Mastering the Observer Pattern: Common Pitfalls in Java
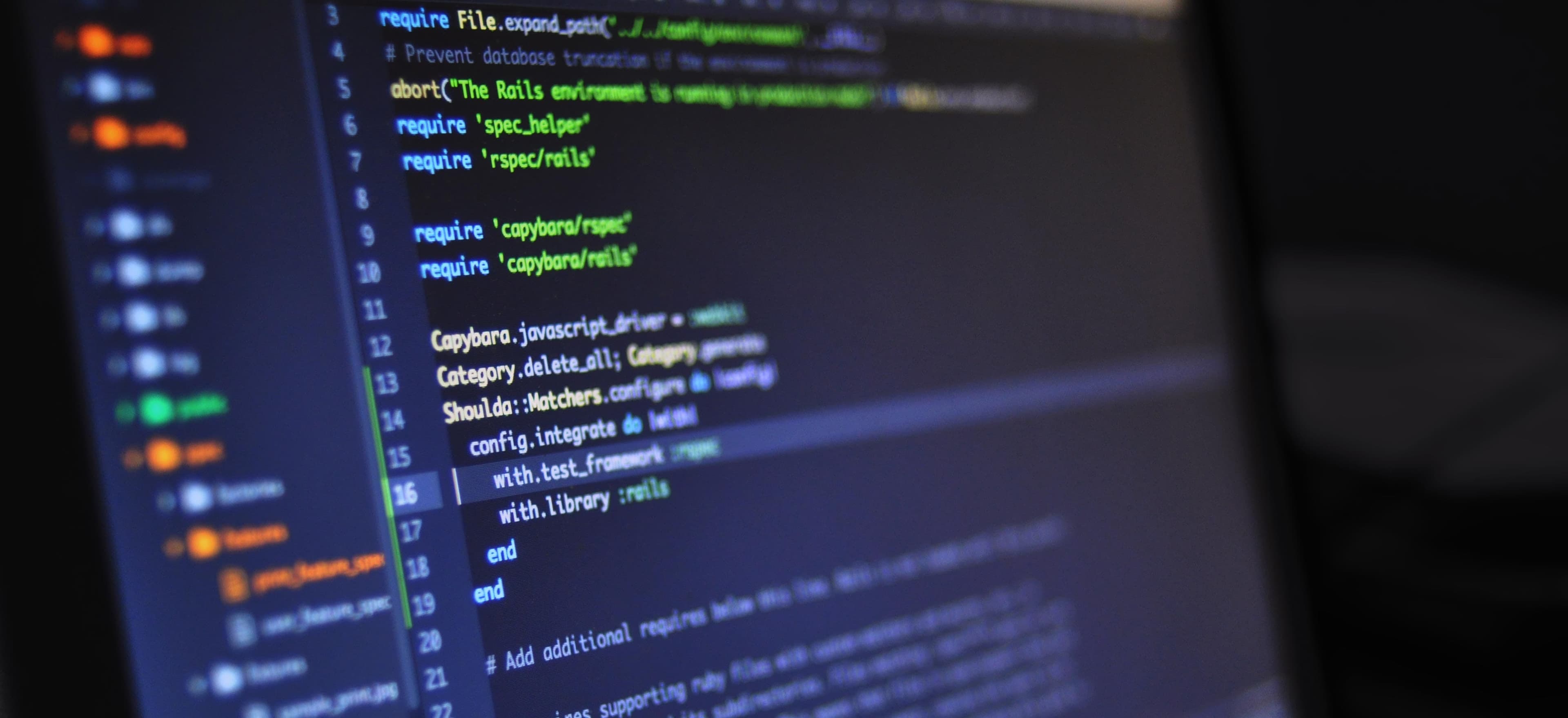
- Published on
Mastering the Observer Pattern: Common Pitfalls in Java
The Observer Pattern is one of the most fundamental design patterns in software engineering. This pattern empowers a one-to-many dependency between objects, encouraging a loose coupling system where changes are communicated efficiently. While it's widely used in frameworks like Java's own Swing and JavaFX, mastering it requires avoiding common pitfalls. In this blog post, we'll explore the principles of the Observer Pattern, discuss common mistakes, and present best practices to ensure its effective implementation in Java.
What is the Observer Pattern?
At its core, the Observer Pattern defines a one-to-many relationship. When one object (the subject) changes its state, all dependent objects (observers) are notified of the change.
Key Components:
- Subject: The object that holds the state and notifies observers about changes.
- Observer: The object that wants to be notified when the subject's state changes.
