Avoiding NullPointerExceptions: Key Strategies for Developers
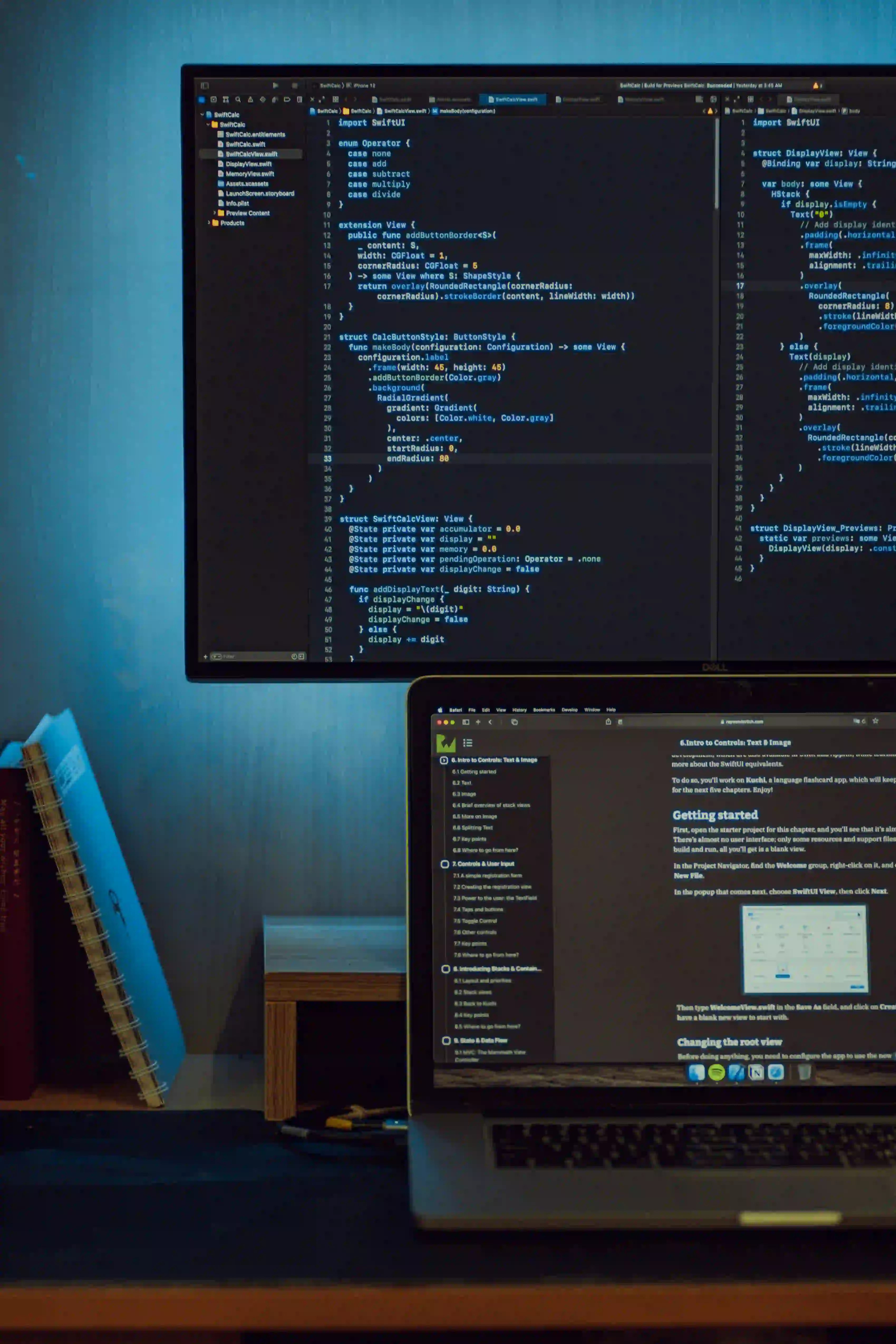
Avoiding NullPointerExceptions: Key Strategies for Developers
Java developers often encounter NullPointerExceptions
(NPEs), one of the most common errors that can disrupt the execution of their applications. Understanding how to avoid these can greatly enhance stability and reduce debugging time. In this blog post, we will explore effective strategies to prevent NullPointerExceptions
, along with relevant code snippets and best practices.
What Is a NullPointerException?
A NullPointerException
occurs when the Java Virtual Machine (JVM) attempts to access an object or variable that has not been instantiated yet. This can lead to system crashes, data inconsistencies, and a poor user experience, which is why avoiding them is critical.
Example of a NullPointerException
Consider the following code snippet:
public class Example {
public static void main(String[] args) {
String name = null;
System.out.println(name.length()); // Throws NullPointerException
}
}
In the example above, we attempt to access the length of a null
string, leading to a NullPointerException
. Now that we understand what causes NPEs, let’s discuss key strategies to avoid them.
