Mastering JSF: Common Challenges and How to Overcome Them
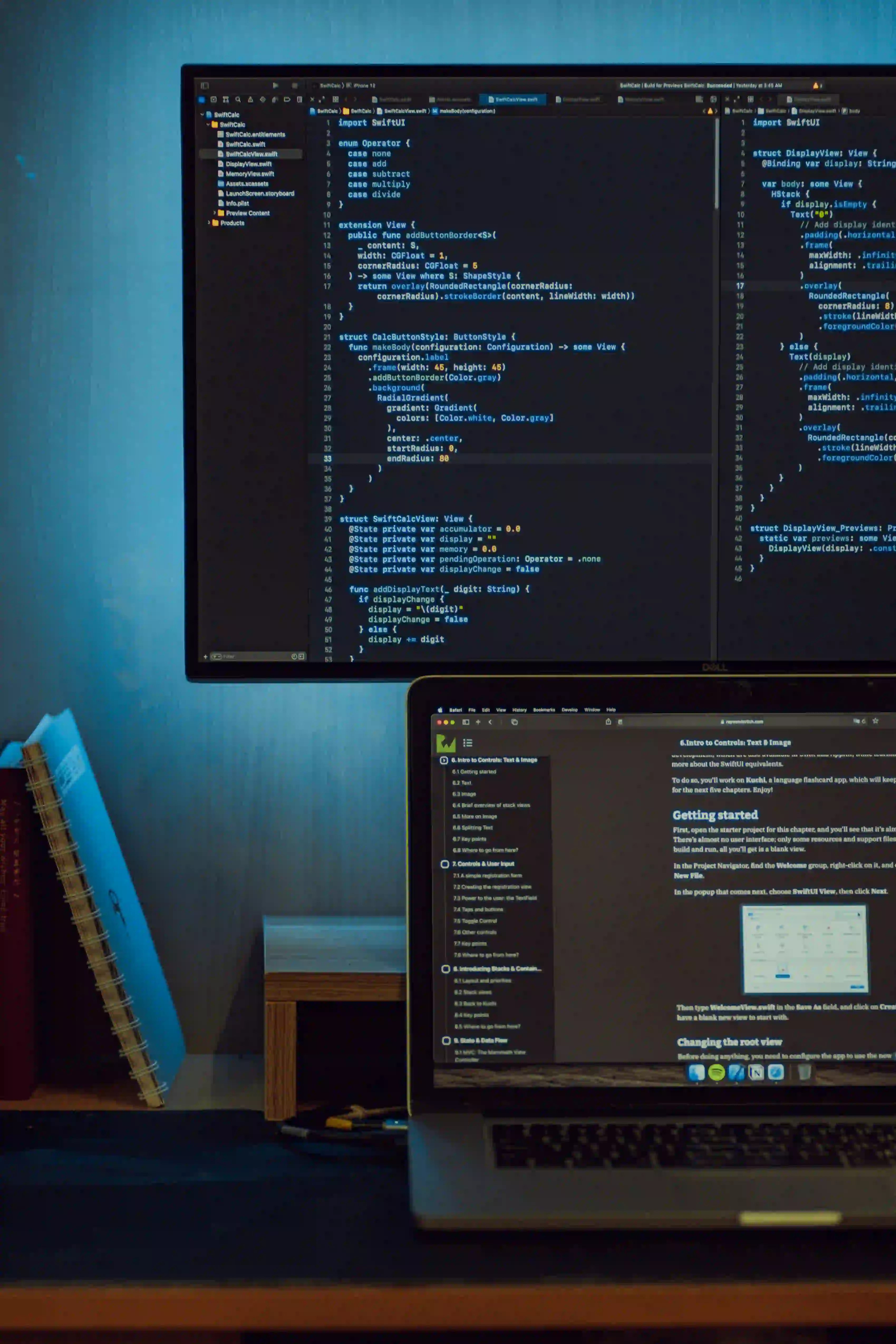
Mastering JSF: Common Challenges and How to Overcome Them
JavaServer Faces (JSF) has been a cornerstone for building web applications in Java. As a component-based framework, it simplifies the development of user interfaces. However, like any technology, it comes with its own set of challenges. In this post, we will explore common JSF challenges and how to overcome them, ensuring that you can build efficient and effective web applications using this powerful framework.
Understanding JSF Framework
Before we dive into the challenges, let's clarify what JSF is. JSF is a Java specification for building component-based user interfaces for web applications. It is part of the Java EE (Enterprise Edition) and allows developers to create reusable UI components that can be connected to Java back-end models.
For a deeper understanding of how JSF works, you might want to check out the official JavaServer Faces documentation.
