Common Pitfalls in Apache Ant Build Automation
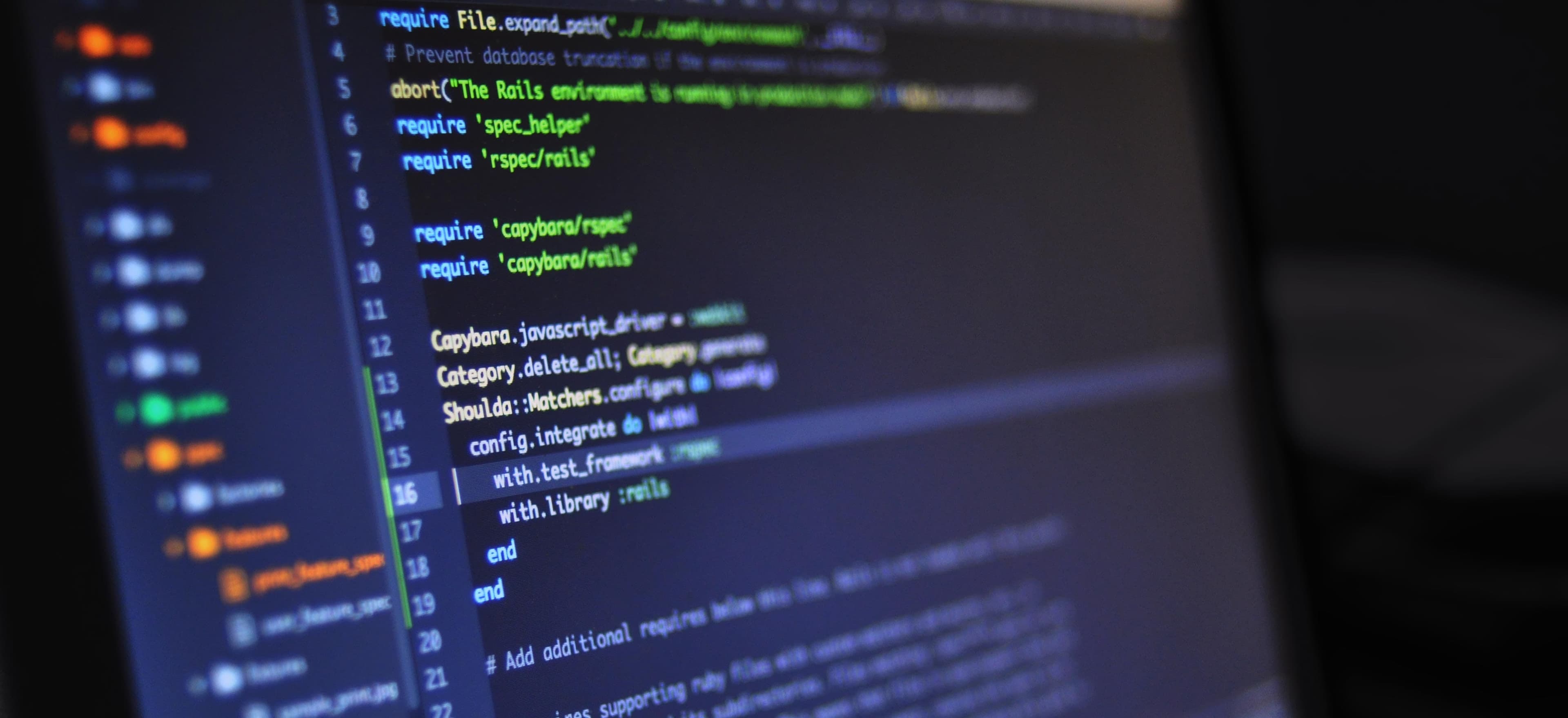
- Published on
Common Pitfalls in Apache Ant Build Automation
Apache Ant is a powerful tool that revolutionized how developers handle build automation. It provides a way to streamline the build process by using XML build scripts, paving the way for consistent and repeatable builds. However, even seasoned developers can fall victim to common pitfalls when using Apache Ant. In this blog post, we will discuss these pitfalls, how to avoid them, and provide illustrative code snippets for better understanding.
Table of Contents
- Over-complicating Build Files
- Ignoring Property Management
- Not Using Inheritance Wisely
- Failure to Leverage Built-in Tasks
- Mismanaging Dependencies
- Not Validating XML Files
- Using Ant for Everything
1. Over-complicating Build Files
It is tempting to over-engineer your build scripts, especially with the plethora of features that Ant provides. However, overly complex build files can lead to maintainability issues.
