Overcoming Common Pitfalls in Eclipse MicroProfile Integration
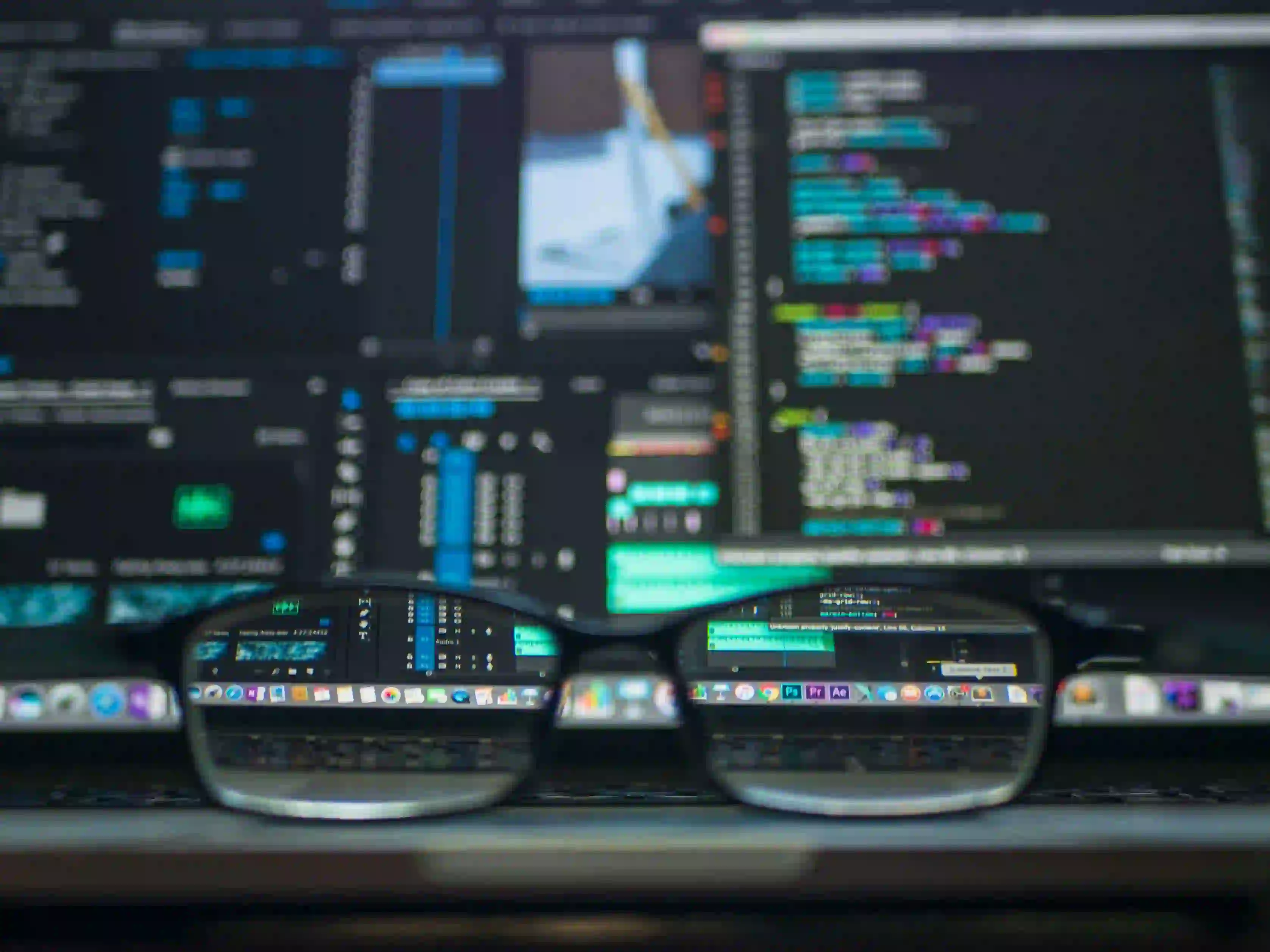
Overcoming Common Pitfalls in Eclipse MicroProfile Integration
Eclipse MicroProfile is designed to optimize Enterprise Java for microservices by offering a suite of specifications. As you dive deeper into integrating MicroProfile into your projects, you’ll likely encounter various challenges. In this blog post, we will discuss common pitfalls you may face during Eclipse MicroProfile integration and how to overcome them effectively.
Understanding Eclipse MicroProfile
Before we dig into the pitfalls, let’s briefly understand what Eclipse MicroProfile is. MicroProfile provides APIs and specifications that are highly advanced yet easy to use. It incorporates core features like fault tolerance, metrics, configuration, and more, tailored for microservices architecture.
Popular MicroProfile Specifications
- MicroProfile Configuration: Simplifies the way to manage application configurations.
- MicroProfile Metrics: Collects and reports metrics data for monitoring.
