Common Pitfalls When Implementing REST Clients in Java
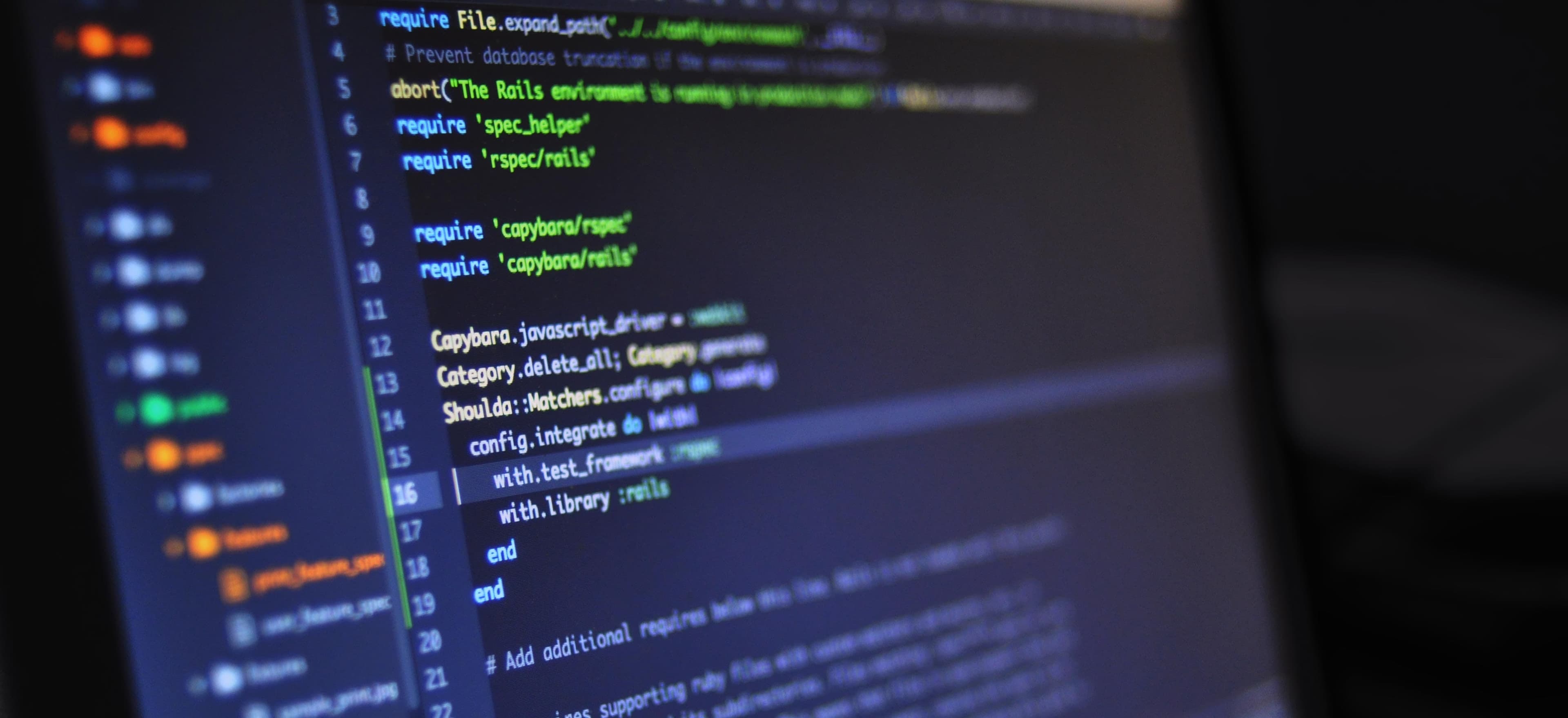
- Published on
Common Pitfalls When Implementing REST Clients in Java
Creating robust REST clients in Java can significantly enhance how applications communicate with RESTful services. However, there are common pitfalls developers encounter that can impact reliability, performance, and maintainability. This blog post outlines these pitfalls and offers best practices, ensuring your REST clients are not just functional, but also efficient and scalable.
1. Ignoring HTTP Methods and Status Codes
Understanding HTTP Methods
RESTful APIs rely on HTTP methods (GET, POST, PUT, DELETE) to perform different operations. Each method has a specific purpose:
- GET: Retrieve data from the server.
- POST: Create new resources.
- PUT: Update existing resources.
- DELETE: Remove resources.
Using the wrong HTTP method can lead to unexpected behavior. For instance, sending a GET request to submit data might not work as intended.
Example Code Snippet
import java.net.HttpURLConnection;
import java.net.URL;
public class RestClient {
public void getData() {
try {
URL url = new URL("http://api.example.com/resource");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
int responseCode = connection.getResponseCode();
if (responseCode == HttpURLConnection.HTTP_OK) {
// Process successful response
} else {
// Handle errors based on response code
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
Importance of Status Codes
Always check the HTTP status codes returned by the server. A code in the 200 range usually means success, while codes in the 400 or 500 range indicate client-side or server-side errors. Ignoring these could lead to ambiguous states in your application.
