Overcoming Common Pitfalls in Java Continuous Deployment
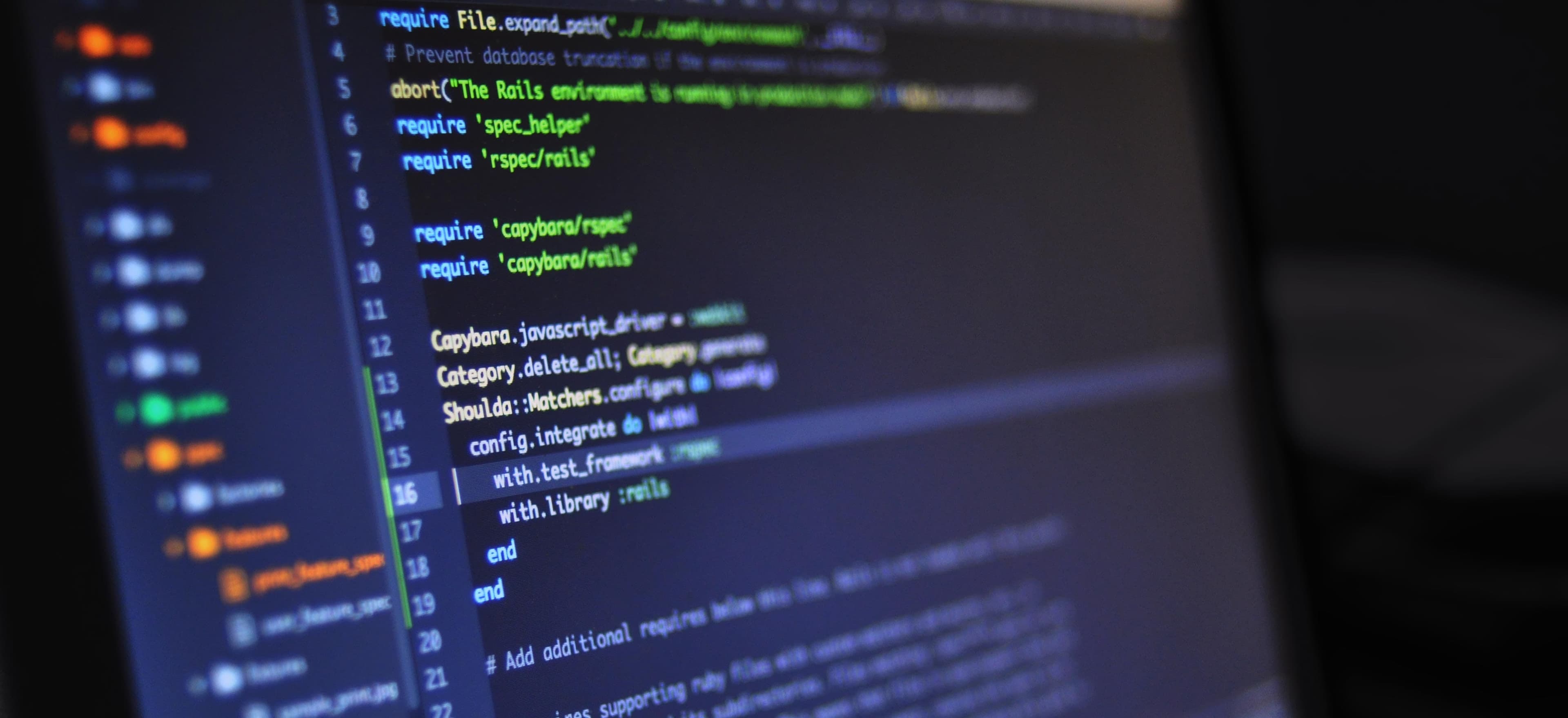
- Published on
Overcoming Common Pitfalls in Java Continuous Deployment
Continuous deployment has changed the way software is developed and delivered. In a fast-paced environment, the need to continuously integrate code changes and deliver them efficiently to production is paramount. For Java developers, however, this journey is laden with numerous challenges. In this post, we'll explore common pitfalls in Java continuous deployment and provide actionable solutions to overcome these challenges.
What is Continuous Deployment?
Continuous deployment is the practice of automatically deploying code changes to production after passing automated tests. This approach reduces the manual intervention needed in the deployment process and speeds up delivery. However, it's not without its hurdles, especially in a language as old and robust as Java.
The Importance of Continuous Deployment for Java
More often than not, Java applications power the backend of enterprise-level applications. Rapid changes in business requirements necessitate a responsive approach to software development. Continuous deployment offers several benefits:
- Faster time to market: Features and fixes are delivered quickly.
- Immediate feedback: Developers receive real-time insights into how new code impacts production.
- Improved collaboration: DevOps teams can work more efficiently.
While the benefits are undeniable, let's dive into some of the common pitfalls Java developers face in continuous deployment.
