Maximizing Performance Monitoring with Java Mission Control
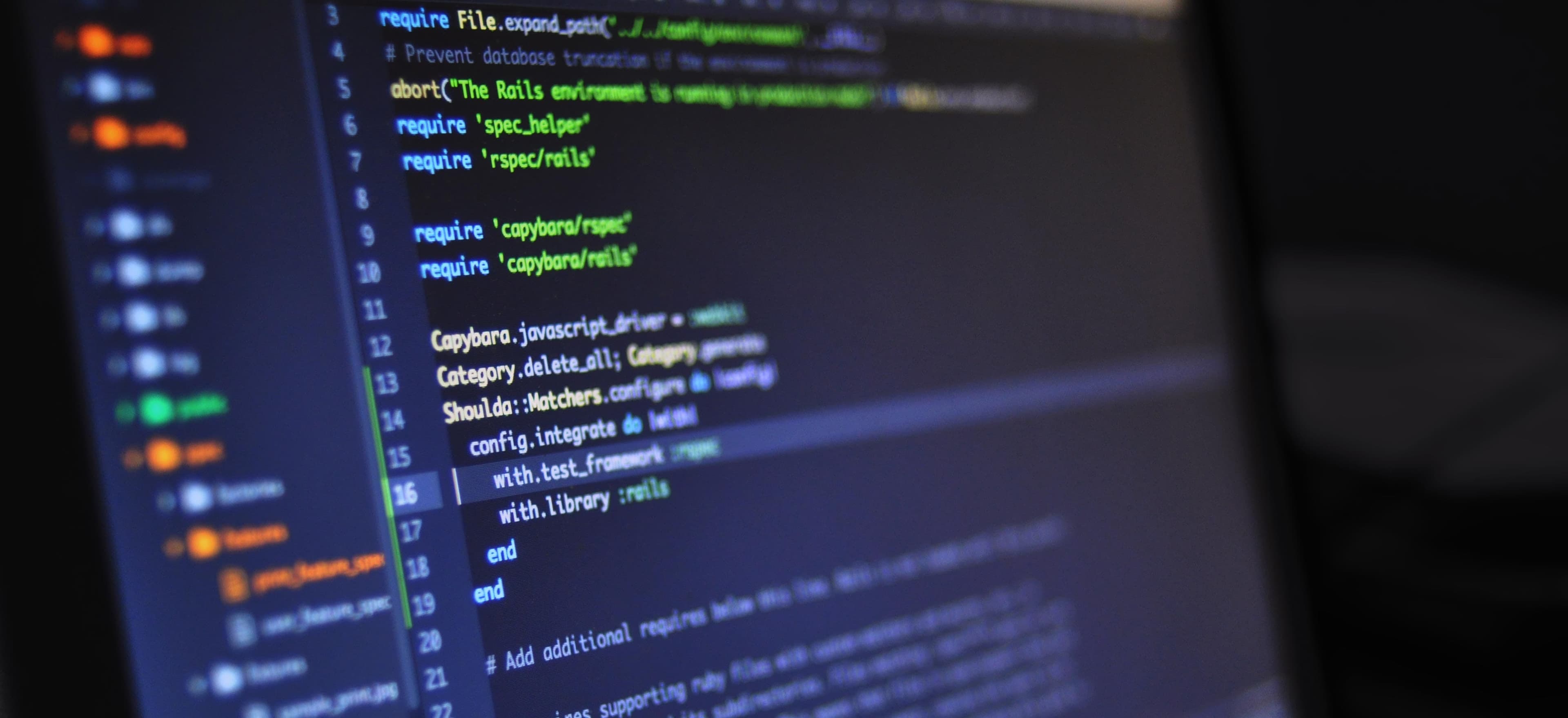
- Published on
Maximizing Performance Monitoring with Java Mission Control
Performance monitoring is critical in ensuring that Java applications run efficiently. With the advent of Java Mission Control (JMC), developers have access to powerful tools that help in profiling and optimizing their applications. This blog post will explore the capabilities of JMC, its components, and best practices for maximizing performance monitoring in your Java applications.
What is Java Mission Control?
Java Mission Control is a set of tools provided by Oracle for monitoring and managing Java applications. It originally emerged as a commercial product but is now available as an open-source tool. JMC provides developers and system administrators with the ability to analyze application performance and diagnose issues via a graphical interface and extensive telemetry data.
Key Features of Java Mission Control
- Low Overhead Profiling: JMC utilizes the Java Flight Recorder (JFR), which captures runtime information from running Java applications with minimal performance impact.
