Common Pitfalls When Adopting Java 11 Features
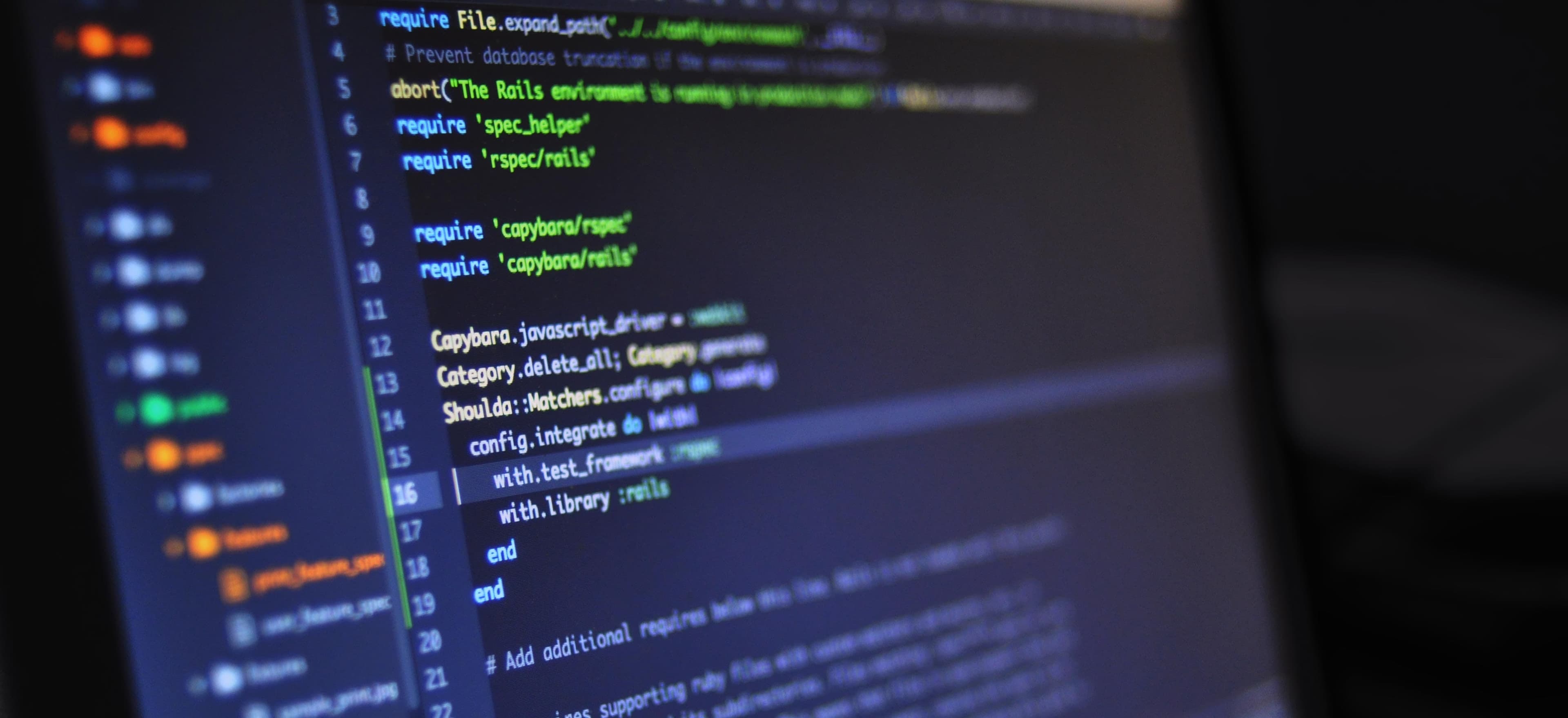
- Published on
Common Pitfalls When Adopting Java 11 Features
With the release of Java 11, many developers are eager to leverage its new features for better performance, cleaner code, and enhanced capabilities. However, transitioning to Java 11 doesn't come without its challenges. Understanding the common pitfalls associated with its features can help developers prepare and mitigate issues efficiently. In this blog, we will explore these pitfalls and provide clarity on how to avoid them.
Table of Contents
- Understanding Java 11 Features
- Pitfall #1: Overlooking Deprecated Features
- Pitfall #2: Misusing the HttpClient
- Pitfall #3: Ignoring Performance Implications
- Pitfall #4: Choosing the Wrong Module System
- Pitfall #5: Not Fully Utilizing New String Methods
- Conclusion
Understanding Java 11 Features
Java 11 introduced several new features, including the new HttpClient, var for local variables, string methods such as isBlank()
, lines()
, and many more enhancements that improve productivity. Knowing these features is just the first step, but we will focus on common pitfalls developers encounter when adopting them.
