Common Misconceptions About Java Garbage Collection
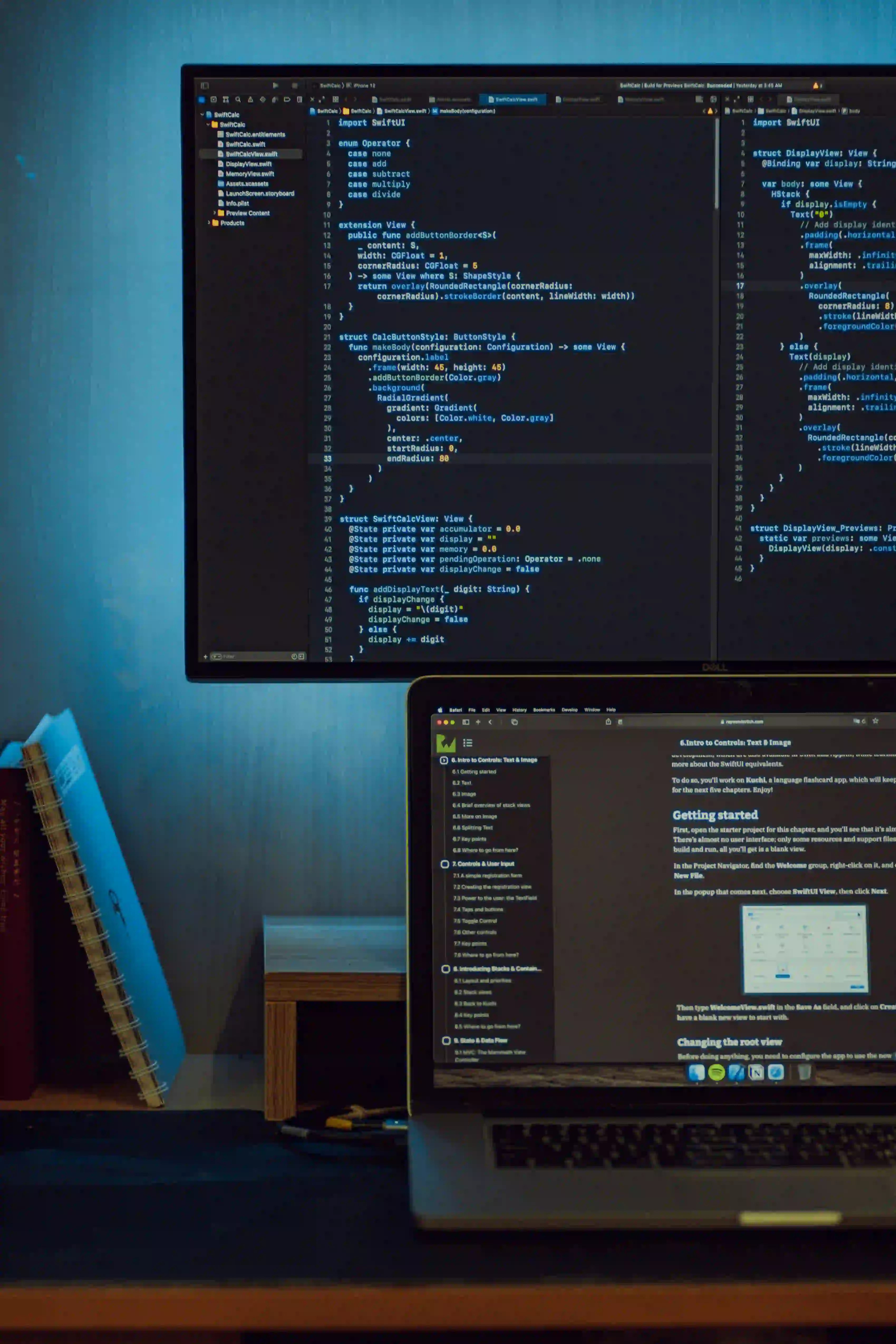
Common Misconceptions About Java Garbage Collection
Java is renowned for its powerful abstraction layers, which allow developers to concentrate on writing code rather than managing memory. One of the key components of this memory management is the Java Garbage Collector (GC). However, despite its reliability, there are numerous misconceptions surrounding Java’s garbage collection mechanism. In this blog post, we will explore these misconceptions, provide clarity, and help you leverage GC effectively in your Java applications.
What is Java Garbage Collection?
Garbage Collection (GC) in Java is a process by which the Java Virtual Machine (JVM) automatically identifies and discards objects that are no longer needed, thereby reclaiming memory. This automatic memory management feature prevents memory leaks and allows developers to focus on application logic.
The Basics of Garbage Collection
Before diving deeper, let's discuss how garbage collection works:
- Memory Allocation: When an object is created, memory is allocated on the heap.
- Reachability: The JVM identifies whether an object is still reachable. If not, it is considered eligible for garbage collection.
- Finalization: Before reclaiming an object's memory, the finalize method is called.
