Overcoming Common Pitfalls in Java Annotation Processing
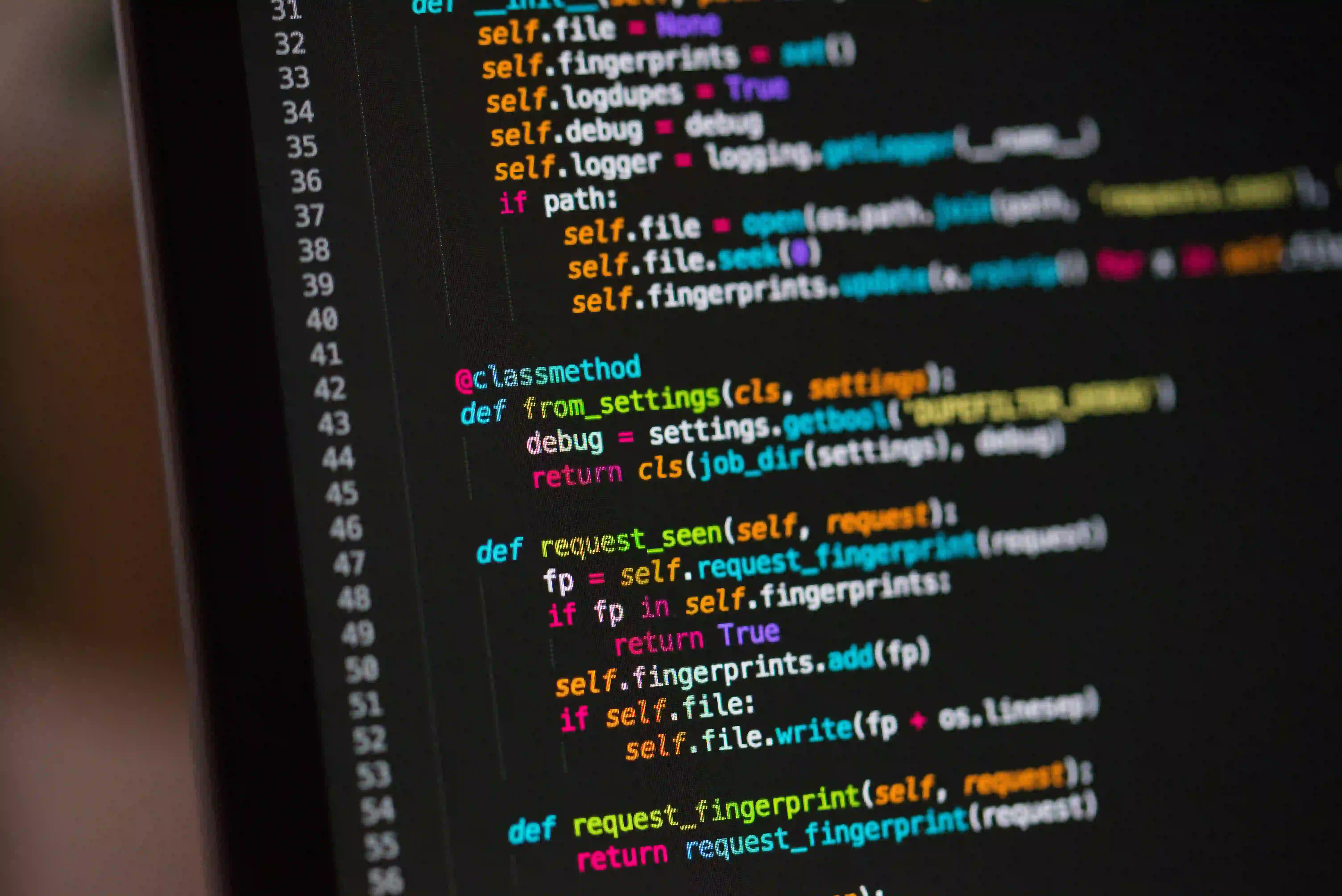
Overcoming Common Pitfalls in Java Annotation Processing
Java annotation processing can be a powerful tool for developers aiming to streamline their code and enforce design constraints, but it is also fraught with potential pitfalls. Many developers encounter issues that can make their use of annotation processing cumbersome or error-prone. In this blog post, we’ll go through some of the most common issues that arise with Java annotation processing, why they happen, and how you can overcome them.
What is Annotation Processing?
Annotation processing in Java is a powerful tool that enables programmers to write code that can inspect and modify other code at compile time. Annotations allow developers to provide metadata about their program, which can be used by the annotation processor during the build process. This can lead to automatic code generation, validation, and even configuration.
