Troubleshooting Common JAX-RS API Deployment Issues
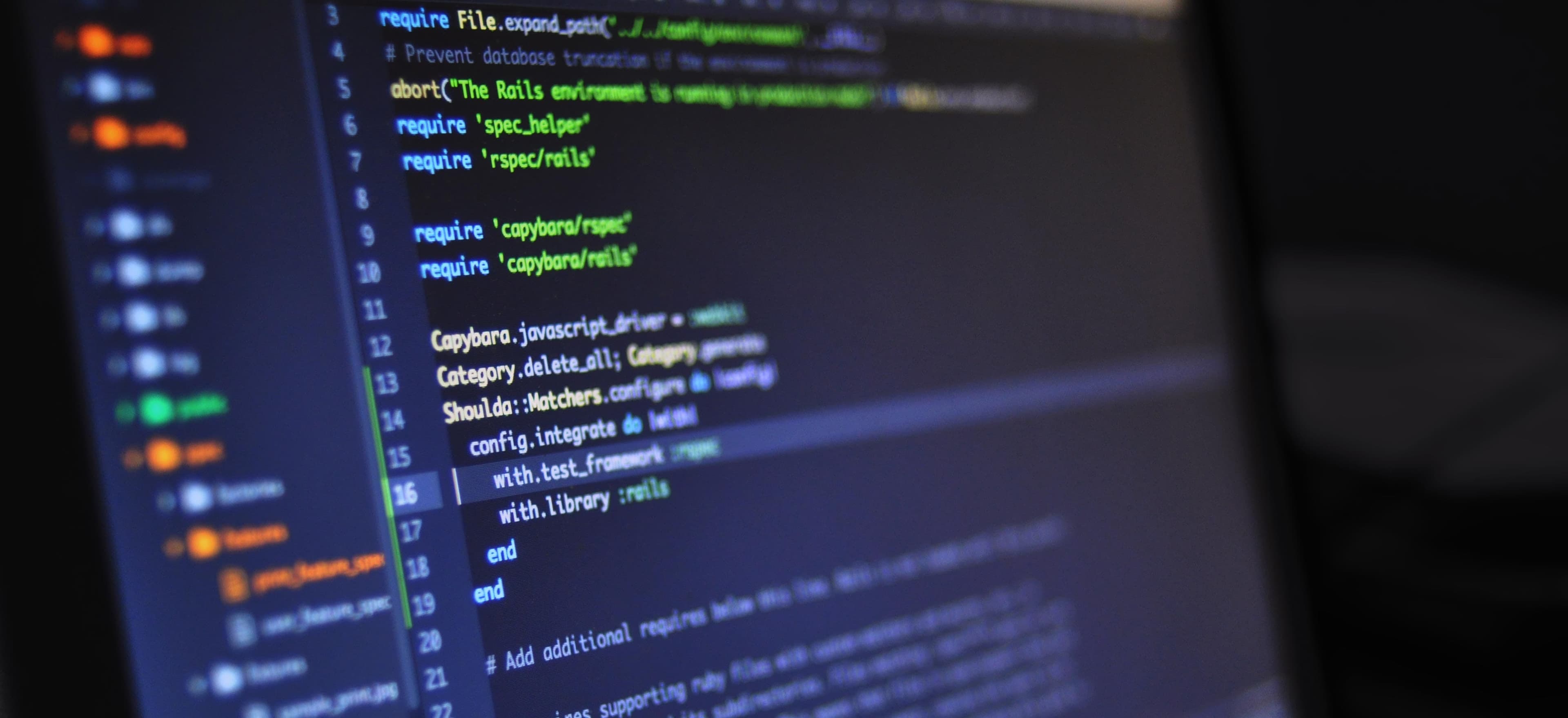
- Published on
Troubleshooting Common JAX-RS API Deployment Issues
Java API for RESTful Web Services (JAX-RS) is a powerful specification for building RESTful web services in Java. JAX-RS simplifies the creation of services that adhere to the principles of the REST architectural style. However, like any technology, deploying a JAX-RS API can sometimes lead to issues. This blog post will discuss common deployment problems, their solutions, and code examples to illustrate key points.
What is JAX-RS?
JAX-RS is a Java EE (Enterprise Edition) API designed to simplify the process of developing RESTful web services. It provides a set of annotations, such as @Path
, @GET
, @POST
, and others, that help developers design APIs in adherence to REST principles.
For thorough documentation, you can refer to the official JAX-RS documentation.
Common JAX-RS Deployment Issues
1. HTTP 500 Internal Server Error
Problem
The HTTP 500 error can occur due to various reasons, such as server configuration issues, exceptions in the application code, or dependencies not being resolved.
Solution
- Check server logs: Always begin by examining your application server logs. Most modern Java EE servers provide detailed logs that can help you identify the underlying problem.
