Common Pitfalls in Big Data Processing with Java
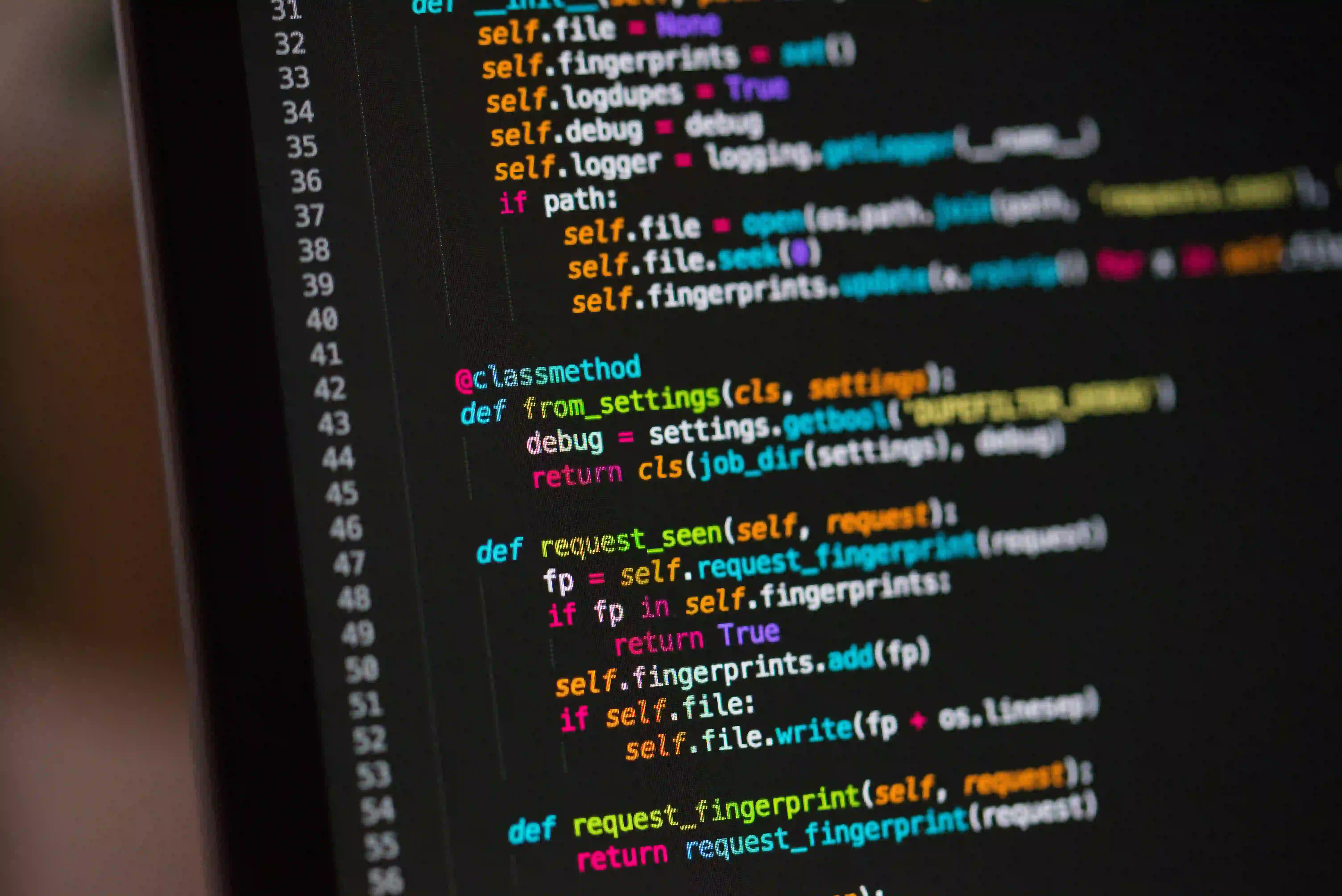
Common Pitfalls in Big Data Processing with Java
Big data processing has become a crucial part of modern data-driven decision-making. Java, known for its speed and robustness, is frequently used in big data frameworks like Apache Hadoop and Apache Spark. However, despite its advantages, there are several common pitfalls developers encounter when working with big data processing in Java. This blog post aims to highlight these pitfalls and discuss best practices to avoid them.
Understanding Big Data Concepts
Before diving into the common pitfalls, it’s important to briefly understand what big data entails. Big data refers to vast volumes of structured and unstructured data that cannot be processed effectively using traditional data processing methods. The three Vs of big data—Volume, Velocity, and Variety—illustrate its complexity.
