Resolving Java Classpath Confusion: Tips and Tricks
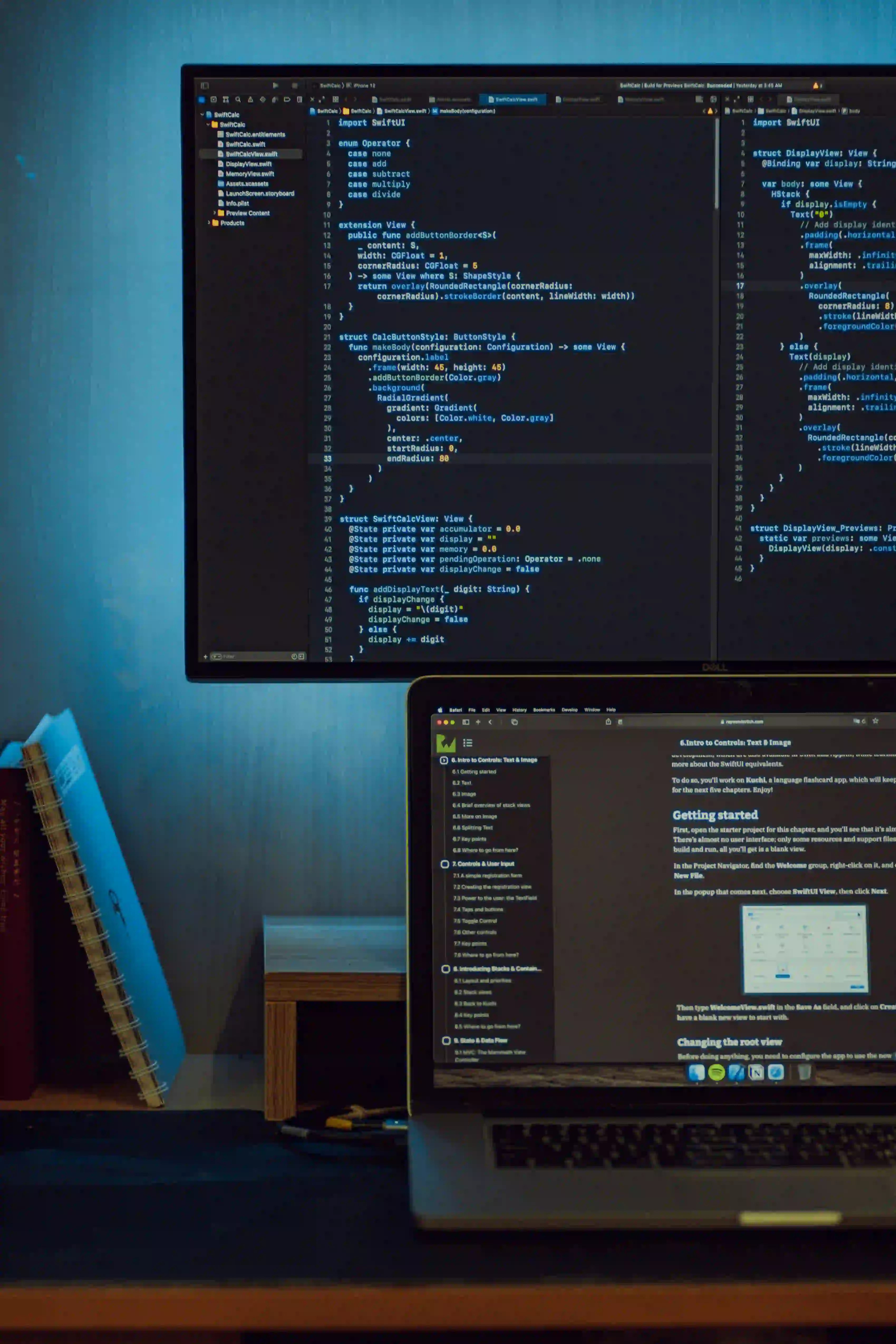
Resolving Java Classpath Confusion: Tips and Tricks
Java developers often find themselves caught in a quagmire due to classpath confusion. The Java classpath is crucial for the Java Runtime Environment (JRE) to locate user-defined classes and packages, as well as third-party libraries. In this blog post, we'll unravel the intricacies of the Java classpath, provide practical tips, and introduce handy tricks to make your life easier.
What is the Java Classpath?
The Java classpath is a parameter that tells the Java Virtual Machine (JVM) and Java compiler where to look for user-defined classes and packages. It is essentially a list of directories, JAR files, and ZIP files that contain classes and resources. Misconfiguring the classpath can lead to a common error: ClassNotFoundException
.