- Debugging exceptions: If your application code produces a NullPointerException or any other runtime exception, ensure to catch and log exceptions effectively. This practice aids in identifying faults.
import javax.ws.rs.GET;
import javax.ws.rs.Path;
import javax.ws.rs.core.Response;
@Path("/example")
public class ExampleResource {
@GET
public Response exampleEndpoint() {
try {
// Simulate logic that may throw an exception
String result = null;
return Response.ok(result).build(); // This line will throw NullPointerException
} catch (Exception e) {
e.printStackTrace(); // Always log exceptions for easier debugging
return Response.serverError().entity("An internal error occurred").build();
}
}
}
Key Takeaway:
Log all exceptions for better error tracking, and always include meaningful error messages in your API responses.
2. Incorrect Deployment Descriptor (web.xml)
Problem
In JAX-RS applications that rely on a web.xml file, misconfiguration can lead to the application not being deployed properly.
Solution
Ensure that your web.xml
is correctly defined with JAX-RS servlet mappings:
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<servlet>
<servlet-name>JAX-RS Application</servlet-name>
<servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class>
<init-param>
<param-name>jersey.config.server.provider.packages</param-name>
<param-value>com.example.rest</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>JAX-RS Application</servlet-name>
<url-pattern>/api/*</url-pattern>
</servlet-mapping>
</web-app>
Key Takeaway:
Verify that the package name in init-param
matches your resource classes. A mismatch here can cause 404 errors or lead to JAX-RS not finding your resources.
3. Missing JAX-RS Dependencies
Problem
Missing or incorrect dependencies can lead to runtime exceptions.
Solution
Make sure that the required JAX-RS libraries are included in your project. If you're using Maven, a common dependency setup might look like this:
<dependency>
<groupId>org.glassfish.jersey.containers</groupId>
<artifactId>jersey-container-servlet-core</artifactId>
<version>2.35</version>
</dependency>
<dependency>
<groupId>org.glassfish.jersey.media</groupId>
<artifactId>jersey-media-json-jackson</artifactId>
<version>2.35</version>
</dependency>
Dependency Resolution
To ensure that Maven correctly fetches these dependencies, you can run:
mvn clean install
Key Takeaway:
Always double-check your dependencies after adding or updating your project, as missing libraries can silently lead to runtime errors.
4. Misconfigured CORS Policy
Problem
When making AJAX calls to your JAX-RS API, the browser may block requests if Cross-Origin Resource Sharing (CORS) is not appropriately configured.
Solution
You can configure CORS in your JAX-RS application using filters or by adding CORS headers manually. Here's an example filter:
import javax.ws.rs.container.ContainerResponseFilter;
import javax.ws.rs.container.ContainerRequestContext;
import javax.ws.rs.container.ContainerResponseContext;
import javax.ws.rs.ext.Provider;
import java.io.IOException;
@Provider
public class CORSFilter implements ContainerResponseFilter {
@Override
public void filter(ContainerRequestContext requestContext, ContainerResponseContext responseContext) throws IOException {
responseContext.getHeaders().add("Access-Control-Allow-Origin", "*");
responseContext.getHeaders().add("Access-Control-Allow-Headers", "origin, content-type, accept, authorization");
responseContext.getHeaders().add("Access-Control-Allow-Credentials", "true");
responseContext.getHeaders().add("Access-Control-Allow-Methods", "GET, POST, PUT, DELETE, OPTIONS, HEAD");
}
}
Key Takeaway:
Implement a CORS filter to allow clients from different origins to interact with your API seamlessly. Adjust the headers based on your security requirements.
5. Unsupported Media Type
Problem
The "415 Unsupported Media Type" error typically arises when the client's Content-Type
header does not match the server's expectations.
Solution
Ensure that your API endpoint accepts the correct media types. You can specify this in your resource methods.
import javax.ws.rs.Consumes;
import javax.ws.rs.POST;
import javax.ws.rs.Path;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
@Path("/example")
public class ExampleResource {
@POST
@Consumes(MediaType.APPLICATION_JSON) // Expect JSON
public Response createExample(String jsonData) {
// Process the incoming JSON data
return Response.ok("Data processed").build();
}
}
Key Takeaway:
Always use @Consumes
and @Produces
to define what content types your service will accept and return, thus preventing unsupported media type errors.
The Closing Argument
Deploying JAX-RS applications does come with its set of challenges; however, the primary issues encountered can often be traced back to a few common pitfalls. By carefully debugging problematic sections, ensuring correct configurations, and confirming that all necessary dependencies are present, many issues can be resolved.
For further insights and topics about JAX-RS, you may explore resources like Baeldung’s JAX-RS Guide and Java Brains. Happy coding!
Checkout our other articles