Mastering Transaction Management in Java Applications
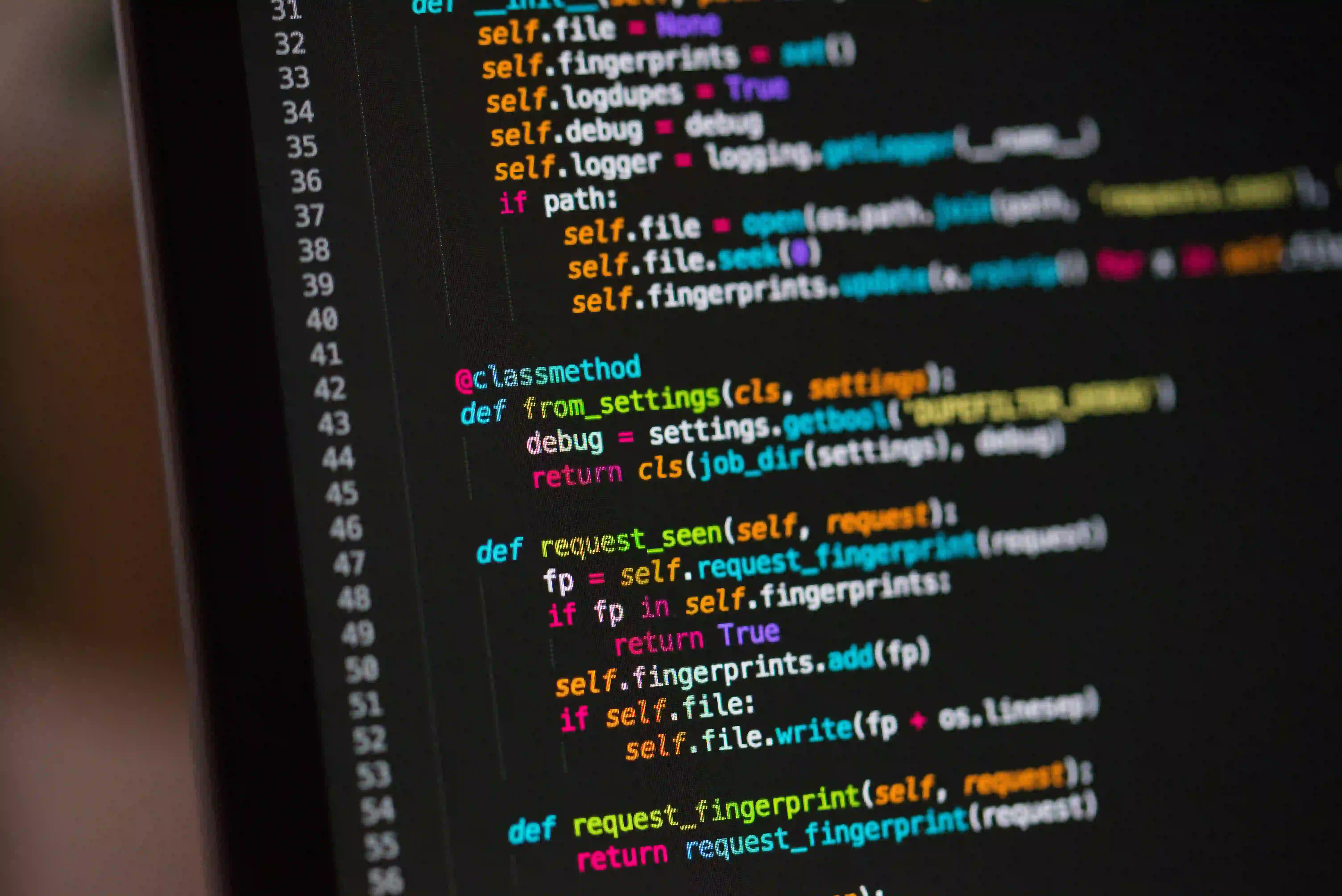
Mastering Transaction Management in Java Applications
In modern software development, managing transactions reliably is essential. Whether you’re working with databases, writing RESTful services, or developing microservices, understanding transaction management can be a critical factor in building robust applications. In this blog post, we will explore various transaction management techniques in Java applications, focusing on best practices, frameworks, and code examples that illustrate these ideas in practice.
What is Transaction Management?
Transaction management refers to the process of handling changes made by a transaction to ensure data integrity, consistency, and durability. In computing, a transaction typically has four properties, commonly known as ACID:
- Atomicity: Ensures that all operations in a transaction are completed successfully. If one operation fails, the entire transaction fails.
