Overcoming Common CI Challenges in Jenkins for Java Projects
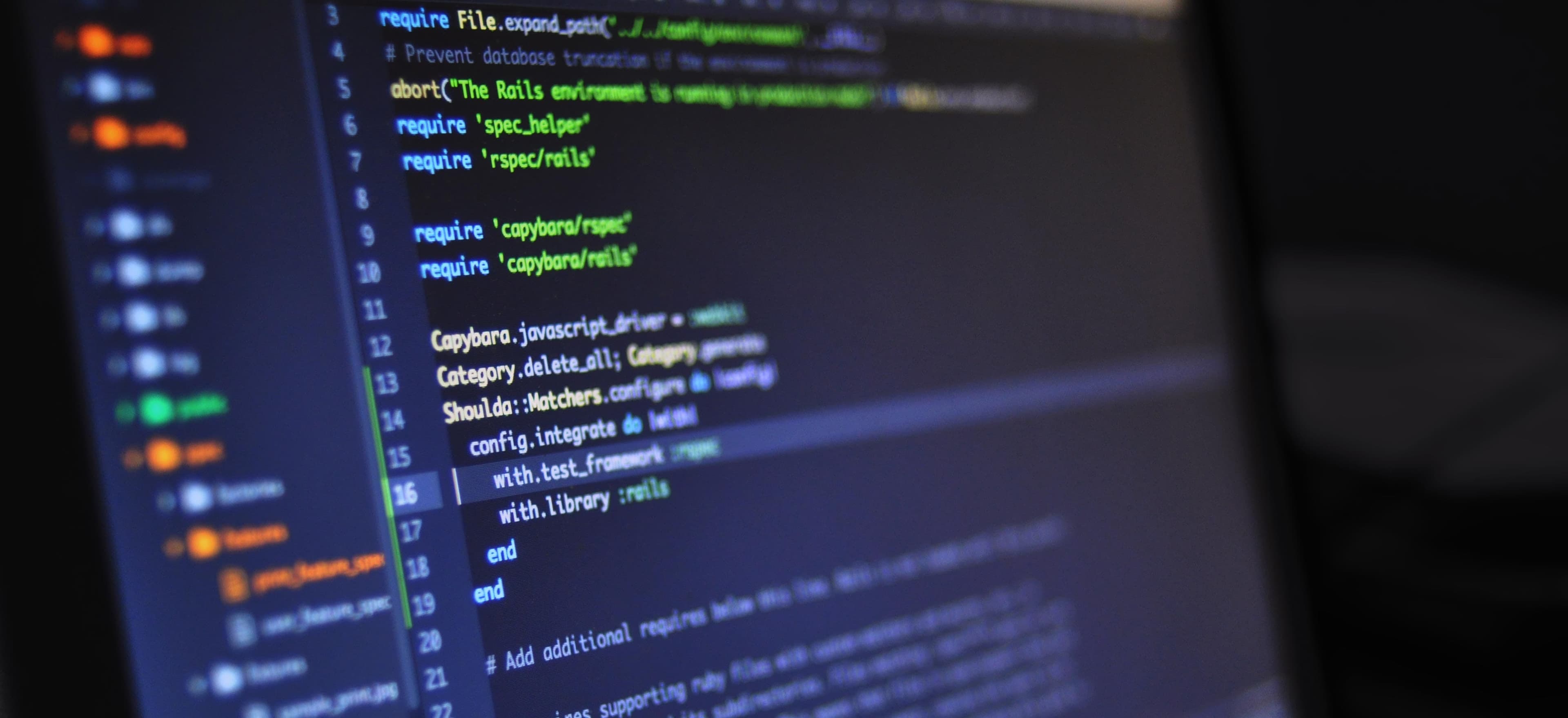
- Published on
Overcoming Common CI Challenges in Jenkins for Java Projects
Continuous Integration (CI) has become an essential part of the software development lifecycle, particularly for Java projects. Jenkins, as one of the most widely used CI/CD tools, offers unmatched flexibility and a plethora of plugins tailored for Java development. However, many teams encounter challenges when integrating Jenkins into their Java projects. This blog post will delve into common CI challenges in Jenkins, providing practical strategies to overcome them, complete with code snippets and valuable insights.
Understanding Continuous Integration
Continuous Integration is a practice where developers frequently integrate their code into a shared repository, triggering automated builds and tests. This process enables teams to detect problems early and ensure reliable software delivery.
