Scaling Challenges in Reactive Microservices with Vert.x
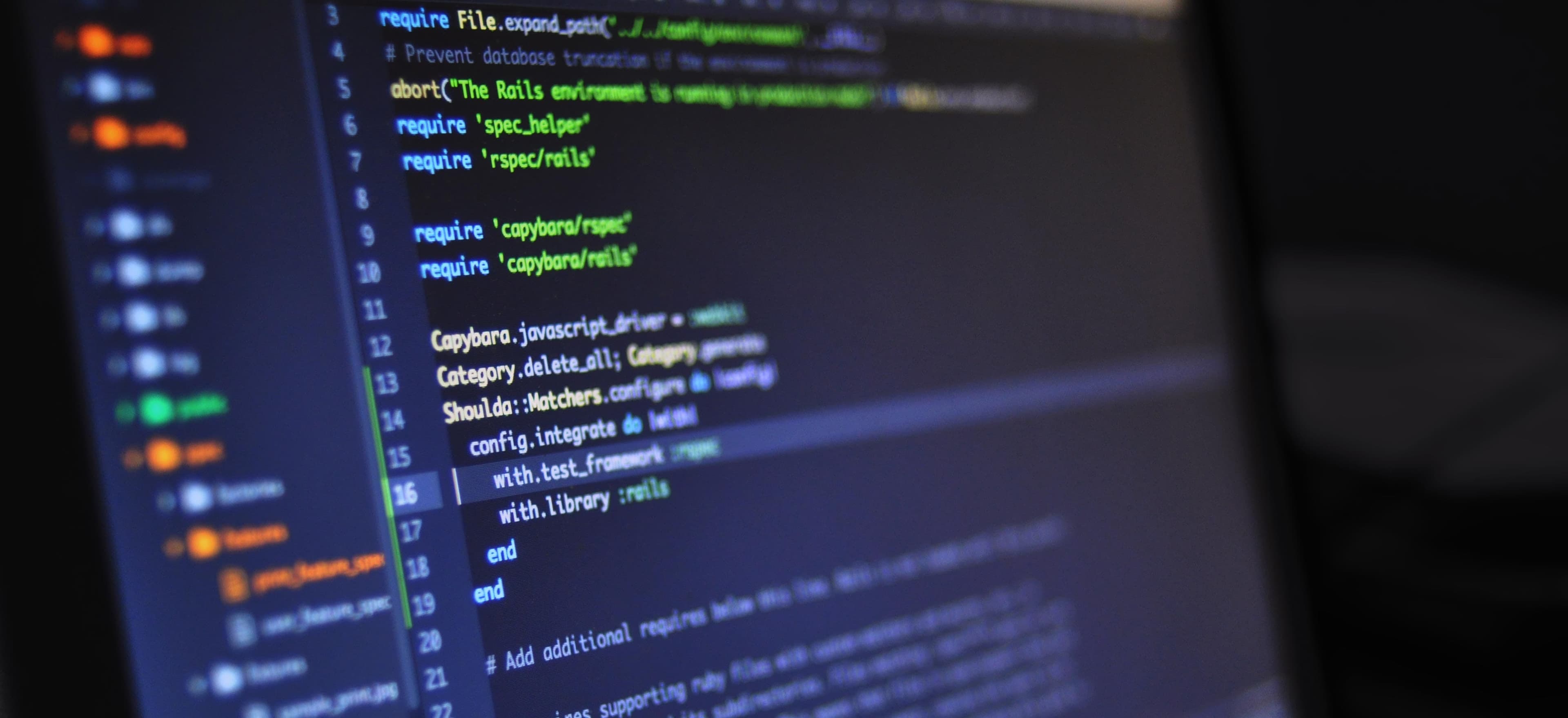
- Published on
Scaling Challenges in Reactive Microservices with Vert.x
In today's fast-paced software development landscape, the demand for scalable and responsive applications has increased dramatically. The introduction of microservices architecture has revolutionized how we build applications, enabling teams to develop, deploy, and scale services independently. However, while microservices provide many advantages, they also bring several challenges, particularly when scaling.
One of the frameworks that have gained popularity for building reactive microservices is Vert.x. This blog post dives deep into the scaling challenges associated with reactive microservices using Vert.x, while also discussing strategies to tackle these issues effectively.
Understanding Vert.x
Vert.x is a polyglot event-driven application framework that simplifies the construction of scalable applications. Built on a non-blocking architecture, it offers high concurrency with a relatively small resource footprint. This framework is designed for creating reactive microservices that can handle a high volume of requests efficiently.
Key features of Vert.x include:
- Event-driven architecture: Using event loops for managing concurrency.
- Polyglot capabilities: Allows coding in multiple languages like Java, JavaScript, Groovy, and Ruby.
- Modularity: Facilitates the creation of independent, reusable service components.
The Scaling Challenges in Reactive Microservices
1. Managing State in a Stateless World
One of the core principles of microservices is the idea of statelessness. Each service should not rely on state from external sources. However, in real-world applications, services often require access to stateful information.
Solution: Distributed Data Management
To overcome state management challenges, you can adopt distributed data stores like Apache Cassandra or Redis. These technologies help maintain state across distributed services. You can cache results in-memory to minimize latency and optimize performance.
