Debugging Errors in Apache Camel Routes with Java
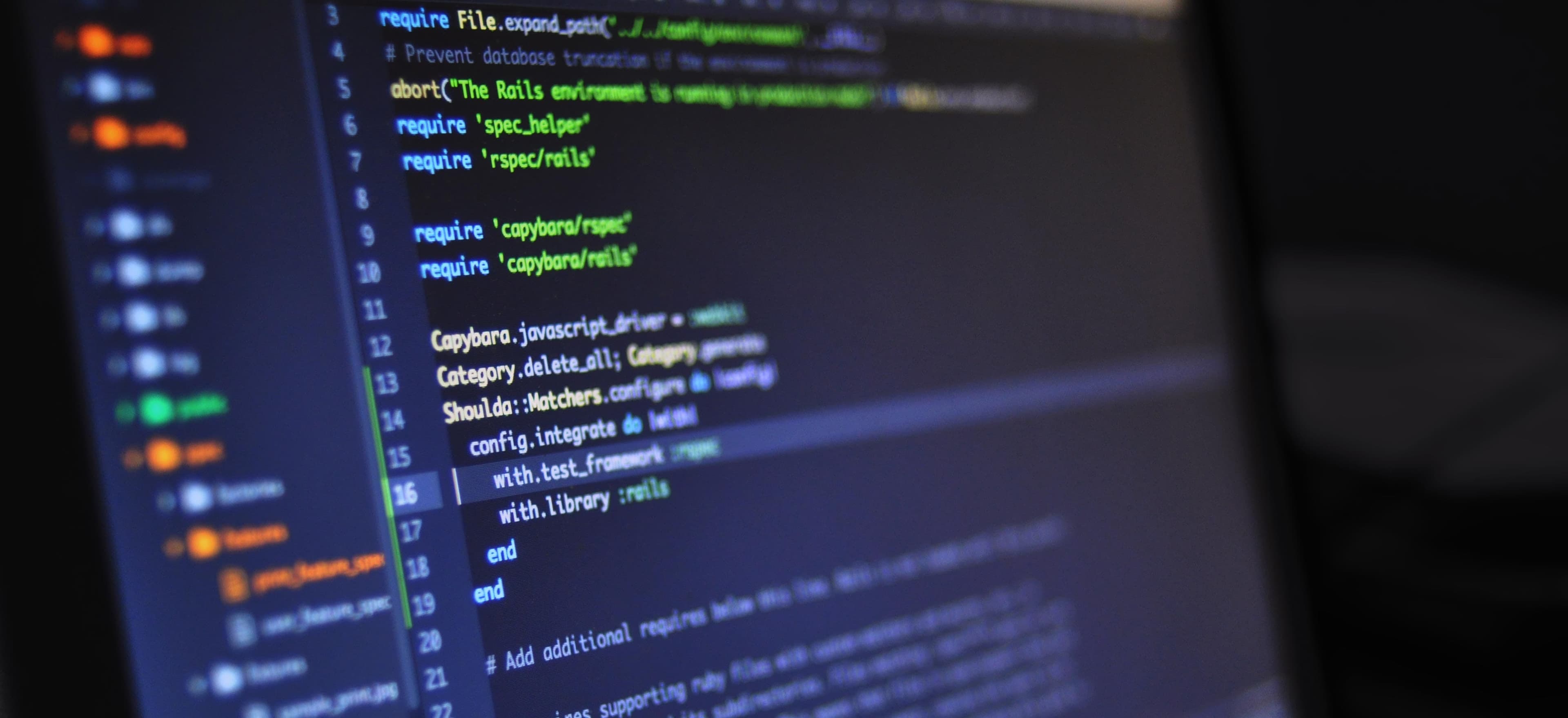
- Published on
Debugging Errors in Apache Camel Routes with Java
Apache Camel is a powerful open-source integration framework that allows you to seamlessly connect various systems, applications, and data sources. While it provides a robust platform for building integration applications, developers often encounter errors during the development and execution phases. Debugging these errors is crucial to ensure smooth data flow and system communication. In this post, we’ll delve into common pitfalls and strategies for debugging errors in Apache Camel routes using Java.
Table of Contents
- Understanding Apache Camel
- Common Errors in Apache Camel Routes
- Debugging Techniques
- Logging
- Camel Error Handling
- Unit Testing
- Using JMX
- Sample Code with Debugging Commentary
- Conclusion
1. Understanding Apache Camel
Apache Camel is built on the Enterprise Integration Patterns (EIP) providing developers with a fluent API to create integration solutions. With its DSL (Domain-Specific Language), Camel allows you to define routes that transfer data between endpoints using various processors and transforms.
