Mastering Exception Handling: Common Pitfalls in Java
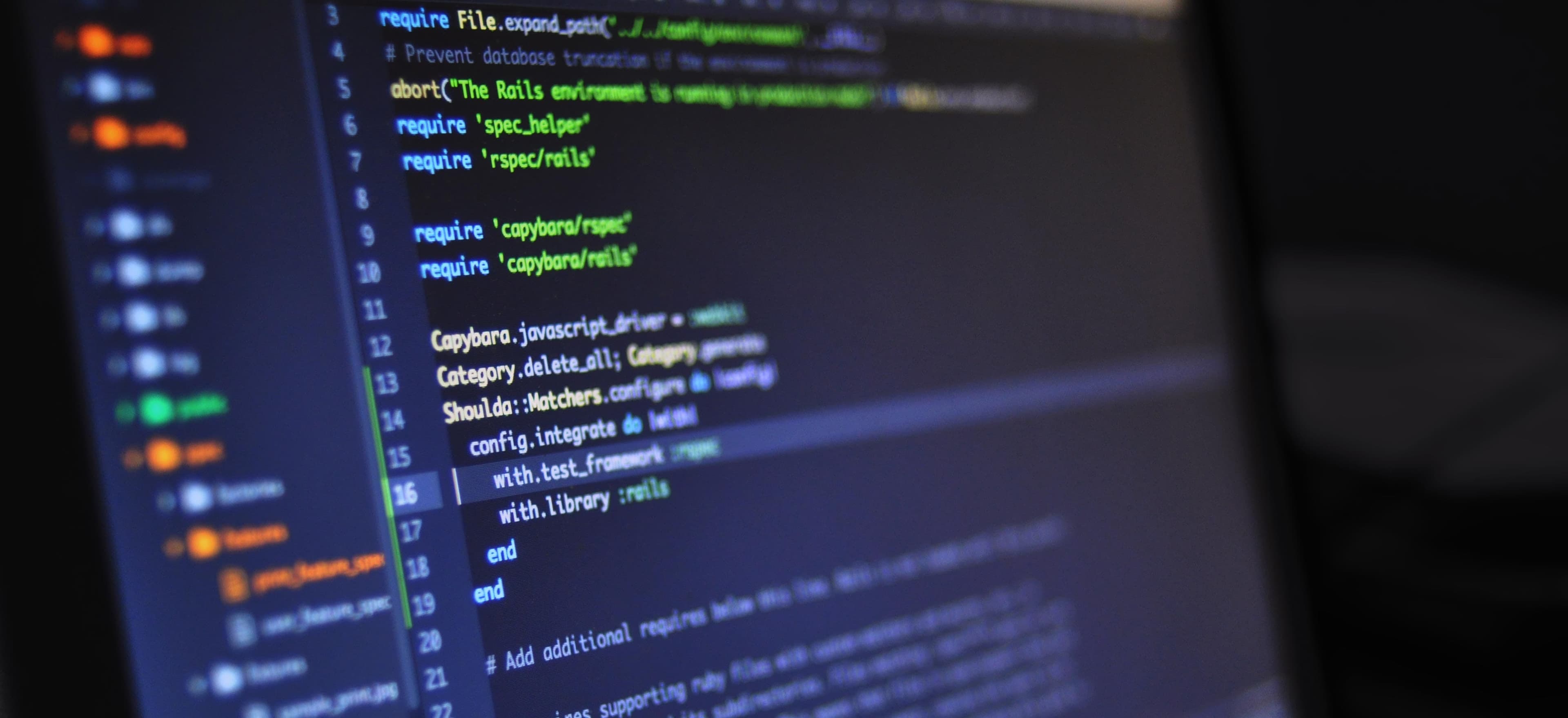
- Published on
Mastering Exception Handling: Common Pitfalls in Java
Exception handling is a crucial aspect of Java programming that ensures the robustness and reliability of applications. This blog post aims to explore common pitfalls in Java exception handling, how you can avoid them, and best practices for optimal error management. By mastering these concepts, you can write cleaner and more efficient code, ultimately leading to better application performance.
Understanding the Basics of Exception Handling in Java
Before diving into pitfalls, let's briefly cover what exception handling is. In Java, an exception is an unchecked error that can disrupt the normal flow of execution. The Java programming language provides a robust framework for handling exceptions, primarily through the use of keywords such as try
, catch
, finally
, and throw
.
Here's a basic example:
public class ExceptionExample {
public static void main(String[] args) {
try {
int result = divide(10, 0);
System.out.println("Result: " + result);
} catch (ArithmeticException e) {
System.out.println("Error: " + e.getMessage());
} finally {
System.out.println("Execution completed.");
}
}
public static int divide(int a, int b) {
return a / b;
}
}
In this snippet:
- The
try
block contains the code that can potentially throw an exception. - The
catch
block handles the specific exception—in this case,ArithmeticException
. - The
finally
block executes regardless of whether an exception occurred, ensuring cleanup.