- MicroProfile Fault Tolerance: Manages failure in your application gracefully.
- MicroProfile Rest Client: Makes working with REST services easier.
Common Pitfalls in MicroProfile Integration
1. Inadequate Understanding of Configuration
Issue: Many developers overlook the importance of configuration management. The MicroProfile Configuration API allows you to externalize configurations. Neglecting this can lead to hard-coded values that are hard to manage and test.
Solution: Always utilize the MicroProfile Configuration API.
import org.eclipse.microprofile.config.inject.ConfigProperty;
import javax.inject.Inject;
public class GreetingService {
@Inject
@ConfigProperty(name = "greeting", defaultValue = "Hello")
String greeting;
public String greet(String name) {
return String.format("%s, %s!", greeting, name);
}
}
Why this works: By injecting the configuration, the application can easily adapt to changes without requiring code changes or redeployment. Make sure to keep your configuration in a .env
file or similar.
2. Ignoring Fault Tolerance
Issue: Implementing microservices without considering fault tolerance can lead to cascading failures when dependent services fail.
Solution: Make use of the Fault Tolerance annotations.
import org.eclipse.microprofile.faulttolerance.*;
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class UserService {
@Timeout(2000)
@Fallback(fallbackMethod = "fallbackGetUser")
public User getUser(String userId) {
// Simulating remote call
return fetchUserFromRemote(userId);
}
public User fallbackGetUser(String userId) {
return new User("default", "User");
}
}
Why this works: The @Timeout
annotation ensures that if the remote call takes too long, it will immediately fail and switch to the fallback method. This improves system robustness and user experience.
3. Neglecting Metrics Collection
Issue: Monitoring applications becomes problematic when metrics are neglected. MicroProfile Metrics allows you to gather and expose metrics for performance measurement.
Solution: Inject the MetricRegistry
and collect metrics.
import javax.enterprise.context.ApplicationScoped;
import javax.inject.Inject;
import org.eclipse.microprofile.metrics.MetricRegistry;
import org.eclipse.microprofile.metrics.annotation.Gauge;
@ApplicationScoped
public class OrderService {
@Inject
MetricRegistry registry;
private int orderCount = 0;
public void placeOrder(Order order) {
orderCount++;
}
@Gauge(name = "order-count", unit = "orders", description = "Total number of orders placed")
public int getOrderCount() {
return orderCount;
}
}
Why this works: This code snippet provides a gauge metric that tracks the number of orders placed, which can be critical for understanding application usage patterns and performance.
4. Poor Exception Handling
Issue: Insufficient handling of exceptions can lead to uninformative errors, making it difficult to debug in production environments.
Solution: Implement proper exception handling using @Retry
and @Fallback
.
import org.eclipse.microprofile.faulttolerance.*;
import javax.enterprise.context.ApplicationScoped;
@ApplicationScoped
public class PaymentService {
@Retry(maxRetries = 3)
@Fallback(fallbackMethod = "fallbackProcessPayment")
public PaymentResponse processPayment(Payment payment) {
// Logic to process payment
if (paymentFails()) {
throw new PaymentException("Payment failed!");
}
return new PaymentResponse("Success");
}
public PaymentResponse fallbackProcessPayment(Payment payment) {
return new PaymentResponse("Failed after retries, please try again later.");
}
}
Why this works: The @Retry
annotation allows for retrial in case of failures, while the fallback can inform the user to try again later, drastically improving the resilience of your application.
5. Mismanagement of Dependencies
Issue: When integrating multiple MicroProfile specifications, developers often neglect to manage dependencies correctly, leading to classpath issues.
Solution: Use a build tool like Maven or Gradle to manage your dependencies effectively.
Maven Example:
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-config-api</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-metrics-api</artifactId>
<version>2.0</version>
</dependency>
<dependency>
<groupId>org.eclipse.microprofile</groupId>
<artifactId>microprofile-fault-tolerance-api</artifactId>
<version>2.0</version>
</dependency>
Why this works: Managing dependencies accurately ensures that all your MicroProfile features work together seamlessly without version conflicts.
Additional Resources
- MicroProfile Official Page: MicroProfile
- MicroProfile Config Documentation: Configuration
- MicroProfile Fault Tolerance Documentation: Fault Tolerance
- MicroProfile Metrics Documentation: Metrics
Bringing It All Together
Integrating Eclipse MicroProfile can significantly enhance your microservices architecture. However, as this blog post illustrates, it’s essential to be aware of common pitfalls during integration. By applying best practices, such as effective configuration management, robust fault tolerance, comprehensive metrics collection, and proper dependency management, you can create resilient, maintainable applications.
As you embark on your MicroProfile journey, stay informed and continue learning about new features and techniques. Happy coding!
Feel free to explore the pitfalls outlined and leverage the solutions provided to ensure a smooth integration process with Eclipse MicroProfile in your enterprise applications.