Common Pitfalls When Using Java Agents: Avoid These Mistakes
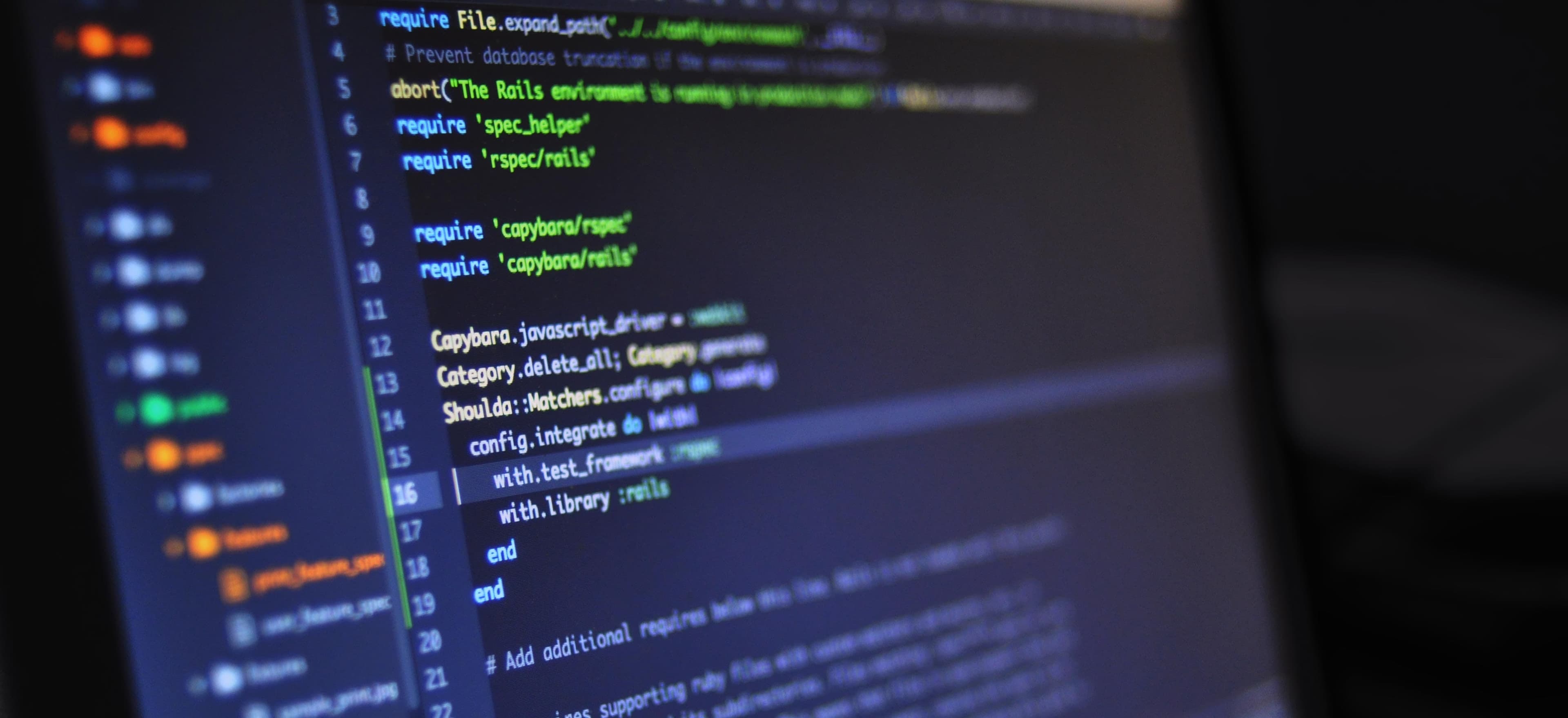
- Published on
Common Pitfalls When Using Java Agents: Avoid These Mistakes
Java agents are powerful tools for modifying the behavior of Java applications at runtime. They enable developers to implement aspects like monitoring, profiling, and instrumentation with relative ease. However, using them comes with its set of challenges and potential pitfalls. This blog post will explore common mistakes developers make when using Java agents and provide solutions to help you avoid them.
Understanding Java Agents
Before diving into the pitfalls, let's clarify what a Java agent is. A Java agent is a special type of Java program that can manipulate the bytecode of Java classes at runtime. This is achieved through the Java Instrumentation API. Java agents can be invoked by the Java Virtual Machine (JVM) at startup or dynamically at runtime.
Key Features of Java Agents:
- Bytecode Manipulation: Modify existing classes or add new ones.
- Event Monitoring: Capture execution data for monitoring performance.
- Dynamic Injection: Inject code into running Java applications.
For more detailed information about Java agents, you can visit the official Java Documentation.
