Mastering Callbacks: Common Pitfalls in Java Async Programming
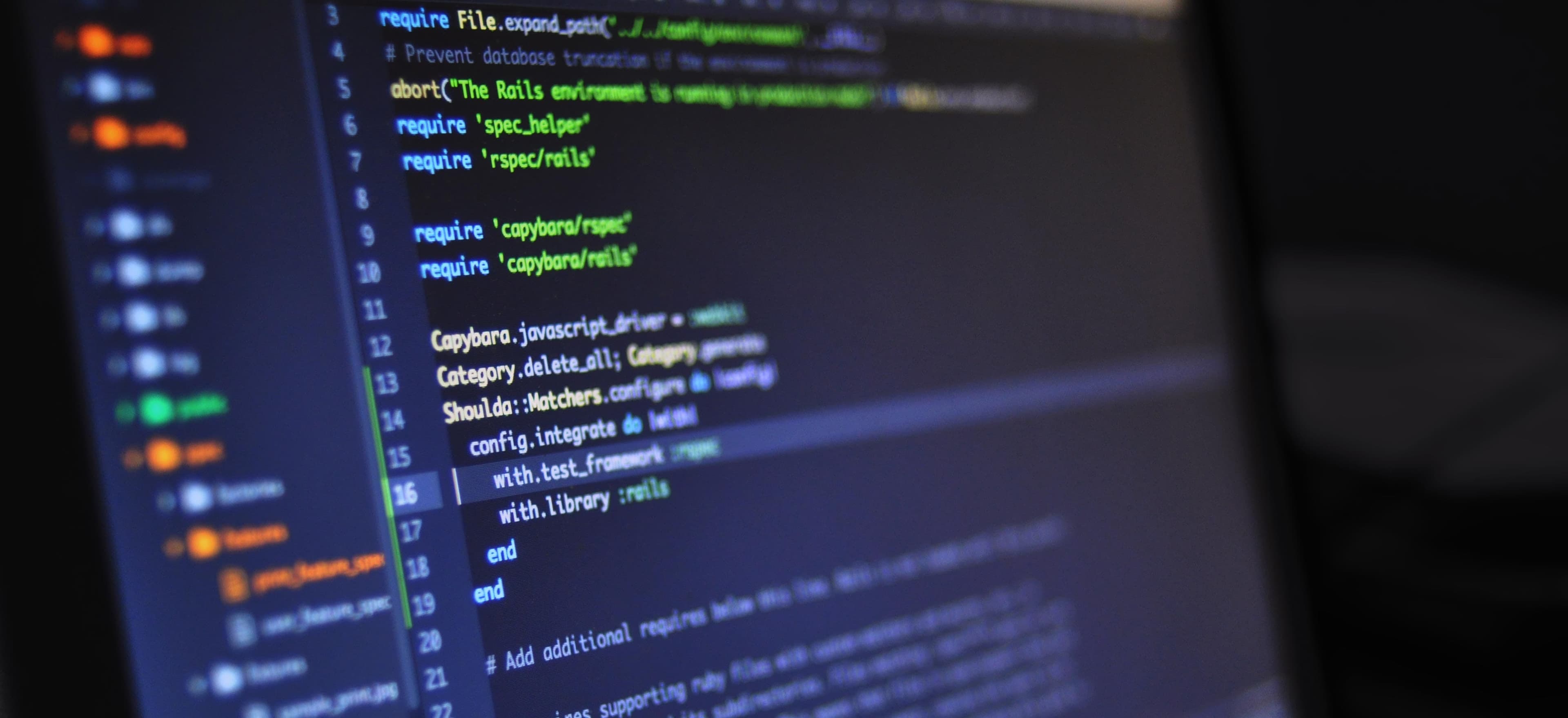
- Published on
Mastering Callbacks: Common Pitfalls in Java Async Programming
Java has long maintained its reputation as a robust and versatile programming language, suitable for various applications. As we transition into a world dominated by asynchronous programming, understanding callbacks becomes crucial. Callbacks allow for efficient task management, enabling developers to execute code after a task completes without blocking the main thread. However, like any powerful tool, callbacks come with their own set of challenges. This blog post will delve into the common pitfalls of async programming in Java, focusing on callbacks, and provide you with best practices to navigate them effectively.
What Are Callbacks?
In Java, a callback is a mechanism that allows a method to execute a piece of code (or function) when a certain event occurs or a task completes.
