How to Identify and Fix Java Memory Leaks Effectively
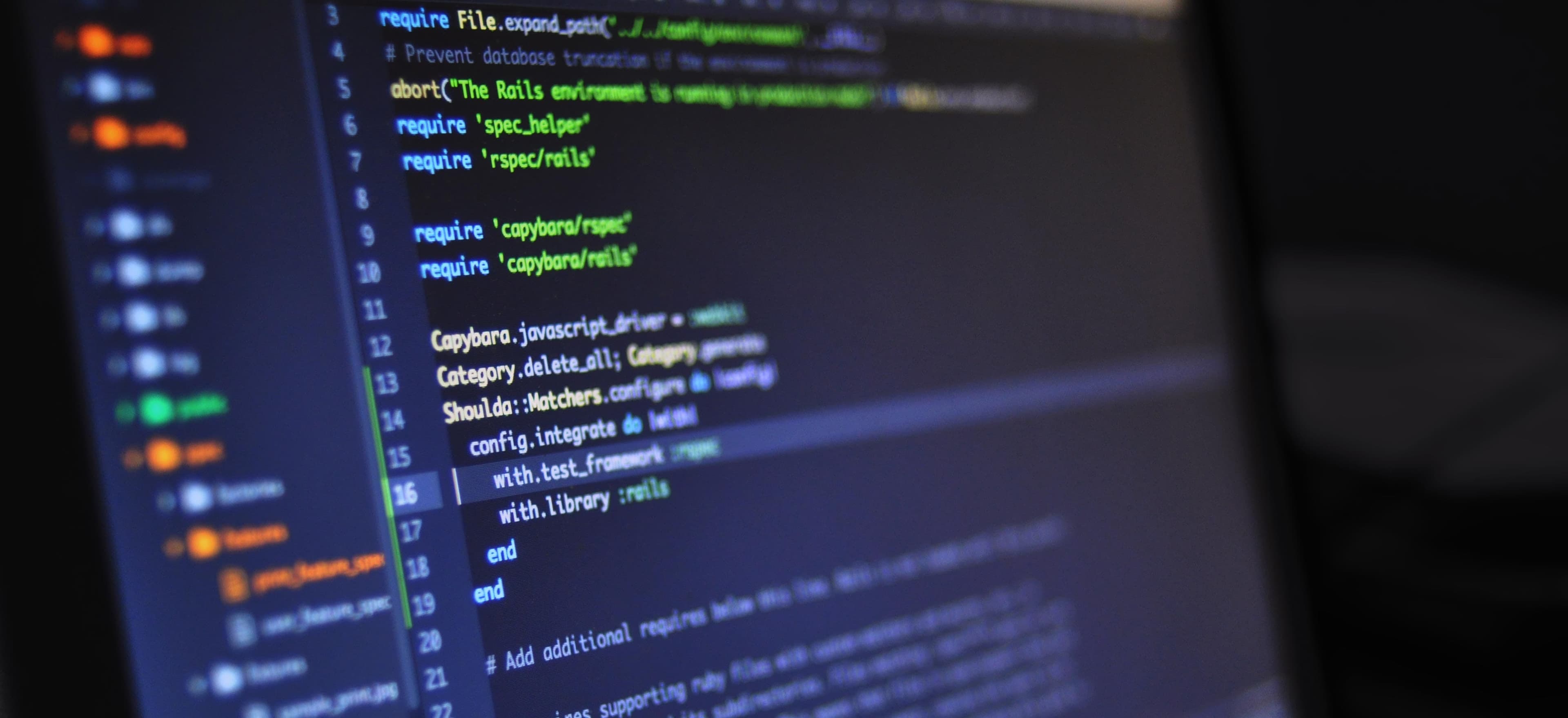
- Published on
How to Identify and Fix Java Memory Leaks Effectively
Memory management in Java is a critical aspect of any robust application. Although Java has a sophisticated garbage collection mechanism, it is still susceptible to memory leaks. In this blog post, we will explore how to identify and fix Java memory leaks effectively, providing you with a roadmap to maintain optimal application performance.