- Detailed Information Visualization: The tool provides a comprehensive user interface for visualizing the data captured by JFR.
- Integrated Analysis Tools: JMC contains several components, such as the JFR analysis tools, the Flight Recorder viewer, and others.
Setting Up Java Mission Control
Before diving into performance monitoring, let’s first discuss how to set up Java Mission Control in your environment.
Installation Steps
- Download JMC: Download the latest JMC version from the Oracle website.
- Install: Follow the installation instructions specific to your operating system. JMC is available on Windows, macOS, and Linux.
- Verify Installation: Launch JMC to ensure it has been installed correctly.
Configuring Your Java Application
To start monitoring, you need to enable Java Flight Recording in your Java application. You can do this by adding the following JVM argument when starting your application:
-javaagent:jmc.jar
This command enables the agent that allows JMC to connect to your Java application during its execution.
Key Components of Java Mission Control
Java Mission Control consists of key components that enable effective performance monitoring.
1. Java Flight Recorder
JFR is the core component of JMC. It records events from the Java Virtual Machine (JVM), such as memory usage, thread activity, and garbage collection. This telemetry data can then be analyzed through JMC to identify performance bottlenecks.
Example of enabling JFR during application runtime:
java -XX:StartFlightRecording=duration=60s,filename=myrecording.jfr -jar myapp.jar
In this command:
-XX:StartFlightRecording
: Starts capturing data.duration=60s
: Specifies the duration of recording.filename=myrecording.jfr
: Output file for captured data.
2. JMC Interface
The JMC user interface offers multiple views, including:
- Overview Chart: Displays overall application performance overview.
- Thread and CPU Utilization: Shows CPU usage patterns over time.
- Memory Usage Details: Provides insights into heap and non-heap memory usage.
3. Custom Events and Application Monitoring
Developers can add custom events to their applications using the java.util.logging
framework. Adding these events allows for more comprehensive monitoring tailored to specific application needs.
Example of custom event in your application:
import java.util.logging.Logger;
public class MyApplication {
private static final Logger LOGGER = Logger.getLogger(MyApplication.class.getName());
public void performTask() {
// Custom Event Logging
LOGGER.info("Task started");
...
LOGGER.info("Task completed");
}
}
By doing this, you can differentiate between various application states and contextualize performance data accordingly.
Analyzing Recorded Data
Once you've recorded data, the next step is to analyze it using the JMC viewer. Here are some tips for effective analysis:
1. Identify Performance Bottlenecks
Use the Memory Analysis and Thread Activity views to identify possible performance bottlenecks. Look for memory leaks, excessive thread contention, or high garbage collection times.
2. Use Profiling Tools
JMC allows you to explore profiling options like heap dumps and CPU profiling. This data helps in identifying what parts of the application consume the most resources.
-XX:HeapDumpOnOutOfMemoryError
This JVM argument can be useful for analyzing heap dumps after an application crashes due to memory exhaustion.
3. Set Up Alerts for Thresholds
Establish thresholds for metrics (like CPU usage, memory consumption, and thread counts) within JMC. Implement notifications that alert you when these thresholds are surpassed, allowing you to react promptly to potential issues.
Best Practices for Performance Monitoring
To get the most out of Java Mission Control, consider the following best practices:
1. Regular Monitoring
Regular monitoring helps track application performance over time, facilitating proactive optimizations.
2. Prioritize Key Metrics
Focus on key application metrics:
- Response Time
- Memory Usage
- Thread Count
- Disk I/O Statistics
By concentrating on these areas, you can quickly identify significant performance issues.
3. Continuously Update JMC
As JMC evolves, so does its performance monitoring capabilities. Make sure to stay up to date with the latest versions and features available.
4. Automate Monitoring and Reporting
Consider automation tools to facilitate ongoing monitoring and reporting, allowing you to maintain application performance without manual intervention.
Wrapping Up
Performance monitoring is essential for maintaining the health and efficiency of your Java applications. Java Mission Control, with its integration of the Java Flight Recorder, offers a robust set of tools for developers to analyze application performance with minimal overhead. By enabling detailed monitoring, identifying bottlenecks, and implementing best practices, you can ensure that your Java applications operate at optimal efficiency.
By employing JMC in your development workflow, you can enhance your application’s performance and ensure a better experience for your users. Don't forget to explore additional resources in the Java community for continuous learning and insights into performance optimization.
Happy coding!
Checkout our other articles