Example of a Simple Callback
Let’s start with a basic example of a callback in Java.
class AsyncTask {
public void executeTask(Callback callback) {
// Simulating an asynchronous task
new Thread(() -> {
try {
Thread.sleep(2000); // Simulating time-consuming task
} catch (InterruptedException e) {
e.printStackTrace();
}
// Task completed; trigger the callback
callback.onComplete("Task Finished!");
}).start();
}
}
interface Callback {
void onComplete(String result);
}
public class Main {
public static void main(String[] args) {
AsyncTask task = new AsyncTask();
task.executeTask(result -> System.out.println(result)); // Using a lambda expression for the callback
}
}
Commentary: In the above code, the method executeTask
performs a simulated time-consuming task by sleeping for 2 seconds and then triggers the callback to signify completion. The use of a lambda expression provides a concise way to define behavior for the onComplete
method.
Common Pitfalls of Callbacks in Java Async Programming
Asynchronous programming with callbacks can lead to various issues if not handled properly. Here are some common pitfalls you should be aware of:
1. Callback Hell
Callback hell refers to the situation when you nest multiple callbacks within each other, making the code harder to read and maintain.
task1.executeTask(result1 -> {
task2.executeTask(result2 -> {
task3.executeTask(result3 -> {
// ... more nested callbacks
});
});
});
Solution: Refactor nested callbacks into separate methods or use CompletableFuture, which allows you to chain tasks in a more readable way.
2. Error Handling
Asynchronous tasks can fail, but improper error handling in callbacks can lead to silent failures or crashes.
task.executeTask(result -> {
// Assume something goes wrong here
throw new RuntimeException("Task failed");
});
Solution: Always encapsulate your callback logic with error handling. Here’s how you can do it:
task.executeTask(result -> {
try {
// Process the result
} catch (Exception e) {
System.err.println("Error occurred: " + e.getMessage());
}
});
3. Memory Leaks
By holding references to outer classes or variables, callbacks can inadvertently create memory leaks.
class SomeClass {
void someMethod() {
AsyncTask task = new AsyncTask();
task.executeTask(result -> {
// This reference to 'this' can potentially cause a memory leak
System.out.println(this);
});
}
}
Solution: Use weak references, or be mindful of the scope in which you define your callbacks. Alternatively, using static context can avoid unnecessary object retention.
Leveraging CompletableFuture for Better Async Management
Java 8 introduced CompletableFuture
, which simplifies asynchronous programming significantly. It allows chaining and better error management. Here is how you can replace callbacks with CompletableFuture
.
import java.util.concurrent.CompletableFuture;
public class Main {
public static void main(String[] args) {
CompletableFuture<String> future = CompletableFuture.supplyAsync(() -> {
try {
Thread.sleep(2000); // Simulating time-consuming task
} catch (InterruptedException e) {
e.printStackTrace();
}
return "Task Finished!";
});
future.thenAccept(result -> System.out.println(result))
.exceptionally(ex -> {
System.err.println("Error occurred: " + ex.getMessage());
return null;
});
}
}
Why Use This Approach: CompletableFuture
makes your asynchronous code easier to read and less prone to the issues mentioned earlier. You can chain multiple operations without nesting, and error handling is straightforward with the exceptionally
method.
Best Practices for Callback and Async Programming in Java
- Use Executors for Better Thread Management: Avoid creating new threads manually. Use the
ExecutorService
to manage thread pools.
ExecutorService executor = Executors.newFixedThreadPool(4);
executor.submit(() -> {
// Task logic here
});
-
Limit Callback Complexity: Keep callbacks simple and short. If they grow too complex, consider refactoring into multiple methods or using a different concurrency mechanism.
-
Stick to Standard APIs: Leverage built-in Java libraries for asynchronous tasks, such as
CompletableFuture
, instead of reinventing the wheel with custom implementations. -
Implement Timeouts: For tasks that could potentially hang or take too long, use timeouts to handle such scenarios gracefully.
future.completeOnTimeout("Default Value", 2, TimeUnit.SECONDS)
.thenAccept(System.out::println);
- Document Your Code: Always comment on your callbacks and asynchronous code. Future maintainers (including yourself) will appreciate your clear documentation.
To Wrap Things Up
Mastering callbacks in Java asynchronous programming is imperative for anyone looking to build robust, scalable applications. The pitfalls of callback hell, error handling, and memory management can be daunting, but understanding and implementing thoughtful coding practices can avert these issues. By leveraging tools like CompletableFuture
, you can create cleaner, more manageable asynchronous code.
The takeaway? Embrace the power of callbacks, but do so carefully. Happy coding!
For more information, check out the official Java Concurrency tutorial.
By adopting these practices and being mindful of potential pitfalls, you'll enhance your Java async programming skills and build more maintainable applications. Would you like to dive deeper into specific async patterns? Let us know in the comments below!
Checkout our other articles