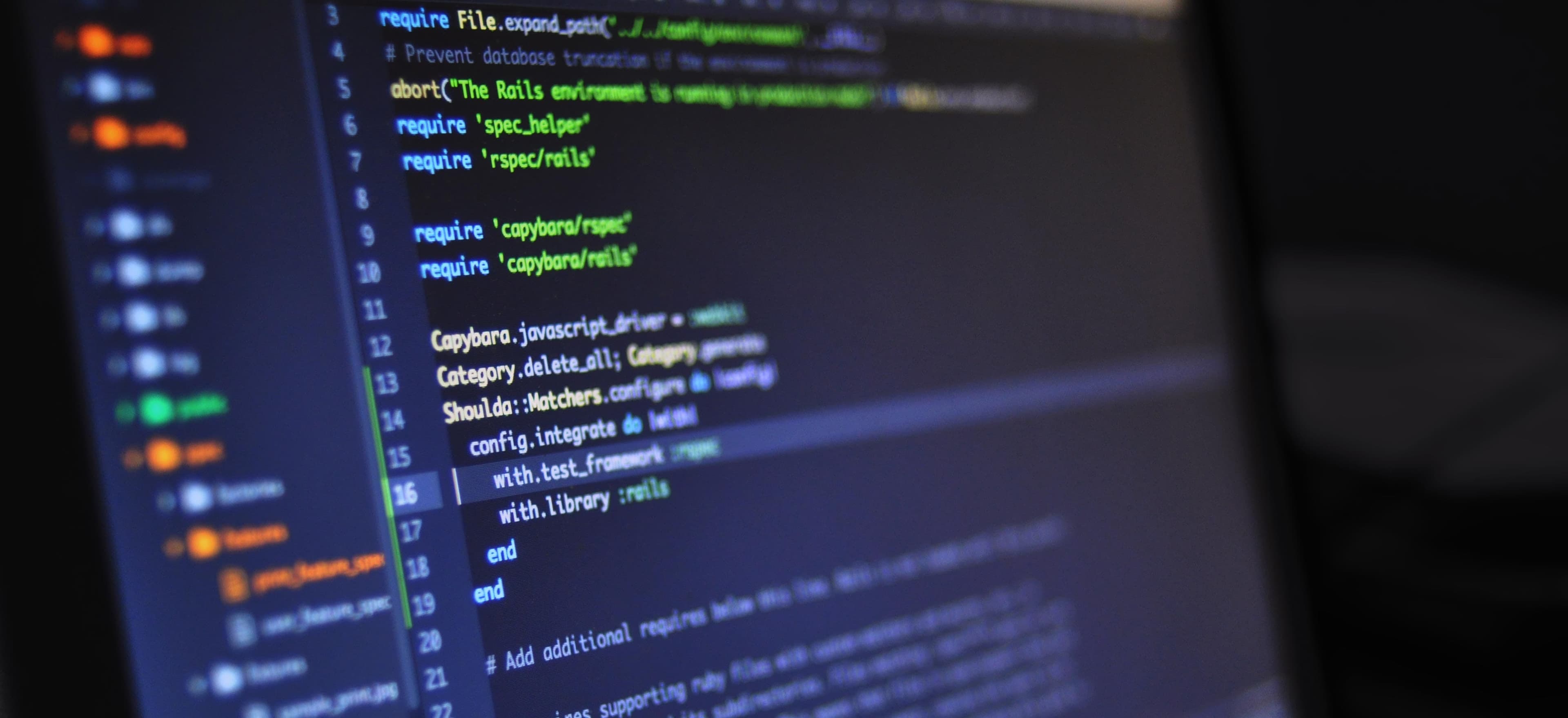
- Published on
Avoiding Deadlocks: Essential Concurrency Design Patterns in Java
Concurrency is one of the most striking features of modern programming languages, including Java. It allows developers to write programs that can perform multiple operations simultaneously, which can lead to significant performance improvements. However, with the power of concurrency comes the risk of creating deadlocks. Consequently, understanding how to design concurrent applications to avoid deadlocks is crucial for Java developers.
In this blog post, we will discuss essential concurrency design patterns in Java that can help prevent deadlocks. We will delve into what deadlocks are, explore concurrency design patterns, and provide relevant code snippets to illustrate these concepts.
What is a Deadlock?
