Common Hibernate ORM Mistakes and How to Avoid Them
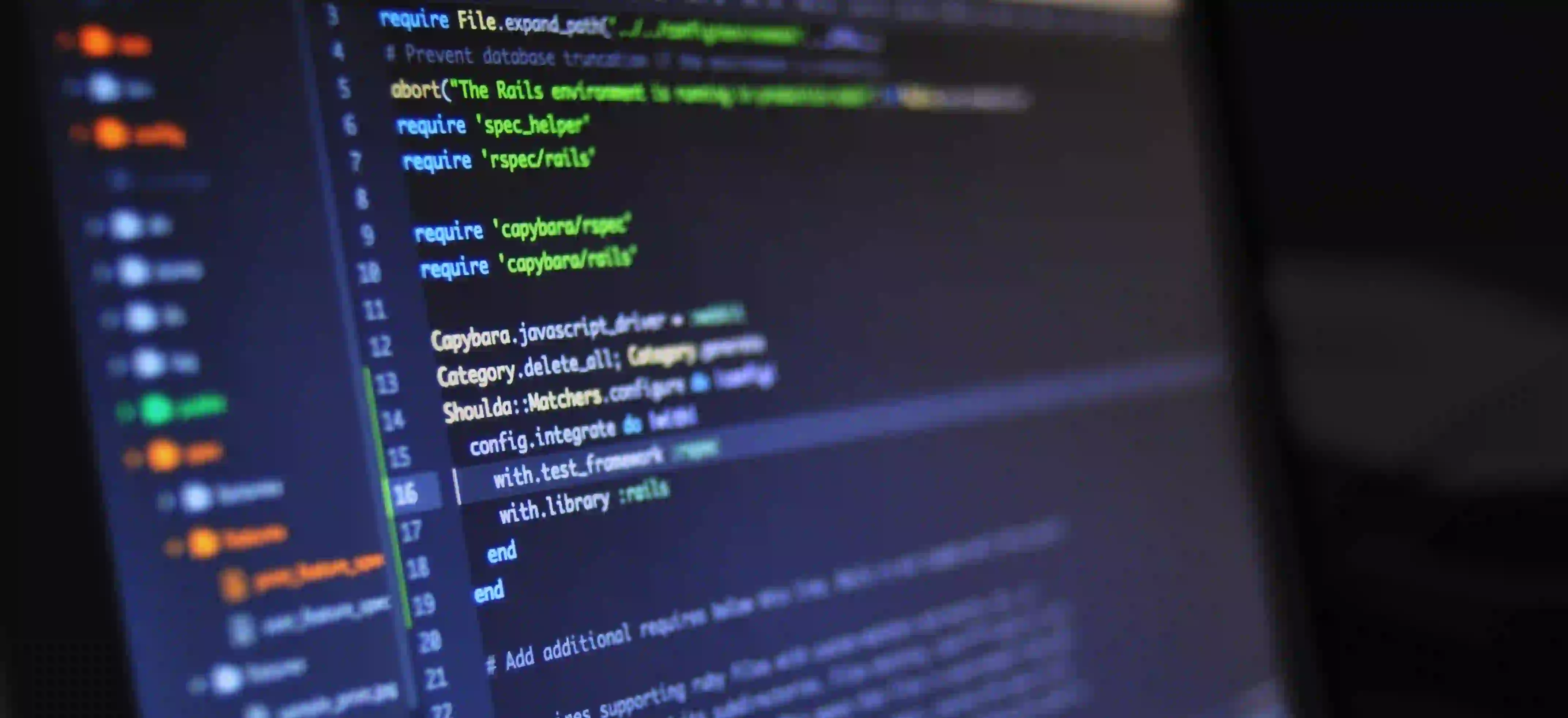
Common Hibernate ORM Mistakes and How to Avoid Them
Hibernate is a popular Object-Relational Mapping (ORM) tool for Java that streamlines database operations by allowing developers to work with Java objects instead of SQL. While it greatly enhances productivity, developers, especially those new to Hibernate, can make several common mistakes. In this post, we'll explore these pitfalls and provide practical solutions to avoid them.
1. Ignoring the Hibernate Configuration
Explanation
The first step in any Hibernate application is proper configuration. Many developers overlook the configuration files, assuming that default settings will suffice.
Common Mistake
Neglecting properties such as hibernate.dialect
, which tells Hibernate how to communicate with a specific database.