Understanding Classpath Structures
The classpath can be specified in several ways:
-
The Command Line: You can set the classpath directly when executing a Java program using the
-cp
(or-classpath
) option.π§snippet.shjava -cp .:lib/* com.example.Main
In this example, the classpath consists of the current directory (
.
) as well as all JAR files in thelib
directory. -
Environment Variables: You can configure the
CLASSPATH
environment variable in your system. However, relying on this can lead to portability issues across different machines. -
Manifest Files: If you are packaging a JAR file, you can specify the classpath within the JAR's manifest file. This allows the JVM to automatically recognize dependencies.
Manifest-Version: 1.0
Class-Path: lib/library1.jar lib/library2.jar
Using these approaches provides flexibility, but it also introduces potential for confusion.
Common Classpath Issues
Recognizing where things can go wrong is essential. Let's consider some prevalent classpath-related issues with solutions:
1. ClassNotFoundException
This is a classic error message that indicates the JVM could not locate a class file. Hereβs a sample scenario:
public class Test {
public static void main(String[] args) {
NewClass obj = new NewClass(); // ClassNotFoundException occurs here
}
}
Solution: Verify that the class is indeed in the defined classpath. Double-check the case sensitivity as many filesystems (especially on Unix-like systems) are case-sensitive.
2. Wrong Directory Structure
If your Java file is stored in a specific package, the directory structure should reflect that package hierarchy.
Example Package Structure:
src/
βββ com/
βββ example/
βββ Test.java
βββ utils/
βββ Helper.java
Compile with:
javac -d out src/com/example/*.java
Run the application with:
java -cp out com.example.Test
Failing to respect this structure may yield ClassNotFoundException
.
3. Missing JAR Files
Sometimes, forgetting to include a required JAR file leads to runtime issues. Always make sure that your JAR files are present in the classpath.
Solution: Use -cp
to include necessary JAR files explicitly:
java -cp .:lib/myLibrary.jar com.example.Main
Tips for Managing the Classpath
It's easy to become overwhelmed with multiple libraries and projects. Here are some strategic tips for mitigating classpath confusion:
Use Build Tools
Using a build automation tool simplifies dependency management. Tools like Maven, Gradle, or Ant can automate the classpath definition process.
Maven Example
Create a pom.xml
file where you can declare dependencies. Maven manages the classpath for you.
<dependencies>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
</dependencies>
When you build the project, Maven handles downloading the necessary JAR files and adding them to the classpath.
Leverage IDEs
Modern IDEs like IntelliJ IDEA, Eclipse, or NetBeans come equipped with tools for managing classpaths easily. These integrated development environments automatically handle the classpath settings for you, reducing friction in development.
Maintain a Consistent Structure
Keep your project structure uniform. A clear directory hierarchy can lend itself to easier management of class files.
myProject/
βββ lib/
βββ src/
βββ bin/
Use Class Loader Strategies
Explore custom class loader strategies if your application dynamically loads classes at runtime. Hereβs a simple example using URLClassLoader:
import java.net.URL;
import java.net.URLClassLoader;
public class DynamicClassLoader {
public static void main(String[] args) throws Exception {
URL[] urls = { new URL("file:lib/myLibrary.jar") };
URLClassLoader classLoader = new URLClassLoader(urls);
Class<?> clazz = classLoader.loadClass("com.example.MyClass");
System.out.println("Loaded class: " + clazz.getName());
}
}
This approach allows for more dynamic management of classes and libraries.
Debugging the Classpath
When things go sideways, debugging becomes essential. Here are some effective strategies to diagnose classpath issues:
Print the Classpath
You can print the current classpath in your program using:
System.out.println(System.getProperty("java.class.path"));
This allows you to verify that the expected paths and JAR files are present.
Use Verbose Flags
Running the JVM with the -verbose:class
flag provides information about each class that's loaded, which can help you figure out what is being found and what isn't.
java -verbose:class -cp .:lib/* com.example.Main
To Wrap Things Up
Classpath confusion can be frustrating, but it is manageable with the right approach. Remember to leverage build tools, use IDEs, and maintain a clear project hierarchy. Keeping your classpath organized reduces errors, ensures proper class loading, and allows you to focus on building great applications.
For further reading on managing Java dependencies and classpaths, check out the Oracle Documentation on Classpaths and delve into various Java build tools for more organized and efficient development.
By embracing these tips and strategies, you can navigate the complexities of the Java classpath with ease and confidence. Happy coding!