A deadlock is a situation in a multitasking or multi-threading environment where two or more threads are blocked forever, waiting for each other to release resources. This scenario usually occurs when threads acquire multiple locks in an inconsistent order.
Real-World Analogy
Imagine two cars at an intersection, each waiting for the other to move. Since neither can proceed, they remain stuck indefinitely. Similarly, in programming, if Thread A holds Lock 1 and waits for Lock 2 while Thread B holds Lock 2 and waits for Lock 1, a deadlock occurs.
The Four Necessary Conditions for Deadlock
For a deadlock to happen, the following four conditions must hold simultaneously:
- Mutual Exclusion: Resources cannot be shared.
- Hold and Wait: Processes holding resources are waiting for additional resources.
- No Preemption: Resources cannot be forcibly taken from a thread.
- Circular Wait: There exists a circular chain of threads, each waiting for a resource held by the next thread in the chain.
Recognizing these conditions can guide developers in avoiding deadlocks through careful design.
Essential Concurrency Design Patterns to Avoid Deadlocks
1. Lock Ordering
One effective way to prevent deadlocks is by establishing a global order in which locks should be acquired. Regardless of the individual thread logic, they will all follow the same order while acquiring resources.
Code Example: Lock Ordering
class LockManager {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void threadA() {
synchronized (lock1) {
System.out.println("Thread A acquired Lock 1");
synchronized (lock2) {
System.out.println("Thread A acquired Lock 2");
}
}
}
public void threadB() {
synchronized (lock1) {
System.out.println("Thread B acquired Lock 1");
synchronized (lock2) {
System.out.println("Thread B acquired Lock 2");
}
}
}
}
In the example above, both threadA
and threadB
acquire lock1
before lock2
. By ensuring all threads request locks in a consistent order, we eliminate circular wait.
2. Timeout Locking
In some scenarios, using a time-out mechanism can be an effective strategy. Threads attempt to acquire locks but will only wait for a specified period before giving up. This approach allows threads to continue executing without getting stuck indefinitely.
Code Example: Timeout Locking
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class TimeoutLockExample {
private final Lock lock1 = new ReentrantLock();
private final Lock lock2 = new ReentrantLock();
public void threadC() {
try {
if (lock1.tryLock(1000, TimeUnit.MILLISECONDS)) {
try {
// Simulate work with Lock 1
System.out.println("Thread C acquired Lock 1");
if (lock2.tryLock(1000, TimeUnit.MILLISECONDS)) {
try {
// Simulate work with Lock 2
System.out.println("Thread C acquired Lock 2");
} finally {
lock2.unlock();
}
}
} finally {
lock1.unlock();
}
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
}
In the above example, if threadC
fails to acquire lock2
within the specified timeout period, it will simply bypass that resource and unlock any held locks. This helps to prevent threads from being deadlocked.
3. Using a Single Lock
Sometimes, it makes sense to encapsulate shared resources within a single lock. By restricting the locking mechanism to a single object, you naturally reduce the potential for deadlocks.
Code Example: Single Lock Approach
class SingleLockExample {
private final Object lock = new Object();
public void sharedResourceMethod() {
synchronized (lock) {
// Access shared resources safely
System.out.println("Accessing shared resource");
}
}
}
While this approach limits concurrency to an extent, it ensures that as long as the resource is accessed through the singular lock, the risk of deadlock is virtually nonexistent.
4. Deadlock Detection and Recovery
Although prevention is the ideal approach, sometimes it is worth implementing deadlock detection mechanisms and having a recovery strategy. This method involves periodically checking for deadlocks and taking corrective actions, such as terminating one of the threads.
For a practical implementation of this concept, consider using a dedicated thread that monitors other threads for potential deadlocks.
5. Resource Allocation Graph
Using resource allocation graphs (RAG) can help visualize the resources and their dependencies. By constructing a graph with nodes as threads and resources, you can identify cycles that indicate potential deadlocks. Once detected, you can alter resource management dynamically.
Closing Remarks
Understanding deadlocks and employing effective concurrency design patterns is crucial for Java developers. Deadlocks can lead to significant performance degradation and application crashes, adversely impacting user experience.
Using strategies like lock ordering, timeout locking, single locks, and deadlock detection will help you design robust concurrent applications.
For more detailed guidance on managing concurrency in Java, you can check the following resources:
By applying these essential patterns, not only will you avoid deadlocks, but you’ll also enhance the efficiency and reliability of your Java applications. Happy coding!
Checkout our other articles