Maximizing Performance Insights with Java Flight Recorder
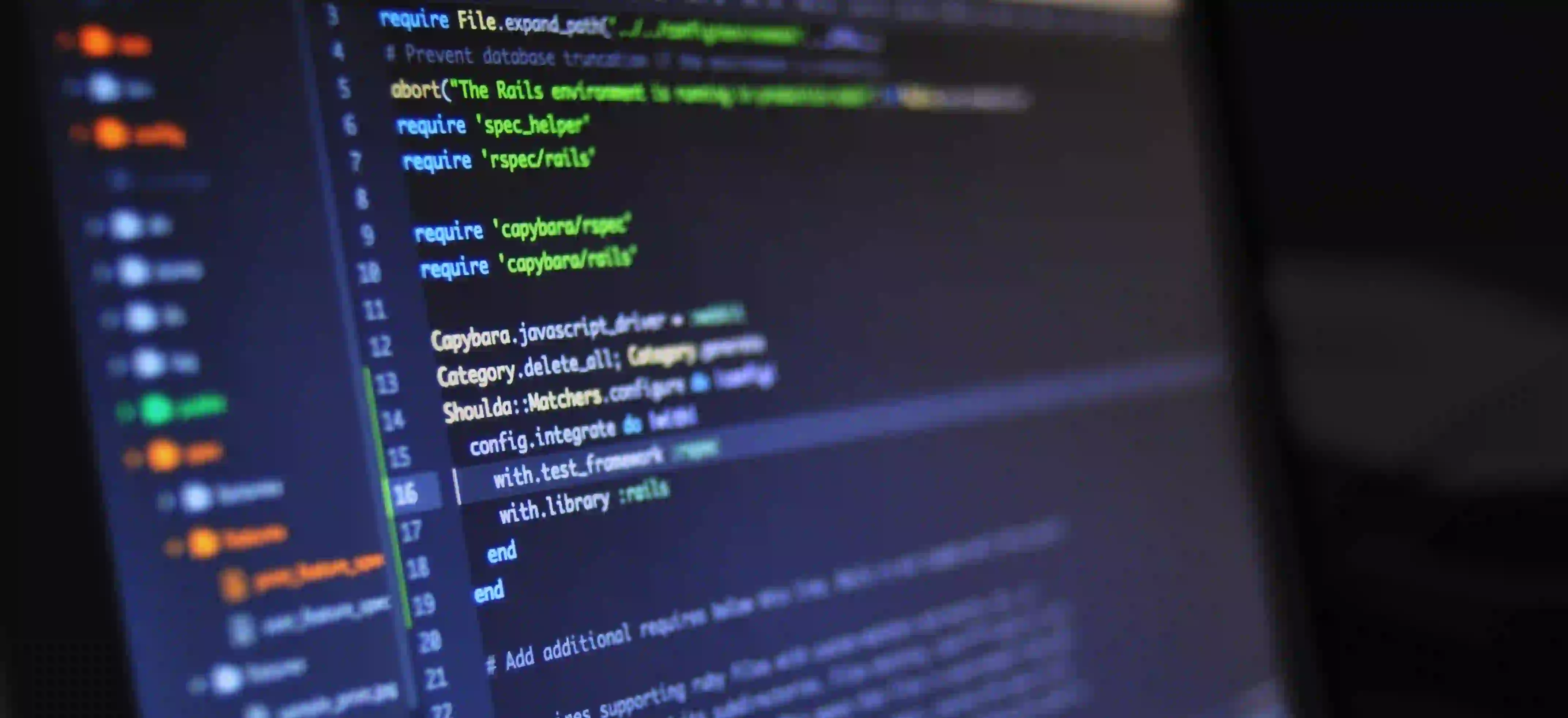
Maximizing Performance Insights with Java Flight Recorder
Java Flight Recorder (JFR) is one of the most powerful yet underutilized tools in the Java ecosystem for performance monitoring and analysis. Built into the Java Virtual Machine (JVM), JFR offers developers and system administrators a low-overhead profiling solution. In this blog post, we will delve into JFR, explore its features, and illustrate its usage with practical code examples. By the end of this article, you will be equipped to leverage JFR for maximizing performance insights in your Java applications.
What is Java Flight Recorder?
Java Flight Recorder is a profiling tool that captures JVM events over time. It collects various metrics, including CPU usage, memory consumption, garbage collection events, thread contention, and much more. The beauty of JFR is its minimal impact on application performance. Unlike traditional profiling methods, which may alter application behavior, JFR operates with low overhead, making it suitable for production environments.