Common Pitfalls in Using Java Agents
1. Ignoring ClassLoader Context
One of the most common pitfalls is misunderstanding the context in which your Java agent operates, particularly the ClassLoader. Java applications may use custom ClassLoaders that affect the visibility of classes.
Mistake: Failing to account for the ClassLoader leads to ClassNotFoundException
or NoClassDefFoundError
.
Solution: Always be mindful of the ClassLoader hierarchy. Use the appropriate ClassLoader for current operations.
Example Code:
ClassLoader classLoader = MyClass.class.getClassLoader();
Class<?> myClass = classLoader.loadClass("com.example.MyClass");
Why this matters: This code ensures you're loading the class in the correct context, thus preventing runtime issues.
2. Non-Deterministic Behavior
Java agents execute code during class loading and method execution, making their behavior non-deterministic. This unpredictability can lead to inconsistent application performance or unintended side effects.
Mistake: Placing too much logic within the agent can cause unpredictable behavior.
Solution: Limit the amount of business logic processed within an agent. Keep it lightweight, relegating heavier computations to your main application.
Example Code:
public class MyAgent {
public static void premain(String agentArgs, Instrumentation inst) {
inst.addTransformer(new Transformer());
// Avoid heavy computation here
}
}
Why this matters: This ensures your agent remains efficient, focusing on instrumentation rather than complex logic that could lead to unexpected behavior.
3. Performance Overhead
Java agents introduce an additional layer of processing. If not designed properly, they can seriously hinder application performance.
Mistake: Heavy instrumentation can slow down method calls, impacting the performance of the application.
Solution: Optimize the transformations performed. For example, avoid instrumenting every method in a class if only a few are of interest.
Example Code:
private static boolean shouldInstrument(String className) {
return "com.example.SomeClass".equals(className);
}
Why this matters: By selectively instrumenting classes, you mitigate performance hits, focusing on critical areas of your application.
4. Not Handling Exceptions Properly
When modifying application classes, exceptions can occur if the target class does not conform to expected structures.
Mistake: Neglecting to catch exceptions can lead to application crashes.
Solution: Wrap your transformation logic with try-catch blocks to handle potential exceptions gracefully.
Example Code:
@Override
public byte[] transform(Module module, Class<?> classfileBuffer, ProtectionDomain protectionDomain) {
try {
// Transformation logic
} catch (Exception e) {
// Log the exception without crashing the application
Logger.getLogger(MyAgent.class.getName()).log(Level.WARNING, "Transformation failed", e);
}
return classfileBuffer; // Return original if transformation fails
}
Why this matters: Proper exception handling avoids catastrophic failures and allows your application to continue running smoothly.
5. Forgetting to Unload Classes
Java keeps loaded classes in memory, which can lead to memory leaks if your agent does not manage the loaded classes properly.
Mistake: Over time, failing to unload classes can lead to high memory usage.
Solution: Regularly check for unused classes and unload them as necessary. You may want to implement strategies such as the Weak References pattern to assist in garbage collection.
Example Code:
WeakReference<MyClass> weakRef = new WeakReference<>(myClassInstance);
// When no strong reference exists to MyClass, it can be collected by GC.
Why this matters: This proactive approach ensures optimal memory utilization, keeping your Java application efficient.
6. Inadequate Testing
When working with Java agents, it is vital to thoroughly test your agent in various environments.
Mistake: Failing to test can result in deployment issues or unexpected crashes in production.
Solution: Create comprehensive unit tests for your agents. Set up staging environments that replicate production scenarios to test thoroughly.
Example Testing Frameworks:
Why this matters: Solid testing minimizes the chances of runtime failures, preparing your agents for real-world conditions.
7. Relying Too Heavily on Reflection
Reflection is a common approach in Java agents to inspect and manipulate classes. However, misuse can lead to performance costs and stability issues.
Mistake: Overusing reflection can result in slower applications and increase complexity.
Solution: Limit the use of reflection in your agents. Instead, choose design patterns that promote better extensibility and maintainability.
Example Code:
Method method = MyClass.class.getMethod("myMethod");
method.invoke(myClassInstance); // Avoid excessive use of reflection
Why this matters: By minimizing reliance on reflection, you can keep your code cleaner and improve application performance.
Final Thoughts
Java agents can be immensely powerful tools for advanced Java programming, but using them effectively requires careful consideration. By avoiding common pitfalls such as ClassLoader misunderstandings, ignoring performance issues, and inadequate testing, you can harness the full potential of Java agents without suffering from their inherent complexities.
Whether you are writing a new agent or maintaining an existing one, keep these pitfalls in mind to streamline your development process. For further reading on Java Instrumentation, consider consulting the Java Instrumentation API.
By following best practices and maintaining a proactive approach, you ensure that your use of Java agents is both effective and efficient. Happy coding!