Understanding JVM Memory Issues: Common Pitfalls and Solutions
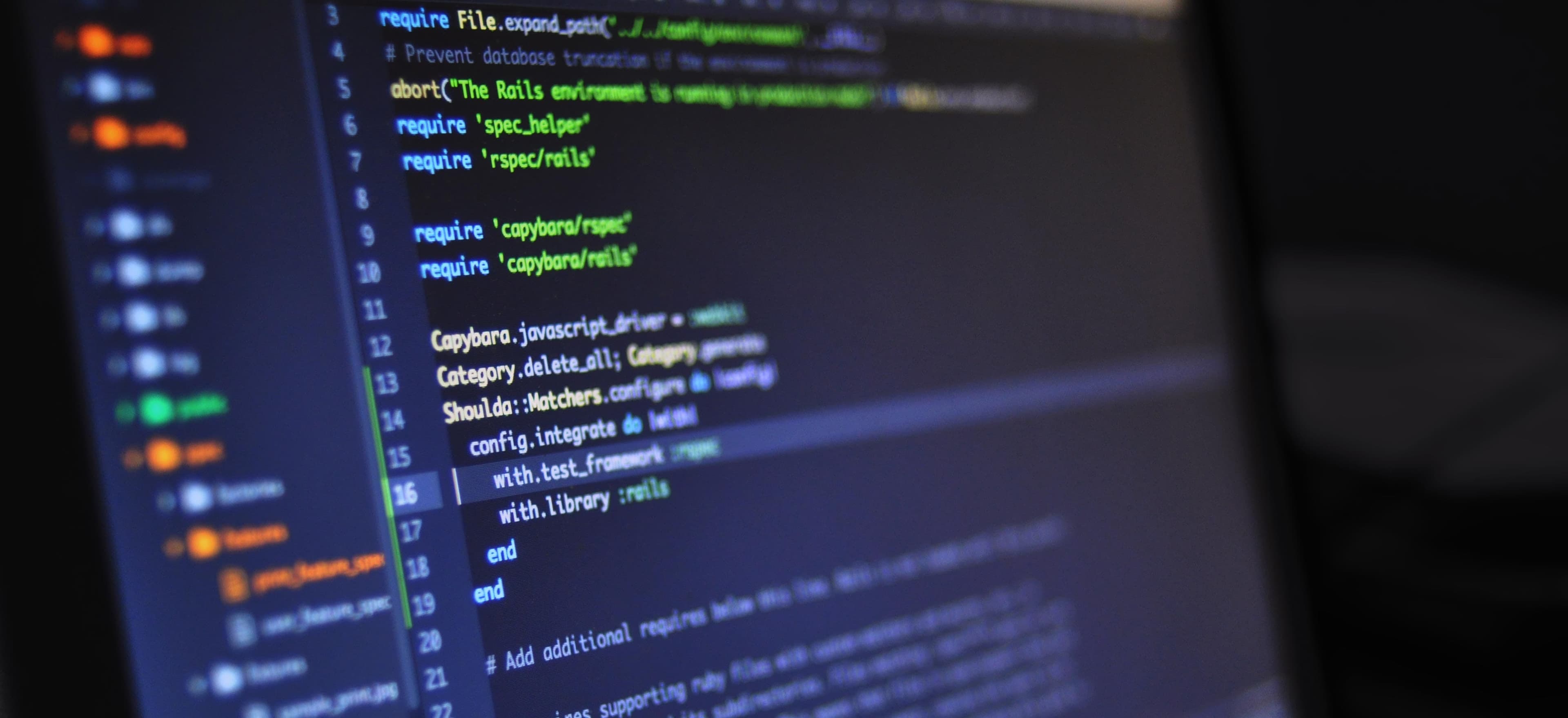
- Published on
Understanding JVM Memory Issues: Common Pitfalls and Solutions
Java is a platform that has become ubiquitous in modern software development. Much of its versatility and effectiveness comes from the Java Virtual Machine (JVM), which abstracts the hardware complexities. However, memory issues can plague even the most seasoned Java developers. Understanding these problems and their solutions is crucial for efficient Java application performance.
What Is JVM Memory Management?
The JVM manages memory through a combination of systems, primarily heap and stack memory. The heap is where all the object instances are created, while the stack is where method calls and local variables are stored.
Key Concepts:
- Heap Space: Used for dynamic memory allocation for Java objects and JRE classes.
- Stack Space: Each thread has its own stack, which stores local variables and stores references to objects in the heap.
- Garbage Collection: The process of automatically freeing memory by removing objects that are no longer in use.
