Common Spring MVC Mistakes to Avoid for Beginners
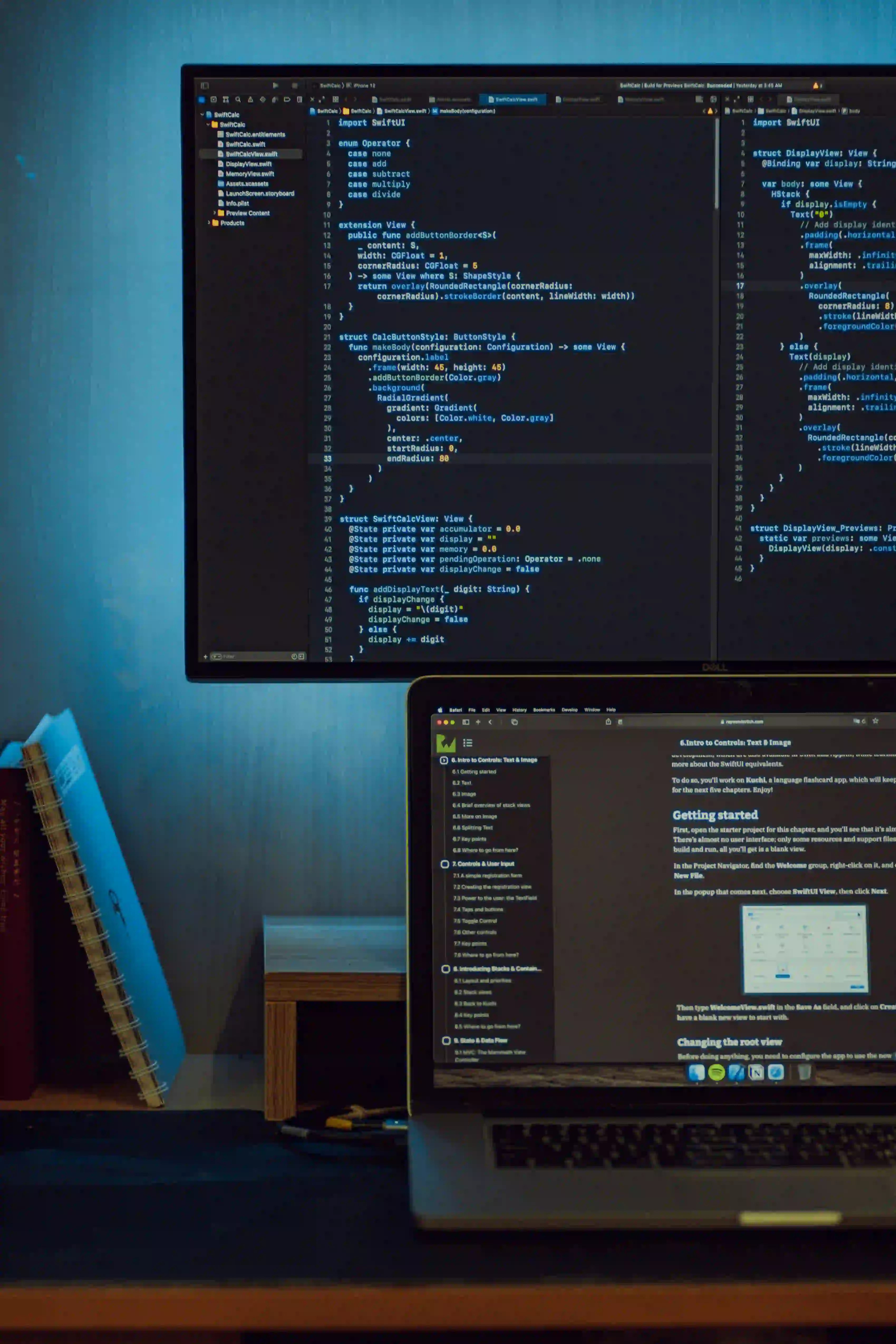
Common Spring MVC Mistakes to Avoid for Beginners
Spring MVC is a powerful framework for building web applications in Java. Its versatility, ease of use, and robust ecosystem make it a popular choice for developers at all levels. However, beginners often stumble upon common pitfalls that can lead to frustrating development experiences. In this blog post, we will discuss the common mistakes that new developers make while working with Spring MVC and how to avoid them.
1. Ignoring Spring's Dependency Injection
One of the core features of Spring is its Dependency Injection (DI) mechanism. DI makes your code more modular and testable. However, many beginners overlook its importance and tend to create rigid, tightly coupled code.
Why It Matters
Dependency Injection allows you to manage your application's dependencies effectively. It simplifies testing by enabling you to inject mock objects rather than actual services.
Example Code Snippet
@Service
public class UserService {
private final UserRepository userRepository;
@Autowired
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
// Business logic methods
}
Commentary
In the above snippet, UserService
is injecting UserRepository
via the constructor. This approach adheres to the Inversion of Control (IoC) principle, which allows Spring to manage the lifecycle of these objects. By doing this, you decouple your class from the concrete implementation of UserRepository
, making your code more testable.
