Common Pitfalls in Java Module System Implementation
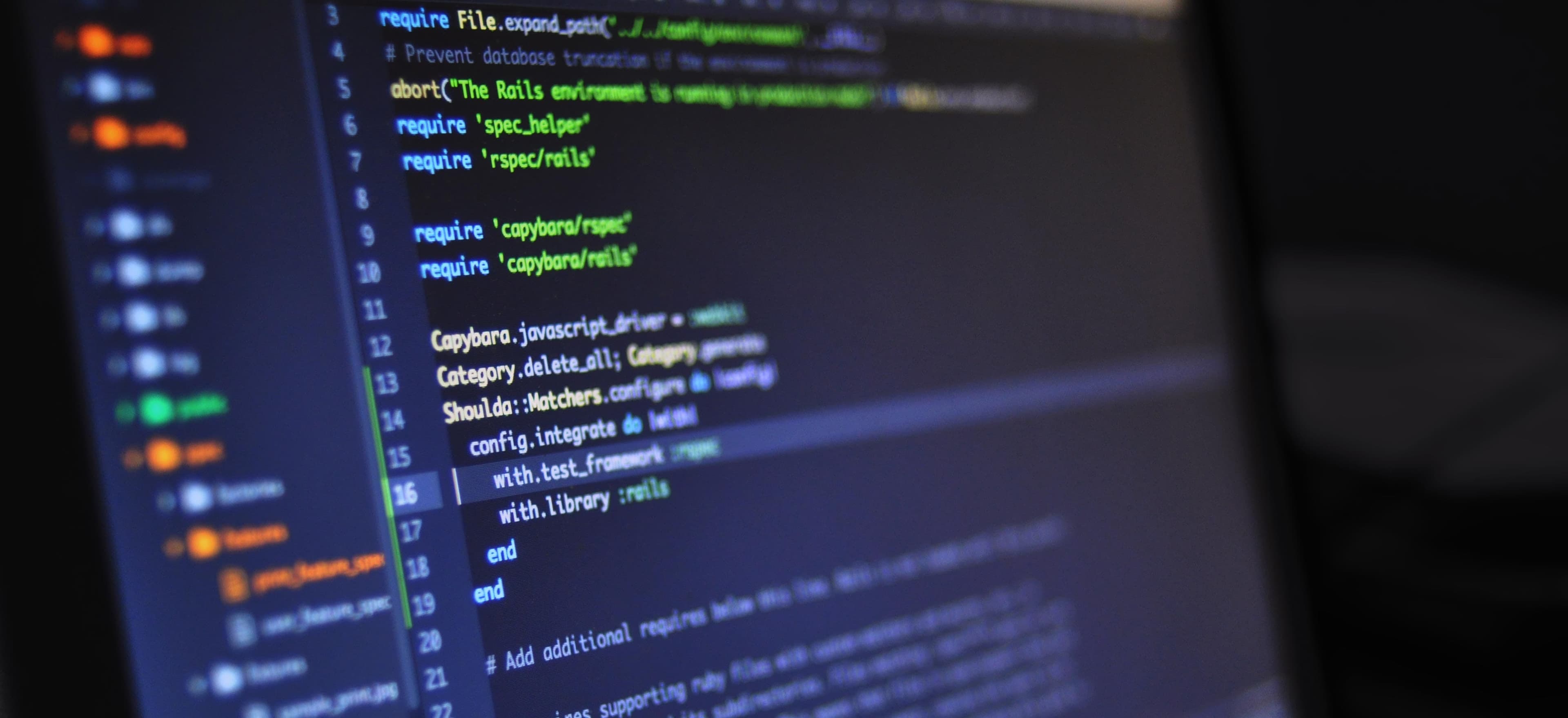
- Published on
Common Pitfalls in Java Module System Implementation
The Java Module System, introduced in Java 9 with Project Jigsaw, was designed to improve Java's modularity, encapsulation, and maintainability. However, its implementation can lead to various pitfalls that developers must navigate. In this blog post, we'll explore common issues encountered when working with the Java Module System and how to overcome them.