Solution
Keep your build files simple and concise. Aim to have a modular structure by breaking larger tasks into smaller, reusable targets.
<project name="SimpleBuild" default="all">
<target name="compile">
<javac srcdir="src" destdir="bin"/>
</target>
<target name="jar">
<jar destfile="output/myapp.jar" basedir="bin"/>
</target>
<target name="all" depends="compile, jar"/>
</project>
In this example, we have a straightforward build process that compiles Java files and packages them into a JAR. Each task is clearly separated, making it easier to maintain.
2. Ignoring Property Management
Properties in Ant provide a way to parameterize your build script. Ignoring properties can lead to hardcoded values that could easily change, making future adjustments more difficult.
Solution
Use properties for values that might change, like compiler options or directory paths.
<property name="src.dir" value="src"/>
<property name="bin.dir" value="bin"/>
<target name="compile">
<javac srcdir="${src.dir}" destdir="${bin.dir}"/>
</target>
By defining src.dir
and bin.dir
as properties, you can easily change them in one place, enhancing flexibility and reducing redundancy.
3. Not Using Inheritance Wisely
Apache Ant supports XML file inheritance, allowing you to create a build script that extends another. Failing to leverage this feature can lead to duplicate configurations.
Solution
Use a parent build file to define common tasks and properties.
Parent Build: build-parent.xml
<project name="ParentBuild" default="common">
<property name="common.dir" value="common"/>
<target name="common">
<echo message="This is a common target."/>
</target>
</project>
Child Build: build.xml
<project name="ChildBuild" default="all" xmlns:ant="ant:">
<import file="build-parent.xml"/>
<target name="all" depends="common">
<echo message="Executing child build."/>
</target>
</project>
This approach creates reusable components, reducing redundancy and errors in your build scripts. You can find out more about Ant Inheritance in the official documentation.
4. Failure to Leverage Built-in Tasks
Ant comes with a multitude of built-in tasks, but some developers insist on building complex custom tasks. This can lead to unnecessarily convoluted implementations.
Solution
Whenever possible, use built-in tasks.
<target name="clean">
<delete dir="bin"/>
</target>
Using the built-in delete
task simplifies your code and makes it easier for others to understand what you’re doing. It is a straightforward solution that removes the bin
directory without complicating matters.
5. Mismanaging Dependencies
Dependencies must be carefully managed in any build process. Neglecting them can lead to issues where a project builds one way in one environment and fails in another.
Solution
Utilize a build tool in conjunction with Ant that handles dependencies automatically, such as Apache Ivy.
Ivy configuration example: ivy.xml
<ivy-module version="2.0">
<info organisation="com.example" module="myapp" />
<dependencies>
<dependency org="junit" name="junit" rev="4.12" />
</dependencies>
</ivy-module>
In Your Ant Build
<ivy:retrieve pattern="lib/[artifact]-[revision].[ext]"/>
By integrating Ivy, you ensure that all dependencies are managed centrally, which reduces conflicts and makes builds more reliable.
6. Not Validating XML Files
XML syntax errors can be difficult to detect and may cause builds to fail unexpectedly. Neglecting XML validation can lead to lengthy debugging sessions.
Solution
Use an XML validation task.
<target name="validate">
<xmlvalidate srcfile="build.xml"/>
</target>
This task lends itself to an earlier detection of issues in your build files. Configuring this task can save developers hours in debugging.
7. Using Ant for Everything
Finally, one of the most common pitfalls is believing that Ant can solve all build-related problems. While Ant is highly capable, overusing it for trivial tasks can lead to unnecessary complexity and poor performance.
Solution
Evaluate the needs of your project and consider other tools that might suit specific tasks better.
For instance, consider using Maven for dependency management and project structure, or even Gradle, which integrates well with modern development practices. Both of these tools offer advanced features that Ant lacks.
Key Takeaways
Apache Ant is a robust tool for build automation, but it’s important to avoid common pitfalls that can diminish its efficacy and maintainability. By adhering to best practices, you can keep your build files modular, manageable, and clear.
Utilizing properties, leveraging inheritance, taking advantage of built-in tasks, and managing dependencies with tools like Ivy will optimize your overall workflow. By validating your build scripts and refraining from over-reliance on Ant, you can create a harmonious and efficient build process.
For further reading on Ant's capabilities, check out the official Apache Ant Documentation.
By carefully navigating these common pitfalls, you can unleash the full potential of Apache Ant in your projects. Happy building!
Checkout our other articles