2. Misusing Annotations
Spring MVC provides a wide range of annotations, but beginners often misuse them or rely on them without fully understanding their context.
Common Misused Annotations
@Controller
vs.@RestController
@GetMapping
vs.@RequestMapping
Why It Matters
Using the incorrect annotation can result in unexpected behavior or errors. Understanding what each annotation does is crucial for building efficient and maintainable applications.
Example Code Snippet
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/{id}")
public User getUser(@PathVariable Long id) {
// Return user by id
}
}
Commentary
In this example, @RestController
is used, which is a specialized version of @Controller
. It combines @Controller
and @ResponseBody
, allowing you to handle web requests and return JSON responses seamlessly. Relying on @RestController
simplifies development while providing you with the right context.
3. Not Utilizing Exception Handling
Handling exceptions gracefully is essential for a robust application. Beginners often overlook this aspect and may end up exposing internal errors to the user.
Why It Matters
Proper exception handling improves user experience and security by preventing sensitive information from being leaked.
Example Code Snippet
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(ResourceNotFoundException.class)
public ResponseEntity<String> handleResourceNotFound(ResourceNotFoundException ex) {
return ResponseEntity.status(HttpStatus.NOT_FOUND).body(ex.getMessage());
}
}
Commentary
In this snippet, we create a global exception handler using @ControllerAdvice
. The @ExceptionHandler
annotation allows the handler to catch specific exceptions across the application. This ensures that users receive a controlled error message, enhancing the application’s professional look.
4. Failing to Implement Proper Logging
Logging is vital for debugging and monitoring application behavior, yet many beginners neglect it. They often resort to using System.out.println()
for logging purposes.
Why It Matters
Effective logging helps you quickly identify issues. It provides insights into application behavior, making it easier to maintain and troubleshoot.
Example Code Snippet
@Slf4j
@Service
public class UserService {
public User findUserById(Long id) {
log.info("Finding user by id: {}", id);
// Logic to find user
}
}
Commentary
Here, we use Lombok's @Slf4j
annotation to create a logging instance. The log.info()
method allows us to log informational messages without cluttering the standard output. This practice promotes a cleaner codebase and enables better monitoring through various logging frameworks.
5. Overlooking Spring Security
Many beginners dive into building features but forget about security. This oversight can lead to vulnerabilities in the application.
Why It Matters
Security is not optional in web applications. Implementing Spring Security can help protect sensitive data and ensure that the application functions as intended.
Example Code Snippet
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/api/**").authenticated()
.and().httpBasic();
}
}
Commentary
In this configuration, we set up in-memory authentication, which is useful for testing. The HttpSecurity
configuration ensures that only authenticated users can access API endpoints. Beginners should take advantage of Spring Security to build secure applications right from the start.
You can read more about Spring Security here.
6. Not Understanding the DispatcherServlet's Role
The DispatcherServlet is a core component of Spring MVC that handles incoming requests and routes them appropriately. Many beginners do not fully understand its role, leading to complications in request handling and mapping.
Why It Matters
Understanding the DispatcherServlet's role helps you design your application better and leverage Spring MVC features more effectively.
Example Code Snippet
@Bean
public ServletRegistrationBean<DispatcherServlet> dispatcherServlet() {
ServletRegistrationBean<DispatcherServlet> registration = new ServletRegistrationBean<>(new DispatcherServlet(), "/");
registration.setName("dispatcher");
return registration;
}
Commentary
In this snippet, we define a custom DispatcherServlet
registration bean. This setup allows for more control over request handling and means that you can route all incoming requests through your DispatcherServlet, leading to a clean architecture.
7. Hardcoding Values
Beginners often hardcode values such as URLs, database credentials, and other configurations directly in the code. This practice can make the application less flexible and harder to maintain.
Why It Matters
Externalizing configurations makes the application more adaptable to different environments (development, production) and improves maintainability.
Example Code Snippet
# application.properties
app.api.base-url=http://api.example.com
Commentary
Using a properties file like application.properties
lets you manage configurations effectively. You can access these values using @Value
annotation in your services or controllers, allowing for easier updates without modifying the codebase.
@Value("${app.api.base-url}")
private String apiBaseUrl;
Key Takeaways
Avoiding these common Spring MVC mistakes can save you time, effort, and headaches in your development journey. Remember that principles like Dependency Injection, effective Logging, and Security are not just good practices but essential to creating robust and maintainable applications.
By being aware of these pitfalls and implementing the suggested practices, you are setting yourself on the path to becoming a proficient Spring MVC developer. Be sure to explore more about Spring MVC and its capabilities at the official Spring Framework documentation.
Starting out with Spring MVC can be overwhelming, but by learning from the mistakes of others, you can foster a cleaner, more effective approach to your web applications. Happy coding!