Maximizing Hibernate Performance: Caching Pitfalls to Avoid
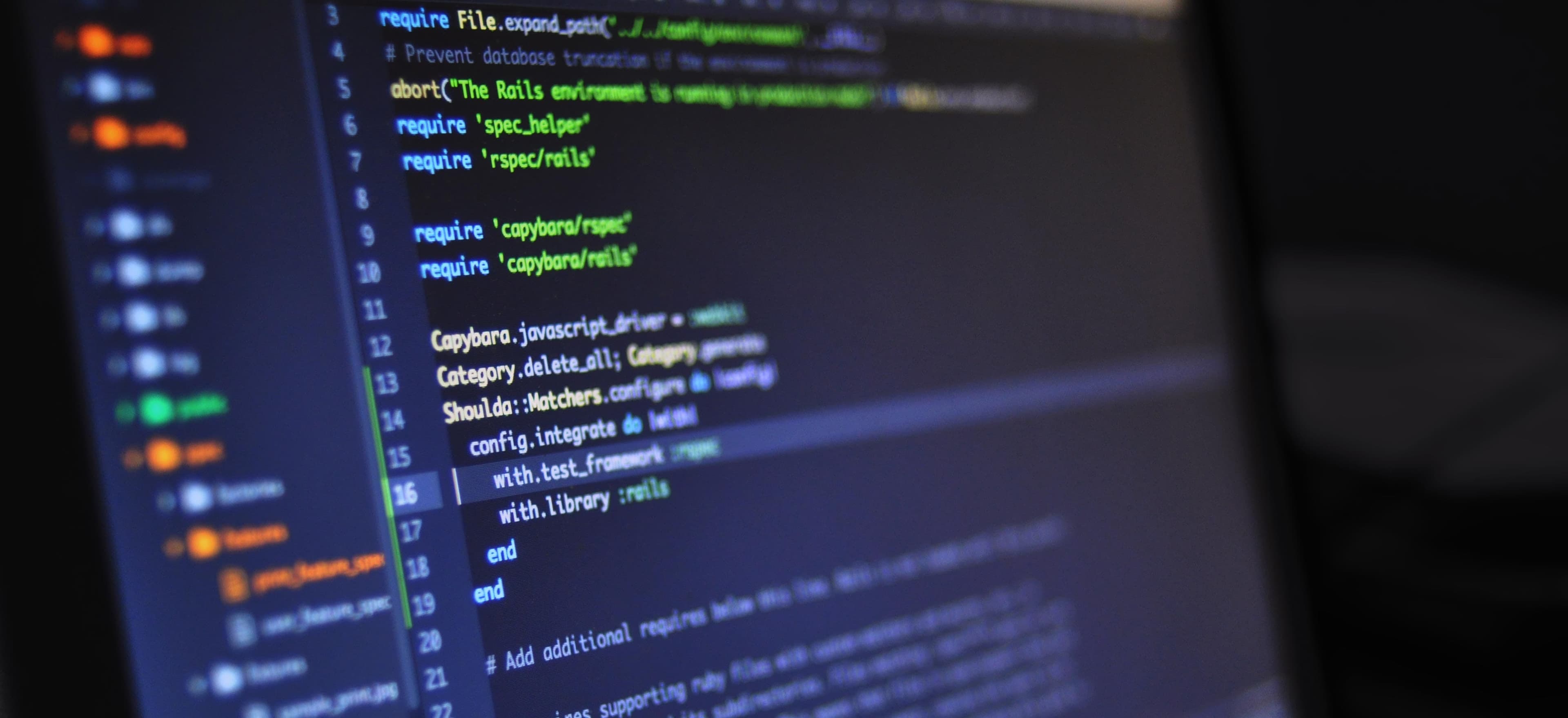
- Published on
Maximizing Hibernate Performance: Caching Pitfalls to Avoid
Hibernate is a powerful Object-Relational Mapping (ORM) framework that simplifies data handling for Java applications. While its capabilities are extensive, one aspect that significantly impacts performance is its caching mechanisms. In this post, we’ll explore the caching pitfalls to avoid, ensuring your Hibernate applications run efficiently.
Understanding Hibernate Caching
Before diving into pitfalls, let’s understand the basics of Hibernate caching. Hibernate uses caching to minimize database calls and improve application performance. It incorporates two cache levels:
-
First-Level Cache (Session Cache): This cache is associated with a session instance. Objects are stored in memory during the session's lifecycle.
-
Second-Level Cache: This is a shared cache among sessions, avoiding repetitive database access for often-retrieved entities. It is not enabled by default and requires configuration.