Understanding Memory Leaks in Java
A memory leak occurs when objects that are no longer needed are still referenced by the application, preventing the garbage collector from reclaiming that memory. Over time, these uncollected objects accumulate in memory, leading to increased pressure on the heap, performance degradation, and ever-diminishing available memory.
Why Should You Care?
Memory leaks can cause not just a performance hit but can also lead to application crashes. In critical systems, even a small memory leak can become catastrophic. Thus, detecting and resolving memory leaks is essential for maintaining application efficiency.
Identifying Memory Leaks
1. Familiarize Yourself with Symptoms
The first step in identifying a memory leak is to recognize its symptoms:
- Increased Memory Usage: Constantly increasing heap memory usage can be indicative of a leak.
- Performance Degradation: A slowdown in application response times may point to hidden memory issues.
- OutOfMemoryError: This is the ultimate sign of a memory leak, where the JVM fails to allocate memory.
2. Utilize Java Profilers
Java profilers are invaluable tools for detecting memory leaks. Some popular profilers include:
- VisualVM: Comes bundled with the JDK and can provide heap dumps along with analysis capabilities.
- Eclipse Memory Analyzer (MAT): This tool allows you to analyze heap dumps and identify potential leakage sources.
Here is how you can generate a heap dump for analysis in your application:
jmap -dump:live,format=b,file=heapdump.hprof <pid>
Replace <pid>
with your Java process ID. Analyzing the resulting heap dump with MAT can help locate unreachable objects.
3. Use Garbage Collection Logs
Enabling garbage collection logs can be beneficial for diagnosing memory leaks. This can be done by adding the following JVM options:
-XX:+PrintGCDetails -XX:+PrintGCTimeStamps -Xloggc:gc.log
Review the logs to identify trends in memory usage and frequency of garbage collection.
4. Conduct Code Review
Sometimes, the best way to identify memory leaks is through regular code review. While reviewing, look for:
- Static References: They persist in memory as long as the class is loaded, which is often longer than intended.
- Collections: If you store objects in collections and do not remove them, those objects will remain in memory indefinitely.
Example of Identifying a Memory Leak
Consider a simple example where we might introduce a memory leak:
import java.util.HashMap;
public class MemoryLeakDemo {
private static HashMap<String, String> cache = new HashMap<>();
public static void addToCache(String key, String value) {
cache.put(key, value); // Cache grows indefinitely
}
}
In this example, the cache
grows indefinitely without ever removing entries, leading to a memory leak.
Fixing Memory Leaks
Once you've identified the cause of a memory leak, the next step is fixing it. Here are some common strategies:
1. Release Unused References
Make sure to nullify references of any objects that are no longer needed. For instance:
public void clearCache() {
cache.clear(); // Thoroughly free memory
}
By calling clear()
on the cache, you ensure all references are released, and the garbage collector can reclaim that memory.
2. Use Weak References
In situations where you want to cache objects but release them if memory is tight, consider using WeakHashMap
:
import java.util.WeakHashMap;
public class MemoryEfficientDemo {
private static WeakHashMap<String, String> cache = new WeakHashMap<>();
public static void addToCache(String key, String value) {
cache.put(key, value); // Automatically removes elements when they are no longer referenced
}
}
With WeakHashMap
, entries are removed once there are no strong references to the key, which allows for more efficient memory management.
3. Optimize Collection Usage
If you need to store a large number of objects, consider whether a different collection type might suit your use case better:
- Use
ArrayList
for efficient object retrieval if the list size is fixed. - Use
LinkedList
for frequent additions and removals.
4. Limit Scope of Variables
Limit the scope of your variables to ensure they are eligible for garbage collection as soon as they are out of scope:
public void processFile() {
String data;
// Logic to retrieve data
data = null; // Now eligible for garbage collection
}
Closing the Chapter
Memory leaks can significantly affect application performance and reliability. By understanding their symptoms, utilizing the right tools, and following best coding practices, you can effectively identify and resolve memory leaks in your Java applications.
Remember, proactive monitoring and regular code reviews are your best line of defense against memory leaks. For more in-depth knowledge on Java memory management, consider visiting the Oracle Java Documentation and participating in developer forums like Stack Overflow to stay updated on best practices.
By following this guide, you'll be equipped to keep your Java applications running smoothly and efficiently. Happy coding!