Common Mistakes When Mocking with Mockito in Java
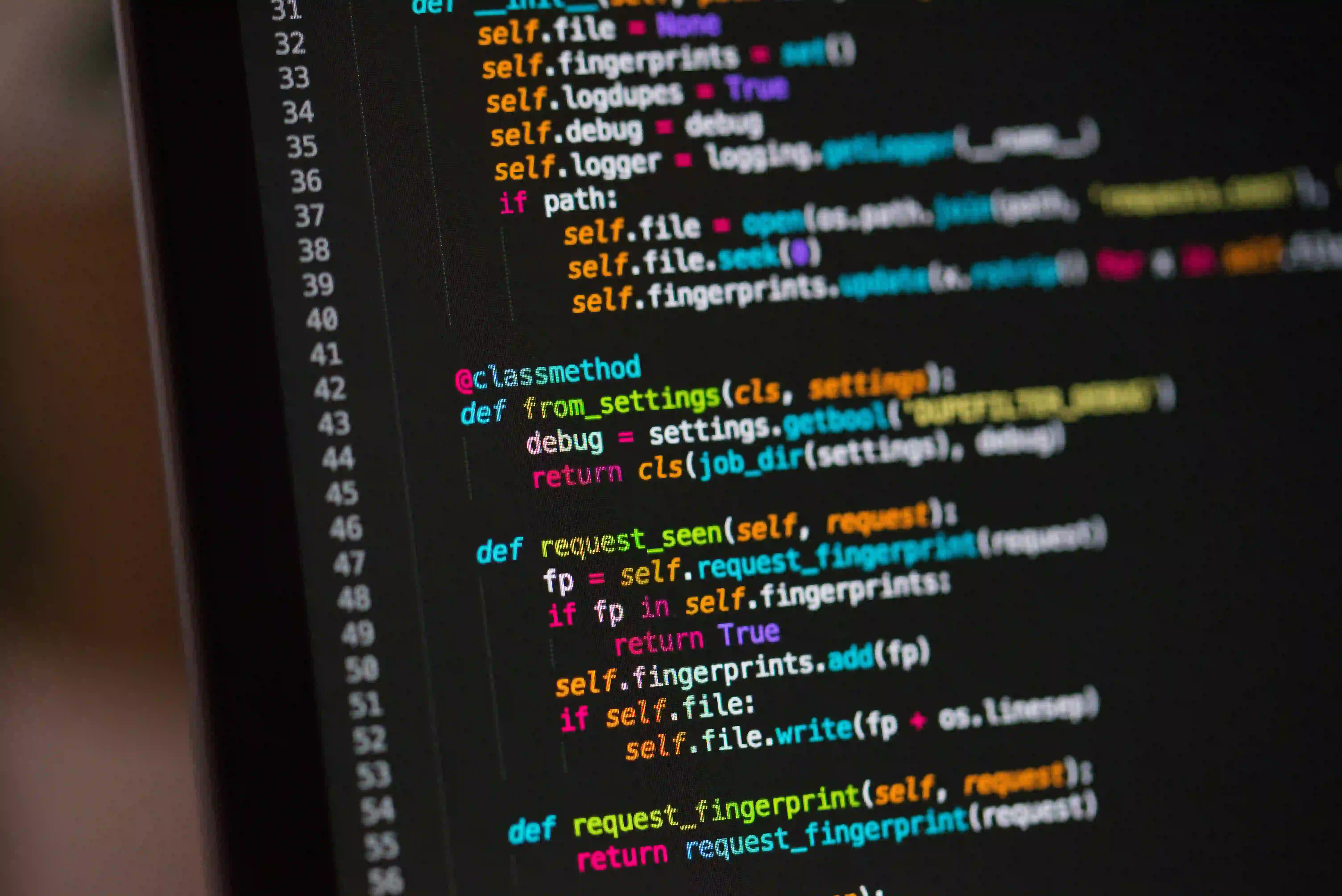
Common Mistakes When Mocking with Mockito in Java
Mockito is a popular mocking framework for Java, making unit testing much simpler and more effective. However, like any tool, it has its nuances. Many developers, especially newcomers, stumble upon common pitfalls while using Mockito, which can lead to confusing tests that are hard to maintain. In this blog post, we will discuss some common mistakes when mocking with Mockito and how to avoid them. We will also provide code snippets with clear commentary to illustrate best practices.
What is Mockito?
Before we dive into the common mistakes, let's briefly discuss what Mockito is. Mockito is a mocking framework that enables developers to create mock objects within their tests. This allows you to isolate the code under test and focus on its behavior rather than the external interactions.
1. Not Using @Mock
Annotations Properly
One of the most frequent mistakes is not using the @Mock
annotation effectively. The @Mock
annotation can enhance code readability and reduce boilerplate code.