Understanding these layers is crucial in applying best practices and avoiding common pitfalls.
Pitfall #1: Not Utilizing the Second-Level Cache
The first mistake many developers make is neglecting to implement the second-level cache. By relying solely on the first-level cache, they miss out on opportunities to optimize.
Why Enable Second-Level Cache?
Enabling the second-level cache can yield significant performance improvements, especially for read-heavy applications. It reduces the load on the database, allowing queries to retrieve data from local memory rather than over the network.
Example Configuration:
Here’s a basic example of enabling the second-level cache using Ehcache:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
Commentary
This configuration activates the second-level cache using Ehcache as the caching provider. By doing so, more objects can be stored in memory, which enhances read access times significantly as entities previously loaded from the database can be reused across different sessions.
Pitfall #2: Misjudging Cache Regionalizing
Cache regionalizing refers to how cache data is segmented or divided. Inappropriately configured cache regions can lead to concurrent access issues, data inconsistencies, and performance bottlenecks.
Best Practices for Regionalizing Cache
-
Understand Your Data Access Patterns: Analyze how frequently data changes. Immutable objects work well in caches, while frequently changing data may not.
-
Use Appropriate Region Names: Ensure that your cache regions are named appropriately based on the entity types. This improves clarity and access speed.
Example Configuration:
<cache name="com.example.model.User" usage="read-write"/>
Commentary
By using read-write caching for the User entity, Hibernate retains the ability to maintain updated values while still delivering high performance for reading operations. Striking a balance between reading and writing helps in avoiding stale data situations.
Pitfall #3: Ignoring Cache Strategies
Various caching strategies like READ_ONLY
, READ_WRITE
, and NONSTRICT_READ_WRITE
control how data is cached. Ignoring these can quickly lead to performance drains.
Choosing the Right Strategy
-
READ_ONLY: Use when objects are never modified. Ideal for lookup data.
-
READ_WRITE: Safe for entities that are frequently read and occasionally modified.
-
NONSTRICT_READ_WRITE: Offers a performance edge with leniency on transactional guarantees.
Example Usage:
<cache name="com.example.model.Order" usage="read-write"/>
Commentary
This example establishes a read-write strategy for the Order entity, which is practical and efficient for commonly modified data. It allows Hibernate to use the cache effectively while maintaining data integrity.
Pitfall #4: Stale Data Issues
Stale data arises when cached entities do not reflect the latest changes in the database. This can occur when relying too heavily on caching without proper cache invalidation.
Solutions to Avoid Stale Data
-
Implementing a Proper Cache Invalidation Strategy: This ensures that updates in the database correctly invalidate stale cache entries.
-
Leveraging Hibernate’s Versioning Feature: By using an optimistic locking mechanism with version fields, Hibernate can automatically update or invalidate cached entries.
Example Entity Configuration:
@Entity
public class Product {
@Id
@GeneratedValue
private Long id;
@Version
private int version;
private String name;
private double price;
}
Commentary
By incorporating a version field, Hibernate automatically manages cache invalidation. If an entity is modified in a way that alters its state or version, the cache gets updated to reflect these changes. This reduces the likelihood of stale data.
Pitfall #5: Over-Caching
Caching everything can often lead to glaring issues. Excessive caching takes up memory and can degrade performance due to the complexity of managing large caches.
Best Practices for Caching
-
Cache Only What’s Necessary: Analyze and only cache data that will provide significant performance benefits.
-
Set Proper Expiration Policies: Define a reasonable expiration for cached entities to prevent old data from lingering too long.
Example Expiration Configuration:
<cache name="com.example.model.Product" usage="read-write">
<expiration strategy="expire" timeout="60000"/>
</cache>
Commentary
The above configuration sets an expiration policy for the cached Product entity. By enforcing a timeout, you ensure that stale data is systematically purged, optimizing memory use over time.
The Last Word
Maximizing Hibernate performance through effective caching practices is crucial for building highly efficient Java applications.
In this post, we discussed the most common caching pitfalls and how to avoid them:
- Enable the second-level cache for enhanced performance.
- Regionalize caches effectively based on entity access patterns.
- Select appropriate cache strategies tailored to your data's behavior.
- Implement proper invalidation mechanisms to combat stale data.
- Avoid over-caching by setting strategic limits and expiration policies.
Adopting these best practices will lead to faster database interactions, improve application responsiveness, and overall enhance user experience.
For more in-depth knowledge and strategies about Hibernate, explore Hibernate Official Documentation and Caching in Hibernate.
By understanding how caching works within Hibernate and preemptively addressing potential pitfalls, developers can harness the full potential of this powerful ORM framework. Happy coding!