Key Features of JFR
- Low Overhead: JFR incurs minimal CPU and memory overhead, allowing for continuous monitoring without affecting application performance significantly.
- Detailed Metrics: It captures a wide range of events, from garbage collection pauses to method invocations, providing deep insights into application performance.
- Integration with JDK: JFR is built into the JDK, starting from Java 11, making it readily available for developers.
- Event Streaming: JFR supports real-time event streaming, which helps in monitoring applications live.
- Historical Analysis: Once flight recordings are collected, they can be analyzed later using tools like Java Mission Control (JMC).
Getting Started with Java Flight Recorder
To start using JFR, ensure that you're running on Java 11 or later. If you are still using an older Java version, consider upgrading to take full advantage of JFR and other performance improvements.
Enabling Java Flight Recorder
You can enable JFR via command line options when starting your Java application. The following command illustrates how to do this:
java -XX:StartFlightRecording=duration=60s,filename=recording.jfc -jar myapp.jar
In this example, we start a flight recording for a duration of 60 seconds and write the output to recording.jfc
.
Creating a Flight Recording Programmatically
For programmatic control and more sophisticated usage, Java provides an API for flight recordings. You can utilize the jdk.jfr
package as follows:
import jdk.jfr.Configuration;
import jdk.jfr.Recording;
public class JFRExample {
public static void main(String[] args) throws InterruptedException {
Configuration configuration = Configuration.getConfiguration("default");
Recording recording = new Recording(configuration);
recording.start();
// Application logic would go here
Thread.sleep(5000); // Simulating an application runtime
recording.stop();
recording.dump("recording.jfr");
}
}
Code Commentary
Configuration.getConfiguration("default")
: This line retrieves configuration settings for the JFR recording.recording.start()
: Starts the flight recording.Thread.sleep(5000)
: Represents the application runtime where performance metrics will be gathered.recording.stop()
andrecording.dump("recording.jfr")
: Stop the recording and save the results.
This example provides a very basic way to gather performance metrics programmatically.
Analyzing Flight Recordings
Once you have created and saved a flight recording, it is essential to analyze the data. For this purpose, Java Mission Control (JMC) is an ideal tool. JMC allows you to visualize the captured data, drill down into specific events, and identify performance bottlenecks.
Importing JFR Files into JMC
- Open Java Mission Control.
- Click on “File” and then “Open.”
- Select the
.jfr
file you saved.
Upon loading the file, JMC provides several graphs and charts to help dissect the performance metrics recorded.
Real-World Use Cases for Java Flight Recorder
1. Monitoring Garbage Collection
Garbage collection (GC) can be a primary source of performance degradation in Java applications. Using JFR, you can monitor the behavior of the garbage collector and adjust settings to optimize performance.
@Event
public class GCEvent {
@Label("Garbage Collection")
@Description("Garbage collection event")
private String collectionType;
// additional metrics...
}
Here, you can define a custom event for garbage collection, which will allow you to gain even more insights during the analysis phase.
2. Performance Tuning
JFR can help you identify areas of your application that could benefit from performance tuning. By analyzing CPU usage metrics, you can gauge which methods are consuming the most resources.
3. Thread Contention
Thread contention issues can lead to significant performance bottlenecks. With JFR, you can identify where threads are waiting on locks:
java -XX:StartFlightRecording=duration=60s -XX:UnlockDiagnosticVMOptions -jar myapp.jar
Look for events labelled "Lock contention" in the recording to understand these bottlenecks better.
4. Analyzing Application Crashes
In scenarios where your application crashes, JFR can provide invaluable data leading up to the event, helping you diagnose what went wrong.
Best Practices for Using Java Flight Recorder
- Use in Production: Don’t hesitate to use JFR in production environments. Its low overhead makes it a safe profiling tool.
- Regular Monitoring: Make flight recordings a part of your deployment process to continuously monitor performance.
- Combine with Other Tools: Consider using JFR in conjunction with other profiling tools like VisualVM for comprehensive insights.
- Automate Recordings: Make periodic recordings based on application metrics to catch irregularities.
The Bottom Line
Java Flight Recorder offers significant advantages for performance profiling with minimal overhead. By granting insights into the Java application runtime, it empowers developers to make informed decisions on performance optimizations. As you integrate JFR into your workflow, you will discover new levels of efficiency and responsiveness in your Java applications.
By using JFR effectively, you position yourself to handle performance issues proactively rather than reactively. Empower yourself with tools that provide clarity in the complexities of application performance.
For more resources on Java Flight Recorder, you can check the official documentation on OpenJDK and deep dive into advanced topics like Java Mission Control for a more enriched analysis experience.
Happy coding, and may your applications run smoother than ever!