Troubleshooting Common JMX Monitoring Issues in Java Apps
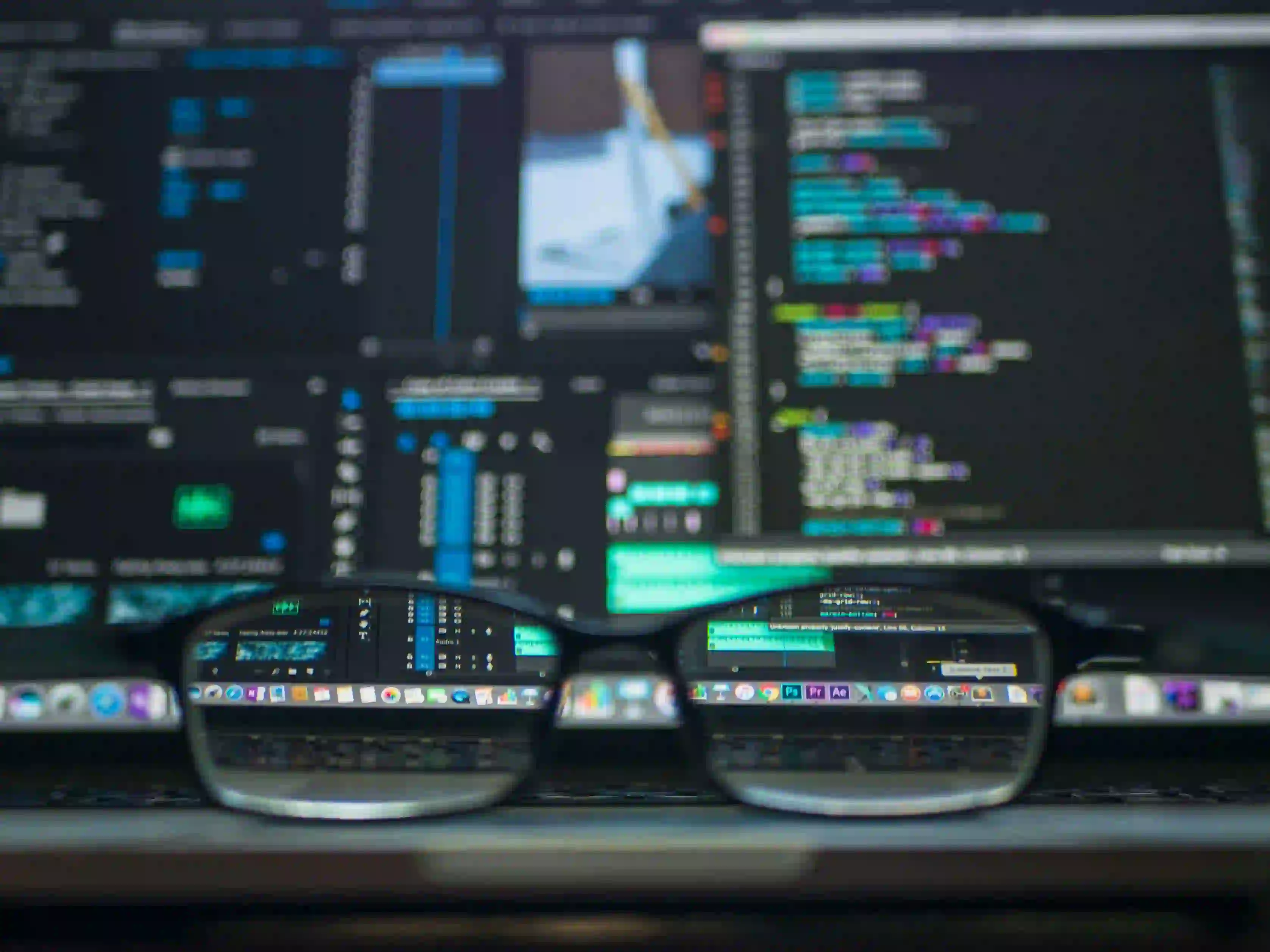
Troubleshooting Common JMX Monitoring Issues in Java Applications
Java Management Extensions (JMX) is a powerful technology that allows developers to monitor and manage Java applications. It provides an interface for interaction and data retrieval from the Java Virtual Machine (JVM), enabling developers to keep track of application performance, resource usage, and other critical metrics. Despite its advantages, many developers encounter issues while implementing JMX monitoring in their applications. In this blog post, we will explore common JMX monitoring issues, troubleshooting techniques, and best practices to enhance your experience with JMX.
