Common Pitfalls for Beginners in Spring Framework
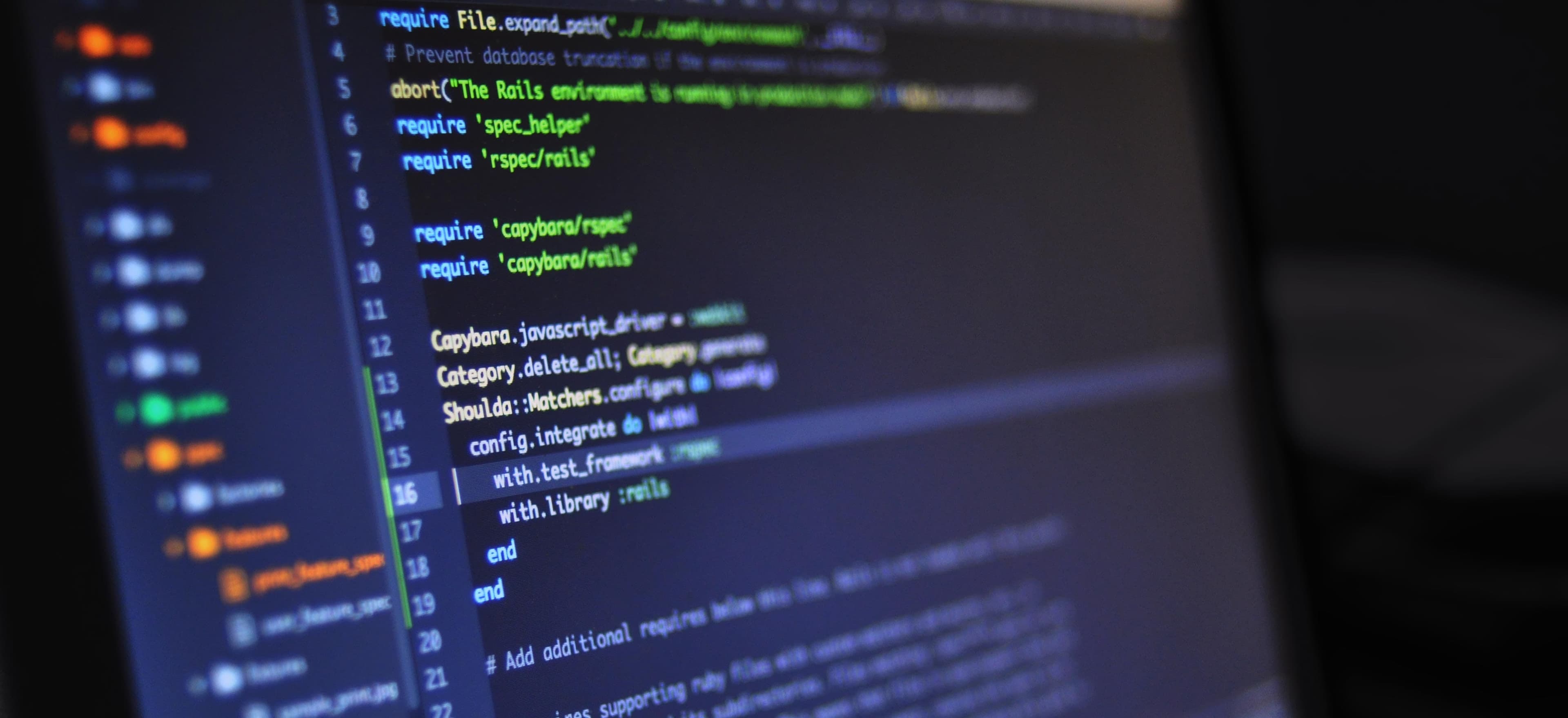
- Published on
Common Pitfalls for Beginners in Spring Framework
The Spring Framework has revolutionized the way we develop Java applications, providing a powerful ecosystem to build robust and scalable applications. However, for beginners, the journey can sometimes feel overwhelming. In this blog post, we will explore some common pitfalls that newcomers often encounter when using the Spring Framework, arming you with the knowledge to navigate these challenges effectively.
Understanding Dependency Injection
What is Dependency Injection (DI)?
