Navigating the Pitfalls of Java 10's Type Inference
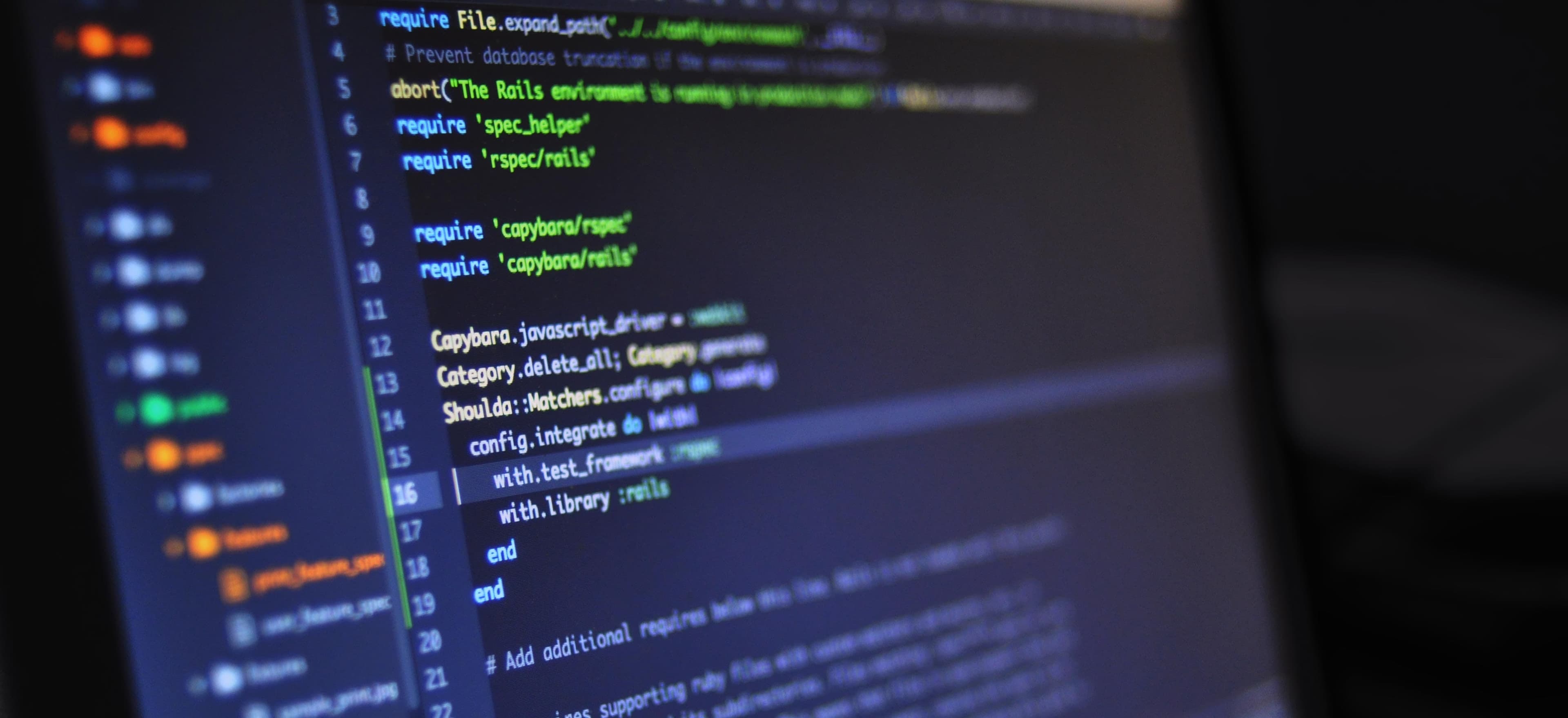
- Published on
Navigating the Pitfalls of Java 10's Type Inference
Java has always been a language that encourages type safety and clarity, but with the introduction of type inference in Java 10, developers have seen both a boon and a bane. This blog post takes you through understanding the intricacies of type inference, its advantages, its pitfalls, and how to navigate them effectively.
What is Type Inference?
Type inference is the ability of the compiler to determine the type of a variable automatically. In earlier versions of Java, developers had to declare types explicitly. However, with the introduction of var
in Java 10, this changed. Java can now infer types based on the context.
Here’s a simple example:
var message = "Hello, World!";
In this case, the compiler infers that message
is a String
. This can reduce boilerplate code and increase readability, especially in complex expressions.
The Benefits of Type Inference
- Conciseness: By eliminating the need to declare types explicitly, developers can write less code. This is especially useful for long generic types.
Example:
List<String> names = new ArrayList<>();
var names = new ArrayList<String>();
-
Readability: While explicit type declarations are often clearer, inferred types can improve readability in certain contexts, especially for anonymous classes.
-
Maintenance: Changes to a type require fewer updates throughout your codebase when using
var
, thereby easing maintenance.
Despite these advantages, there are potential pitfalls that developers must be aware of.
The Pitfalls of Type Inference
1. Loss of Explicitness
While var
makes your code shorter, it can also lead to decreased readability. The type of a variable may not be immediately clear, especially in complex expressions.
var result = performComplexCalculation();
What type is result
? A developer reading this code snippet would need to look up performComplexCalculation()
to understand the type being used.
2. May Lead to Unintended Types
Consider the following example:
var list = new ArrayList<>();
What type is list
? It’s an ArrayList<Object>
. This may not be what you intended. With generics being a first-class citizen in Java, relying on inference here can lead to mixing types unexpectedly.
3. Restrained Usage of var
There are certain constraints where var
cannot be used:
- Local variables only.
- It cannot be used for method parameters, return types, or instance variables.
- It cannot be inferred from
null
.
Here’s an example that illustrates this:
var a; // Compilation error: cannot use var without initialization.
4. Loss of Type Information
In code bases where readability and type safety are paramount, using var
might erase necessary information. This is particularly poignant when it comes to generics.
var map = new HashMap<>(); // What does this hold?
It becomes imperative to strike a balance between brevity and clarity.
Best Practices for Using Type Inference
1. Use var
When It Enhances Readability
The primary rule of thumb is to use var
when it enhances readability, particularly for lengthy or complex types:
var longMap = new HashMap<String, List<Integer>>();
This allows you to skip repetitive type specifications.
2. Keep the Context in Mind
Always consider the context. If the type is not immediately clear from the code, it might be better to stick to explicit types:
var userList = fetchUsers(); // Fetching may return User or List<User>
Instead, specify it:
List<User> userList = fetchUsers();
3. Avoid Overuse
Java's type inference should not be an excuse for a lack of clarity. Avoid using var
excessively, particularly in public APIs where clarity is crucial.
4. Annotate Complex Types
For complex types, it may be beneficial to annotate them for future readers or even for yourself:
var complexDataFunc = (Map<String, List<Integer>> data) -> { /* logic */ };
Adding comments or documentation will help clarify the intent.
My Closing Thoughts on the Matter
Type inference in Java 10 is a powerful feature, but it is not without its pitfalls. Balancing the benefits of concise code with the imperative need for clarity should direct your decision-making.
As a developer, you should assess each situation individually—where is var
genuinely enhancing your code, and where does it obscure meaning? The swiftness of modern Java development shouldn’t come at the expense of maintainability and readable code.
For further reading, you might want to check:
By remaining mindful of these insights and adopting best practices, you’ll find that navigating Java's type inference can be an advantageous aspect of your coding toolkit rather than a hurdle. Happy coding!
Checkout our other articles