Common Pitfalls in Exception Handling
1. Swallowing Exceptions
One of the most frequent mistakes is catching exceptions but not handling them properly. Many developers use a generic catch
clause, log the error, and do nothing else. This practice often leads to issues that go unnoticed.
try {
// risky operation
} catch (Exception e) {
// Swallowed exception; nothing is done
}
Why This is a Problem
When you swallow exceptions, you risk masking serious issues in your application. It becomes challenging to debug errors since important information is lost.
Solution
Always handle exceptions in a meaningful way. At a minimum, log the stack trace to understand what went wrong:
try {
// risky operation
} catch (Exception e) {
e.printStackTrace(); // Log the exception for debugging
}
2. Overusing Checked Exceptions
Java has two types of exceptions: checked and unchecked. Checked exceptions must be declared in a method's throws
clause or caught in a try-catch
block.
public void readFile() throws IOException {
// code that may throw IOException
}
Why This is a Problem
Overusing checked exceptions can lead to verbose code, which may become difficult to maintain.
Solution
Use unchecked exceptions (like RuntimeException
) when you want to indicate programming errors that should not be caught. Reserve checked exceptions for cases that a caller can reasonably recover from.
3. Ignoring Finally Blocks
Sometimes developers forget that the finally
block executes whether an exception is thrown or not. This block is useful for resource cleanup, such as closing files or database connections.
FileInputStream input = null;
try {
input = new FileInputStream("data.txt");
// Read from file
} catch (IOException e) {
e.printStackTrace();
} finally {
if (input != null) {
try {
input.close(); // Ensure resources are released
} catch (IOException e) {
e.printStackTrace();
}
}
}
Why This is a Problem
Neglecting the finally
block may prevent important cleanup actions from happening, leading to resource leaks.
Solution
Always use the finally
block for cleanup. For Java 7 and later, you can also utilize the try-with-resources statement for automatic resource management:
try (FileInputStream input = new FileInputStream("data.txt")) {
// Read from file
} catch (IOException e) {
e.printStackTrace();
}
// No need for finally, resource is closed automatically.
4. Custom Exception Classes Misused
Creating custom exception classes is often essential for giving more context to the exceptions your application throws. However, it's not uncommon to misuse them.
public class MyCustomException extends Exception {
public MyCustomException(String message) {
super(message);
}
}
Why This is a Problem
Custom exceptions should only exist when they provide meaningful context. Overusing them can dilute the ability to catch relevant exceptions or make it confusing for others.
Solution
Use custom exceptions sparingly and only when they add real value to error identification.
5. Failure to Document Exception Behavior
Not documenting what exceptions can be thrown from a method can lead to misuse. This oversight makes it difficult for callers to handle exceptions properly.
/**
* Reads a file from the given path.
*
* @param path The path to the file to read.
* @throws IOException if an I/O error occurs.
*/
public void readFile(String path) throws IOException {
// Implementation
}
Why This is a Problem
Lack of documentation can lead to confusion and improper error handling by users of the method.
Solution
Clearly document exceptions using JavaDoc, indicating what exceptions can be thrown and under what conditions.
6. Insufficient or Excessive Logging
Logging is a double-edged sword. Too little logging can hinder troubleshooting, while too much can create noise.
Why This is a Problem
Absence of logs makes debugging complex issues difficult. Conversely, excessive log entries can clutter your log files, making it hard to find useful information.
Solution
Aim for a balanced approach. Log critical information, such as error messages and stack traces, but avoid logging every minor operation.
try {
// risky operation
} catch (Exception e) {
logger.error("An error occurred: " + e.getMessage(), e);
}
Best Practices for Exception Handling
- Be Specific: Catch specific exceptions rather than using a generic
catch (Exception e)
. - Limit the Scope: Keep your
try
block small to reduce the chances of unexpected exceptions. - Plan for Recovery: Ensure your application can recover gracefully from exceptions.
- Utilize Logging Frameworks: Opt for logging frameworks like SLF4J or Log4J for better management and formatting of log data.
For further reading on best practices in Java exception handling, check out Oracle's Java Tutorials on Exceptions for an in-depth understanding.
The Bottom Line
Exception handling in Java is intricate but necessary. Avoiding these common pitfalls can save you time and headaches in the long run. By applying the solutions and best practices discussed above, you can ensure your application is resilient, maintainable, and ready to face unexpected challenges.
Remember, robust exception handling is not just about preventing crashes; it's about making sure your application behaves predictably under all circumstances.
By continually honing your skills and staying informed about best practices, you will become a more proficient Java developer.
For an excellent overview of exception handling, consider visiting Baeldung's Java Exception Handling Guide. Happy coding!
Checkout our other articles