Overcoming Java's Limitations for Blockchain Development
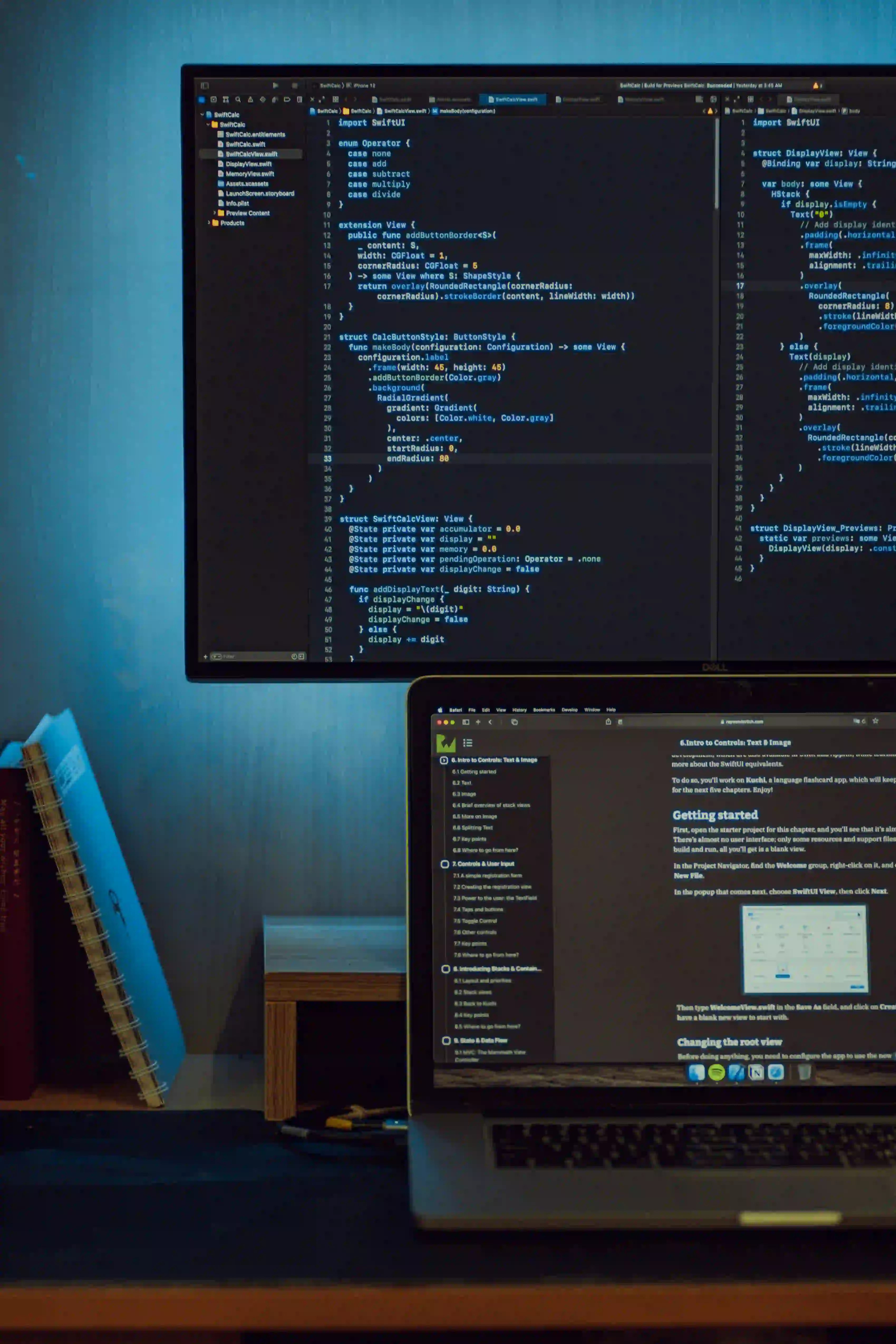
Overcoming Java's Limitations for Blockchain Development
Java, known for its portability, robustness, and vast ecosystem, has been a go-to programming language for many enterprise applications. However, when it comes to blockchain development, it faces certain limitations. This blog post explores these constraints and discusses innovative ways to overcome them, ensuring that Java remains a powerful player in the blockchain arena.
Understanding Java's Position in Blockchain
Before we delve into the limitations, it is crucial to understand why Java is considered for blockchain development. Java provides:
- Cross-Platform Capability: Java runs on any device with the Java Virtual Machine (JVM), making it a perfect candidate for decentralized applications.
- Large Community and Ecosystem: With a massive pool of libraries and frameworks, developers can leverage existing tools to build robust solutions.
- Strong Security Features: Java's built-in security features enhance application security, a crucial aspect of blockchain technology.
However, these advantages come with certain limitations that developers must navigate.
Key Limitations of Java in Blockchain Development
-
Performance Issues: Java, being an interpreted language, can sometimes lag in execution speed compared to languages like C++ or Rust. This is particularly problematic in scenarios where real-time processing is essential.
-
Memory Consumption: Java applications are known for consuming more memory. When dealing with large-scale blockchain networks or smart contracts, this can become a bottleneck.