An example of a simple Camel route could look like this:
from("direct:start")
.to("log:info")
.to("mock:result");
In this snippet, Camel takes input from the direct:start
endpoint, logs the information, and then sends it to a mock:result
endpoint. This is a basic blueprint, but even simple routes can encounter complex errors.
2. Common Errors in Apache Camel Routes
Debugging is necessary when your routes don’t act as expected. Common errors include:
- Route Not Started: Typically arises when you forget to add a route to the Camel context or miss an endpoint configuration.
- Message Transformation Errors: When data formats are mismatched, such as trying to convert a JSON payload when expecting XML.
- Endpoint Not Found: This occurs when attempting to connect to an unregistered or down endpoint.
- Exception Handling Issues: Failing to handle exceptions properly can lead to message loss.
Understanding these errors is the first step towards effective debugging.
3. Debugging Techniques
Logging
One of the simplest yet most effective ways to debug your Camel routes is through logging. Camel provides built-in logging capabilities, allowing you to log message contents at various points in your route.
Using Camel's logging capabilities:
from("direct:start")
.log("Received message: ${body}")
.to("mock:result");
This route logs every incoming message to the console. To further enhance your logging capabilities, consider configuring logging levels in your logback.xml
file or using log4j
to capture detailed information.
Camel Error Handling
Camel comes with various error handling mechanisms that can be configured at both the route and context level. For instance, you can utilize the ErrorHandler by defining a custom onException
clause:
onException(Exception.class)
.handled(true)
.to("log:error");
from("direct:start")
.process(exchange -> {
throw new RuntimeException("Error processing");
});
In this code:
- The
onException
clause catches anyException
thrown in the route. - The error is logged, allowing you to see detailed error information without losing the entire message.
For a more comprehensive overview of handling errors in Camel, check out the official documentation.
Unit Testing
Writing unit tests is an invaluable practice, especially when trying to debug routes. Camel offers the camel-test
component for creating unit tests for routes.
Example Unit Test:
public class MyRouteTest extends CamelTestSupport {
@Override
protected RouteBuilder createRouteBuilder() {
return new MyRoute();
}
@Test
public void testRoute() throws Exception {
template.sendBody("direct:start", "Test Message");
getMockEndpoint("mock:result").expectedBodiesReceived("Expected Output");
assertMockEndpointsSatisfied();
}
}
In this example, a unit test is created to verify that the route produces the expected output. It ensures the integrity of your route logic before even running it in production.
Using JMX
Java Management Extensions (JMX) can be a powerful ally when debugging Apache Camel routes. Enabling JMX in your Camel context will expose various management endpoints for your routes.
To enable JMX, configure your Camel context like this:
camelContext.setManagementStrategy(new ManagementStrategy());
With JMX enabled, you can use tools like JConsole to connect to your Camel application and inspect the state of various routes, manage instances, and monitor performance.
4. Sample Code with Debugging Commentary
Let's take a look at a more integrated example using various debugging techniques, so you can see how they are applied in a single Camel route:
import org.apache.camel.builder.RouteBuilder;
public class MyComplexRoute extends RouteBuilder {
@Override
public void configure() throws Exception {
// Enable error handling
onException(NullPointerException.class)
.handled(true)
.to("log:error?showAll=true");
// Start the route
from("direct:start")
// Log incoming message
.log("Input Message: ${body}")
.process(exchange -> {
String body = exchange.getIn().getBody(String.class);
if (body == null) {
throw new NullPointerException("Null body received");
}
// Simulated transformation
String transformedBody = body.toUpperCase();
exchange.getIn().setBody(transformedBody);
})
.to("mock:result")
.log("Processed Message: ${body}");
}
}
In this route:
- The
onException
block capturesNullPointerException
s to prevent message loss. - Logging statements provide insights into the original and processed message.
- The
process
block simulates a transformation, ensuring it doesn’t silently fail.
Debugging could include checking logs to ensure that the transformation occurs as expected and that no null values slip through.
5. Conclusion
Debugging errors in Apache Camel routes is essential for maintaining the integrity and reliability of integration solutions. By employing effective logging, robust error handling, diligent unit testing, and utilizing JMX, you can significantly enhance your debugging capabilities.
Understanding the common errors, combined with practical techniques and examples, will prepare you to tackle the challenges in your integration projects. Empower your development with these tools, and you’ll be well on your way to mastering Apache Camel.
For further reading on Apache Camel, consider exploring the detailed official documentation and the rich community resources. Happy coding!
Checkout our other articles