Pitfall #1: Overlooking Deprecated Features
One of the first hurdles in migrating to Java 11 is dealing with deprecated features. While Java maintains backward compatibility, relying on deprecated methods can lead to broken applications down the road.
Example:
import java.util.Collections;
public class Main {
public static void main(String[] args) {
// Deprecated method:
// Collections.enumeration(List.of("a", "b", "c")).asIterator();
// More modern approach:
var iterator = List.of("a", "b", "c").iterator();
while (iterator.hasNext()) {
System.out.println(iterator.next());
}
}
}
Why This Matters: The old way using Collections.enumeration()
is not only inconvenient but could lead to broken functionality as updates progress. Always check the Java 11 JDK documentation for deprecated methods.
Pitfall #2: Misusing the HttpClient
The introduction of the new HttpClient
API makes it easier to perform HTTP requests, but its asynchronous capabilities can confuse developers accustomed to synchronous code.
Example:
import java.net.http.HttpClient;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
public class HttpExample {
public static void main(String[] args) {
HttpClient client = HttpClient.newHttpClient();
HttpRequest request = HttpRequest.newBuilder()
.uri(URI.create("https://api.github.com"))
.build();
client.sendAsync(request, HttpResponse.BodyHandlers.ofString())
.thenApply(HttpResponse::body)
.thenAccept(System.out::println) // Handle response here
.join(); // Wait for completion
}
}
Why This Matters: Developers sometimes forget that sendAsync
returns a CompletableFuture
, which runs in the background. Not using .join()
or .get()
could lead to unpredictable program flow. Familiarize yourself with CompletableFutures to mitigate such issues.
Pitfall #3: Ignoring Performance Implications
Java 11 brought various performance improvements, but there are also situations where performance can degrade when features are misused. For instance, using the var
keyword excessively may lead to unoptimized code insights later on.
Example:
public class PerformanceExample {
public static void main(String[] args) {
var list = List.of(1, 2, 3, 4, 5);
// Instead of this inefficient stream operation:
var squared = list.stream()
.map(i -> i * i)
.collect(Collectors.toList());
// Prefer direct iteration for performance-sensitive operations
for (var i : list) {
System.out.println(i * i);
}
}
}
Why This Matters: Streams provide convenience but can introduce overhead. Dive deeper into Java Streams to understand better performance implications and when to use them effectively.
Pitfall #4: Choosing the Wrong Module System
Java 11 promotes a modular approach, allowing developers to encapsulate code. However, mistakes during the setup can lead to significant issues.
Example:
A developer might set up a project in a modular fashion but fail to define module dependencies correctly.
- Ensure that a module-info.java is defined correctly in each module.
- Use the
requires
keyword appropriately.
module app {
requires utils; // Ensure this matches the defined module name in the utils project
}
Why This Matters: Mismanagement of modules can lead to class-not-found exceptions or runtime errors. Read more about the Java Platform Module System for guidance.
Pitfall #5: Not Fully Utilizing New String Methods
Java 11 introduced several new string methods, but many developers underestimate their utility and stick with older implementations.
Example:
public class StringExample {
public static void main(String[] args) {
String multilineString = "Line 1\nLine 2\nLine 3";
// Instead of using older methods, leverage newer features:
multilineString.lines().forEach(System.out::println);
// Check for blank:
String empty = " ";
System.out.println(empty.isBlank()); // true
}
}
Why This Matters: By being unaware of new methods such as lines()
and isBlank()
, developers miss an opportunity for cleaner and more efficient code. For an in-depth look at new string methods, read the Java 11 Release Notes.
Key Takeaways
Java 11 offers a myriad of features designed to enhance developer experience and application performance. However, developers must tread carefully during the adoption process to avoid pitfalls that can hinder these advantages.
By understanding the nuances of deprecated features, effectively using the new HttpClient
, making performance-conscious choices, managing the module system correctly, and using the latest string methods, you can maximize your productivity in Java 11.
As the software landscape evolves, embracing changes in a structured way will pave the path for successful projects. Remember to assess your codebase continually and refer to the official Java documentation for the latest practices and recommendations.
By avoiding these common pitfalls, you can ensure a successful migration to Java 11 and harness its powerful features effectively. Happy coding!
Checkout our other articles