Unlocking Java Cryptography: Common Pitfalls to Avoid
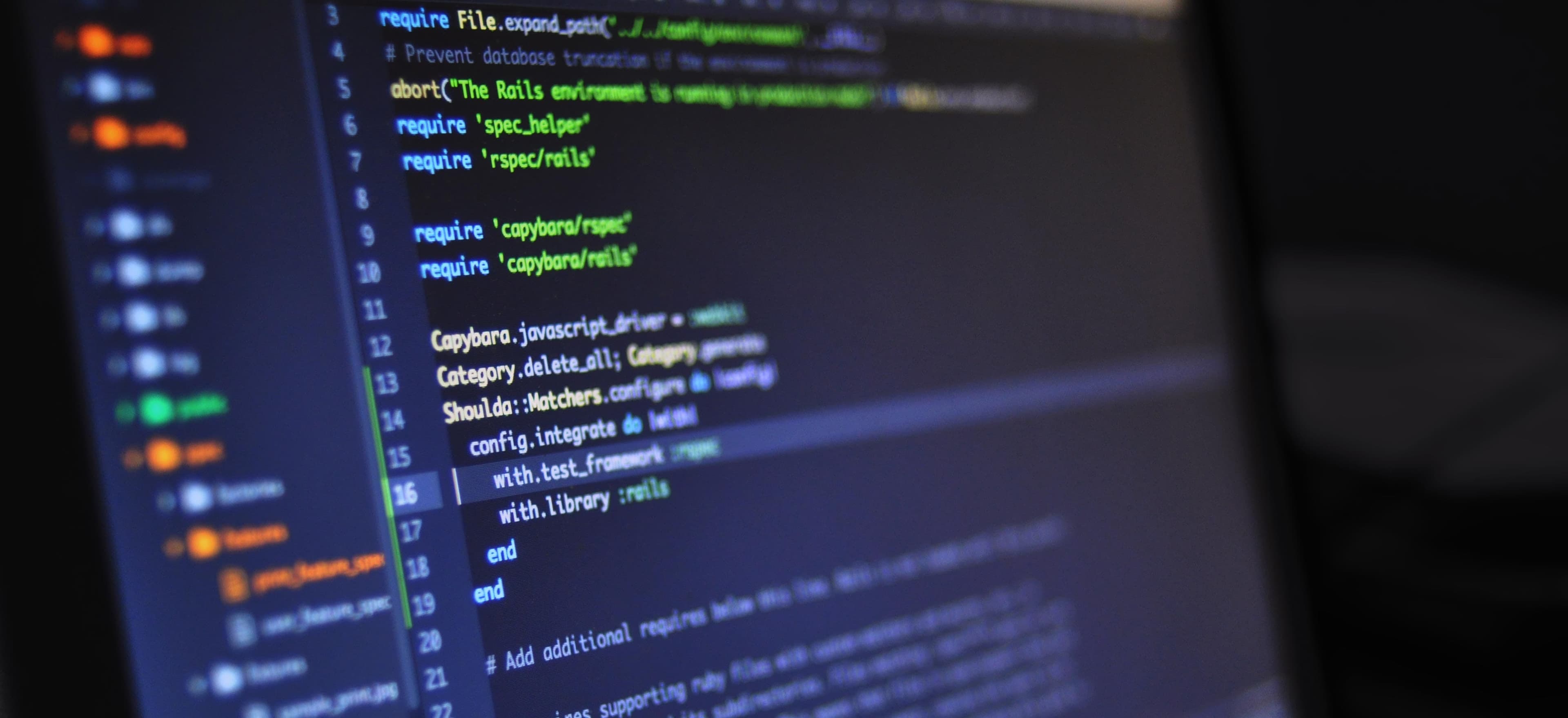
- Published on
Unlocking Java Cryptography: Common Pitfalls to Avoid
Java is a versatile programming language that excels in various domains, including the critical area of cryptography. As developers, ensuring the security of our applications is paramount. However, many stumble when implementing cryptographic solutions. In this blog post, we will delve into common pitfalls in Java cryptography and provide best practices to avoid them.
Understanding Java Cryptography
Before we explore the pitfalls, it's essential to understand the key concepts of Java Cryptography. Java provides a rich set of APIs through the Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE). They provide mechanisms for accessing cryptographic operations such as encryption, decryption, key generation, and message digests.
Common Pitfalls
Here are some of the prevalent mistakes developers make when working with Java cryptography:
- Using Weak Algorithms
- Improper Key Management
- Neglecting Secure Randomness
- Forgetting to Validate Input
- Hardcoding Cryptographic Keys
- Using ECB Mode
- Ignoring Exception Handling
Let’s dive into each of these pitfalls in more detail.