-
Limited Native Support for Cryptographic Functions: While Java has libraries for cryptographic algorithms, the support is often not as extensive as that in other languages specifically tailored for cryptography.
-
Complexity with Asynchronous Programming: Java has a more complex setup for asynchronous programming than languages like JavaScript. This can complicate the development of decentralized applications that require non-blocking behavior.
Strategies for Overcoming Java's Limitations
Despite the challenges, developers can employ various strategies to enhance Java's effectiveness in blockchain development:
1. Optimize Performance with Java Native Interface (JNI)
JNI allows Java code to interact with native applications and libraries written in other languages such as C or C++. By utilizing JNI, developers can significantly reduce computational delays.
// Simple JNI example
public class NativeLib {
static {
System.loadLibrary("NativeLib");
}
public native void performHeavyComputation();
public static void main(String[] args) {
new NativeLib().performHeavyComputation();
}
}
Why Use JNI?
This example shows how JNI can optimize performance-sensitive sections of a Java application by leveraging C, which is closer to the hardware and typically faster. However, it adds complexity and requires careful management of native resources.
2. Efficient Memory Management
To tackle Java's memory consumption issues:
- Use lightweight data structures.
- Optimize garbage collection settings.
- Implement pooling for frequent object creation.
import java.util.ArrayList;
import java.util.List;
public class ObjectPool {
private List<MyObject> available = new ArrayList<>();
public MyObject borrowObject() {
if (available.isEmpty()) {
return new MyObject();
} else {
return available.remove(available.size() - 1);
}
}
public void returnObject(MyObject obj) {
available.add(obj);
}
}
Why Use an Object Pool?
This pattern minimizes the overhead of object creation and destruction, which can lead to better memory usage and performance.
3. Utilize Efficient Cryptographic Libraries
Java developers can leverage powerful third-party libraries such as Bouncy Castle or JCA (Java Cryptography Architecture) to handle cryptographic processes more effectively.
import org.bouncycastle.jce.provider.BouncyCastleProvider;
import java.security.Security;
import javax.crypto.Cipher;
public class CryptographyUtil {
static {
Security.addProvider(new BouncyCastleProvider());
}
public static byte[] encryptData(byte[] data) throws Exception {
Cipher cipher = Cipher.getInstance("AES/GCM/NoPadding", "BC");
// encryption steps ...
return cipher.doFinal(data);
}
}
Why Use Bouncy Castle?
Bouncy Castle provides a comprehensive set of cryptographic functions and is well-maintained. Choosing a library like this ensures that developers do not have to reinvent the wheel regarding cryptographic algorithms.
4. Embrace Asynchronous Programming with CompletableFuture
Java 8 introduced CompletableFuture
, which allows developers to write non-blocking code more elegantly.
import java.util.concurrent.CompletableFuture;
public class AsyncExample {
public CompletableFuture<String> fetchDataAsync() {
return CompletableFuture.supplyAsync(() -> {
// Simulating a network call
return "Data from Blockchain";
});
}
public static void main(String[] args) {
AsyncExample example = new AsyncExample();
example.fetchDataAsync()
.thenAccept(System.out::println);
}
}
Why Use CompletableFuture?
This not only simplifies asynchronous programming but also enhances readability. It allows developers to execute non-blocking operations effectively, a necessity for many blockchain applications.
Building Blockchain Solutions with Java
To illustrate the practical application of these strategies, let’s discuss a simple blockchain prototype created in Java.
Blockchain Prototype
Here’s an outline for a minimalist blockchain implementation using Java:
import java.util.ArrayList;
import java.util.List;
class Block {
String previousHash;
long timestamp;
String data;
String hash;
public Block(String previousHash, String data) {
this.previousHash = previousHash;
this.data = data;
this.timestamp = System.currentTimeMillis();
this.hash = calculateHash();
}
public String calculateHash() {
// Implement a hashing algorithm (like SHA-256)
return String.valueOf(this.data.hashCode() + this.timestamp + previousHash);
}
}
class Blockchain {
private List<Block> chain = new ArrayList<>();
public void addBlock(String data) {
String previousHash = chain.isEmpty() ? "0" : chain.get(chain.size() - 1).hash;
Block newBlock = new Block(previousHash, data);
chain.add(newBlock);
}
public List<Block> getChain() {
return chain;
}
}
Why Create a Blockchain Prototype?
This basic prototype demonstrates the fundamental concepts of a blockchain, including blocks, hashes, and the chain itself. Developers can build upon it to implement more complex features like consensus algorithms and smart contracts.
Closing the Chapter
Java's features make it a valuable tool in building blockchain applications, but developers must navigate its limitations creatively. By combining Java's strengths with the outlined strategies—like leveraging JNI for performance, optimizing memory management, and using third-party libraries for cryptography—developers can enhance its formidable capability.
As the blockchain landscape continues to evolve, Java remains a pertinent option for building resilient and scalable solutions. For more about programming in Java and its applications, consider visiting Oracle's Java Tutorials and exploring Bouncy Castle’s documentation to harness advanced cryptography for your blockchain projects.
By remaining adaptable and leveraging existing technologies, Java developers can conquer the blockchain realm efficiently and effectively. Happy coding!