Solution
Always ensure your hibernate.cfg.xml
or the annotations in Spring are correctly set. Here is an example of a well-configured Hibernate file:
<hibernate-configuration>
<session-factory>
<property name="hibernate.dialect">org.hibernate.dialect.MySQLDialect</property>
<property name="hibernate.connection.driver_class">com.mysql.cj.jdbc.Driver</property>
<property name="hibernate.connection.url">jdbc:mysql://localhost:3306/mydb</property>
<property name="hibernate.connection.username">user</property>
<property name="hibernate.connection.password">password</property>
<property name="show_sql">true</property>
</session-factory>
</hibernate-configuration>
Why This Matters
Correct configuration enables Hibernate to interact seamlessly with your database. Always double-check your configuration for any typo or missing properties to avoid runtime errors.
2. Not Using Caching Properly
Explanation
Hibernate provides both first-level and second-level caching features to enhance performance. However, improper use of these features can lead to excessive memory consumption or stale data.
Common Mistake
Failing to understand the implications of caching, such as using the second-level cache indiscriminately without a proper cache provider.
Solution
Leverage caching but only when necessary. Here's how you can set up second-level caching with EhCache:
<property name="hibernate.cache.use_second_level_cache">true</property>
<property name="hibernate.cache.region.factory_class">org.hibernate.cache.ehcache.EhCacheRegionFactory</property>
Why This Matters
Using caching effectively can significantly increase application performance. However, it is essential to understand the life cycle of cached data to avoid stale data issues and unnecessary memory overhead.
3. Failing to Handle Lazy Initialization
Explanation
Lazy loading is one of Hibernate’s powerful features but can lead to lazy initialization exceptions if not managed properly.
Common Mistake
Accessing a lazily loaded association outside of an active session context.
Solution
Always ensure that session management aligns with data access patterns. For example, instead of directly accessing a lazy collection outside of a session, you can perform eager fetching:
List<Author> authors = session.createQuery("FROM Author a JOIN FETCH a.books", Author.class).getResultList();
Why This Matters
This prevents LazyInitializationException
, ensuring you do not attempt to access something that is no longer available. Understanding when to use eager vs. lazy fetching significantly impacts application behavior.
4. Not Handling Transactions Properly
Explanation
Transactions are critical for ensuring data integrity and consistency. Incorrect handling can lead to partial updates or inconsistencies.
Common Mistake
Executing multiple operations without a transactional context or failing to commit the transaction.
Solution
Always use a transactional context. Here is an example of proper transaction handling:
Transaction transaction = session.beginTransaction();
try {
session.save(newEntity);
transaction.commit();
} catch (Exception e) {
if (transaction != null) {
transaction.rollback();
}
e.printStackTrace();
}
Why This Matters
Properly managing transactions ensures that either all operations complete successfully, or none do, maintaining the integrity of your database.
5. Overcomplicating Entity Relationships
Explanation
Hibernate supports complex relationships like one-to-many, many-to-one, and many-to-many. However, poor structure or excessive complexity can lead to performance issues.
Common Mistake
Creating overly complex relationships and not using efficient fetching strategies can hinder performance.
Solution
Keep relationships simple and utilize joining strategies appropriately. For multi-level relationships, consider denormalization or using DTOs for specific queries.
@Entity
public class User {
@OneToMany(mappedBy="user", fetch=FetchType.LAZY)
private List<Order> orders;
}
Why This Matters
Maintaining a clean design for entity relationships directly impacts application performance and maintainability. Always evaluate the necessity of each relationship.
6. Not Utilizing HQL/Criteria Query Language
Explanation
Hibernate Query Language (HQL) provides a more object-oriented way to query entities compared to SQL.
Common Mistake
Favoring native SQL over HQL or Criteria API can lead to less maintainable code.
Solution
Embrace HQL and the Criteria API for queries. For instance, using HQL can look like this:
List<User> users = session.createQuery("FROM User WHERE status = :status", User.class)
.setParameter("status", "ACTIVE")
.getResultList();
Why This Matters
HQL and Criteria API enable you to leverage the full power of Hibernate and make your codebase cleaner and more maintainable.
Final Thoughts
Hibernate is indeed a powerful tool when utilized correctly. By understanding these common pitfalls and implementing the suggested best practices, you can avoid common mistakes that lead to performance issues and runtime errors.
Incorporating performance optimization techniques, adhering to proper configurations, managing transactions, and utilizing HQL are all part of building efficient applications with Hibernate.
For more on effective data management in Java, check Baeldung's Hibernate Guide and consider reading the official Hibernate documentation.
By recognizing these potential errors and adopting proactive solutions, you can experience the full benefits of Hibernate while building robust Java applications.