- Consistency: Guarantees that a transaction will bring the database from one valid state to another.
- Isolation: Ensures that transactions do not affect each other’s execution.
- Durability: Once a transaction has been committed, it will remain so even in the event of a system failure.
Understanding these properties is critical for building applications that can handle complex datasets and transactions.
Types of Transaction Management
In Java applications, there are generally two approaches to transaction management: programmatic and declarative.
1. Programmatic Transaction Management
In programmatic transaction management, developers have to manage transaction boundaries using code. This approach gives you fine-grained control over your transactions.
Here’s a simple example using JDBC:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
public class TransactionExample {
public void executeTransaction() {
Connection connection = null;
try {
// Connect to the database
connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydb", "user", "password");
// Disable auto-commit mode
connection.setAutoCommit(false);
// Execute SQL statements
PreparedStatement stmt1 = connection.prepareStatement("UPDATE users SET balance = balance - ? WHERE id = ?");
stmt1.setDouble(1, 100.0);
stmt1.setInt(2, 1);
stmt1.executeUpdate();
PreparedStatement stmt2 = connection.prepareStatement("UPDATE users SET balance = balance + ? WHERE id = ?");
stmt2.setDouble(1, 100.0);
stmt2.setInt(2, 2);
stmt2.executeUpdate();
// Commit the transaction
connection.commit();
System.out.println("Transaction committed successfully!");
} catch (Exception e) {
if (connection != null) {
try {
// Rollback the transaction in case of exception
connection.rollback();
System.out.println("Transaction rolled back!");
} catch (Throwable t) {
t.printStackTrace();
}
}
e.printStackTrace();
} finally {
try {
if (connection != null) connection.close();
} catch (Throwable t) {
t.printStackTrace();
}
}
}
}
Why Use Programmatic Transaction Management?
- Fine-grained control: You can control transaction behavior on an operation-by-operation basis.
- Flexibility: Transaction logic can be placed directly alongside business logic.
However, this approach can lead to complex and harder-to-maintain code.
2. Declarative Transaction Management
Declarative transaction management allows you to define transaction boundaries using configuration and annotations instead of code. Spring Framework provides robust support for declarative transaction management, offering a cleaner and more maintainable approach.
Here’s an example using Spring:
import org.springframework.transaction.annotation.Transactional;
public class UserService {
@Transactional
public void transferFunds(int fromUserId, int toUserId, double amount) {
// Logic for transferring funds
updateUserBalance(fromUserId, -amount);
updateUserBalance(toUserId, amount);
}
private void updateUserBalance(int userId, double amount) {
// Implementation for updating user balance
}
}
Why Use Declarative Transaction Management?
- Clean code: By separating transaction management from business logic, your codebase remains clean and manageable.
- Ease of integration: Spring can automatically manage transactions based on method annotations.
To learn more about Spring's transaction management capabilities, visit the Spring Framework documentation.
Best Practices for Transaction Management
Regardless of the approach you choose, following best practices can help ensure your transaction management is effective:
-
Keep Transactions Short and Lean: Long-running transactions can lead to lock contention and decreased performance. Aim to limit transaction scopes only to necessary operations.
-
Use Appropriate Isolation Levels: Depending on your use case, you may need to adjust transaction isolation levels to balance consistency with performance. Review options like READ_COMMITTED or SERIALIZABLE for your specific needs.
-
Handle Exceptions Gracefully: Make sure to roll back transactions in case of exceptions. Adopting a consistent approach to handling exceptions will make it easier to maintain your application.
-
Transaction Timeouts: Configure timeouts to prevent transactions from running indefinitely. This is especially useful in high-concurrency scenarios.
-
Testing: Ensure that you thoroughly test your transaction handling code. Use integration testing to verify the entire transaction flow, including rollbacks.
Closing Remarks
Mastering transaction management in Java applications is essential for maintaining data integrity, consistency, and application performance. Whether opting for programmatic or declarative management, implementing best practices will help you create more robust applications.
As applications scale and evolve, the complexity of transaction management increases. By understanding the principles and practices laid out in this blog post, you can make informed decisions that enhance your application's reliability and user experience.
For more information on Java and transaction management, you can check out Oracle's official Java documentation.
By incorporating sound transaction management techniques, you'll be better equipped to tackle challenges and implement solutions that can stand the test of time. Happy coding!