The Java Advantage
Java’s versatility, platform independence, and extensive ecosystem make it an ideal choice for big data processing. However, mishandling its features can lead to performance issues and functional bottlenecks.
Common Pitfalls
Here are some pitfalls developers face when processing big data with Java, along with practical solutions to mitigate these risks.
1. Inefficient Memory Management
The Pitfall: Java’s garbage collection (GC) can lead to unexpected performance problems when dealing with large datasets. Developers often underestimate the impact of memory management on big data applications.
The Solution: Optimize memory usage to avoid frequent garbage collection events. Here’s an example of how to manage memory efficiently in a Java application.
public void processData(List<Data> dataList) {
for (Data data : dataList) {
// Process data
}
// Explicitly nullify references
dataList.clear();
}
Why? Nullifying references helps the garbage collector reclaim memory faster. It reduces the memory footprint, providing smoother operation, especially when handling larger data sets.
2. Ignoring Data Serialization
The Pitfall: Many developers overlook the importance of data serialization, which is crucial in big data applications that frequently transfer large volumes of data across networks. Neglecting efficient serialization can introduce significant overhead.
The Solution: Use a suitable serialization framework. Java’s default serialization can be slow and bulky, so consider using alternatives like Protocol Buffers or Avro.
// Sample using Avro serialization
GenericRecord user = new GenericData.Record(schema);
user.put("name", "John Doe");
user.put("age", 28);
ByteArrayOutputStream out = new ByteArrayOutputStream();
DatumWriter<GenericRecord> writer = new GenericDatumWriter<>(schema);
Encoder encoder = EncoderFactory.get().binaryEncoder(out, null);
writer.write(user, encoder);
encoder.flush();
Why? Avro offers compact serialization, which is critical for performance in distributed systems. It also provides schema evolution, ensuring greater flexibility in data processing.
3. Poor Parallelism Management
The Pitfall: Failing to take full advantage of Java’s threading capabilities can lead to underutilized system resources, which directly affects performance.
The Solution: Use Java’s concurrency utilities effectively to manage parallelism in big data applications. For instance, consider using the ForkJoinPool or the CompletableFuture framework.
public void parallelProcess(List<Data> dataList) {
ForkJoinPool.commonPool().submit(() -> dataList.parallelStream()
.forEach(data -> {
// Process each data element
})
);
}
Why? Leveraging parallel streams allows you to execute concurrent operations with minimal coding overhead, distributing workload and optimizing data processing speed.
4. Not Using Proper API Features
The Pitfall: Big data frameworks like Spark and Hadoop come with a plethora of APIs offering various functionalities. Developers sometimes underutilize these features, limiting capability and efficiency.
The Solution: Familiarize yourself with the full capabilities of the libraries and frameworks at your disposal. For example, use DataFrames in Spark for more efficient processing of structured data.
Dataset<Row> df = spark.read().json("data.json");
df.filter("age > 21").show();
Why? DataFrames support optimization and are generally much faster than RDDs for processing structured data, meaning better performance for your applications.
5. Ignoring Logging and Monitoring
The Pitfall: In the world of big data, it's easy to overlook logging and monitoring due to the sheer volume of data being processed. This can lead to silent failures that are hard to debug.
The Solution: Implement comprehensive logging and monitoring right from the start. Tools like Apache Log4j can help maintain logs in production efficiently.
import org.apache.log4j.Logger;
public class DataProcessor {
private static final Logger logger = Logger.getLogger(DataProcessor.class);
public void process(List<Data> dataList) {
logger.info("Processing started for " + dataList.size() + " records.");
// Processing logic
logger.info("Processing completed.");
}
}
Why? Proper logging offers insight into application performance and helps debug issues effectively, ultimately leading to more stable applications.
Final Considerations
Big data processing with Java offers impressive capabilities, but developers must be mindful of common pitfalls that can derail performance and efficiency. By focusing on effective memory management, utilizing appropriate serialization techniques, managing parallelism wisely, leveraging framework features, and ensuring robust logging and monitoring, developers can overcome these challenges.
Additional Resources
For further reading, consider these resources:
- Apache Pig for Big Data
- Hadoop: The Definitive Guide
By adopting these best practices and continuously staying informed, you can maximize the potential of big data processing in your Java applications, paving the way for more efficient and scalable data solutions. Happy coding!