1. Using Weak Algorithms
Many developers default to algorithms that are widely known but have been shown to be vulnerable. For example, using MD5 or SHA-1 for hashing can lead to serious security risks.
Why Avoid Weak Algorithms?
Attackers can easily break weak algorithms, compromising the integrity and confidentiality of your data.
To implement a stronger hash function, you might consider using SHA-256. Here’s how to do it:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class HashUtil {
public static String hashString(String input) {
try {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(input.getBytes());
// Convert byte array into hexadecimal format
StringBuilder hexString = new StringBuilder();
for (byte b : hash) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("Error hashing the input string", e);
}
}
}
2. Improper Key Management
Key management is another critical area of concern. Mismanaging cryptographic keys can lead to unauthorized access.
Why Proper Key Management?
If keys are exposed, the entire cryptographic system is compromised. Developers should leverage secure vaults like AWS Secrets Manager or HashiCorp Vault rather than hardcoding keys.
For example, consider this ill-advised approach:
// Hardcoded key (DO NOT DO THIS)
private static final String SECRET_KEY = "mysecretkey"; // Not secure!
Instead, use environment variables or configuration files that are not included in your version control system.
3. Neglecting Secure Randomness
Java provides a secure random number generator, which is essential for generating keys and initialization vectors (IVs). Using Math.random()
for cryptographic purposes is a major mistake.
Why Use Secure Randomness?
Math.random()
does not provide sufficient randomness for cryptographic operations, exposing your application to predictable attacks.
Here’s how to use the SecureRandom
class in Java:
import java.security.SecureRandom;
public class SecureRandomUtil {
public static byte[] generateRandomBytes(int numBytes) {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[numBytes];
secureRandom.nextBytes(randomBytes);
return randomBytes;
}
}
4. Forgetting to Validate Input
When working with cryptographic functions, it is crucial to validate input rigorously. Failing to do so can lead to vulnerabilities such as buffer overflows or injection attacks.
Why Validate Input?
Unvalidated input can lead to unexpected behavior or security flaws that attackers can exploit.
You should always sanitize and validate user inputs before using them in any cryptographic function.
5. Hardcoding Cryptographic Keys
We touched on this earlier, but it bears repeating. Hardcoding keys in your source code is a significant security risk. This practice exposes your application to potential breaches.
Why Avoid Hardcoding?
Hardcoded keys can be extracted from the binary, leading to unauthorized access. Always store keys securely in dedicated key management solutions.
6. Using ECB Mode
The Electronic Codebook (ECB) mode is often seen as simple to implement. However, it is notoriously insecure because it encrypts identical plaintext blocks to identical ciphertext blocks.
Why Avoid ECB Mode?
ECB mode does not provide semantic security. An attacker can infer patterns and gain insights into the original data.
Instead of ECB mode, consider using Cipher Block Chaining (CBC) mode. Here’s an example:
import javax.crypto.Cipher;
import javax.crypto.spec.IvParameterSpec;
import javax.crypto.spec.SecretKeySpec;
public class AESCrypto {
private static final String ALGORITHM = "AES/CBC/PKCS5Padding";
private static final byte[] IV = new byte[16]; // Initialization vector
public static byte[] encrypt(byte[] data, byte[] key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key, "AES");
Cipher cipher = Cipher.getInstance(ALGORITHM);
cipher.init(Cipher.ENCRYPT_MODE, secretKey, new IvParameterSpec(IV));
return cipher.doFinal(data);
}
}
7. Ignoring Exception Handling
Cryptographic operations can throw exceptions that may lead to application crashes if not handled correctly. Ignoring or improperly handling exceptions can expose your system to vulnerabilities.
Why Proper Exception Handling?
Effective logging and handling of exceptions can aid in debugging and securing your application.
Here's a snippet that demonstrates proper exception handling:
try {
byte[] encryptedData = AESCrypto.encrypt(plainText.getBytes(), secretKey);
} catch (Exception e) {
// Log the exception safely
System.err.println("Encryption failed: " + e.getMessage());
}
Wrapping Up
In conclusion, Java cryptography is an integral part of secure software development. By avoiding common pitfalls such as using weak algorithms, improper key management, and neglecting secure randomness, you can significantly enhance the security of your applications.
Follow best practices, stay updated on the latest cryptographic standards, and continuously review your implementations. Secure coding is not just a best practice; it is a necessity in today's digital landscape.
For further reading, check out the official Java Cryptography Documentation for an in-depth understanding and up-to-date practices in cryptography.
Happy coding!
Checkout our other articles