1. Use Optional
Java 8 introduced the Optional
class, which is a container for nullable objects. It provides a way to explicitly handle the absence of a value, thereby reducing the likelihood of NPEs.
Example Implementation
import java.util.Optional;
public class OptionalExample {
public static void main(String[] args) {
Optional<String> name = Optional.ofNullable(null);
// Instead of .get() which may cause NPE, use .orElse()
System.out.println(name.orElse("Default Name")); // Prints: Default Name
}
}
In this code, Optional.ofNullable()
creates an Optional
that can either hold a value or be empty. Instead of throwing an NPE, we can provide a default value using orElse()
.
2. Initialize Variables
Another effective way to avoid NPEs is to ensure that we always initialize our variables. This simple step can save developers a lot of trouble.
Example of Initialization
public class InitializationExample {
private String value = ""; // Initialized to an empty string
public String getValue() {
return value;
}
public static void main(String[] args) {
InitializationExample example = new InitializationExample();
System.out.println(example.getValue()); // Prints: ""
}
}
By initializing value
to an empty string, we reduce the chances of encountering an NPE later on in the code.
3. Use Annotations
Annotations such as @NonNull
and @Nullable
can help document your code and make it easier to manage nullability at compile time. Tools like SpotBugs can analyze your code and warn about potential NPEs.
Example of Annotations
public class AnnotationsExample {
public void display(@NonNull String name) {
System.out.println("Hello, " + name);
}
public static void main(String[] args) {
AnnotationsExample example = new AnnotationsExample();
example.display(null); // This should prompt a warning if the annotation is respected
}
}
In this example, the @NonNull
annotation indicates that name
cannot be null. Using good IDEs like IntelliJ or Eclipse with support for these annotations helps catch potential issues before runtime.
4. Perform Explicit Null Check
Sometimes, the simplest solution is to check for null explicitly. This ensures that your program can gracefully handle situations where a variable might not be initialized.
Example of Null Check
public class NullCheckExample {
private String name;
public void setName(String name) {
this.name = name;
}
public void printGreeting() {
if (name != null) {
System.out.println("Hello, " + name);
} else {
System.out.println("Hello, World!");
}
}
public static void main(String[] args) {
NullCheckExample example = new NullCheckExample();
example.printGreeting(); // Prints: Hello, World!
}
}
This code snippet checks if name
is null before trying to use it, providing a safe fallback.
5. Use Collection Classes Judiciously
When working with collections such as lists or maps, ensure that you do not accidentally add nulls. This can lead to NPEs during iterations or when trying to access specific keys in the collection.
Example with Collection
import java.util.HashMap;
import java.util.Map;
public class CollectionExample {
private Map<String, String> map = new HashMap<>();
public void putValue(String key, String value) {
// Avoid adding null values
if (key != null && value != null) {
map.put(key, value);
}
}
public static void main(String[] args) {
CollectionExample example = new CollectionExample();
example.putValue("name", null); // Will not add to the map
}
}
In this case, the putValue
method ensures that both key
and value
are not null before inserting them into the map.
6. Consider Using Default Values
Sometimes, providing a default value can be a good practice when handling potential nulls. This can help avoid the need for multiple null checks throughout your code.
Example of Default Values
public class DefaultValueExample {
private static final String DEFAULT_NAME = "Default Name";
public String getName(String name) {
return name != null ? name : DEFAULT_NAME;
}
public static void main(String[] args) {
DefaultValueExample example = new DefaultValueExample();
System.out.println(example.getName(null)); // Prints: Default Name
}
}
In this code, we provide a default name when name
is null, keeping our logic simple and clean.
7. Leverage Lombok's @NonNull
If you're using Lombok in your Java project, the @NonNull
annotation can automatically generate null checks for you.
Example with Lombok
import lombok.NonNull;
public class LombokExample {
public void greet(@NonNull String name) {
System.out.println("Hello, " + name);
}
public static void main(String[] args) {
LombokExample example = new LombokExample();
example.greet("Alice");
// example.greet(null); // This line will trigger a NullPointerException
}
}
By utilizing Lombok, you can reduce boilerplate code while still effectively managing nullability.
Lessons Learned
Avoiding NullPointerExceptions
is a crucial skill for Java developers. By integrating the strategies we discussed—like leveraging Optional
, performing null checks, using annotations, and possibly employing libraries such as Lombok—you can significantly reduce the chances of encountering these errors in your applications.
For further reading, check out resources such as Effective Java by Joshua Bloch, which offers in-depth insights into best coding practices in Java.
Adopting these strategies will not only help you write cleaner code but will also improve your overall software quality and enhance user satisfaction.
This blog post aims to provide developers with a comprehensive guide to avoiding NullPointerExceptions
. By incorporating thoughtful coding practices and tools, developers can create robust applications and maintain a smoother user experience. Let us know in the comments if you have additional methods to prevent NPEs!