Understanding the Java Module System
Before delving into the pitfalls, it's essential to understand a few core concepts of the Java Module System:
-
Modules: A module is a named, self-describing collection of code and data. It consists of a
module-info.java
file that declares the module's dependencies and exported packages. -
Encapsulation: Modules promote strong encapsulation of packages. Only packages declared in the
module-info.java
file can be accessed by other modules. -
Dependencies: The
requires
directive establishes dependencies between modules, which can prevent conflicts and improve the module pathway.
Understanding these concepts allows developers to make informed decisions while implementing modules.
Common Pitfalls of the Java Module System
1. Forgetting the module-info.java
File
The module-info.java
file is integral to Java's Module System, serving as the gateway for defining module boundaries and dependencies. A common pitfall is neglecting its creation.
Solution:
Always create a module-info.java
file in the root of your module. Here's a simple example:
module com.example.myapp {
requires transitive com.example.utils;
exports com.example.myapp.api;
}
In this snippet, com.example.myapp
declares a dependency on another module, com.example.utils
, and exports the package com.example.myapp.api
. The use of transitive
means any module that depends on com.example.myapp
will also have access to com.example.utils
.
2. Inadequate Module Visibility
Another common mistake is incorrect package visibility. Developers may assume all packages within a module are accessible, leading to IllegalAccessError
at runtime.
Solution:
Be explicit about what packages to export in the module-info.java
. Only export the packages that need to be accessible by other modules.
module com.example.service {
exports com.example.service.publicapi;
// Do not export com.example.service.internal, as it's intended for internal use.
}
This snippet demonstrates how to control access to certain packages, improving encapsulation.
3. Circular Dependencies
Circular dependencies among modules can create complex issues. They can result in runtime errors or prevent modules from loading correctly.
Solution:
Ideally, design your module structure to avoid circular dependencies. However, if unavoidable, consider restructuring the dependencies or creating a new module to house shared functionality.
// Example demonstrating potential circular dependency.
module com.example.moduleA {
requires com.example.moduleB; // Avoid circular dependency
}
module com.example.moduleB {
requires com.example.moduleC; // Create a third module for shared functionality
}
In this example, by introducing a third module, you prevent the circular dependency between moduleA
and moduleB
.
4. Lack of Transitive Requirements
Modules often rely on other modules indirectly. Forgetting to declare transitive dependencies can lead to runtime failures.
Solution:
Always analyze module dependencies. If a module A relies on module B, and module B further relies on module C, declaring requires transitive
in module A ensures that consumers of A can access C.
module com.example.moduleA {
requires transitive com.example.moduleB; // Module B will also make module C available to dependents
}
This declaration ensures that anyone depending on moduleA
will automatically have access to moduleB
and its transitive dependencies.
5. Poor Tooling Support
While the Java Module System is a powerful feature, some IDEs and build tools may not have full support yet, leading to confusion.
Solution:
Stay updated with your IDE's capabilities. For example, in Maven, ensure that you are using plugins compatible with the module system such as the maven-compiler-plugin
and specify the release
option:
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>11</source>
<target>11</target>
<compilerArgs>
<arg>--release</arg>
<arg>11</arg> <!-- Use the appropriate release version -->
</compilerArgs>
</configuration>
</plugin>
This specifies that you're targeting a specific version, which ensures compatibility with the module system.
6. Dependency Versioning Issues
Using incompatible versions of end-user library modules can lead to ClassNotFoundException
or NoClassDefFoundError
. These errors arise when there is a mismatch in the expected modules.
Solution:
Utilize a Dependency Management System like Maven, Gradle, or others to keep track of your module versions. Use a bill of materials (BOM) to manage dependencies easily.
Here’s an example of how you can declare a BOM in Maven:
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>my-bom</artifactId>
<version>1.0.0</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
This approach helps ensure that your modules are consistent and functioning correctly.
Key Takeaways
The Java Module System offers powerful benefits in terms of code organization and encapsulation. However, it also introduces unique challenges that developers must be aware of when implementing modules. By understanding the common pitfalls discussed in this article, you can set your modules up for success.
For further reading, you may explore the official Java Tutorials on Module System for an in-depth understanding of the subject. Additionally, consider reading Project Jigsaw's insights to appreciate the motivations behind these changes.
Navigating the Java Module System effectively can enhance your development process, making it more modular and maintainable. Always keep your code clean, adhere to good practices, and stay informed on updates in the Java ecosystem to fully leverage this powerful feature.