- Memory Reclamation: The memory occupied by unreachable objects is reclaimed.
public class GarbageCollectionExample {
public static void main(String[] args) {
// Object allocation
MyObject obj1 = new MyObject();
MyObject obj2 = new MyObject();
// obj1 goes out of scope here
obj1 = null;
// Force garbage collection
System.gc();
}
}
class MyObject {
@Override
protected void finalize() throws Throwable {
System.out.println("MyObject is being garbage collected");
}
}
The above code demonstrates the basic flow of garbage collection in Java. By setting obj1
to null
, we make the object eligible for garbage collection when System.gc()
is called. However, calling System.gc()
is merely a suggestion to the JVM; it does not guarantee immediate collection.
Common Misconceptions about Garbage Collection
1. Garbage Collection is the Same as Manual Memory Management
One significant misconception is that garbage collection replaces the need for manual memory management entirely. While GC abstracts away the complexity of memory allocation and deallocation, it is not a panacea. Developers still need to understand how memory works to avoid creating unnecessary objects.
Example:
If you constantly create objects without disposing of them properly (perhaps by not nullifying references when done), you may still encounter memory issues.
2. Garbage Collection Happens Instantly
Many believe that garbage collection occurs instantaneously when the application runs out of memory. This is misleading. The garbage collection process is not synchronous; it runs based on the JVM's internal algorithms and heuristics.
Garbage Collection Algorithms
Java employs several garbage collection algorithms, including:
- Serial GC: Good for single-threaded applications.
- Parallel GC: Suited for multi-threaded applications, minimizes pause times.
- G1 GC: Aimed at large heaps with predictable pause times.
Understanding which algorithm you are using can significantly improve performance:
-XX:+UseSerialGC // Use Serial GC
-XX:+UseParallelGC // Use Parallel GC
-XX:+UseG1GC // Use G1 GC
3. The GC Can Reclaim All Memory
Another misconception is that garbage collection can reclaim all unused memory. However, GC only works on unreachable objects. Objects that are still referenced will not be collected, regardless of their use (or lack thereof).
4. Garbage Collection is Free
While garbage collection automates memory management, it requires CPU cycles. Therefore, garbage collection is not "free" in terms of performance. Heavy usage can lead to pauses in application execution, which might be detrimental in real-time applications.
Performance Consideration:
Using a profiling tool can help track and pinpoint GC issues. For example, the Java VisualVM is a powerful tool for monitoring garbage collection and optimizing memory usage.
5. Garbage Collection Completely Eliminates Memory Leaks
Although GC helps mitigate memory leaks, it does not eliminate them. Memory leaks occur when objects remain reachable unintentionally, leading the GC to consider them still in use.
Imagine a static list that keeps references to old objects:
List<MyObject> myList = new ArrayList<>();
public void addObject(MyObject obj) {
myList.add(obj); // Hold onto the reference, causing potential leaks
}
In this example, if you keep adding MyObject
instances without removing them, memory usage will continue to rise, leading to performance degradation.
6. The finalize Method Guarantees Cleanup
Many developers think that overriding the finalize()
method guarantees cleanup of resources. However, this method is inherently unreliable. Objects can be collected without their finalize()
method being invoked, hence it should not be relied upon for resource management.
Alternative Solution:
Using the try-with-resources
construct introduced in Java 7 is preferable:
try (MyResource resource = new MyResource()) {
// Use resource
} // Automatically cleaned up after use
Best Practices for Effective Garbage Collection
Understanding these misconceptions is only part of the journey. Let's explore some best practices for optimizing garbage collection in your Java applications:
1. Minimize Object Creation
Reduce the number of objects created. Reuse existing objects when possible. For example, consider using StringBuilder for string manipulation to avoid creating multiple String objects.
2. Choose the Right Garbage Collector
Choose a garbage collector that aligns with your application needs. For high-performance applications, consider experimenting with G1 GC or ZGC.
3. Monitor and Tune the JVM
Use profiling tools like Java VisualVM or other JVM monitoring tools to observe memory usage and GC activity. Tune JVM parameters like heap size to suit your application's needs.
java -Xms512m -Xmx4g -XX:+UseG1GC -jar YourApplication.jar
4. Avoid Strong References
Use weak references or soft references when applicable. This will allow the garbage collector to reclaim memory more effectively.
In Conclusion, Here is What Matters
Java garbage collection is a powerful feature that greatly simplifies memory management. However, understanding its intricacies can help prevent potential pitfalls – from memory leaks to performance hits.
By acknowledging these common misconceptions of garbage collection, you can write more efficient and effective Java applications. To stay abreast of best practices, continue to explore resources on garbage collection and JVM performance optimization.
For deeper insights, consider checking out this Oracle documentation on Garbage Collection and the Java Garbage Collection Basics.
By enriching your knowledge on garbage collection, you can ensure your Java applications remain performant and reliable. Happy coding!