Understanding JMX Basics
Before diving into troubleshooting, it is essential to grasp the basics of JMX. JMX provides three main components:
-
MBeans (Managed Beans): These are the resources you want to manage. An MBean can be an instance of a Java class that follows specific interface and naming conventions.
-
MBeanServer: This acts as a registry for MBeans, facilitating their registration and retrieval.
-
Connectors and Adaptors: These allow various applications to access the MBeanServer and interact with the MBeans.
// Example MBean interface
public interface MyMBean {
void setValue(int value);
int getValue();
}
// Implementation of MBean
public class My implements MyMBean {
private int value;
public void setValue(int value) {
this.value = value;
}
public int getValue() {
return value;
}
}
Why JMX Is Important
JMX offers various advantages, such as:
- Real-time monitoring of system performance.
- Dynamic configuration of application parameters.
- Easy integration with third-party monitoring tools.
However, without proper setup, you can run into various monitoring issues that could hinder your application's performance visibility.
Common JMX Monitoring Issues
1. JMX Not Exposing MBeans
If you find that your MBeans are not being listed or exposed, there are a few factors to consider:
- JMX Configuration: Ensure that your application is correctly set up for JMX. This includes starting the JVM with the necessary system properties.
-javaagent:your-agent.jar -Dcom.sun.management.jmxremote
-Dcom.sun.management.jmxremote.port=12345
-Dcom.sun.management.jmxremote.authenticate=false
-Dcom.sun.management.jmxremote.ssl=false
- MBean Registration: Confirm that you have actually registered your MBeans within the MBeanServer. If the MBeans are not registered, clients will not be able to access them.
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.example:type=My");
mbs.registerMBean(new My(), name);
2. Connection Refused Errors
Attempting to connect to JMX and encountering connection refused errors can stem from various causes:
-
Incorrect Port or Host: Verify that the hostname and port are correct, and the application is indeed running. Use tools like
netstat
to check if the specified port is in use. -
Firewall Issues: Ensure that no firewall rules are blocking the connection to the JMX port. You might need to configure your firewall to allow inbound connections.
3. Authentication and Security Issues
When enabling JMX remote access, consider security for production applications. If your application is configured with authentication, ensure that the username and password are correct. Incorrect credentials will lead to access denial.
- Setting up Authentication:
-Dcom.sun.management.jmxremote.password.file=/path/to/password.file
-Dcom.sun.management.jmxremote.access.file=/path/to/access.file
Make sure these files are correctly formatted, with the necessary permissions set.
4. JMX Client Connectivity Problems
JMX clients like JConsole or VisualVM may experience connectivity challenges. This usually occurs if:
- The JMX properties are incorrectly set up. Revisit your JVM options.
- The client has network access issues. Ensure the client is allowed to communicate with the server's IP address and port.
5. Unregistered MBeans After Deployment
Sometimes, MBeans may not appear after deploying a new version. This can happen due to:
- Lifecycle Management: Ensure that application components are correctly initialized and that MBeans are registered when the application starts. Implement robust lifecycle management to register and unregister MBeans properly.
@Override
public void start() throws Exception {
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.example:type=My");
mbs.registerMBean(new My(), name);
}
@Override
public void stop() throws Exception {
ObjectName name = new ObjectName("com.example:type=My");
mbs.unregisterMBean(name);
}
Strategies for Effective JMX Monitoring
To avoid these common issues, consider implementing the following strategies:
-
Robust Error Handling: Always include error handling when dealing with MBean registration. This provides insights into what might be going wrong.
-
Logging: Enhance your application logging to capture JMX-related events. This will facilitate easier troubleshooting by providing insights into MBean registration and connection attempts.
-
Advanced Monitoring Tools: Leverage advanced tools like Prometheus and Grafana for visualizing JMX metrics or JavaMelody for detailed monitoring.
-
Testing in Development: Always ensure you test your JMX configurations during the development phase. Use tools like
JConsole
to easily view your MBeans and their values. -
Documentation and Configuration Management: Maintain thorough documentation of your JMX setup. Track changes in configuration to avoid discrepancies during deployment.
Bringing It All Together
JMX monitoring is a powerful feature of Java applications, providing invaluable access to metrics and management interfaces. However, it can also come with various obstacles that need careful handling. By understanding the common issues and their resolutions discussed here, you can enhance your JMX experience and contribute to the overall performance optimization of your Java applications.
For additional reading on JMX features and capabilities, consider visiting the official Oracle documentation Java Management Extensions (JMX) Technology and JConsole official guide.
By following best practices and employing effective troubleshooting techniques, JMX can become a seamless part of your Java development and management toolkit. Happy coding!