1. Navigation Complexity
Challenge
One of the most common challenges faced by developers is navigating between views. JSF offers different navigation options such as implicit, explicit, and faces-config navigation rules. Managing complex navigation flows can lead to confusion, especially when the application grows.
Solution
To simplify navigation, you can opt for implicit navigation wherever possible. This allows you to use the outcome of a managed bean action to direct users based on their actions.
@ManagedBean
public class NavigationBean {
public String goToHome() {
return "home"; // Implicit navigation to home.xhtml
}
}
Keeping the navigation rules straightforward improves maintainability. Explicit navigation can also be defined in the faces-config.xml
if you want more control.
<navigation-rule>
<from-view-id>/login.xhtml</from-view-id>
<navigation-case>
<from-outcome>success</from-outcome>
<to-view-id>/home.xhtml</to-view-id>
</navigation-case>
</navigation-rule>
Why This Approach Works
Using implicit navigation reduces the number of configuration points and enhances readability. Developers can quickly understand the navigation logic, thus minimizing the learning curve in larger teams.
2. View State Management
Challenge
JSF maintains two modes of view state: server-side and client-side. Managing the view state can be tricky, particularly when developers are not familiar with the implications of each state management technique.
Solution
Choose the view state management that best fits your application needs. For small applications, server-side state management using @ViewScoped
works seamlessly. However, for larger applications with fewer data changes, consider the @SessionScoped
approach.
@ManagedBean
@SessionScoped
public class UserBean {
private String username;
// Getters and setters
}
Utilize the @ViewScoped
annotation for views that require fresh data while retaining data across user interactions.
Why This Choice Matters
Choosing the right scope is crucial for performance and resource management. Large objects in session scope can consume memory, leading to performance degradation. In contrast, view scope can exacerbate the problem of frequent view instantiations if too much state is stored.
3. Component Lifecycle
Challenge
JSF features a complex lifecycle, which includes phases like restore view, apply request values, process validations, update model values, invoke application events, and render response. Understanding this lifecycle is vital for debugging and optimizing your application.
Solution
To tackle lifecycle issues, it is essential to understand and log each phase when necessary. Furthermore, create a clear separation of concerns between UI and business logic.
For instance, consider a typical lifecycle logging example:
@FacesServlet
public class LifecycleLoggingServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
System.out.println("RESTORING VIEW");
// Implement logic
}
}
Why Lifecycle Understanding Enhances Development
Knowing what's happening during the lifecycle phases allows developers to avoid common pitfalls, such as state mismatches and unintentional data loss. By logging, you can pinpoint exactly where errors occur, making debugging more straightforward.
4. Integration with Other Frameworks
Challenge
Integrating JSF with other frameworks, such as JPA, Spring, or even Java EE components, can lead to confusion and additional complexity. Data injection and transaction management are areas where things often go awry.
Solution
Use dependency injection through CDI (Contexts and Dependency Injection) to manage beans effectively. For instance, instead of managing JPA entities directly inside beans, use a service layer that handles business logic.
@Stateless
public class UserService {
@PersistenceContext
EntityManager em;
public void saveUser(User user) {
em.persist(user);
}
}
Why a Service Layer Matters
Separating concerns by using a service layer keeps your JSF managed beans lean. This allows them to remain focused on presentation while business logic and data access are handled elsewhere.
5. Error Handling
Challenge
Effective error handling in JSF can be daunting, especially due to its multi-layered architecture. Improper handling can lead to poor user experiences and loss of data integrity.
Solution
Implement a global exception handler using @FacesConverter
or create a custom exception class. You can override the processValidate
method for custom error handling.
@FacesConverter("customConverter")
public class CustomConverter implements Converter {
@Override
public Object getAsObject(FacesContext context, UIComponent component, String value) {
// Convert String to Object
try {
// conversion logic
} catch (ConversionException e) {
context.addMessage(component.getClientId(), new FacesMessage("Conversion error"));
return null;
}
}
}
Why Error Handling is Crucial
Applying a centralized approach to error handling prevents users from being faced with raw stack traces that can leak sensitive information. Instead, provide clear user feedback, thus enhancing the overall user experience.
The Bottom Line
JSF is a robust framework with its own set of challenges, but understanding these challenges is the first step toward mastering it. By adopting best practices such as proper navigation handling, efficient view state management, lifecycle understanding, effective integration strategies, and robust error handling, you'll be on your way to becoming a proficient JSF developer.
If you want to learn more about JSF best practices, consider exploring resources like Java EE 8 Application Development that offer in-depth insights and practical examples.
By continually refining your skills and understanding of JSF, you can overcome potential pitfalls and build high-quality web applications that meet users' needs. Remember, persistence and practice are key to mastering any technological domain!