At the heart of the Spring Framework lies the concept of Dependency Injection. DI enables objects to be injected into a class rather than the class creating the objects itself. This promotes loose coupling and easier unit testing.
Pitfall 1: Not Understanding Bean Scopes
Spring has several bean scopes that determine the lifecycle of a bean. The four primary scopes are:
- Singleton (default)
- Prototype
- Request (web applications)
- Session (web applications)
Why it matters:
New developers often assume that all beans are singletons. While this is the default, understanding the nuance of bean scopes is crucial for resource management and performance. Using the wrong scope can lead to unexpected behaviors and memory leaks.
Code Example:
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Component;
@Component
@Scope("prototype") // Each request gets a new instance
public class ExampleBean {
public ExampleBean() {
System.out.println("New instance created!");
}
}
Commentary:
In the above example, every time ExampleBean
is requested from the application context, a new instance will be created. This can be particularly useful for objects that maintain state or require fresh configurations.
Configuration Management
Pitfall 2: Mismanagement of Application Context
Spring manages the lifecycle of beans and their dependencies through an application context. However, beginners may misuse this feature.
Why it matters:
Using multiple application contexts can introduce complications. Creating too many contexts not only affects performance but can also lead to memory leaks.
Code Example:
import org.springframework.context.ApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
public class Main {
public static void main(String[] args) {
ApplicationContext context = new AnnotationConfigApplicationContext(AppConfig.class);
ExampleBean bean = context.getBean(ExampleBean.class);
}
}
Commentary:
In this example, we create a single ApplicationContext
using AnnotationConfigApplicationContext
. Proper management ensures that beans are reused and prevents unnecessary overhead.
Aspect-Oriented Programming (AOP)
Pitfall 3: Ignoring AOP
AOP is one of Spring’s powerful features that can help separate cross-cutting concerns (like logging and transaction management) from business logic.
Why it matters:
Beginners often overlook AOP and end up duplicating code, which is both error-prone and inefficient.
Code Example:
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore() {
System.out.println("Method is about to be invoked");
}
}
Commentary:
With the aspect defined above, every time a method in the specified package is invoked, a log message is printed automatically. This approach minimizes repetitive logging code throughout your application.
Error Handling
Pitfall 4: Not Handling Exceptions Properly
Spring’s exception handling can be complex, and not handling exceptions correctly can lead to lost information and poor user experiences.
Why it matters:
Beginners may not understand how to centralize exception handling, leading to inconsistent error responses.
Code Example:
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(NullPointerException.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
public void handleNullPointerException() {
// Log the error
}
}
Commentary:
Using @ControllerAdvice
, you can define a centralized error handling strategy for your application. This is more manageable than handling exceptions in each controller and improves maintainability.
Testing Framework
Pitfall 5: Neglecting Unit and Integration Tests
One of the main motives of using Spring is ease of testing due to DI. However, neglecting unit tests can lead to fragile codebases.
Why it matters:
Without proper tests, any changes to your application may introduce bugs. Spring offers excellent support for testing.
Code Example:
import static org.mockito.Mockito.*;
import static org.junit.Assert.*;
import org.junit.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
@SpringBootTest
public class ExampleServiceTest {
@Autowired
private ExampleService service;
@MockBean
private ExampleRepository repository;
@Test
public void testServiceMethod() {
when(repository.findSomething()).thenReturn("Mocked Response");
String response = service.methodUnderTest();
assertEquals("Mocked Response", response);
}
}
Commentary:
In the above test, we mock the ExampleRepository
to simulate database behavior, allowing us to test ExampleService
in isolation. This approach leverages Spring’s testing support to improve the stability of your application.
Closing Remarks
The Spring Framework offers immense power and flexibility, but it can also pose challenges for beginners. Understanding the core concepts of dependency injection, application context management, AOP, proper error handling, and integration testing is crucial for any developer aiming to become proficient in Spring.
Avoid these common pitfalls, and you'll be well on your way to developing successful Spring applications. For further reading, consider checking out the official Spring Documentation or diving into more advanced topics like Spring Boot.
Happy coding, and may your Spring adventures be fruitful!