Learn More
For an in-depth explanation of HTTP status codes, visit the MDN Web Docs on HTTP Response Status Codes.
2. Failing to Handle Exceptions
When working with network operations, exceptions are a part of the game. Network issues, timeouts, or incorrect configurations can trigger exceptions. Not handling these gracefully can crash your application or lead to undesirable states.
Example Code Snippet
public void executeRequest() {
try {
// Assume prepareConnection is a method that prepares a HttpURLConnection
HttpURLConnection connection = prepareConnection();
connection.connect();
} catch (IOException e) {
System.err.println("Network error occurred: " + e.getMessage());
// Consider implementing retry logic or fallback mechanisms
}
}
Best Practice
Implement retries with exponential backoff for transient errors. This enhances resilience against temporary failures.
3. Not Considering Asynchronous Processing
Blocking calls in REST clients can lead to performance bottlenecks, particularly in high-latency environments. By using asynchronous processing, you can free up resources for other tasks, improving overall application performance.
Using CompletableFuture
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.concurrent.CompletableFuture;
public class AsyncRestClient {
public CompletableFuture<String> asyncGetData() {
return CompletableFuture.supplyAsync(() -> {
try {
HttpURLConnection connection = (HttpURLConnection) new URL("http://api.example.com/resource").openConnection();
connection.setRequestMethod("GET");
// Check the response code and process the input stream as needed.
return "Response"; // Placeholder return statement
} catch (Exception e) {
throw new RuntimeException("Error in async call", e);
}
});
}
}
Benefit of Asynchronous Calls
Using CompletableFuture
allows non-blocking operations, improving responsiveness and throughput in your applications.
4. Relying on a Single Library
Java developers have many libraries at their disposal for implementing REST clients. While using a single library simplifies dependencies, it may also limit flexibility and performance. Each library offers different features, optimizations, and community support.
Popular REST Client Libraries
- OkHttp: Known for its efficiency and ease of use.
- Spring RestTemplate: A robust option ideal for Spring applications.
- Apache HttpClient: Flexible and suited for advanced use cases.
Choosing the Right Library
Evaluate your project's requirements against what each library offers. For example, use Spring’s RestTemplate if you’re already using the Spring Framework for better integration.
5. Neglecting Request Retry Logic
Network requests are inherently unreliable due to various factors such as server downtime or packet loss. Implementing retry logic can guard against temporary failures.
Example Code Snippet with Retry Logic
public void safeGetData() {
int retries = 3;
while (retries > 0) {
try {
HttpURLConnection connection = (HttpURLConnection) new URL("http://api.example.com/resource").openConnection();
connection.setRequestMethod("GET");
// Handle response over here
break; // exit loop on success
} catch (IOException e) {
retries--;
System.err.println("Request failed, attempts left: " + retries);
sleep(1000); // Implementing backoff strategy
}
}
}
Considerations
Ensure that your retry mechanism accounts for significant failures. It's also a good practice to log errors and monitor failed requests for troubleshooting.
6. Not Managing Timeouts
Timeouts are crucial in avoiding scenarios where a request hangs indefinitely. Not setting timeout values could result in unresponsive applications.
Example Code Snippet
public HttpURLConnection prepareConnection() throws IOException {
URL url = new URL("http://api.example.com/resource");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("GET");
connection.setConnectTimeout(5000); // 5 seconds
connection.setReadTimeout(5000); // 5 seconds
return connection;
}
Why Set Timeouts?
Setting appropriate timeout values prevents your application from waiting indefinitely. This assures users receive timely feedback, maintaining a good user experience.
7. Failing to Decode Responses
REST APIs often return JSON responses; failing to decode them correctly can lead to application crashes or incorrect data being processed.
Using Libraries for JSON Parsing
Java has several libraries like Jackson and Gson that can simplify JSON parsing.
Example with Gson
import com.google.gson.Gson;
public class JsonParserExample {
public MyResponse parseResponse(String jsonResponse) {
Gson gson = new Gson();
return gson.fromJson(jsonResponse, MyResponse.class);
}
private class MyResponse {
// Define fields according to the JSON structure
}
}
Why Use Libraries?
Using libraries like Gson or Jackson allows you to focus on business logic instead of parsing details, enhancing code maintainability.
Key Takeaways
Implementing REST clients in Java may seem straightforward at first glance, but you can easily find yourself facing certain pitfalls if you are not careful. By paying attention to HTTP methods, exception handling, and performance optimization techniques like asynchronous calls or retry logic, you can create a robust REST client that's not just functional but also performs well under various conditions.
Follow best practices and choose the right libraries tailored to your project's requirements. With diligence, your Java REST client can become a reliable asset in your software architecture. For further reading, check the RESTful Web Services Tutorial by Baeldung, which dives deeper into REST implementation in different contexts.