Example of the Observer Pattern in Java
Let's look at a simple implementation of the Observer Pattern:
import java.util.ArrayList;
import java.util.List;
// Subject
class Subject {
private List<Observer> observers = new ArrayList<>();
private String state;
public void attach(Observer observer) {
observers.add(observer);
}
public void detach(Observer observer) {
observers.remove(observer);
}
public void notifyObservers() {
for (Observer observer : observers) {
observer.update(state);
}
}
public void setState(String state) {
this.state = state;
notifyObservers();
}
}
// Observer
interface Observer {
void update(String state);
}
// ConcreteObserver
class ConcreteObserver implements Observer {
private String name;
public ConcreteObserver(String name) {
this.name = name;
}
@Override
public void update(String state) {
System.out.println(name + " received update: " + state);
}
}
// Main
public class ObserverPatternDemo {
public static void main(String[] args) {
Subject subject = new Subject();
ConcreteObserver observer1 = new ConcreteObserver("Observer1");
ConcreteObserver observer2 = new ConcreteObserver("Observer2");
subject.attach(observer1);
subject.attach(observer2);
subject.setState("State 1");
subject.setState("State 2");
}
}
Explanation of the Code:
- Subject Class: The core class that holds a list of observers and notifies them on state changes.
- Observer Interface: Defines the method
update
for responding to changes. - ConcreteObserver Class: Implements the
Observer
interface and provides actual functionality for how an observer should respond to updates.
The notifyObservers
method in the Subject
class is critical. It ensures that all observers are informed of state changes, thus maintaining cohesiveness.
Common Pitfalls in Implementing the Observer Pattern
While the Observer Pattern can promote high cohesion and low coupling, implementing it incorrectly can lead to various problems:
1. Lack of Deregistration
One common mistake is failing to remove observers from the subject, which leads to memory leaks. If observers are not deregistered, they can retain strong references to the subject even after they are no longer of interest, preventing garbage collection.
Solution: Always provide a method to detach observers.
// Detach method to ensure memory management
public void detach(Observer observer) {
observers.remove(observer);
}
2. Not Handling Concurrency
In multi-threaded environments, if observers are updated while other threads are attaching or detaching observers, it can lead to inconsistent states and eventual application crashes.
Solution: Synchronize access to the observer list.
public synchronized void attach(Observer observer) {
observers.add(observer);
}
public synchronized void detach(Observer observer) {
observers.remove(observer);
}
public synchronized void notifyObservers() {
for (Observer observer : observers) {
observer.update(state);
}
}
3. Unclear Notification Design
Sometimes the updates sent to observers might not be relevant for all. Sending unnecessary information can lead to inefficiencies or confusion.
Solution: Make notifications more targeted. Consider passing an event object with relevant data.
public void notifyObservers(Event event) {
for (Observer observer : observers) {
observer.update(event);
}
}
class Event {
// Fields and methods for event details
}
4. Infinite Loops
When observers update the subject state, it can sometimes lead to infinite notification loops, especially if observers also maintain their own states based on the subject's state.
Solution: Implement a mechanism to prevent recursive updates. Use flags indicating whether the notification is already in progress.
5. Observer Initialization
Observers should be properly initialized before they start receiving updates. If they depend on the state of the subject at the moment of attachment, it could lead to unexpected behaviors.
Solution: Consider invoking a method to initialize the observer with the current state of the subject immediately after attaching.
public void attach(Observer observer) {
observers.add(observer);
observer.update(state); // Initialize the observer with the current state
}
Best Practices for Implementing the Observer Pattern
1. Use Strong Typing
Ensure that the observer interface is strongly typed to avoid runtime errors. This also improves readability and maintainability.
2. Document the Purpose
Document both the subject and observer classes clearly. Understanding the life cycle of the observer pattern can save time during maintenance.
3. Keep It Simple
Avoid over-complicating the observer pattern implementation. Sometimes a simple implementation can satisfy the requirements without adding unnecessary complexity.
4. Follow SOLID Principles
Ensure that your design follows the SOLID principles. Pay special attention to the Interface Segregation Principle - keep interfaces focused.
5. Consider Alternative Patterns
Depending on your requirements, consider alternatives like the Event Bus Pattern which can simplify communication between components in larger applications.
The Bottom Line
Mastering the Observer Pattern in Java requires vigilance against common pitfalls. By implementing the recommended best practices and understanding the fundamental principles, developers can harness the power of the Observer Pattern efficiently.
For additional reading on design patterns, you might find these resources useful:
Embrace these insights, and elevate your Java applications with a robust and maintainable observer implementation!