Benefits of Annotation Processing:
- Compile-time checks: Helps catch errors early in the development lifecycle.
- Code Generation: Can automatically produce boilerplate code, thus reducing development time.
- Custom Metadata: Provides a way to add specific behavior to classes without altering their implementation.
A Simple Example
To illustrate the concept of annotation processing, let’s start with a simple custom annotation and an annotation processor.
// Define a custom annotation
@Retention(RetentionPolicy.SOURCE)
@Target(ElementType.FIELD)
public @interface NotNull {
}
// Custom Annotation Processor
@SupportedAnnotationTypes("NotNull")
@SupportedSourceVersion(SourceVersion.RELEASE_8)
public class NotNullProcessor extends AbstractProcessor {
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element element : roundEnv.getElementsAnnotatedWith(NotNull.class)) {
if (element instanceof VariableElement) {
// Add logic to ensure field is not null
System.out.println(element.getSimpleName() + " must not be null");
}
}
return true;
}
}
In this example, we have defined a custom annotation @NotNull
and a processor that checks for fields annotated with this annotation.
Dealing with Errors and Pitfalls
Even though annotation processing can save time and reduce errors, several common pitfalls can undermine its effectiveness.
1. Ignoring Annotation Retention Policy
One common mistake is defining an annotation without the proper retention policy. Annotations can have three types of retention policies: SOURCE
, CLASS
, and RUNTIME
.
- SOURCE: Annotations are discarded by the compiler.
- CLASS: Annotations are recorded in the class file but ignored by the JVM at runtime.
- RUNTIME: Annotations are recorded in the class file and will be available to the JVM at runtime.
Using the wrong retention policy can cause your annotation processor not to find the annotations during compilation.
Solution: Always use the appropriate retention policy based on how you plan to use the annotation. In most cases with annotation processing, SOURCE
is the most suitable choice.
@Retention(RetentionPolicy.SOURCE) // This ensures the annotation is available during compilation.
public @interface MyAnnotation {}
2. Not Handling Multiple Annotations
Another common pitfall is not preparing your annotation processor to handle multiple occurrences of the same annotation on a single element.
For instance, consider the following usage:
@NotNull
@NotNull
private String name;
If your processor doesn't handle this appropriately, you might end up with an incomplete validation or, worse, an error.
Solution: Modify your processor to correctly handle multiple instances. Here’s a simple modification to our previous processor setup:
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element element : roundEnv.getElementsAnnotatedWith(NotNull.class)) {
List<NotNull> annotationsOnElement = element.getAnnotationsByType(NotNull.class);
// Logic to handle multiple NotNull annotations
// For illustration, simply printing the count
System.out.println(element.getSimpleName() + " is annotated " + annotationsOnElement.size() + " times with @NotNull");
}
return true;
}
3. Inadequate Error Reporting
When writing annotation processors, clear and helpful error messages are vital for developers using your annotations. Underestimating this aspect can lead to frustration and confusion.
Solution: Use the Messager
class from javax.annotation.processing
to send precise error (or warning) messages.
@Override
public boolean process(Set<? extends TypeElement> annotations, RoundEnvironment roundEnv) {
for (Element element : roundEnv.getElementsAnnotatedWith(NotNull.class)) {
if (element.getKind() != ElementKind.FIELD) {
processingEnv.getMessager().printMessage(Diagnostic.Kind.ERROR,
"Only fields can be annotated with @NotNull");
}
}
return true;
}
4. Not Considering Module Systems
A common oversight when working with modules in Java 9 and above is not registering your annotation processor. If you miss this point, it might seem like your annotations are being ignored.
Solution: You need to define a module-info.java file and register the processor. Here's a basic structure:
module my.module {
requires java.compiler;
provides javax.annotation.processing.Processor with my.package.NotNullProcessor;
}
Resources for Further Learning
For those wanting to deepen their understanding of Java annotation processing, here are two useful resources:
- Java Tutorial: Annotation Processing - A comprehensive guide to understanding how annotation processing works. Explore Here
- Learn Annotation Processing - A detailed article that dives into the intricacies of creating annotation processors. Read More
A Final Look
Annotation processing can significantly reduce boilerplate code and enforce constraints within your Java applications. However, developers must navigate several pitfalls carefully to harness its full potential. By understanding common issues such as retention policy mishaps, multiple annotation handling, inadequate error reporting, and module registration, you can create more robust and effective annotation processors.
By being mindful of these pitfalls, you can turn annotation processing from a source of headaches into a valuable ally in your Java programming toolkit. Happy coding!