Overcoming Challenges in Java Machine Learning Libraries
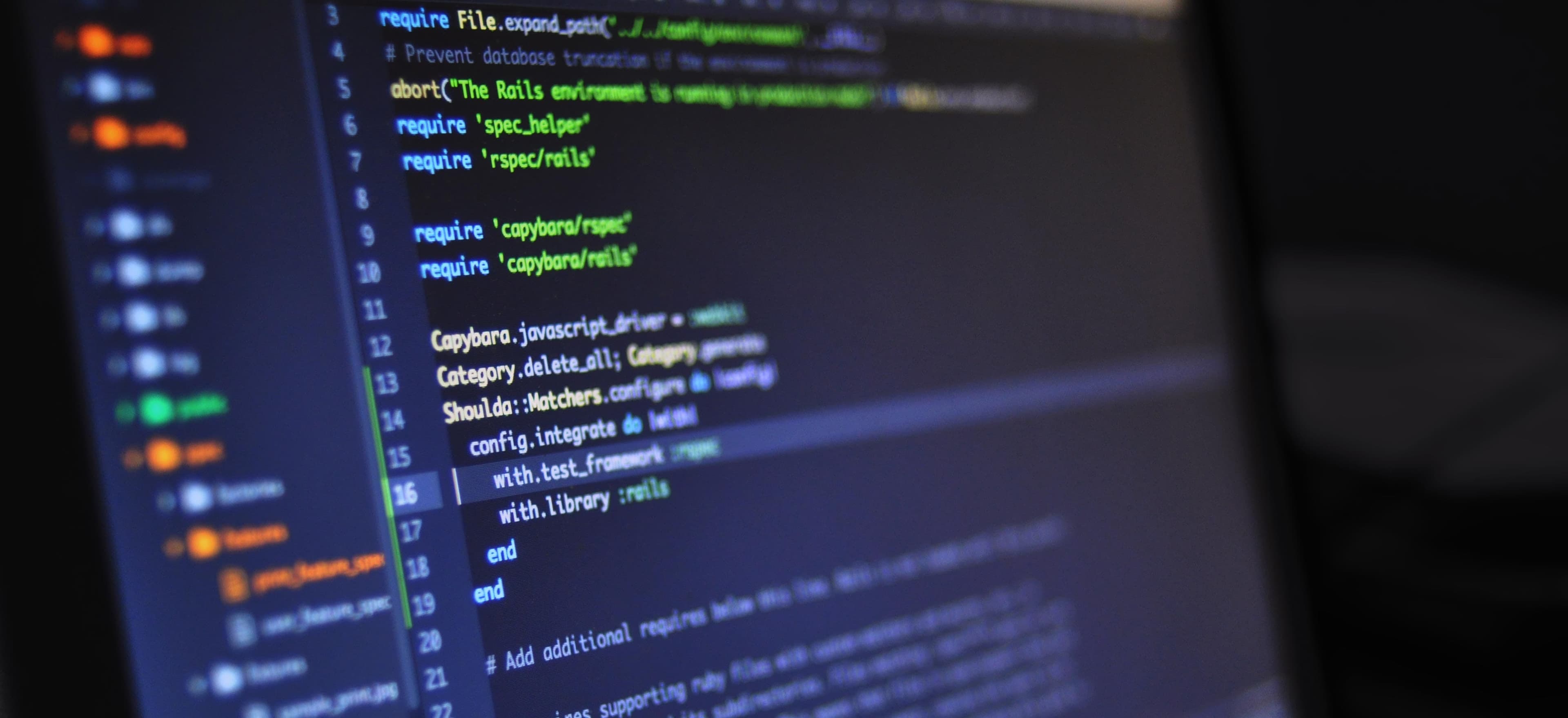
- Published on
Overcoming Challenges in Java Machine Learning Libraries
Java has long been a cornerstone in the world of software development, characterized by its robustness, scalability, and extensive ecosystem. With the evolving field of machine learning (ML), Java remains relevant, largely due to its well-established libraries and frameworks. However, diving into machine learning with Java is not without challenges. This blog post will discuss some common issues faced when utilizing Java machine learning libraries and how to overcome them.
Why Java for Machine Learning?
Before we delve into the challenges, let's focus on why one might choose Java for machine learning:
- Performance: Java offers high performance through its Just-In-Time (JIT) compiler and efficient garbage collection, making it suitable for high-scale applications.
- Concurrency: Java’s built-in support for multi-threading helps in efficiently managing large datasets common in machine learning.
- Integration: Java integrates seamlessly with various systems, making it easier to build applications that require machine learning.
Some widely-used ML libraries in Java include:
- Weka: A collection of algorithms for data mining tasks.
- Deeplearning4j: A deep learning library designed for enterprise environments.