Common JVM Memory Issues
Let's delve into some common JVM memory issues that developers may encounter:
1. OutOfMemoryError
An OutOfMemoryError
occurs when the JVM cannot allocate an object because it is out of memory. This error can manifest in different forms:
- Java heap space: Insufficient heap size to accommodate new objects.
- GC overhead limit exceeded: Too much time spent performing garbage collection with minimal success.
- Metaspace: Out of native memory used for JVM class metadata.
Code Example
public class MemoryLeakExample {
private static List<Object> objects = new ArrayList<>();
public static void main(String[] args) {
while (true) {
objects.add(new Object()); // Continuously adding objects will eventually fill the heap space.
}
}
}
Why This Matters: The above code illustrates a common memory leak where the list continues to grow indefinitely, leading to OutOfMemoryError
. Always ensure that you release large collections or avoid infinite reference retention.
Solution
- Analyze heap dumps using tools like Eclipse Memory Analyzer to identify memory leaks.
- Refactor code to eliminate unnecessary object references.
2. High Garbage Collection Overhead
Garbage collection (GC) should manage memory efficiently, but sometimes, it can take up too much CPU time, exhibiting "GC thrashing." You might notice performance degradation or slow application response times.
Code Example
public class GCOverheadExample {
public static void main(String[] args) {
List<Double> numbers = new ArrayList<>();
while (true) {
for (int i = 0; i < 1_000_000; i++) {
numbers.add(Math.random());
}
numbers.clear(); // Clearing the list for garbage collection.
}
}
}
Why This Matters: GC overhead happens when there is excessive object creation and deletion. In this example, the program generates vast numbers of Double
objects in a loop, leading to constant GC activity.
Solution
- Tune JVM parameters to manage heap size and GC behavior. For example, increasing the heap size via the
-Xmx
flag. - Use
ConcurrentMarkSweep
orG1 GC
collectors that are optimized for throughput and can manage memory more efficiently.
3. StackOverflowError
This error is no stranger to Java developers. It occurs due to the stack memory being exhausted, typically from excessively deep recursion or uncontrolled object instantiation.
Code Example
public class StackOverflowExample {
public static void recursiveMethod() {
recursiveMethod(); // A recursive method without a base case.
}
public static void main(String[] args) {
recursiveMethod();
}
}
Why This Matters: Without a proper base case, recursion can spiral out of control, leading to a StackOverflowError
. Recursive algorithms must always have terminating conditions.
Solution
- Refactor recursive methods to use iterative solutions where feasible.
- If recursion is necessary, ensure that it terminates correctly to avoid stack overflow.
Profiling and Monitoring JVM Memory
To confront these JVM memory issues effectively, one must not only understand them but also monitor and profile applications.
Tools for Profiling
Several tools can help you monitor and analyze your Java application's memory usage:
- VisualVM: Great for monitoring memory and CPU usage along with GC details.
- Java Mission Control: A commercial but powerful tool for monitoring in production environments.
Runtime Parameters for Monitoring
You can use JVM flags to give you insights into memory usage:
-XX:+HeapDumpOnOutOfMemoryError
: Generates a heap dump when anOutOfMemoryError
occurs.-XX:+PrintGCDetails
: Provides detailed output on every garbage collection, allowing better analysis.
Best Practices for Managing JVM Memory
Now that we've identified common pitfalls and solutions, here are some best practices that can help in effective JVM memory management:
- Always Keep JVM Updated: Java regularly introduces improvements in memory management and performance.
- Use Strong vs Weak References: Appropriate reference types help mitigate memory leaks. Use
WeakReference
for objects that can be garbage collected. - Optimize Data Structures: Choose the right data structures to minimize memory footprint. For instance, prefer
ArrayList
overLinkedList
for scenarios where random access is required. - Use Object Pools: If object creation is frequent, consider implementing an object pool to limit unnecessary allocations.
- Test Under Realistic Load: Simulate the production load while testing memory performance to identify potential leaks or issues.
My Closing Thoughts on the Matter
Understanding JVM memory management is pivotal in the software development lifecycle. By recognizing common pitfalls, applying best practices, and utilizing monitoring tools, you can ensure optimal application performance.
The JVM is robust, but even the best systems require vigilant monitoring and occasional refinements. As you advance your Java development skills, make memory management a core area of your expertise—your applications will thank you for it.
For additional reading on this topic, you can check out the official Oracle Java documentation, which covers JVM memory management in great detail. Remember—knowledge is power in the realm of software development!
Checkout our other articles