Before diving into challenges, let’s ensure that our Jenkins is set up correctly for a Java project.
Setting Up Jenkins for a Java Project
Here’s a basic Jenkins pipeline configuration for a Java project using Maven:
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
// Clean and build the project using Maven
sh 'mvn clean package'
}
}
}
stage('Test') {
steps {
script {
// Run tests using Maven
sh 'mvn test'
}
}
}
}
}
In this pipeline, we define stages for building and testing our Java application using Maven. Each stage contains a specific command executed on the Jenkins server.
Common CI Challenges
Now that we have our pipeline set up, let's explore some of the challenges that may arise and strategies to mitigate them.
1. Build Failures
Problem: Build failures are arguably the most common issue in CI. They can occur due to dependency issues, compilation errors, or configuration errors.
Solution:
-
Always Check Out Newest Version: Ensure your Jenkins job is pulling the latest code from the version control system (VCS).
-
Manage Dependencies: Use a dependency management tool like Maven or Gradle. Ensure that your
pom.xml
(for Maven) specifies exact version numbers.
Example:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.10</version>
</dependency>
Why: Specifying exact versions minimizes the chances of pulling incompatible library versions unexpectedly.
2. Test Failures
Problem: Automated tests fail intermittently, often referred to as flaky tests, leading to mistrust in the CI pipeline.
Solution:
-
Monitor Test Stability: Keep track of test results over time. If specific tests are flaky, investigate their dependencies and execution environment.
-
Isolate Tests: Run tests in isolation to avoid interference from one another.
-
Use Test Containers: Add containers to replicate the production environment during testing.
Here’s an example of how to integrate TestContainers with JUnit in your tests:
import org.junit.jupiter.api.Test;
import org.testcontainers.containers.GenericContainer;
public class MyServiceTest {
@Test
public void testService() {
try (GenericContainer<?> redis = new GenericContainer<>("redis:5.0.3")) {
redis.start();
// Run tests that depend on the service
}
}
}
Why: Using TestContainers ensures your tests are environment-agnostic and reduces flakiness.
3. Environment Configuration Issues
Problem: Different environments (development, testing, production) often lead to configuration headaches.
Solution:
- Use Jenkins Environment Variables: Map environment variables to your Jenkins job to manage configurations dynamically.
Example:
pipeline {
agent any
environment {
JAVA_HOME = '/usr/lib/jvm/java-11-openjdk-amd64'
}
stages {
stage('Build') {
steps {
script {
sh '''
echo "Using JAVA_HOME: $JAVA_HOME"
mvn clean install
'''
}
}
}
}
}
Why: By setting environment variables, you maintain flexibility in your pipeline, minimizing hardcoded values in your script.
4. Resource Management
Problem: Jenkins jobs are resource-intensive and may overwhelm shared resources, leading to slow builds and deployment bottlenecks.
Solution:
-
Leverage Jenkins Nodes and Executors: Distribute your builds across multiple nodes. Each Jenkins node can handle separate builds, preventing resource constraints.
-
Limit Concurrent Jobs: Configure job limits to control how many builds can run simultaneously.
pipeline {
agent {
label 'linux'
}
options {
lock(resource: 'my-resource', inversePrevention: true)
}
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
}
}
Why: Using resource locking ensures that builds do not compete for the same resource, maintaining efficiency in shared environments.
5. Version Control Setup
Problem: The integration between Jenkins and version control systems can present challenges, particularly around webhooks and branches.
Solution:
-
Webhooks: Configure your VCS to send webhooks to Jenkins on specific events (like code pushes).
-
Branch Specifier: Ensure that your Jenkins job is configured to listen to the branches you need.
Example configuration in Jenkins:
Branches to build: */main
Why: This setup ensures that only relevant branches trigger builds, thus maintaining pipeline clarity.
Final Considerations
Mastering CI with Jenkins in Java projects requires a strategic approach to overcome common challenges. From handling build and test failures to managing configuration and resources, each problem presents an opportunity for improvement.
With the aforementioned strategies and best practices, you can make the CI/CD pipeline smooth, reliable, and efficient. To explore further about Jenkins, consider checking the official Jenkins documentation.
Continuous integration enables teams to focus on delivering high-quality software at a faster pace, reinforcing the importance of overcoming these CI challenges. Happy coding!