Example: Caching with Vert.x
Here’s a basic example of caching responses in Vert.x:
import io.vertx.core.Vertx;
import io.vertx.core.json.JsonObject;
import io.vertx.redis.client.Redis;
import io.vertx.redis.client.RedisAPI;
public class CacheExample {
private final Vertx vertx = Vertx.vertx();
private RedisAPI redis;
public CacheExample() {
Redis.createClient(vertx, "redis://localhost:6379")
.connect(ar -> {
if (ar.succeeded()) {
redis = RedisAPI.api(ar.result());
}
});
}
public void cacheData(String key, JsonObject data) {
redis.set(List.of(key, data.encode()), res -> {
if (res.succeeded()) {
System.out.println("Data cached successfully!");
} else {
System.out.println("Could not cache data: " + res.cause().getMessage());
}
});
}
}
In the example above, we cache data in Redis to ensure faster responses later, which helps in scaling the application. When caching data, it's essential to manage cache invalidation to prevent stale data usage.
2. Service Discovery and Load Balancing
In a microservices architecture, as services grow, service discovery and load balancing become crucial. Without proper mechanisms, the efficiency and speed of requests can decline, leading to bottlenecks.
Solution: Implementing Service Mesh
Using a service mesh like Istio or Linkerd can help manage service discovery and load balancing effectively. They provide a dedicated layer to handle communication and routing between services without modifying the application code.
Additionally, you can leverage the capabilities of Vert.x's service proxy for seamless service-to-service communication.
Code Example for Service Proxy
import io.vertx.core.Vertx;
import io.vertx.serviceproxy.ServiceProxyBuilder;
public class ServiceProxyExample {
private final Vertx vertx;
public ServiceProxyExample(Vertx vertx) {
this.vertx = vertx;
}
public void initializeService() {
MyService service = new MyServiceImpl();
ServiceProxyBuilder<MyService> builder = new ServiceProxyBuilder<>(vertx);
builder.setAddress("my.service.address").build(MyService.class);
// Now you can call the service using the proxy
}
}
Here, a service proxy is created, allowing communication between various microservices. This decouples service details, enhancing maintainability.
3. Handling Backpressure
In reactive systems, propagating backpressure is critical. When a service is overwhelmed with requests, it must signal upstream services to slow down. Failing to implement backpressure can lead to resource exhaustion and service crashes.
Solution: Circuit Breakers and Rate Limiting
You can use a library like Hystrix or Resilience4j for managing backpressure through circuit breakers. Vert.x also provides vertx-circuit-breaker for fault tolerance.
Example: Circuit Breaker with Vert.x
import io.vertx.circuitbreaker.CircuitBreaker;
import io.vertx.core.Vertx;
public class CircuitBreakerExample {
private final Vertx vertx;
private CircuitBreaker circuitBreaker;
public CircuitBreakerExample(Vertx vertx) {
this.vertx = vertx;
this.circuitBreaker = CircuitBreaker.create("my-circuit-breaker", vertx, options -> {
options.setTimeout(5000);
options.setMaxFailures(5);
options.setResetTimeout(20000);
});
}
public void executeServiceCall() {
circuitBreaker.executeWithFallback(promise -> {
// Make network call or heavy computation
promise.complete("Service response");
}, ar -> {
if (ar.succeeded()) {
System.out.println(ar.result());
} else {
System.out.println("Service call failed: " + ar.cause().getMessage());
}
});
}
}
In the above code, we set up a simple circuit breaker configuration. This mechanism protects services from becoming overwhelmed by managing call failures gracefully.
4. Asynchronous Communication
While Vert.x promotes asynchronous programming, managing complex workflows with multiple service calls can become convoluted. Services may often rely on one another to complete a task.
Solution: Event-Driven Architecture
By employing an event-driven architecture, you can decouple services and enhance scalability. Use message brokers like Apache Kafka or RabbitMQ to manage events and data flows across your services seamlessly.
Event Bus Example
import io.vertx.core.Vertx;
public class EventBusExample {
private final Vertx vertx;
public EventBusExample(Vertx vertx) {
this.vertx = vertx;
}
public void publishEvent() {
vertx.eventBus().publish("news.feed", "New article published!");
vertx.eventBus().consumer("news.feed", message -> {
System.out.println("Received message: " + message.body());
});
}
}
In this example, services can publish or subscribe to events. This not only improves decoupling but also allows for real-time updates across services.
In Conclusion, Here is What Matters
Scaling reactive microservices with Vert.x presents significant challenges, from managing state to handling backpressure effectively. As we've discussed, employing strategies such as distributed data management, leveraging service proxies, implementing circuit breakers, and utilizing event-driven architectures proves essential for successful scaling.
The framework's modularity and event-driven nature makes it an excellent choice for developing high-performance applications, but it's crucial to understand the pitfalls and prepare your services to overcome them. By embracing the recommended practices and solutions, your team can effectively scale reactive microservices and meet the growing demands of modern applications.
For more in-depth knowledge about Vert.x and microservices architectures, consider exploring additional resources like Vert.x Documentation and Microservices Patterns.
Happy coding!