- Apache Spark MLlib: A scalable machine learning library that operates on the open-source Apache Spark framework.
While these libraries provide a robust foundation for building machine learning models, several challenges often arise. Let us explore some of the most common issues.
Challenge 1: Limited Resources and Community Support
The Problem
Although Java is a well-established language, resources specifically for machine learning in Java are relatively limited compared to languages like Python. This limitation can make it difficult for developers, especially beginners, to find tutorials, documentation, or community help.
Solution
To counteract this challenge, consider the following strategies:
-
Utilize Comprehensive Documentation: Libraries like Weka and Deeplearning4j have extensive documentation. Make use of their user guides and Javadoc for better understanding.
-
Engage in Forums and Online Communities: Websites such as Stack Overflow and GitHub repositories are excellent platforms to seek help. Combining efforts with other learners can yield favorable results.
// Example of loading a dataset in Weka
import weka.core.Instances;
import weka.core.converters.ConverterUtils;
public class WekaExample {
public static void main(String[] args) throws Exception {
// Load a dataset file
ConverterUtils.DataSource source = new ConverterUtils.DataSource("path/to/dataset.arff");
Instances data = source.getDataSet();
// Set class attribute
if (data.classIndex() == -1) {
data.setClassIndex(data.numAttributes() - 1);
}
System.out.println(data);
}
}
Why this code matters:
- Data Loading: The
ConverterUtils.DataSource
class is a utility for loading datasets. This abstraction simplifies file I/O operations. - Setting Class Index: By setting the class index, we ensure that the model knows which attribute to predict, a crucial step in any machine learning task.
Challenge 2: Complexity in API Usage
The Problem
Java machine learning libraries often come with complex APIs that can seem daunting to newcomers. The intricate interfaces and methods might lead to misuse or underutilization of the libraries' capabilities.
Solution
To demystify the complexity, consider the following:
-
Start with Basics: Focus on understanding fundamental concepts like classification and regression before diving deep into complex API functionalities.
-
Follow Tutorials: There are many blogs and YouTube tutorials aimed at Java ML libraries. Following these can provide practical examples of how to implement various models.
// Example of building and evaluating a simple decision tree model using Weka
import weka.classifiers.trees.J48;
import weka.classifiers.Evaluation;
public class DecisionTreeExample {
public static void main(String[] args) throws Exception {
// Load dataset
Instances data = // load dataset as shown earlier
// Build a J48 classifier (a type of decision tree)
J48 tree = new J48(); // new instance of tree
tree.buildClassifier(data); // build classifier
// Evaluate model
Evaluation eval = new Evaluation(data);
eval.crossValidateModel(tree, data, 10, new Random(1));
System.out.println(eval.toSummaryString()); // print evaluation results
}
}
Why this code matters:
- J48 Classifier: The J48 algorithm is an implementation of the C4.5 decision tree algorithm. It’s popular for classification tasks and relatively easy to understand.
- Cross-Validation: This snippet shows how to perform cross-validation, which helps assess model performance by partitioning the data into training and testing sets multiple times.
Challenge 3: Lack of Advanced Features
The Problem
While libraries like Deeplearning4j are robust, Java machine learning frameworks may sometimes lag behind other languages in adopting newer algorithms or advanced features, such as those based on deep learning or neural networks.
Solution
To overcome this constraint, integrate Java with other tools and technologies:
-
Use Java with Python: Through the Java Native Interface (JNI) or by leveraging tools like Jython, you can call Python libraries while maintaining your Java codebase.
-
Explore Newer Libraries: Keep an eye on emerging Java libraries that specialize in specific areas of machine learning or deep learning.
// Connecting Java with TensorFlow.js for a Deep Learning Model
import org.tensorflow.Session;
import org.tensorflow.Graph;
public class TensorFlowExample {
public static void main(String[] args) {
// Create a graph
try (Graph graph = new Graph()) {
// Define a simple computation graph
// ...
// Start a new TensorFlow session
try (Session session = new Session(graph)) {
// Execute the session and fetch results
// ...
}
}
}
}
Why this code matters:
- TensorFlow Integration: This snippet illustrates how to create and run a simple TensorFlow graph using Java. TensorFlow’s extensive capabilities allow Java developers to utilize state-of-the-art machine learning techniques.
The Closing Argument
While Java remains a powerful option for machine learning applications, developers must navigate certain challenges. By leveraging comprehensive documentation, engaging with communities, simplifying the learning curve with tutorials, and integrating advanced features from other languages, one can maximize the effectiveness of Java machine learning libraries.
Additional Resources
For more insights on leveraging machine learning in Java, visit Weka’s Official Documentation or check out the Deeplearning4j GitHub page.
In summary, with persistence and strategic approaches, you can successfully overcome these challenges and harness Java's capabilities in machine learning. Happy coding!
Checkout our other articles