Example
import org.junit.Before;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.MockitoAnnotations;
public class MyServiceTest {
@Mock
private MyDependency myDependency;
private MyService myService;
@Before
public void setUp() {
MockitoAnnotations.initMocks(this);
myService = new MyService(myDependency);
}
@Test
public void testDoSomething() {
// Test implementation
}
}
In this example, MockitoAnnotations.initMocks(this)
initializes the mock objects. If you call mock creation methods instead, like Mockito.mock(MyDependency.class)
, it can introduce repetitive boilerplate code that makes it hard to read.
2. Forgetting to Specify Behavior
Another common mistake is not specifying the behavior of the mock objects. When you mock an object, it will return null
or default values if you don't define its behavior. This can lead to misleading test results.
Example
import static org.mockito.Mockito.*;
@Test
public void testDoSomething() {
when(myDependency.getData()).thenReturn("Mocked Data");
String result = myService.doSomething();
assertEquals("Expected Data", result);
}
In this snippet, note how the when(...).thenReturn(...)
method is used. It tells Mockito what to return when getData()
is called on the mocked myDependency
. Forgetting to set this up can lead to NullPointerExceptions
and unclear results.
3. Not Verifying Interactions
Tests can be less effective if they solely focus on the output. It’s equally important to verify interactions with the mocks, ensuring that the expected methods are called.
Example
@Test
public void testDoSomething() {
myService.doSomething();
verify(myDependency).getData();
}
Here, the test verifies that the getData()
method was called on the myDependency
mock. Not performing such verifications can cause tests to pass when, in reality, the interactions are not as expected.
4. Over-Mocking
Over-mocking occurs when developers create too many mocks unnecessarily. This can lead to complicated test scenarios that are hard to maintain and understand.
Example
@Test
public void testComplexScenario() {
MyDependency dep1 = mock(MyDependency.class);
MyDependency dep2 = mock(MyDependency.class);
MyDependency dep3 = mock(MyDependency.class);
when(dep1.method()).thenReturn("result1");
when(dep2.method()).thenReturn("result2");
when(dep3.method()).thenReturn("result3");
// Too many mocks – leads to confusion
}
In this situation, over-mocking creates complexity and tests that are hard to follow. Focus on mocking only what is necessary for the test. Simplicity is a virtue.
5. Not Using Argument Captors
When verifying arguments passed to mocked methods, developers often forget to use Argument Captors, which can cause issues when testing interactions that involve complex parameters.
Example
import org.mockito.ArgumentCaptor;
@Test
public void testDoSomethingWithCaptor() {
myService.doSomethingWith(myDependency);
ArgumentCaptor<String> captor = ArgumentCaptor.forClass(String.class);
verify(myDependency).sendData(captor.capture());
assertEquals("Expected Data", captor.getValue());
}
Here, the ArgumentCaptor
captures the argument passed to the sendData
method, allowing you to assert the expected value. Omitting this can lead to ambiguous tests.
6. Improper Stubbing
Stubbing consists of providing mock methods with a predefined response. A common mistake is not understanding how to stub correctly, leading to confusion in unit tests.
Example
@Test
public void testDoSomething() {
when(myDependency.getData()).thenReturn("Mock Data").thenThrow(new RuntimeException());
String result = myService.doSomething();
assertEquals("Mock Data", result);
}
Here, the second call to getData()
throws an exception. This can be confusing when the test fails unexpectedly. Simplicity is key; keep stubs straightforward and focused on the test's purpose.
7. Confusing Mocking with Spy
Mixing up mocks and spies is a common error. Mocks are great for unit testing, while spies can wrap real objects but track how the tests interact with them.
Example
@Spy
private MyService myServiceSpy = new MyService(realDependency);
@Test
public void testSpy() {
myServiceSpy.doSomething();
verify(myServiceSpy).doSomething();
}
In this code, the spy allows you to verify method calls on a real object while still monitoring interactions. Misusing spies can lead to fragile tests.
8. Ignoring Mockito Version Updates
Mockito, like any other library, evolves. Failing to check for updates can lead to missed enhancements and new features that facilitate clearer testing practices.
Example
If you're still using older constructs, consider migrating to the latest version for enhanced features and improved usability. You can check the official Mockito documentation for the latest updates and best practices.
Conclusion
Mockito is a powerful tool that, when used correctly, can simplify unit testing in Java. However, as with any tool, common mistakes can lead to confusing outcomes and maintenance issues. By avoiding the pitfalls we've discussed in this post, such as improper use of @Mock
, neglecting behavior definitions, and confusing mocks with spies, you can improve your tests significantly.
Embrace the nuances, stay updated, and write clean, effective tests that provide confidence in your code’s behavior. If you're interested in more advanced Mockito topics, be sure to check out Mockito: The Guide for deeper insights and explanations.
By incorporating these strategies, you will lead your Java projects towards better testing practices, yielding cleaner code and a more robust application. Happy mocking!