Common Pitfalls and Solutions
1. Poor Test Coverage
One of the most significant challenges is inadequate test coverage. When tests fail due to insufficient validation, it can cause deployment delays or, worse, lead to production issues.
Solution:
Developers should prioritize reaching a minimum of 80% code coverage, focusing on both unit and integration tests. Tools like JUnit and Mockito are widely used to create comprehensive tests. Here's a simple example:
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.*;
public class CalculatorTest {
private Calculator calculator;
@BeforeEach
public void setup() {
calculator = new Calculator();
}
@Test
public void testAdd() {
assertEquals(5, calculator.add(2, 3)); // Simple addition test
}
@Test
public void testAddWithMock() {
Calculator mockedCalculator = mock(Calculator.class);
when(mockedCalculator.add(2, 3)).thenReturn(5);
assertEquals(5, mockedCalculator.add(2, 3)); // Using mock to validate
}
}
In this test suite, we've used JUnit and Mockito to ensure that the Calculator
class works as intended. The mock showcases how dependencies can be simulated, thereby isolating the unit testing.
2. Manual Deployment Processes
Manual deployment can lead to human errors—overwriting configuration files, deploying incorrect versions, or missing critical steps.
Solution:
Automate your deployment processes with tools like Jenkins, GitLab CI, or GitHub Actions. These tools help create pipelines that can automatically build, test, and deploy your Java applications.
Here's a basic example of a Jenkins pipeline configuration for a Java project:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh './gradlew build' // Build with Gradle
}
}
stage('Test') {
steps {
sh './gradlew test' // Run tests
}
}
stage('Deploy') {
steps {
sh 'docker build -t my-java-app .'
sh 'docker push my-java-app' // Push Docker image
}
}
}
}
In this script, we define stages for building, testing, and deploying our application using Gradle and Docker. This ensures a streamlined, repeatable process.
3. Ignoring Environment Configurations
Each environment (development, testing, production) comes with its unique configuration settings. Hardcoding these values can lead to issues during deployment.
Solution:
Utilize environment variables for configuration settings. Java frameworks like Spring Boot offer application.properties
or application.yml
configurations that support different profiles.
Example of using profiles in Spring Boot:
# application.properties
spring.datasource.url=jdbc:mysql://localhost:3306/devdb
spring.datasource.username=dev_user
spring.datasource.password=dev_pass
# application-test.properties
spring.datasource.url=jdbc:mysql://localhost:3306/testdb
spring.datasource.username=test_user
spring.datasource.password=test_pass
By leveraging different properties files for different environments, changes can seamlessly adapt to various configurations without code changes. Get started with Spring Profiles for better environment management.
4. Lack of Monitoring and Feedback
Deploying new code is just the beginning. Without proper monitoring and feedback mechanisms, you lose visibility into how changes impact production, leading to frustrating downtimes and performance issues.
Solution:
Integrate application performance monitoring (APM) tools like New Relic, Datadog, or Prometheus. These tools provide real-time insights into application health, performance, and user experience.
For instance, integrating Prometheus with a Java application can be as simple as adding the dependency:
<dependency>
<groupId>io.prometheus</groupId>
<artifactId>simpleclient</artifactId>
<version>0.10.0</version>
</dependency>
And then exposing metrics:
import io.prometheus.client.Counter;
public class Metrics {
static final Counter requests = Counter.build()
.name("requests_total")
.help("Total requests.")
.register();
public void handleRequest() {
requests.inc(); // Increment the counter on request handling
}
}
By instrumenting your application with metrics, you can monitor usage patterns and identify performance bottlenecks immediately.
5. Failing to Roll Back
Despite best efforts, sometimes things go wrong in production. Not having a rollback strategy can exacerbate problems, leading to extended downtimes.
Solution:
Implement strategies like blue/green deployments or canary releases. These strategies minimize the risk of deploying new features to all users simultaneously.
For a simple blue/green strategy, consider:
- Production Environment (Blue): The current live version.
- Staging Environment (Green): The new version to be deployed.
You can switch traffic between environments using a load balancer. If something goes wrong with the green environment, you can revert to blue quickly, ensuring minimal disruption.
Closing the Chapter
Continuous deployment is not just a buzzword; it's a critical practice for modern Java development that enables teams to deliver quality software rapidly. Addressing common pitfalls such as test coverage, manual processes, configuration management, monitoring, and rollback strategies can empower Java developers to enhance their deployment pipelines.
By adopting a proactive approach to these challenges, you can unlock the full potential of continuous deployment, foster a culture of innovation, and ultimately deliver exceptional software solutions.
With practice, overcoming these pitfalls will become second nature, leading to an efficient, reliable, and agile development lifecycle. For a deeper dive into continuous deployment practices, consider exploring DevOps practices that accompany this essential methodology.
Checkout our other articles